Common Pitfalls to Avoid in Java EE 7 Development
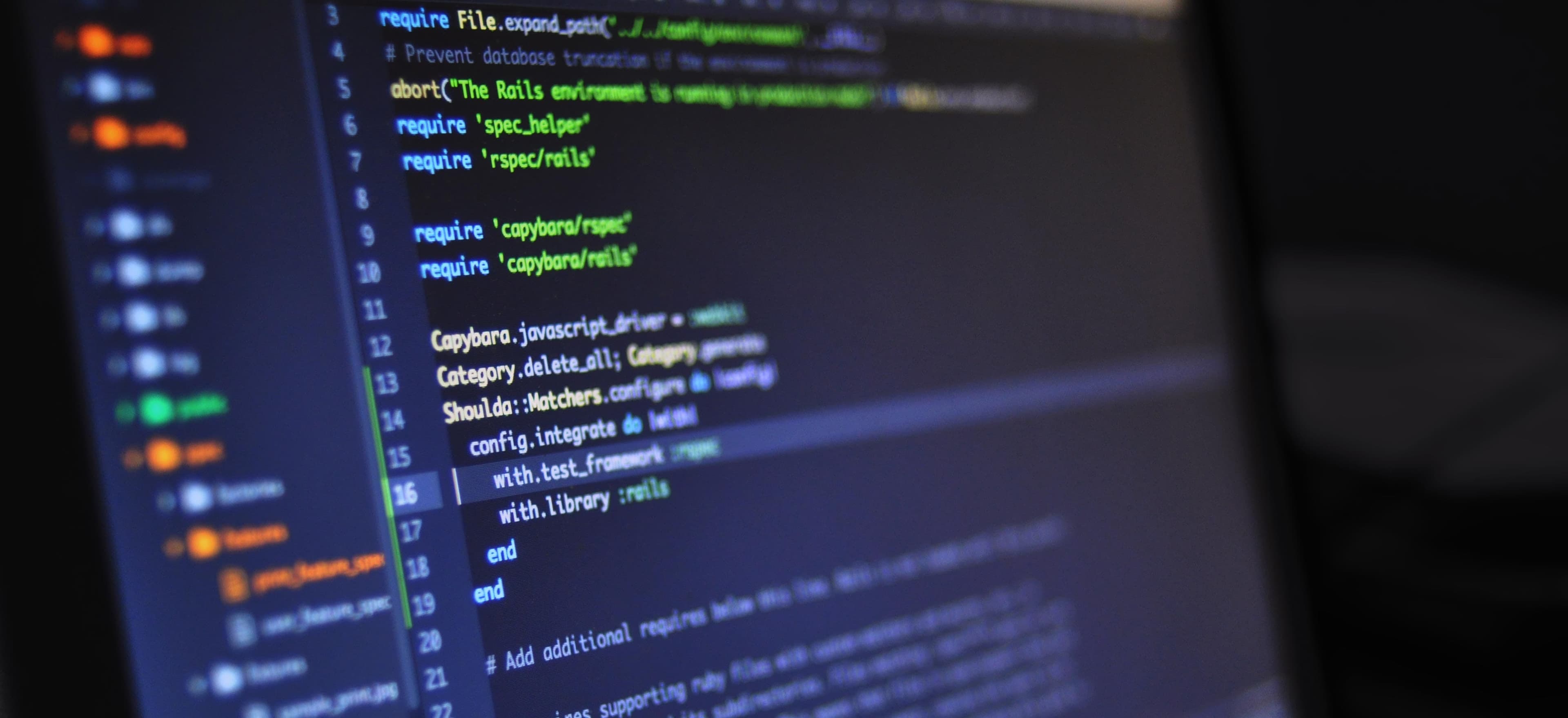
- Published on
Common Pitfalls to Avoid in Java EE 7 Development
Java EE (now Jakarta EE) has simplified enterprise application development by providing a robust platform. However, even experienced developers can stumble into common pitfalls. Here's a guide to help you avoid these issues and develop more efficient and maintainable Java EE applications.
Table of Contents
- Java EE Overview
- Pitfall 1: Ignoring Dependency Injection
- Pitfall 2: Overcomplicating Configuration
- Pitfall 3: Misunderstanding Transaction Management
- Pitfall 4: Not Leveraging JPA Effectively
- Pitfall 5: Poor Exception Handling
- Conclusion
Java EE Overview
Java EE 7 brings a suite of features aimed at simplifying large-scale enterprise applications. With advancements such as HTML5 support, improved batch processing, and RESTful web services, Java EE 7 encourages developers to build scalable applications.
