Transitioning to Android's New Build System: Common Pitfalls
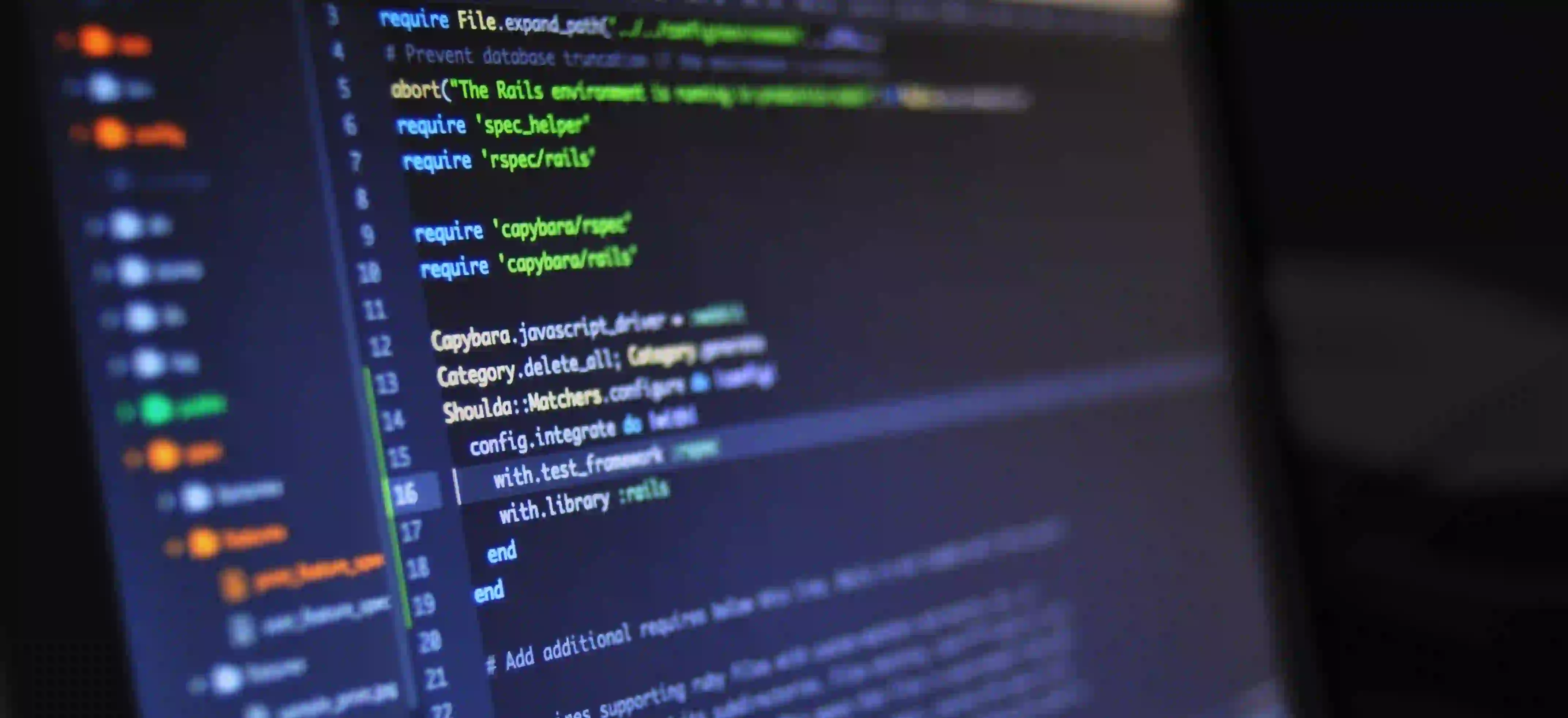
Transitioning to Android's New Build System: Common Pitfalls
The shift to Android's new build system has proven to be a game changer for many developers. However, while the transition can enhance productivity and performance, it's not without its challenges. This blog post will explore common pitfalls when moving from the old build system to Gradle, Google's officially recommended tool since Android Studio 0.1. Each issue will be accompanied by insights and code snippets to help you navigate the labyrinth of Android development more effectively.
Understanding the Build System
Before diving into the pitfalls, it's crucial to understand how the new build system works. Gradle is a build automation tool that integrates and optimizes the build lifecycle. Here’s why Gradle is favored:
- Flexibility: It supports multiple languages and platforms.
- Incremental Builds: Builds only what has changed, improving build times.
- Gradle Scripts: Uses Groovy or Kotlin DSL, making builds easily customizable.
Common Pitfalls
1. Dependency Management Issues
One of the most frequent problems developers face involves improper handling of dependencies. In the old build system, dependencies were often managed manually, making it easy to overlook version conflicts.
