Overcoming Data Drift in Machine Learning Microservices
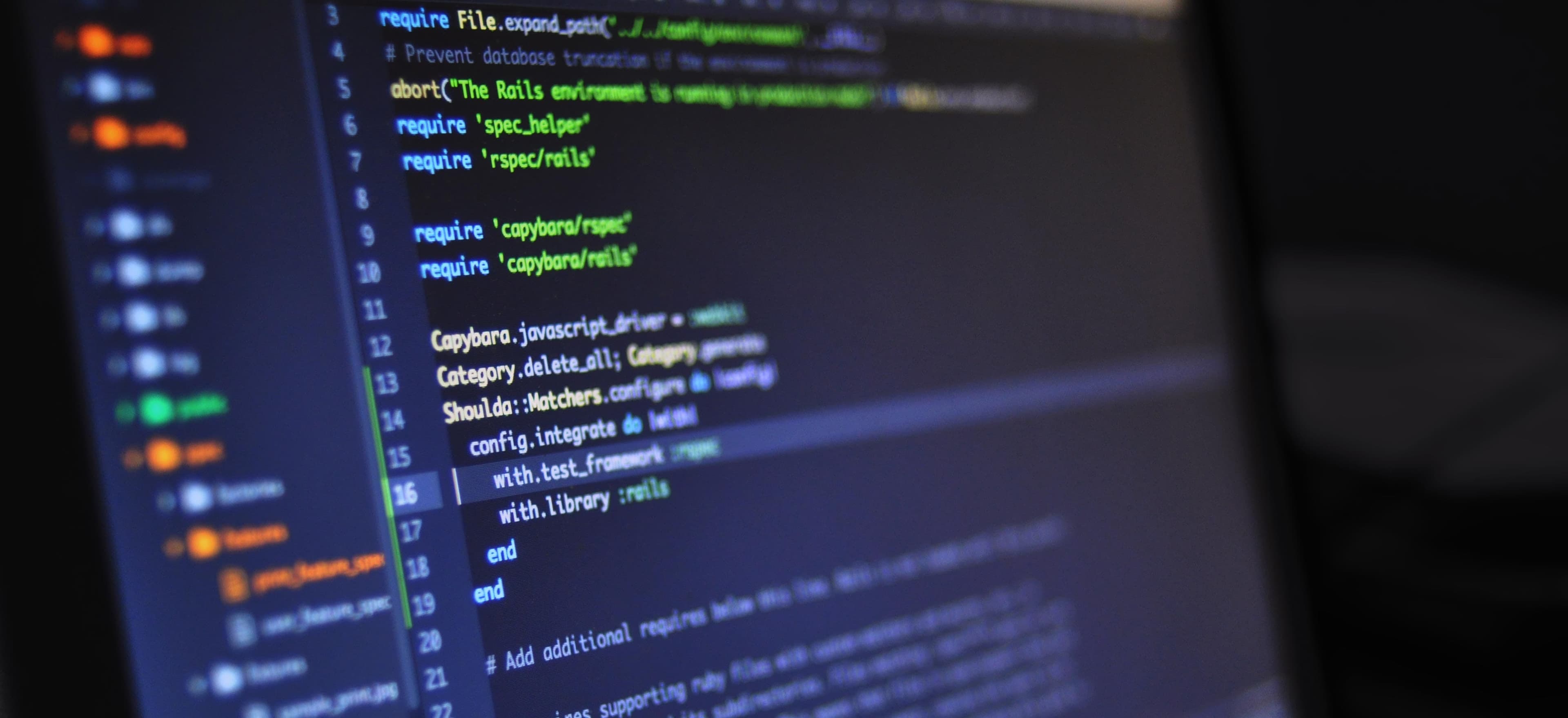
- Published on
Overcoming Data Drift in Machine Learning Microservices
In the rapidly evolving world of machine learning (ML), the deployment of models into production environments represents only the beginning of a complex journey. One of the foremost challenges faced is data drift. This phenomenon occurs when the statistical properties of model input data change over time, leading to a drop in performance. In this blog post, we will explore strategies for mitigating data drift specifically in the context of machine learning microservices.
What is Data Drift?
Data drift can be categorized into two main types:
-
Covariate Shift: This involves changes in the input data distribution while the relationship between the input data and the target variable remains unchanged. An example might be a seasonal shift in user behavior impacting user engagement-related features.
-
Prior Probability Shift: This happens when the distribution of the target variable changes. For instance, if a previous model was trained on data with a specific distribution of customer purchase behavior that later shifts.
