Common Pitfalls in Converting Collections with JDK 8
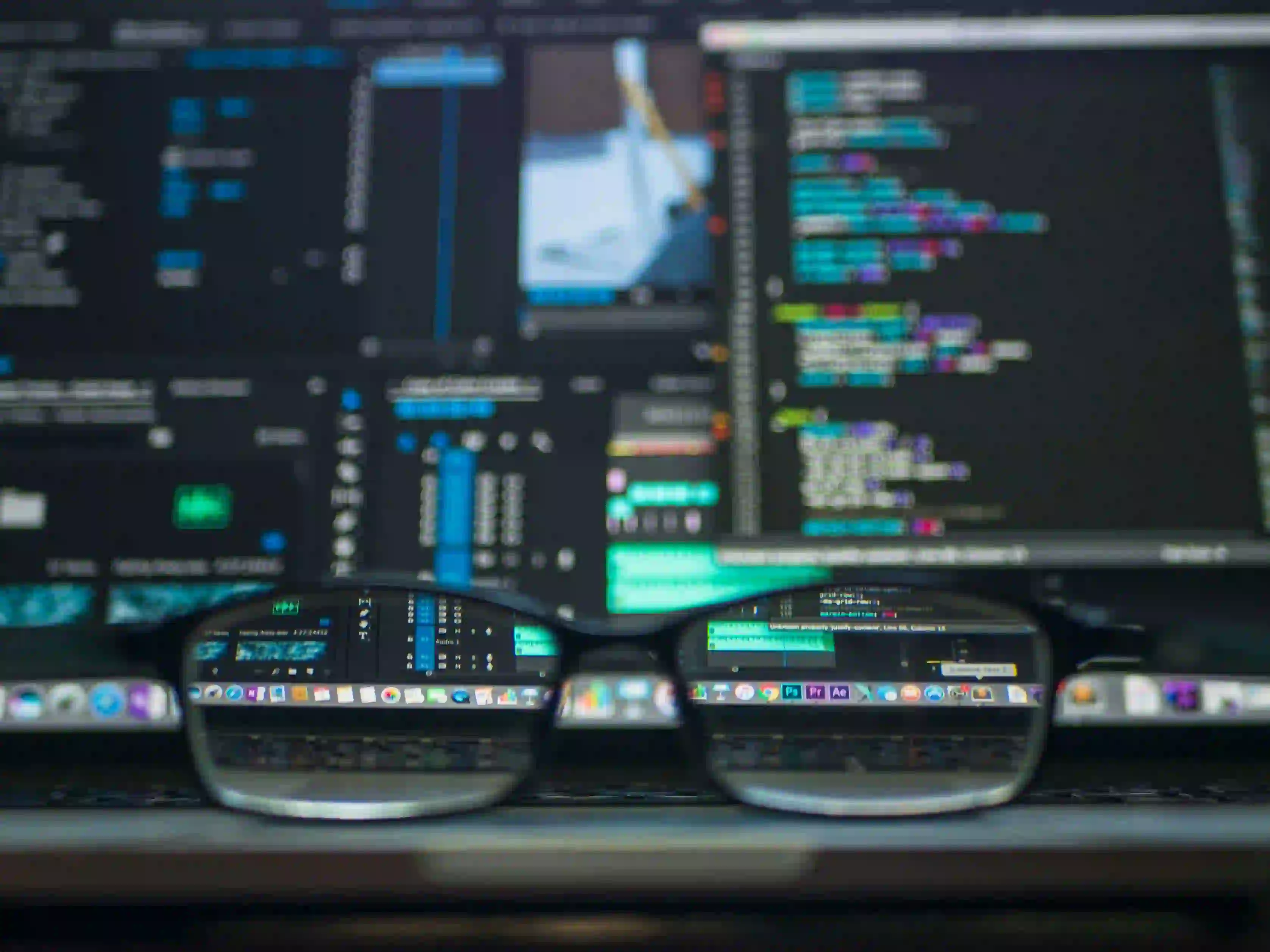
Common Pitfalls in Converting Collections with JDK 8
Java 8 revolutionized the way we handle collections with the introduction of the Stream API. This powerful feature allows developers to express complex operations on collections succinctly and declaratively. However, while the benefits are clear, there are several common pitfalls that developers might encounter when converting collections. Understanding these pitfalls will help you achieve better performance and maintainability in your code.
