Mastering IntelliJ IDEA’s Complex Internal Design Issues
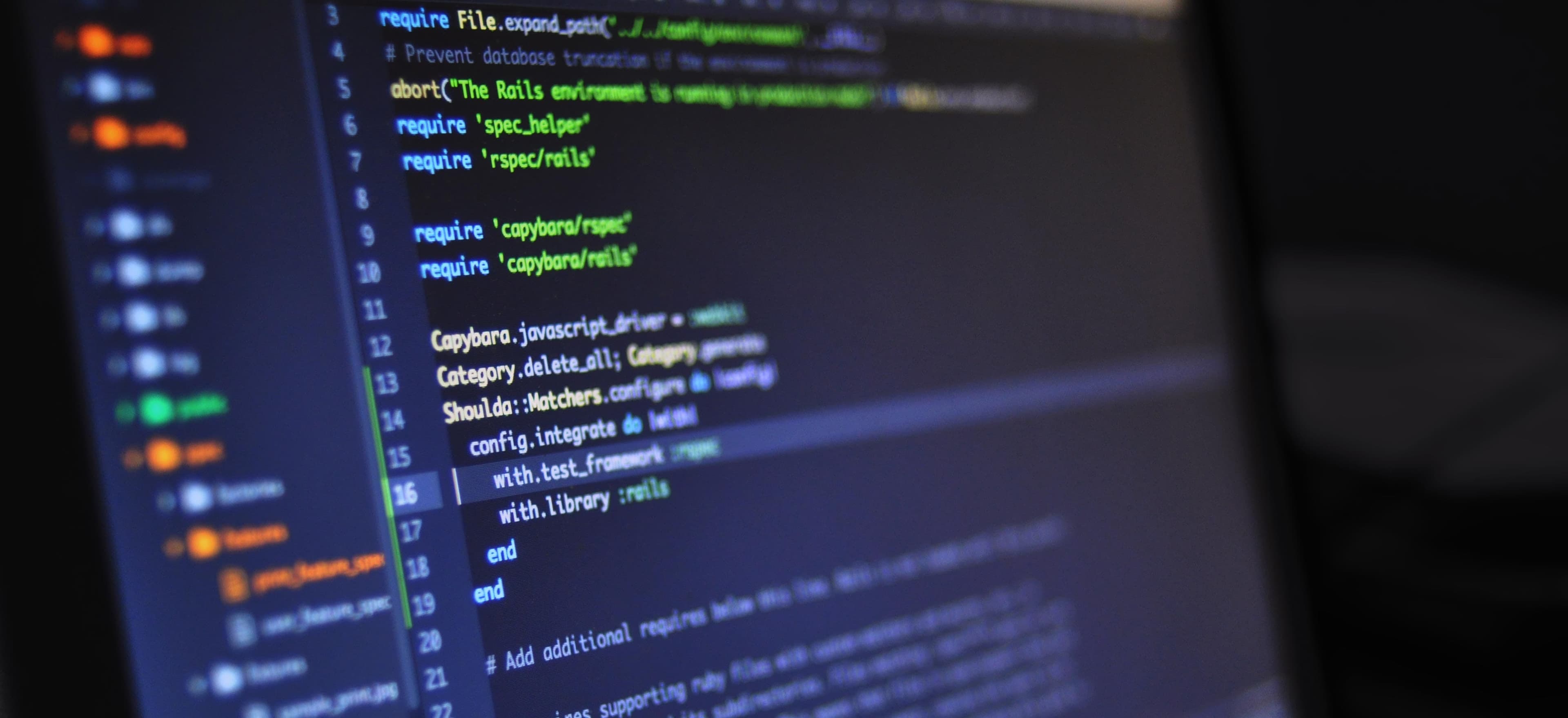
- Published on
Mastering IntelliJ IDEA’s Complex Internal Design Issues
IntelliJ IDEA, a popular integrated development environment (IDE) for Java, is widely renowned for its rich features and efficient user experience. However, like any sophisticated software, it carries with it a complexity that can often bewilder even the most seasoned developers. This blog post delves deep into IntelliJ IDEA’s intricate internal design issues, helping you to master its functionalities while gaining insights into its architecture.
Understanding IntelliJ IDEA’s Architecture
High-Level Overview
At its core, IntelliJ IDEA is built on a multi-layer architecture. This architecture is composed of several modules, including the platform core, editor framework, project model, and various plugins that enhance its functionality. Understanding how these modules interact is crucial for addressing design issues effectively.
