Troubleshooting Inverse Kinematics in JavaFX: Common Issues
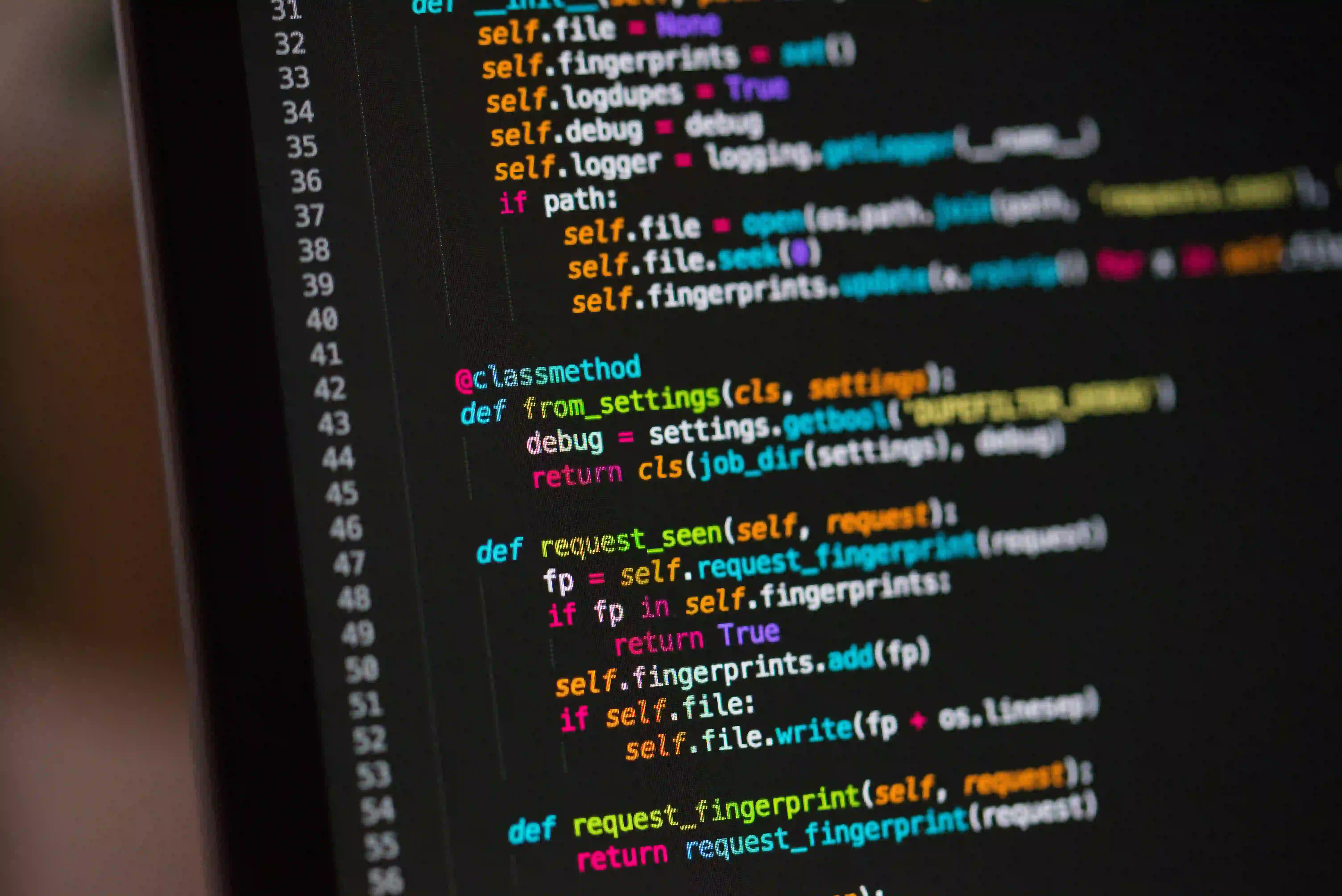
Troubleshooting Inverse Kinematics in JavaFX: Common Issues
Inverse Kinematics (IK) is a fascinating field that intersects robotics and computer graphics, enabling characters and robotic systems to reach desired positions. JavaFX, a rich Java library for creating GUI applications, can also be used for implementing IK algorithms. However, they can be tricky to implement, leading to common issues that developers may face. In this blog post, we'll explore those issues and provide practical troubleshooting tips.
