How to Effectively Eliminate Null Pointer Exceptions in Java
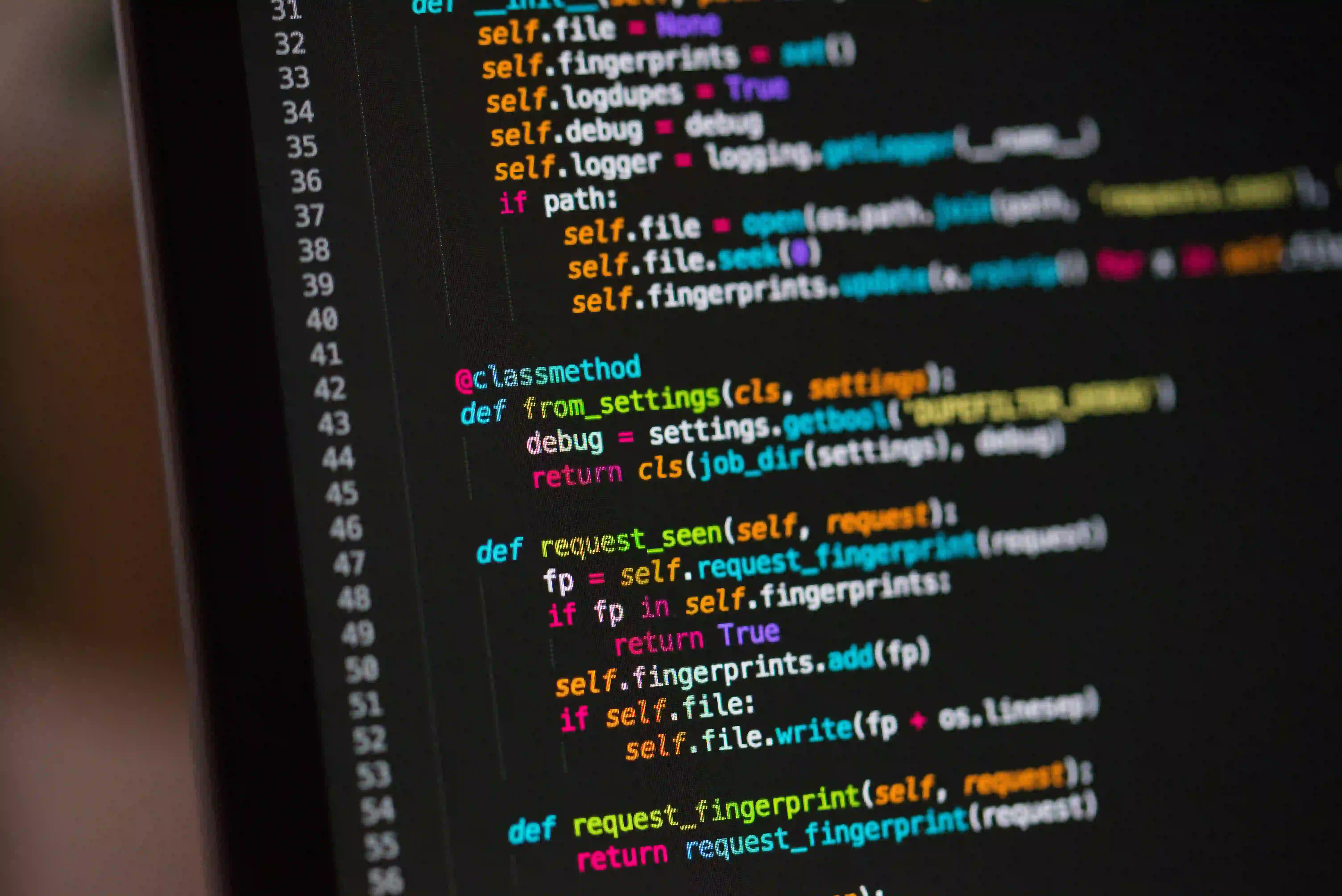
How to Effectively Eliminate Null Pointer Exceptions in Java
Null Pointer Exceptions (NPEs) are often considered the bane of Java developers. There’s no doubt about it: encountering an NPE during runtime can be frustrating. But fear not! This blog post will guide you on how to effectively eliminate Null Pointer Exceptions in Java, ensuring that your code is not only clean but also robust and reliable.
