Choosing Between Identity and Sequence Generators in Hibernate
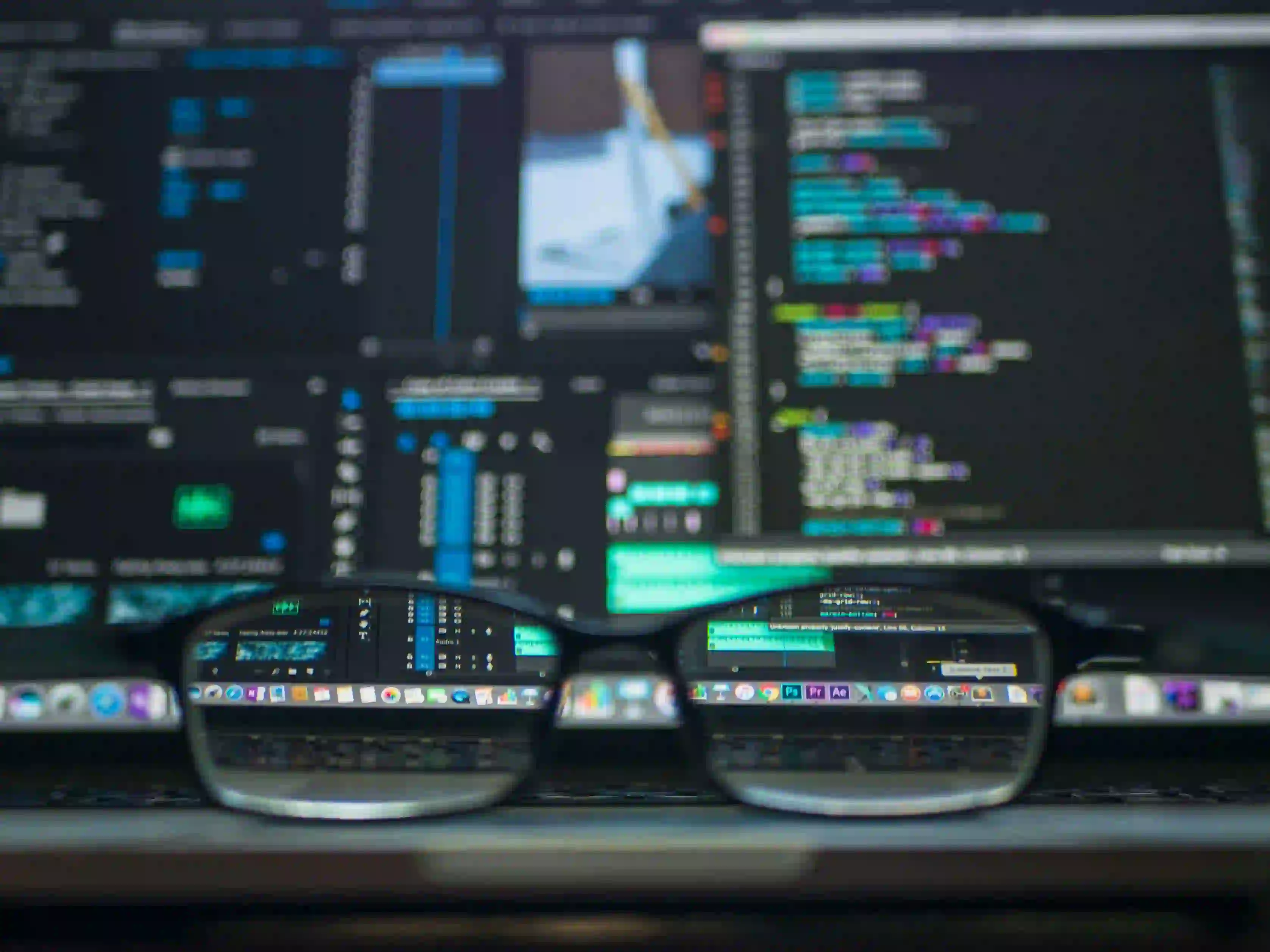
Choosing Between Identity and Sequence Generators in Hibernate
In the world of Java programming, particularly when employing the Hibernate framework for object-relational mapping (ORM), one key decision developers face is the choice of primary key generation strategy. Two popular approaches to this are Identity and Sequence generators. Choosing the right generator can have significant implications for performance, portability, and overall data integrity. This blog post will delve into these two strategies, their use cases, and how to implement them effectively.