Pitfall Example: Conflicting library versions can arise, resulting in runtime crashes or unexpected behavior.
Solution
Utilizing Gradle's dependency management effectively is the solution. Always declare dependencies with specific versions, and when possible, use dependency constraints to avoid version conflicts.
dependencies {
implementation 'com.squareup.retrofit2:retrofit:2.9.0' // Specific version
implementation 'com.squareup.retrofit2:converter-gson:2.9.0' // Specific version
}
Why It Works: Specifying versions helps Gradle manage dependencies accurately, reducing the risk of bugs caused by mismatched library versions.
2. Ignoring Gradle Caching
Gradle's caching mechanism is one of its most powerful features. Unfortunately, many developers tend to ignore this, leading to longer builds.
Pitfall Example: Having to recompile unaffected modules because caching wasn't utilized.
Solution
Make sure to follow best practices for Gradle caching. Here is how to configure it:
android {
buildCache {
local {
enabled = true // Enable local caching
directory = "$rootDir/.gradle/build-cache" // Set location
}
}
}
Why It Works: When caching is utilized properly, it significantly reduces build times, as Gradle doesn't have to rebuild parts of the project that haven't changed.
3. Misconfiguring the Build Variants
Android's new build system provides robust support for multiple build variants. However, misconfiguring them can lead to chaos in your project.
Pitfall Example: Failing to set up product flavors and build types correctly might result in incorrect APK outputs.
Solution
Define your build variants carefully using the productFlavors
and buildTypes
blocks. Here’s an example:
android {
productFlavors {
free {
applicationIdSuffix ".free"
versionNameSuffix "-free"
}
paid {
applicationIdSuffix ".paid"
versionNameSuffix "-paid"
}
}
buildTypes {
debug {
buildConfigField "boolean", "IS_PRODUCTION", "false"
}
release {
buildConfigField "boolean", "IS_PRODUCTION", "true"
}
}
}
Why It Works: This setup allows you to create different versions of your app easily while managing configurations and resources specifically for each flavor.
4. Ignoring Build Script Optimization
Over time, projects can become cluttered with multiple build scripts that are poorly organized, leading to difficulty in maintenance and longer build times.
Pitfall Example: Redundant code in multiple build files makes it difficult to apply changes across the board.
Solution
Refactor your build scripts and move common configurations into a separate build.gradle
file. This can enhance maintainability and clarity.
ext {
commonDependencies = [
'com.android.support:appcompat-v7:28.0.0',
'com.android.support:design:28.0.0'
]
}
dependencies {
implementation commonDependencies
}
Why It Works: By centralizing common configurations, you reduce redundancy and improve the clarity of your build setup.
5. Not Utilizing Dynamic Feature Modules
Dynamic Feature Modules allow you to modularize your application further, which can be advantageous for large projects. Many developers are unaware of this feature or fail to implement it.
Pitfall Example: Keeping all app features in a single module makes the initial download size larger than necessary.
Solution
Consider splitting your application into multiple modules strategically. Here’s a simple implementation.
Add a Dynamic Feature Module into your app’s build.gradle
:
dynamicFeatures {
dynamicFeatureModule 'my.dynamic.feature'
}
Why It Works: This approach enables users to download only the core application features initially and provides the flexibility to download additional features on demand.
6. Skipping Build Scans
Build scans provide valuable insight into your build process, yet many developers fail to leverage this powerful debugging tool.
Pitfall Example: Unidentified performance bottlenecks can slow down development.
Solution
Enable build scans by adding the following line to your gradle.properties
file:
org.gradle.scan=true
After a build, you can retrieve a link that leads to a comprehensive scan of the build process.
Why It Works: Understanding build performance via scans can pinpoint areas needing optimization, making it easier to improve build efficiency.
In Conclusion, Here is What Matters
Transitioning to Android's new build system can be overwhelming, but being aware of these common pitfalls will arm you with the knowledge to navigate your way to a successful implementation. By properly managing dependencies, optimizing build scripts, and utilizing Gradle's powerful features, you can transform your Android development experience.
If you are looking for more advanced implementations, consider diving deeper into the official Android Developer Documentation for comprehensive guidelines and best practices.
Remember, each project is unique, so while these pitfalls may not apply to everyone, they are a great starting point for enhancing your Android build experience. Happy coding!
Extra Resources
- Gradle Build System Overview - A deeper dive into Gradle for Android development.
- Common Gradle Tasks - Understand the various tasks Gradle can handle in your project.
By being proactive and informed, you can ensure a smoother transition and an effective build process in your Android projects.