5 Common Pitfalls in Code Reviews and How to Avoid Them
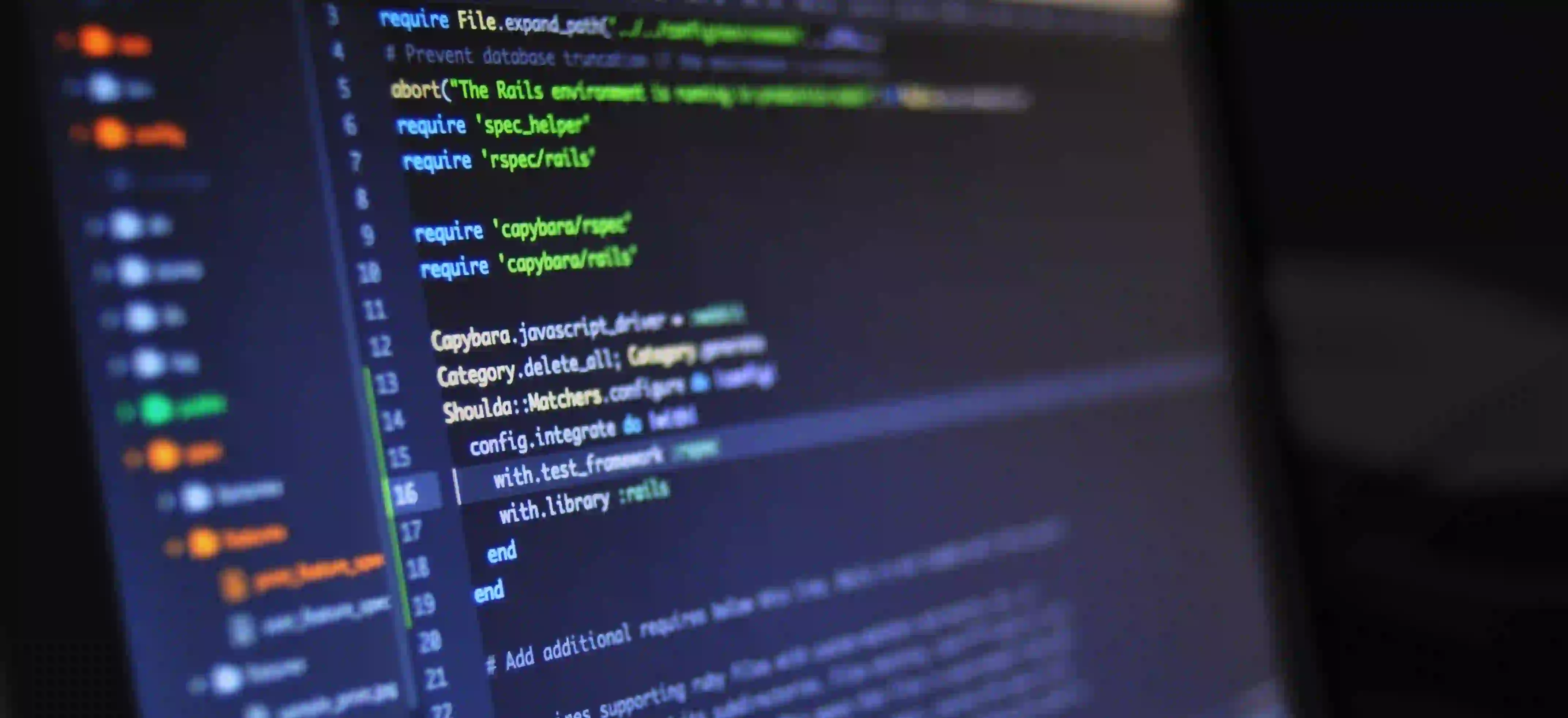
5 Common Pitfalls in Code Reviews and How to Avoid Them
Code reviews are a vital part of the software development process. They create opportunities for knowledge sharing, quality improvement, and collaboration among team members. However, they can also lead to confusion and frustration, particularly when certain common pitfalls arise. In this blog post, we'll explore five common pitfalls in code reviews and offer practical advice on how to avoid them.