Understanding Null Pointer Exceptions
A Null Pointer Exception occurs when your code attempts to access or manipulate an object reference that is null
. In simpler terms, it means you're trying to use something that doesn't exist. In Java, when you see a NullPointerException, it typically looks something like this:
java.lang.NullPointerException
at com.example.MyClass.myMethod(MyClass.java:10)
This could happen for various reasons, such as:
- Forgetting to initialize an object before use.
- Data returned from a method being
null
. - Accessing elements of a collection that may hold
null
values.
It’s fundamental to manage null values effectively to maintain application stability.
Strategies to Avoid Null Pointer Exceptions
1. Use Java’s Built-In Null Checks
Java provides several ways to perform null checks. The most straightforward method is to use if
statements.
public void processUser(User user) {
if (user != null) {
// Proceed with processing the user object
System.out.println(user.getName());
} else {
System.out.println("User is null");
}
}
Why this helps: This method guarantees that your program checks the object’s existence before attempting to proceed, thus avoiding NPEs.
2. Utilize Optional
The Optional
class was introduced in Java 8 to provide a more elegant way to deal with values that might be absent. Instead of returning null
, consider returning an Optional
.
import java.util.Optional;
public Optional<User> getUserById(String userId) {
User user = userRepository.findById(userId);
return Optional.ofNullable(user);
}
Why this helps: Optional
enforces a contract that requires the developer to consider the absence of a value, providing useful methods like isPresent()
, ifPresent()
, and orElse()
to handle such cases.
3. Use Annotations
Leveraging annotations like @NonNull
or @Nullable
can help by providing documentation and tooling support. While they do not enforce checks at runtime, they serve as guidelines for developers.
public void saveUser(@NonNull User user) {
// Code to save user
}
Why this helps: Annotations can guide static analysis tools to alert developers when they may be misusing null references.
4. Avoid Returning Null
One best practice is to avoid returning null
. Return an empty list, set, or other suitable default values instead.
public List<User> findUsers() {
List<User> users = userRepository.findAllUsers();
return users != null ? users : Collections.emptyList();
}
Why this helps: By ensuring your methods return valid objects regardless of content, you maintain consistency and avoid questionable calls.
5. Use Assertions
For debugging, assertions can help catch situations where a variable should not be null.
public void assertUser(User user) {
assert user != null : "User should not be null";
// Additional processing
}
Why this helps: Assertions clearly indicate during development that a certain condition must hold true, thereby helping you catch potential issues early.
6. Defensive Programming
Defensive programming promotes writing code that anticipates user or external input failures. This matter-of-fact approach involves validating input parameters.
public void updateUser(User user) {
if (user == null) {
throw new IllegalArgumentException("User cannot be null");
}
// Update the user
}
Why this helps: By preemptively checking for issues, your code becomes more resilient against misuse.
Code Example
Here’s a consolidated code snippet combining several methods discussed above.
import java.util.Collections;
import java.util.List;
import java.util.Optional;
public class UserService {
public Optional<User> findUserById(String userId) {
return Optional.ofNullable(userRepository.findById(userId));
}
public List<User> getAllUsers() {
List<User> users = userRepository.findAll();
return users != null ? users : Collections.emptyList();
}
public void updateUser(@NonNull User user) {
if (user == null) {
throw new IllegalArgumentException("User cannot be null");
}
// Perform update
}
}
Why this helps: This UserService class employs multiple techniques to avoid NPEs, showcasing a robust structure.
Final Thoughts
Null Pointer Exceptions are a common issue in Java programming, but they can be effectively mitigated. By employing null checks, utilizing Optional
, using annotations, and embracing defensive programming techniques, you can write cleaner, safer code that resists the frustrations associated with NPEs.
By keeping your code clear and implementing these strategies, you create a more reliable application. Interested in exploring more on error handling in Java? Check out this comprehensive guide on exception handling for additional context.
Remember, a solid understanding of null management is a pillar of proficient Java programming! Happy coding!