Understanding Inverse Kinematics
Before diving into troubleshooting, let's briefly discuss what inverse kinematics entails. IK generally refers to the mathematical process that calculates the joint movements needed to position an end-effecter (like a hand or foot) at a desired location. In animations or robotics simulations, achieving realistic movements requires correctly implementing IK, which can become complex.
For a more in-depth understanding of IK and its applications, take a look at Resources on Inverse Kinematics.
Common Issues in Inverse Kinematics
Despite the theoretical underpinnings of IK, real implementations can suffer from various issues. Here are the most common problems along with solutions:
1. Joint Limitation Errors
Issue: One of the most common issues in IK is that the computed angles for joints exceed their operational limits, causing unrealistic movements.
Solution: Apply constraints to your joint models. You can enforce limits on the angles by clamping the calculated values. Here's a quick example:
public double clampAngle(double angle, double min, double max) {
if (angle < min) {
return min;
} else if (angle > max) {
return max;
}
return angle;
}
Why: Clamping the angle ensures that joint rotations remain within realistic bounds, ultimately yielding more believable animations.
2. Gimbal Lock
Issue: Gimbal lock is a common problem in three-dimensional rotations where you lose a degree of freedom. This can occur while interpolating between two orientations.
Solution: Use quaternion-based representation instead of Euler angles for rotations. Quaternions do not suffer from gimbal lock and can represent rotation more smoothly.
import javafx.geometry.Point3D;
public class Quaternion {
private double w;
private double x, y, z;
// Quaternion operations...
public Point3D rotate(Point3D point) {
// Convert point to quaternion
Quaternion pQuat = new Quaternion(0, point.getX(), point.getY(), point.getZ());
return this.multiply(pQuat).multiply(this.inverse()).toPoint3D();
}
}
Why: Quaternions allow for smooth and continuous rotations, making them ideal for animated characters or robotic appendages.
3. Distance to Target Issues
Issue: If the endpoint (like the hand of a character) cannot reach the target, it leads to unwarranted behavior. The most common reason is that the target is too far from the base of the arm or leg.
Solution: Always calculate the reachable range before proceeding with IK calculations. Use the Law of Cosines to derive possible endpoint positions.
public boolean isTargetReachable(double distanceToTarget, double armLength) {
return distanceToTarget <= armLength;
}
Why: Pre-checking the reachability condition can save time and effort by avoiding unnecessary calculations when a target is simply out of reach.
4. Convergence Problems
Issue: Convergence problems happen when the algorithm fails to reach the desired target position due to unexpected iterations or loops.
Solution: Set a maximum number of iterations during the IK calculation. If the solution is not found within the specified range, adjust the algorithm or notify the user.
public Point3D calculateIK(Point3D targetPosition) {
int maxIterations = 100;
for (int i = 0; i < maxIterations; i++) {
// IK calculation logic here...
if (isCloseEnough(currentPosition, targetPosition)) {
return currentPosition;
}
}
throw new RuntimeException("Failed to converge to the target.");
}
Why: Setting iteration limits helps prevent infinite loops, leading to more predictable and manageable outcomes.
5. Poor Initial Positioning
Issue: The IK algorithm relies on the initial position of joints. If these are set poorly, your algorithm might converge to suboptimal solutions.
Solution: Implement initialization routines that can estimate reasonable joint positions based on previous states or physical constraints.
public void initializeJointPositions() {
// Reset joints to a neutral position
this.joint1.setPosition(0, 0);
this.joint2.setPosition(0, 0);
this.joint3.setPosition(0, 0);
}
Why: Proper initial positioning acts as a better starting point, improving the chances that the algorithm can successfully reach the target.
6. Performance Bottlenecks
Issue: As your models become complex, performance can degrade, leading to noticeable lag in animations.
Solution: Optimize your IK algorithms by simplifying calculations and using computational techniques like memoization or iterative methods to reduce resource usage.
Use data structures thoughtfully. If you frequently access data points, consider using a HashMap
for O(1) look-up times.
Conclusion
Implementing inverse kinematics in JavaFX presents various challenges. By recognizing and addressing these common issues, developers can enhance their simulations and character animations, leading to improved user experiences.
Always remember that debugging IK scenarios often requires iterative testing and refinement. Leverage the solutions shared in this post to overcome significant roadblocks.
For further reading and deeper insights, consider checking out:
- A Beginner's Guide to Inverse Kinematics
- JavaFX 8 Tutorial: Introducing Inverse Kinematics
Happy coding! If you have any questions or need clarification, feel free to ask in the comments below.