Mastering Command Line Parsing in Java with Args4j
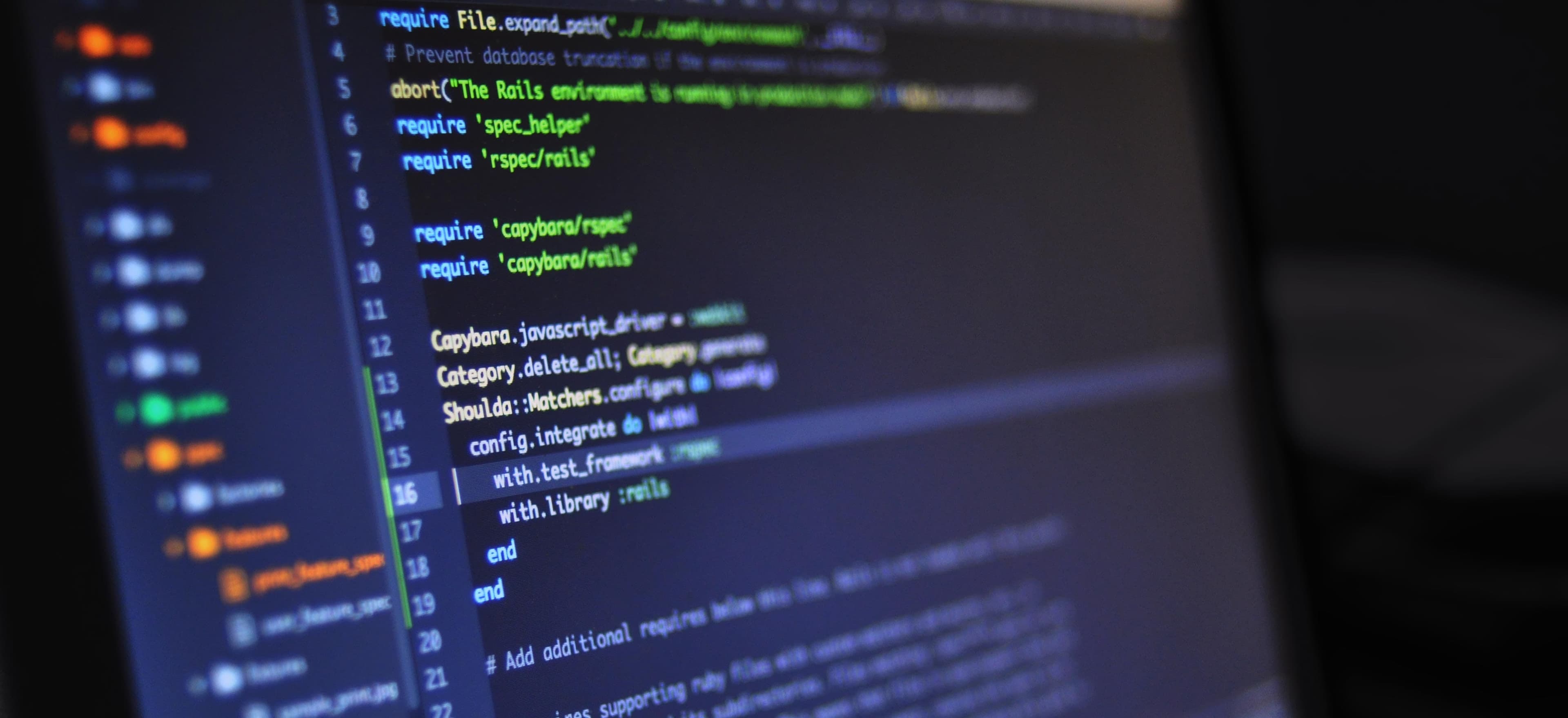
- Published on
Mastering Command Line Parsing in Java with Args4j
Command line parsing is a vital aspect of many Java applications, especially those that involve automation, configuration management, or data processing. Handling command line arguments can be cumbersome, but libraries like Args4j simplify the process significantly. In this blog post, we'll dive deep into the world of command line parsing in Java using Args4j, making it an essential tool in your Java toolkit.
Why Use Args4j?
Before we dive into the code, let’s briefly discuss the importance of command line parsing tools.
-
Simplification: Manually parsing command line arguments can lead to complex, hard-to-maintain code. Using a library like Args4j helps eliminate boilerplate code.
-
Type Safety: Args4j enables type-safe parsing, ensuring that the command line arguments conform to the expected data types.
-
Readability: Code becomes much more readable with annotations and built-in validation.
-
Support for Complex Types: Args4j can manage lists, enums, and even complex objects as command line parameters.