Understanding Identity and Sequence Generators
Hibernate offers various strategies for primary key generation:
-
Identity Generation: This approach relies on the database to generate primary key values automatically. When a new record is inserted, the database generates a unique identifier for it, which is typically an auto-incrementing integer.
-
Sequence Generation: This method works by utilizing a database sequence. A sequence is a database object that generates a sequence of unique integers. This generation is usually handled more flexibly and can be managed outside of the actual insert command.
When to Use Identity Generator
The identity generation strategy is often suitable in scenarios where:
- The underlying database supports identity columns (e.g., MySQL, SQL Server).
- Simplicity is a top priority, especially in smaller applications.
Implementing Identity Generation
To implement an Identity generator in Hibernate, annotate your entity like so:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String username;
// Getters and Setters
}
Advantages of Identity Generator
- Simplicity: Configuration is straightforward, requiring minimal setup.
- No additional database object: It eliminates the need to manage separate sequences.
Disadvantages of Identity Generator
- Portability Issues: If you switch databases, you may run into compatibility issues because not all databases support identity generation.
- Limited Flexibility: If you need to manage key generation more finely, identity columns can sometimes be restrictive.
When to Use Sequence Generator
In contrast, Sequence generators are suitable when:
- Your application has a high volume of inserts and requires better performance.
- You are using databases like Oracle that leverage sequences efficiently.
- You need cross-database compatibility and scalability.
Implementing Sequence Generation
To implement a Sequence generator, you can modify your entity as follows:
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.SEQUENCE, generator = "user_seq")
@SequenceGenerator(name = "user_seq", sequenceName = "user_sequence", allocationSize = 1)
private Long id;
private String username;
// Getters and Setters
}
Advantages of Sequence Generator
- Performance: Batch inserts can benefit significantly from using sequences, which can reduce round trips to the database.
- Portability: Sequences can be more easily adapted to different database systems.
- Flexibility: Allows for pre-fetching IDs, which can enhance performance in high-volume scenarios.
Disadvantages of Sequence Generator
- Complexity: The setup process is more involved than simply using identity columns.
- Database Dependency: You need to manage the sequence explicitly, which means additional overhead.
Performance Considerations
Selecting between Identity and Sequence generators can have a direct impact on the performance of your application. In high-load scenarios, sequences usually outperform identity generation because they reduce the round trips required to the database when generating new IDs.
However, your specific use case matters. Always profile your application under expected loads to identify potential bottlenecks. For more details on performance tuning in Hibernate, you can refer to the Hibernate Performance Tuning Guide.
Best Practices for Using Generators
To maximize efficiency and maintain ease of use, consider these best practices:
-
Use Sequence Generation for High Load Applications: If your application experiences heavy write activity, opt for sequence generators.
-
Fallback on Identity Generators for Simplicity: For smaller projects or prototypes, using identity can significantly reduce initial complexity.
-
Be Consistent Across Entities: Try to maintain uniformity in key generation across your application design to avoid confusion and maintain integrity.
-
Test with Different Database Engines: If you anticipate needing to switch databases, conduct tests to ascertain the impact of your chosen key generation strategy.
Key Takeaways
Choosing between Identity and Sequence generators in Hibernate is a critical decision that can affect your application's performance, scalability, and maintainability. While Identity generators are straightforward, they may fail to meet the performance demands of larger applications. Conversely, Sequence generators provide flexibility and efficiency at the cost of added complexity.
By carefully weighing the advantages and disadvantages of each approach, you can find a balance that best serves your project's specific needs. Don't forget to monitor and profile your application, as the performance of these strategies can vary greatly depending on your specific usage patterns.
For further reading on Hibernate and its capabilities, visit the Hibernate ORM Documentation.
In the end, the right choice largely depends on your application’s requirements, so make your decisions wisely!
Feel free to leave your thoughts and experiences in the comments below. What challenges or successes have you faced when working with primary key generation in Hibernate?