Key Components
- Platform Core: The backbone of IntelliJ IDEA, it manages the IDE's basic functionalities like project structure, file management, and plugin integration.
- Editor Framework: Provides capabilities for syntax highlighting, code completion, and code navigation.
- Project Model: Allows management of project configurations, dependencies, and build systems.
This multi-layered approach provides flexibility and modularity, enabling developers to customize the IDE through plugins while maintaining robust core functionalities.
Impact of Complexity
Given its intricate architecture, several challenges arise:
- Performance Issues: Inefficiencies in code processing can slow down the IDE.
- Debugging Difficulties: The complex interdependencies among modules complicate traceability.
- Plugin Compatibility: Plugins might conflict due to underlying architectural assumptions.
Mastering IntelliJ IDEA requires not only using the features but also understanding how to navigate these issues.
Handling Performance Issues
Code Efficiency Optimization
Performance concerns frequently stem from inefficient code. For instance, if your application starts lagging, it can often be traced back to how data is managed within the IDE.
Here's a simple code snippet that showcases file reading optimization using a buffer:
import java.io.BufferedReader;
import java.io.FileReader;
import java.io.IOException;
public class FileHandler {
public void readFile(String filepath) {
try (BufferedReader br = new BufferedReader(new FileReader(filepath))) {
String line;
while ((line = br.readLine()) != null) {
processLine(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
private void processLine(String line) {
// Processing logic here
System.out.println(line);
}
}
Why Use BufferedReader?
Using BufferedReader
is advantageous because it reduces the number of I/O operations. Instead of reading each character individually, it reads larger chunks of data at a time. This leads to improved performance, especially when dealing with large files.
Additional Resources:
For those interested in delving deeper into Java I/O performance, consider exploring the Java I/O documentation.
Debugging Challenges
Debugging in IntelliJ IDEA can sometimes feel like finding a needle in a haystack. The IDE’s complex design makes it tricky to pinpoint issues in code, especially when working across multiple modules.
Enhancing Traceability
To improve the traceability of errors, using log4j
or a similar logging framework is a best practice. Here’s an example of how to set it up:
<dependency>
<groupId>org.apache.logging.log4j</groupId>
<artifactId>log4j-core</artifactId>
<version>2.14.1</version>
</dependency>
And in your Java code:
import org.apache.logging.log4j.LogManager;
import org.apache.logging.log4j.Logger;
public class Application {
private static final Logger logger = LogManager.getLogger(Application.class);
public static void main(String[] args) {
logger.info("Application started");
try {
// Some logic
} catch (Exception e) {
logger.error("An error occurred: ", e);
}
}
}
Why Logging Matters
Using logs helps in keeping track of application behavior and aids in troubleshooting by providing a clear historical account of actions taken and errors encountered. It keeps the stack traces manageable and relevant.
Plugin Compatibility Issues
IntelliJ IDEA encourages a vibrant ecosystem of plugins. However, conflicts between plugins can lead to unexpected behavior. Understanding how to manage these conflicts can save you significant time.
Debugging Plugins
When a plugin causes issues, isolate the problem by disabling it and re-enabling it one by one.
To identify plugin compatibility issues, ensure you follow best practices when developing plugins:
- Keep dependencies explicit.
- Document your plugin's expected environment.
- Regularly test against the latest version of IntelliJ IDEA.
Plugin Example
Here’s a simple example of implementing a basic plugin that demonstrates how to use the IDE’s API correctly:
import com.intellij.openapi.components.ServiceManager;
import com.intellij.openapi.project.Project;
public class MyPlugin {
public static void performAction(Project project) {
// Your plugin code here
System.out.println("Plugin action executed for project: " + project.getName());
}
}
Why Follow Best Practices?
Adhering to best practices ensures that your plugin will play nicely within the broader ecosystem of IntelliJ IDEA. Understanding the design principles of the platform will lead to fewer conflicts and better user experiences.
Lessons Learned
Mastering IntelliJ IDEA entails navigating its complex internal design issues while utilizing its powerful features. Focus on optimizing performance, enhancing debugging processes, and ensuring plugin compatibility.
By comprehending the intricacies of its architecture, you will not only become a more proficient developer but will also unlock the full potential of IntelliJ IDEA.
Additional Learning
For more insights into IntelliJ IDEA’s capabilities, consider checking out the official JetBrains IntelliJ IDEA Documentation and the community support forums for collective knowledge and troubleshooting strategies.
Stay curious, keep exploring, and happy coding!