Understanding these concepts is crucial because they underline why the output of a model may degrade over time.
Why Data Drift Matters in Microservices
Microservices architecture offers a great deal of flexibility and scalability for deploying machine learning models. However, this architecture also introduces its own complexities regarding model management and monitoring.
Microservices may serve dozens of predictions per second and could be receiving data from various sources, each subject to its own changes. Without robust monitoring, data drift can go unnoticed, potentially leading to poor business decisions based on inaccurate predictions.
Detecting Data Drift
Before we can overcome data drift, we first need to detect it. Here are some techniques commonly used:
Statistical Hypothesis Tests
A common approach is to utilize statistical tests such as the Kolmogorov-Smirnov test or Chi-Squared test to compare the distributions of the training dataset with live incoming data. This allows you to determine if a significant shift has occurred.
public boolean kolmogorovSmirnovTest(double[] sample1, double[] sample2) {
// Implement Kolmogorov-Smirnov Test to detect data drift
// This is a simplified version for explanation
double dStat = calculateKolmogorovStatistic(sample1, sample2);
double threshold = 0.05; // Significance level
return dStat < threshold; // Return true if drift detected
}
Monitoring Feature Distributions
Using visualization tools, such as histograms, boxplots, or even more advanced analytics like drift detection visuals, can help you monitor feature distributions over time.
public void plotFeatureDistribution(List<Double> featureValues, String featureName) {
// Use a library like JFreeChart to plot the distribution of the feature
JFreeChart chart = createHistogram(featureValues, featureName);
ChartPanel chartPanel = new ChartPanel(chart);
// Display the chart for monitoring
}
Visual tools make the problem more tangible and immediate.
Strategies to Overcome Data Drift
Here are some robust strategies for managing and overcoming data drift effectively.
1. Continuous Monitoring
Establish a dedicated monitoring system for live data feed. Tools like Prometheus and Grafana can automate the monitoring process and send alerts for any detected drifts.
Implementation Steps:
- Instrument your microservice to log input data statistics.
- Set up a dashboard using Grafana to visualize trends and anomalies.
- Create an alerting mechanism for timely intervention.
2. Retraining Models
When drift is detected, retraining your model on the most recent data can help recapture lost accuracy. You can automate this process as well.
public void retrainModel(TrainingData newData) {
MachineLearningModel model = new MachineLearningModel();
model.train(newData); // Train on new data, perhaps with a sliding window approach
}
This code snippet lays out a structure for retraining a model with the latest data.
3. Algorithmic Approach
Incorporate algorithms sensitive to changes over time. Techniques such as online learning or rolling window strategies can adapt your model to new data without the overhead of a complete retrain.
public void onlineLearn(NewData newData) {
model.update(newData); // Use incremental learning to update the model in real-time
}
Using incremental learning techniques allows models to update weights and biases efficiently, hence adapting to more recent data trends.
4. Ensembling Approaches
Employ ensemble techniques by maintaining multiple models. Each model could be trained on different data snapshots or approaches, thus providing diverse predictions.
public Prediction ensemblePredict(InputData input) {
List<Prediction> predictions = new ArrayList<>();
for (Model model : models) {
predictions.add(model.predict(input));
}
return combinePredictions(predictions);
}
private Prediction combinePredictions(List<Prediction> predictions) {
// Implement an averaging or majority voting strategy
}
With this code, your system can aggregate predictions from several models, which often leads to better performance and robustness against drift.
The Role of Data Management
Maintaining a pristine dataset is crucial. Ensure you are regularly updating your datasets, possibly with data augmentation techniques. Techniques like synthetic data generation can provide more examples, hence widening the feature space your models can learn.
You can delve deeper into robust data management strategies by reading this article about data quality, which emphasizes the importance of organized datasets in successful ML projects.
The Last Word
Addressing data drift in machine learning microservices is not only essential for maintaining accuracy but also for ensuring the reliability of your services. With continuous monitoring, effective retraining strategies, and leveraging ensemble approaches, the challenges posed by data drift can be successfully managed.
In summary:
- Data drift detection is crucial.
- Continuous monitoring allows you to act swiftly.
- Retraining and algorithmic adjustments can mitigate drift effects.
- Diverse approaches through ensemble learning contribute to robustness.
By integrating these principles, your models will not only survive but thrive in changing environments, ultimately supporting your business objectives.
For further reading, consider exploring this comprehensive guide on machine learning model deployment which focuses on operationalizing models for real-world applications.