However, to harness these features effectively, developers must remain vigilant about common pitfalls that could hinder software quality. Let's dive deeper into these challenges.
Pitfall 1: Ignoring Dependency Injection
The Problem
Many Java developers come from a traditional Java SE background, where manual instantiation is common. In Java EE, managed beans and dependency injection (DI) are essential.
Why It Matters
By leveraging DI, you enhance code readability and reduce dependencies among your classes, which promotes better software design.
Example Code
Consider this simple class without DI:
public class UserService {
private UserRepository userRepository;
public UserService() {
this.userRepository = new UserRepository(); // Manual instantiation
}
}
The class above tightly couples UserService
with UserRepository
. Here's how to utilize @Inject
:
import javax.inject.Inject;
public class UserService {
@Inject
private UserRepository userRepository; // Managed by the container
public void someMethod() {
// Use userRepository
}
}
Bringing It All Together
By injecting dependencies like this, you promote flexibility and testability in your application. Be sure to use DI where suitable.
Pitfall 2: Overcomplicating Configuration
The Problem
Java EE offers many configurations. Developers sometimes over-complicate their environments—leading to difficult maintenance and troubleshooting.
Why It Matters
Simple configurations are easier to manage, and they lead to faster development workflows.
Example Configuration
Instead of using multiple XML configuration files, consider using annotations along with a single persistence.xml
for JPA:
<persistence xmlns="http://xmlns.jcp.org/xml/ns/persistence" version="2.1">
<persistence-unit name="examplePU">
<provider>org.hibernate.jpa.HibernatePersistenceProvider</provider>
<class>com.example.model.User</class>
<properties>
<property name="javax.persistence.jdbc.url" value="jdbc:database_url"/>
<property name="javax.persistence.jdbc.user" value="username"/>
<property name="javax.persistence.jdbc.password" value="password"/>
</properties>
</persistence-unit>
</persistence>
Bringing It All Together
Focus on using annotations and minimizing XML files to streamline your configuration setup.
Pitfall 3: Misunderstanding Transaction Management
The Problem
Transaction management can be complex. Many developers mismanage transactions, leading to data inconsistencies.
Why It Matters
Proper transaction handling ensures data integrity and application stability.
Example Code
Make use of the @Transactional
annotation or programmatic transaction management as shown:
import javax.ejb.Stateless;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
// Using EJB to manage transactions
@Stateless
public class UserService {
@PersistenceContext
private EntityManager entityManager;
public void createUser(User user) {
entityManager.persist(user); // Automatically transaction-managed
}
}
Bringing It All Together
Understand the transaction context provided by Java EE. Using annotations simplifies management and ensures that your application behaves predictably.
Pitfall 4: Not Leveraging JPA Effectively
The Problem
Some developers approach JPA with a limited mindset, often resorting to entities without mastering the associated features.
Why It Matters
JPA offers powerful capabilities for managing data. Misunderstanding it can lead to performance issues and poor application design.
Example Code
Using lazy loading effectively:
@Entity
public class Course {
@OneToMany(mappedBy = "course", fetch = FetchType.LAZY)
private List<Student> students = new ArrayList<>();
}
By using FetchType.LAZY
, you ensure that students are only loaded when accessed.
Bringing It All Together
Master JPA and its configurations to ensure your application runs efficiently.
Pitfall 5: Poor Exception Handling
The Problem
Another common pitfall is neglecting proper exception handling, both at the business logic level and presentation layer.
Why It Matters
Handling exceptions appropriately can enhance user experience and help debug issues effectively.
Example Code
Using @ApplicationException
can assist in defining custom application-level exceptions.
import javax.ejb.ApplicationException;
@ApplicationException
public class UserNotFoundException extends RuntimeException {
public UserNotFoundException(String message) {
super(message);
}
}
Bringing It All Together
Take exceptions seriously. Create custom exceptions to enhance the user experience and provide clear feedback on issues.
Bringing It All Together
Avoiding pitfalls in Java EE 7 development is essential for creating robust applications. By understanding the role of dependency injection, simplifying configurations, properly managing transactions, leveraging JPA effectively, and handling exceptions correctly, developers can build scalable and maintainable enterprise applications.
For further insights, refer to the official Jakarta EE documentation. Embrace the best practices discussed here, and you'll find yourself building successful Java EE applications with confidence. Happy coding!