Managing Your IDE Project Files: Eliminate the Clutter!
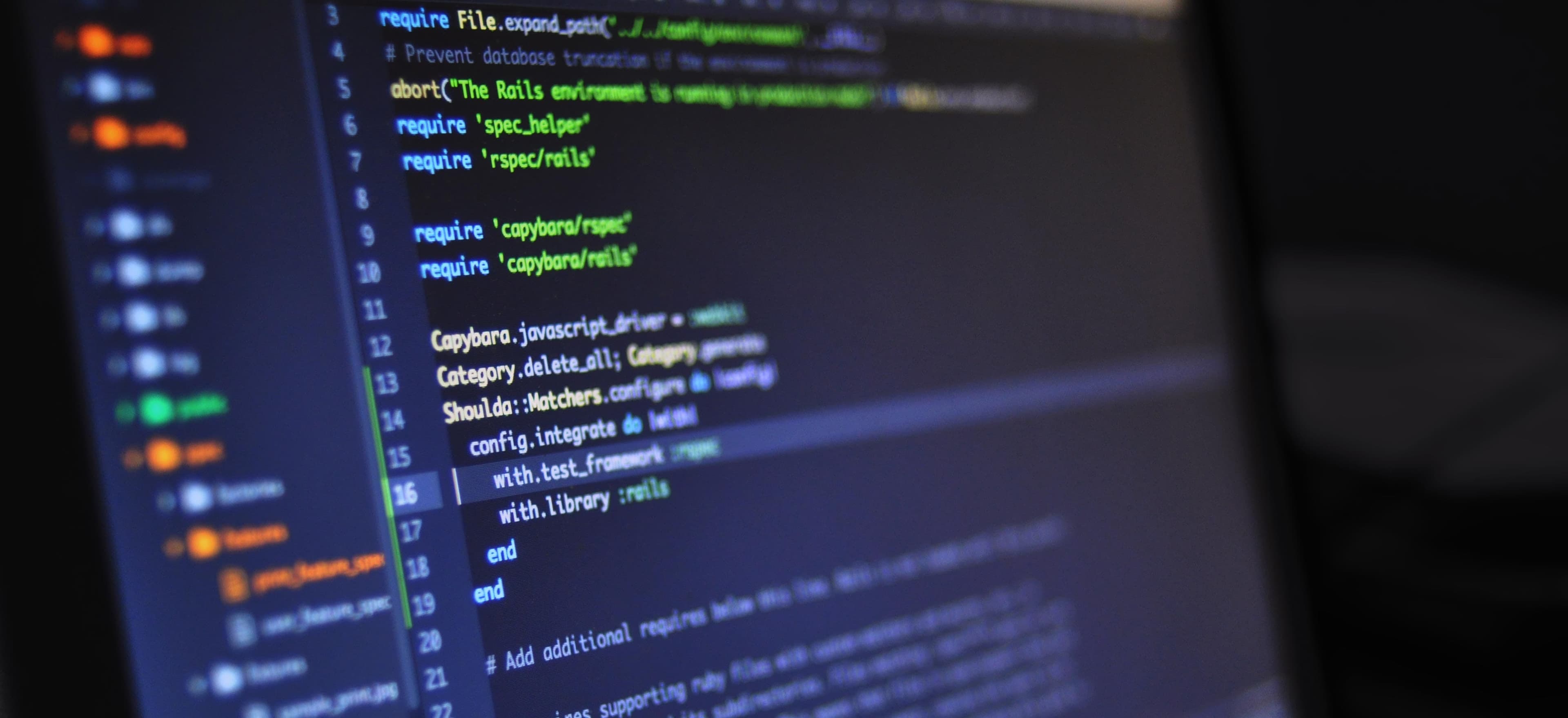
- Published on
Managing Your IDE Project Files: Eliminate the Clutter!
In the coding world, particularly when using Java, an Integrated Development Environment (IDE) becomes an essential tool. IDEs like IntelliJ IDEA, Eclipse, and NetBeans simplify the development process, but over time, managing project files can become overwhelming. This blog post will guide you on managing your Java project files effectively, maintaining organization, improving efficiency, and, most importantly, eliminating clutter.
Why File Management Matters
File management is not just about aesthetics; it directly impacts productivity. A cluttered workspace can slow down your development process and introduce unnecessary confusion. Here are a few reasons why you should prioritize file management:
- Increased Efficiency: Finding files quickly boosts your productivity.
- Reduced Errors: Organized files reduce the risk of editing the wrong file or having outdated resources in your project.
