Mastering NIO.2: Solving Common File Handling Issues
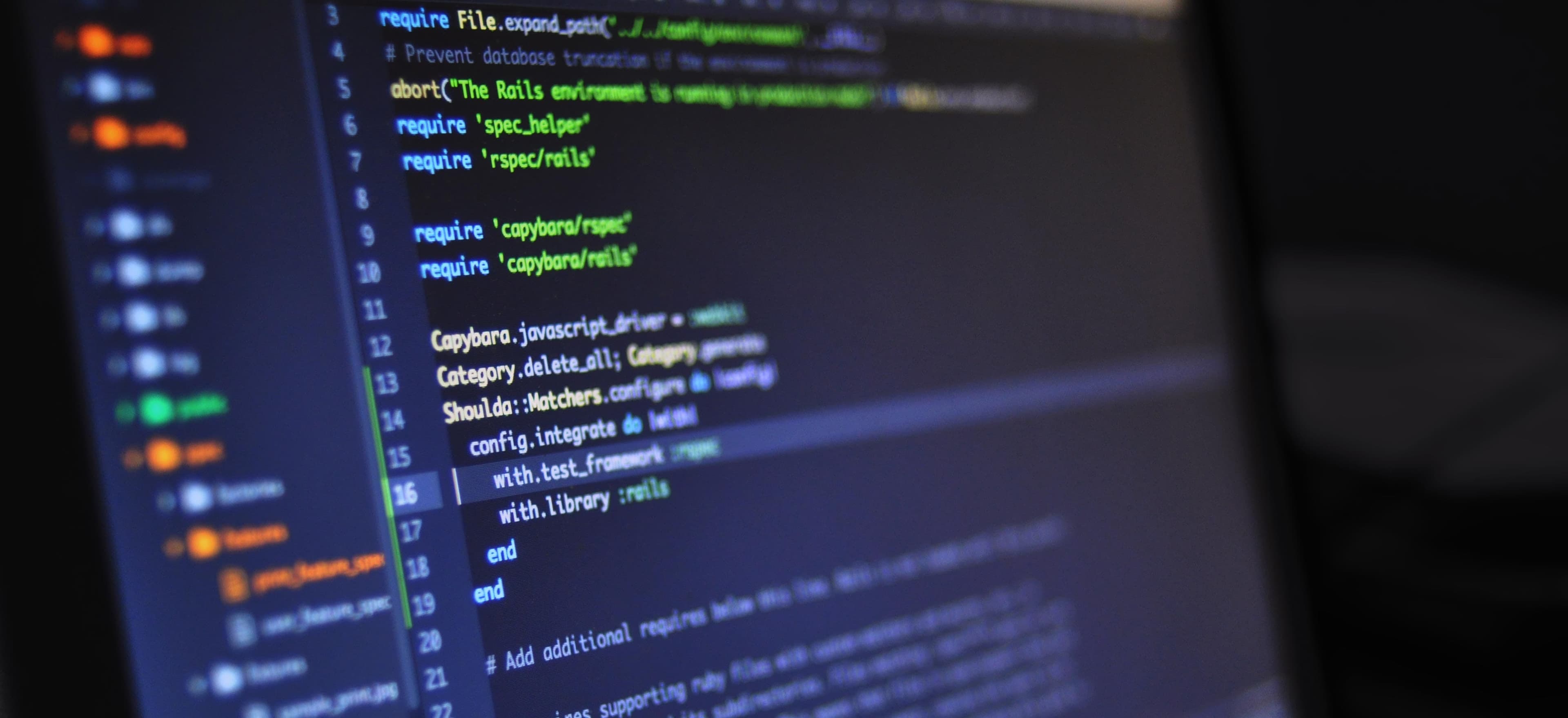
- Published on
Mastering NIO.2: Solving Common File Handling Issues
Java's New Input/Output (NIO.2) package, introduced in Java 7, revolutionizes file handling in the Java ecosystem. It brings an array of functionalities to manage file and directory operations more efficiently. If you're looking to enhance your file handling capabilities and tackle common issues, this blog post is for you.
In this comprehensive guide, we'll delve into various aspects of NIO.2, including code examples, best practices, and common pitfalls. By the end, you'll have a firm grasp of how to utilize NIO.2 to handle files seamlessly in Java.
What is NIO.2?
NIO.2, known as the Java NIO or New Input/Output, caters to advanced file system operations. It builds on the earlier NIO package and introduces the java.nio.file
package, enhancing file handling by providing a new file system interface and a range of utilities.
Benefits of NIO.2
- Simplicity: NIO.2 simplifies file and directory management operations.
- Asynchronous Capabilities: It allows for non-blocking I/O operations, which are useful for applications that require high performance.