Understanding the Stream API
The Stream API in Java 8 provides a high-level abstraction for processing sequences of elements. It allows for bulk operations on collections, such as filtering, mapping, and reducing. Here's a simple example of using the Stream API to filter and collect names from a list:
import java.util.Arrays;
import java.util.List;
import java.util.stream.Collectors;
public class StreamExample {
public static void main(String[] args) {
List<String> names = Arrays.asList("John", "Jane", "Jack", "Doe");
List<String> filteredNames = names.stream()
.filter(name -> name.startsWith("J"))
.collect(Collectors.toList());
System.out.println(filteredNames); // Output: [John, Jane, Jack]
}
}
In this example, the filter
method is used to select names that start with the letter "J." Finally, the collect
method gathers the results back into a List
. But there are traps to be wary of while using this powerful tool.
Common Pitfalls
1. Not Understanding Lazy Evaluation
One of the key features of the Stream API is that it employs lazy evaluation. This means that stream operations like filter
, map
, and sorted
are not executed until a terminal operation (like collect
, count
, or forEach
) is invoked.
Example:
List<String> names = Arrays.asList("John", "Jane", "Jack", "Doe");
Stream<String> nameStream = names.stream().filter(name -> {
System.out.println("Filtering: " + name);
return name.startsWith("J");
});
// No output yet; stream operations have not been executed
nameStream.collect(Collectors.toList()); // "Filtering: John", "Filtering: Jane", "Filtering: Jack"
Pitfall: Developers mistakenly think their filtering logic is executed immediately when they call filter
. Use terminal operations carefully to ensure you're aware of when processing occurs.
2. Modifying Collections While Stream Processing
Attempting to modify the underlying source collection while processing a stream can lead to ConcurrentModificationException
.
Example:
List<String> names = new ArrayList<>(Arrays.asList("John", "Jane", "Jack", "Doe"));
names.stream()
.filter(name -> name.startsWith("J"))
.forEach(name -> names.remove(name)); // ConcurrentModificationException
Pitfall: Instead of modifying the original list during stream processing, consider using a separate collection to hold the results first.
3. Confusing map
and flatMap
The difference between map
and flatMap
can be subtle, but it is essential to understand them. The map
function transforms elements, while flatMap
flattens nested structures.
Example with map:
List<List<String>> namesList = Arrays.asList(
Arrays.asList("John", "Jack"),
Arrays.asList("Jane"),
Arrays.asList("Doe")
);
List<String> names = namesList.stream()
.flatMap(List::stream) // Flattens the nested lists
.collect(Collectors.toList());
System.out.println(names); // Output: [John, Jack, Jane, Doe]
Pitfall: Usage of map
instead of flatMap
causes the collection to be nested, leading to potentially unexpected results.
4. Using Collectors Incorrectly
Incorrect use of collectors, such as failing to specify the appropriate downstream collector in grouping or partitioning operations, can yield unintended results.
Example:
List<String> names = Arrays.asList("John", "Jane", "Jack", "Doe");
Map<Integer, List<String>> groupedNames = names.stream()
.collect(Collectors.groupingBy(String::length));
System.out.println(groupedNames); // Output: {3=[Doe], 4=[John, Jack], 4=[Jane]}
Pitfall: Forgetting that keys must be unique can lead to confusion. It's important to understand how your data properties affect the result.
5. Overusing Streams
While streams offer great flexibility, they are not always the best fit for every scenario. Streams introduce some overhead and may lead to reduced performance if overused in simple tasks.
Example:
List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
// Simple sum operation
int sum = 0;
for (Integer number : numbers) {
sum += number;
}
System.out.println(sum); // Output: 15
// Overusing streams
int streamSum = numbers.stream().mapToInt(Integer::intValue).sum();
System.out.println(streamSum); // Output: 15
Pitfall: A simple for-loop can be more efficient than streams for simple operations.
Best Practices for Collection Conversion
1. Use Appropriate Data Structures
Choose the right collection type based on your needs. For instance, prefer a List
for ordered elements and Set
for unique elements.
2. Handle Empty Streams Gracefully
Consider what should happen when a stream is empty. Using Optional
can help you manage operations cleanly.
3. Profile Your Code
Always profile your code when optimizing. Measure the performance impact of using streams compared to traditional iteration before deciding.
4. Readability Matters
Clarity is key. While streams can shorten and simplify syntax, they can also obfuscate logic. Aim to maintain readability, especially for more complex operations.
5. Chain Wisely
Be judicious in chaining operations. Long chains can reduce readability. Break them down into intermediate variables if necessary.
To Wrap Things Up
The Stream API in Java 8 is a powerful addition that can significantly enhance your ability to work with collections. However, it's essential to be aware of the common pitfalls that can trip up even the most seasoned developers. Awareness and understanding will guide you in writing cleaner, more efficient code. By following best practices and leveraging the strengths of streams thoughtfully, you can unlock the full potential of Java's collection framework.
For further reading, consider Java SE 8 Documentation and dive deeper into the intricacies of the Stream API.
If you're interested in more advanced topics, check out our articles on functional programming in Java and the Executor framework for better concurrency management.