Getting Started with Args4j
To begin, you’ll need to download the Args4j library. The easiest way to include it in your project is through Maven. Here’s how to do so:
Maven Dependency
<dependency>
<groupId>org.kohsuke.args4j</groupId>
<artifactId>args4j</artifactId>
<version>2.33</version>
</dependency>
Make sure to check the Args4j GitHub repository for the latest version.
Basic Usage
Let’s dive into some examples. Start with a simple command line application that accepts various parameters.
Step 1: Define Options with Annotations
We first need to define the expected command line options. Here’s a basic Java class:
import org.kohsuke.args4j.Option;
public class CommandLineOptions {
@Option(name = "-h", usage = "display help message")
private boolean help = false;
@Option(name = "-n", usage = "name of the user")
private String name;
@Option(name = "-a", usage = "age of the user")
private int age;
public boolean isHelp() { return help; }
public String getName() { return name; }
public int getAge() { return age; }
}
Explanation of the Code
-
@Option Annotation: Each field is annotated with
@Option
, specifying the command line switch that corresponds to the field. Theusage
attribute describes what the option does, serving as in-built documentation for your users. -
Default Values: The boolean
help
default value is set to false. If the user does not specify this flag, the value will automatically remain false.
Step 2: Implementing the Parser
Now, we’ll implement the main logic to parse the command line arguments.
import org.kohsuke.args4j.CmdLineParser;
import org.kohsuke.args4j.IllegalOptionException;
import org.kohsuke.args4j.OptionDef;
import org.kohsuke.args4j.spi.Setter;
public class Main {
public static void main(String[] args) {
CommandLineOptions options = new CommandLineOptions();
CmdLineParser parser = new CmdLineParser(options);
try {
parser.parseArgument(args);
} catch (IllegalOptionException ex) {
System.err.println("Error: " + ex.getMessage());
return;
} catch (Exception e) {
parser.printUsage(System.err);
return;
}
if (options.isHelp()) {
parser.printUsage(System.out);
return;
}
if (options.getName() != null) {
System.out.println("Hello " + options.getName());
}
if (options.getAge() > 0) {
System.out.println("Your age is " + options.getAge());
}
}
}
Explanation of the Implementation
-
CmdLineParser Initialization: After creating an instance of
CommandLineOptions
, we create aCmdLineParser
passing in our options object. -
Parsing Arguments: We call
parseArgument(args)
, which processes the command line arguments according to the defined options. -
Error Handling: By catching exceptions, we can handle invalid options gracefully. If the user requests help with the
-h
switch, we output the usage instructions.
Step 3: Running the Application
To run the application, package it, and execute it with various arguments:
java -jar myapp.jar -n Alice -a 30
Example Output
Hello Alice
Your age is 30
Advanced Features of Args4j
Args4j provides several advanced features to make your command line applications more powerful:
1. Lists of Values
To accept multiple values for a single option, you can define a List field, as shown below:
import java.util.List;
public class CommandLineOptions {
@Option(name = "-f", usage = "files to process")
private List<String> files;
// Getter for files
public List<String> getFiles() { return files; }
}
With this setup, users can now specify multiple files:
java -jar myapp.jar -f file1.txt -f file2.txt
2. Enums
Args4j can also parse enum types, improving type safety. Consider this example:
public enum Mode {
CREATE, UPDATE, DELETE;
}
public class CommandLineOptions {
@Option(name = "-m", usage = "operational mode (CREATE, UPDATE, DELETE)")
private Mode mode;
public Mode getMode() { return mode; }
}
Running the application with the mode option would automatically convert the string representation into the corresponding enum value.
3. Custom Validators
In scenarios where you want additional validation, you can create custom validators. Implementing the OptionValidator
interface allows you to enforce specific rules on your command line inputs.
Best Practices for Command Line Parsing
-
Provide Help Information: Always ensure that users can leverage the help option. It makes your application user-friendly.
-
Clear and Concise Naming: Use meaningful names for options to convey their purpose effectively.
-
Validate Input: Ensure the provided data adheres to expected formats or ranges; this will prevent runtime errors.
-
Documentation: Comment on your code to explain specific functionalities. This will help maintain the code better in the long run.
-
Log Useful Information: Instead of just printing error messages, consider logging them for better traceability.
In Conclusion, Here is What Matters
Arg4j is an exceptional library that can significantly ease the burden of command line parsing in Java applications. It is not only simple to set up but also powerful enough to handle complex scenarios seamlessly. Whether you are writing scripts, tools, or full-fledged applications, understanding how to leverage Args4j can elevate your programs to a professional standard.
If you want to explore more about command line argument parsing, consider reading the official Args4j Documentation or check out other libraries like JCommander or Apache Commons CLI for various use cases.
With that, happy coding!