- Cross-platform Support: NIO.2 operates uniformly across different platforms, ensuring consistent behavior.
- Improved Error Handling: With better exception handling features, diagnosing issues becomes easier.
Common File Handling Issues and Solutions
Let's explore some common file handling challenges developers often face, along with practical NIO.2 solutions.
1. Reading Files
Issue: Reading files using traditional methods can be cumbersome and often leads to resource leaks.
NIO.2 Solution
Using Files.readAllLines()
, we can effortlessly read the contents of a file into a list of strings.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
import java.util.List;
public class FileReaderExample {
public static void main(String[] args) {
Path filePath = Paths.get("example.txt");
try {
List<String> lines = Files.readAllLines(filePath);
lines.forEach(System.out::println);
} catch (IOException e) {
System.err.println("Error reading file: " + e.getMessage());
}
}
}
Why This Works: Utilizing Files.readAllLines()
abstracts away the complexities of handling file streams. It efficiently manages resources and ensures the file is closed after reading, preventing potential leaks.
2. Writing to Files
Issue: Writing data to files can often lead to overwriting existing content inadvertently.
NIO.2 Solution
By using Files.write()
, we can specify the StandardOpenOption
to prevent overwriting.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
import java.io.IOException;
import java.util.Arrays;
public class FileWriterExample {
public static void main(String[] args) {
Path filePath = Paths.get("output.txt");
try {
// This will append text
Files.write(filePath, Arrays.asList("Line 1", "Line 2"),
StandardOpenOption.CREATE,
StandardOpenOption.APPEND);
} catch (IOException e) {
System.err.println("Error writing file: " + e.getMessage());
}
}
}
Why This Works: The use of StandardOpenOption.APPEND
allows us to add new content without erasing existing data, maintaining file integrity.
3. Copying Files
Issue: Copying files can sometimes result in unexpected failures, especially when paths don't resolve correctly.
NIO.2 Solution
Using Files.copy()
, we can easily copy files while managing exceptions effectively.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class FileCopyExample {
public static void main(String[] args) {
Path sourceFile = Paths.get("source.txt");
Path targetFile = Paths.get("target.txt");
try {
Files.copy(sourceFile, targetFile);
System.out.println("File copied successfully");
} catch (IOException e) {
System.err.println("Error copying file: " + e.getMessage());
}
}
}
Why This Works: The Files.copy()
method not only provides a straightforward way to duplicate files, but it also throws exceptions for errors like non-existent source files. This helps in debugging.
4. Deleting Files
Issue: Deleting files may leave behind unhandled exceptions, especially if the file doesn't exist.
NIO.2 Solution
We can reliably delete files using Files.deleteIfExists()
, which ensures safe deletion.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class FileDeleteExample {
public static void main(String[] args) {
Path filePath = Paths.get("deleteMe.txt");
try {
boolean deleted = Files.deleteIfExists(filePath);
if (deleted) {
System.out.println("File deleted successfully");
} else {
System.out.println("File not found");
}
} catch (IOException e) {
System.err.println("Error deleting file: " + e.getMessage());
}
}
}
Why This Works: By using Files.deleteIfExists()
, you avoid throwing exceptions if the file does not exist, making your code more robust.
5. Traversing Directories
Issue: Recursively searching through directories can be a tedious process.
NIO.2 Solution
Java NIO.2 provides an efficient way to traverse directories using the Files.walk()
method.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class DirectoryWalkExample {
public static void main(String[] args) {
Path start = Paths.get("myDirectory");
try {
Files.walk(start)
.filter(Files::isRegularFile)
.forEach(System.out::println);
} catch (IOException e) {
System.err.println("Error walking through directory: " + e.getMessage());
}
}
}
Why This Works: Using Files.walk()
, you can conveniently navigate through directory trees, applying filters and actions on the files easily.
Best Practices with NIO.2
- Use Path Instead of File: Prefer using
Path
objects overFile
for handling file and directory paths. Paths offer more capabilities and flexibility. - Always Handle Exceptions: Properly manage exceptions, especially with I/O operations. Clear error messages help identify issues quickly.
- Use Try-With-Resources: Whenever you handle streams, always consider using the try-with-resources statement to guarantee the closure of resources.
Closing Remarks
Java NIO.2 is a powerful toolset that simplifies file handling while enabling robust management capabilities. By mastering NIO.2, you can effectively tackle common file handling issues and write cleaner, more efficient Java code.
For further reading and advanced techniques on NIO.2, consider visiting Java NIO Official Documentation. Dive deeper into asynchronous I/O, file system attributes, and file watching to harness the full potential of NIO.2 in your applications.
Implement these techniques, and you'll find that handling files in Java can be as straightforward as reading and writing text. Happy coding!
Checkout our other articles