- Collaboration-Friendly: Clear file structures facilitate teamwork and make it easier for new developers to understand your project.
Establish a Consistent Folder Structure
The backbone of any well-managed project is a consistent folder structure. Here is a standard structure suitable for Java projects:
YourProject/
├── src/
│ ├── main/
│ │ ├── java/
│ │ └── resources/
│ ├── test/
│ │ ├── java/
│ │ └── resources/
├── lib/
├── bin/
└── docs/
Explanation:
src/main/java
: This directory contains all your Java source files.src/main/resources
: Non-Java resources like properties files or configuration files.src/test/java
: Test files for unit testing your application.lib
: For external libraries or dependencies.bin
: Compiled classes and binaries.docs
: Documentation of the project.
A clean and clear structure like this makes it easy for you and your collaborators to navigate the project.
Using Naming Conventions
Consistent naming conventions further enhance organization. Here are some tips:
- Camel Case for Classes: Use CamelCase for class names, e.g.,
UserProfile
. - Lowercase for Packages: Always use lowercase for package names, e.g.,
com.example.myapp
. - Self-Explanatory Filenames: Choose filenames that indicate their purpose, e.g.,
UserService.java
instead ofService.java
.
By adhering to a consistent naming standard, you create a predictable environment that aids in understanding the project at a glance.
Version Control: The Lifesaver
A version control system, such as Git, is a powerful tool that not only helps track changes but also assists in clutter management. Here’s why you should use version control:
- Change Tracking: Easily see what has changed over time.
- Collaboration: Multiple developers can work on the same files without overwriting changes.
- Branching and Merging: Experiment with new features without affecting the main codebase.
To get started, here’s a simple Git command sequence to initialize a repository:
# Navigate to your project folder
cd YourProject
# Initialize a Git repository
git init
# Add all files to the staging area
git add .
# Commit the files
git commit -m "Initial commit"
By using Git, you can not only manage file changes but also keep your project directory clear by ignoring unnecessary files with a .gitignore
file. You can include entries in .gitignore
such as:
/bin/
target/
*.class
*.log
Optimize Your Build Process
Build tools like Maven or Gradle can help manage dependencies and create a cleaner build process. Both tools facilitate efficient project management by segregating libraries and build scripts from your code, thus reducing clutter.
For instance, if you’re using Maven, your pom.xml
file will handle all dependencies:
<dependencies>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-core</artifactId>
<version>5.3.9</version>
</dependency>
</dependencies>
Why Maven?
Using a build tool like Maven:
- Automates Dependency Management: Automatically fetches and manages different versions of libraries.
- Standardizes Builds: Ensures that all team members are running the same build setup.
This leads to a more organized and manageable workspace.
Keep Your Code Clean and Refactored
Regularly refactoring your codebase is essential for managing clutter within your project files. Code that is clean and well-organized not only improves readability but also reduces technical debt.
Example of Refactoring:
Let’s look at a simple refactoring example for a user service:
Before refactoring:
public class UserService {
public void createUser(String name, String email) {
// Create user logic
}
public void deleteUser(String id) {
// Delete user logic
}
}
After refactoring:
public class UserService {
private UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
public void createUser(User user) {
validateUser(user);
userRepository.save(user);
}
public void deleteUser(User user) {
userRepository.delete(user);
}
private void validateUser(User user) {
// Validation logic
}
}
Why Refactor?
- Improves Readability: The purpose and logic are clearer.
- Encourages Reusability: Can be leveraged in other parts of the application.
- Enhances Maintainability: Easier to manage and modify in the future.
By keeping your code clean, you significantly declutter your project.
Utilizing IDE Features for Clutter Reduction
Most modern IDEs have built-in features that can help manage your project's files effectively:
- Code Cleanup Tools: Tools like IntelliJ IDEA offer code cleaning and analyzing features.
- File Search and Navigation: Use shortcut keys (like Ctrl + N in IntelliJ IDEA) to find files quickly.
- Refactoring Tools: Built-in refactoring capabilities assist in the rearrangement of your code without manual changes.
Wrapping Up
Managing your IDE project files doesn’t have to be a daunting task. By establishing a consistent folder structure, adhering to naming conventions, leveraging version control, optimizing your build process, maintaining clean code, and utilizing IDE features, you can eliminate clutter and create an efficient workspace.
Further resources to explore include Java Project Structure and Effective Java. By engaging with these materials, you'll deepen your understanding of effective project management and file organization.
Start implementing these practices today, and enjoy a clutter-free, streamlined development experience. Happy coding!