1. Vague Feedback
The Issue
Vague feedback can lead to misunderstandings and a lack of direction for the developer. Comments like “This could be better” or “Consider refactoring” don’t provide clear guidance on what needs to change.
The Solution
Be specific in your feedback. Instead of vague remarks, provide concrete suggestions. For example:
Before:
// Consider optimizing this code
After:
// This for-loop can be optimized by using Streams for better readability.
List<String> names = new ArrayList<>();
for (User user : users) {
names.add(user.getName());
}
// Suggested Change
List<String> names = users.stream()
.map(User::getName)
.collect(Collectors.toList());
Why This Matters
Specific feedback helps developers understand the reasoning behind your suggestions, leading to improved learning and code quality.
2. Focusing Solely on Style
The Issue
While code styling is important, nitpicking minor stylistic choices can waste valuable time. Excessive focus on style can detract from more substantive issues like logic errors or security vulnerabilities.
The Solution
Establish a consistent style guide for the team. Tools like Checkstyle or Spotless can help automate style checks. This allows code reviews to focus on more critical aspects.
For example, instead of commenting on bracket placement, encourage developers to use the formatter configured in your IDE.
// No need to comment on this
if (condition) {
doSomething();
}
// Instead, focus on why the logic might not be clear
if (isUserActive(user)) {
notifyUser(user);
}
Why This Matters
By minimizing minor disputes over style, you can devote more time to critical issues, enhancing the overall quality of the codebase.
3. Ignoring Edge Cases
The Issue
Code that performs well under normal conditions may fail under edge cases. Ignoring these scenarios can lead to bugs that are harder to trace down the line.
The Solution
Encourage reviewers to think critically about edge cases. Ask questions such as, “What happens if the input is null?” or “How does this code behave in extreme conditions?”
Here is an example of handling an edge case in a Java method:
Before:
public int divide(int a, int b) {
return a / b; // Potential Division by Zero Exception
}
After:
public int divide(int a, int b) {
if (b == 0) {
throw new IllegalArgumentException("Divider cannot be zero");
}
return a / b;
}
Why This Matters
Identifying and handling edge cases upfront can save time fixing critical issues that arise during later stages of development or in production.
4. Rushing Through Reviews
The Issue
Time constraints can pressure reviewers to rush through the process, missing important considerations and leaving critical comments unmade. This can lead to subpar code getting approved.
The Solution
Set realistic timelines for code reviews. Consider dedicating specific blocks of time for reviews to ensure they are thorough and meaningful. Here are some best practices:
- Limit Pull Request Size: Smaller, focused pull requests are easier and quicker to review.
- Establish Review Deadlines: Set deadlines that encourage quality over speed.
Why This Matters
A well-paced review process fosters higher-quality feedback, leading to a more robust codebase and a learning environment for everyone involved.
5. Assuming Context
The Issue
Assuming that others are aware of the context behind a code change may lead to unnecessary confusion. Without proper context, valuable comments may go unmade.
The Solution
Always include context when submitting code for review. This could be a clear description of what the code does, why the change was made, and any relevant links or documentation.
For instance:
// This code adds validation to user input for the registration form
// Relevant Ticket: XYZ-123
public void registerUser(User user) {
if (user.getName() == null || user.getEmail() == null) {
throw new InvalidUserException("Name and Email are required");
}
// Continue Registration
}
Why This Matters
Clear context helps reviewers understand your intentions, ensuring they can contribute constructively to the discussion.
Wrapping Up
Code reviews can significantly enhance the quality of your software, boost team collaboration, and facilitate knowledge sharing. By avoiding these common pitfalls, you can make the code review process smoother and more effective.
Remember, the goal is not to critique for the sake of critiquing but to create an environment that fosters improvement and teamwork. Consider implementing the strategies discussed here in your next code review.
For further reading on this topic, check out The Code Review Checklist or Best Practices for Code Reviews.
Happy coding!