Top 5 Android Programming Pitfalls and How to Avoid Them
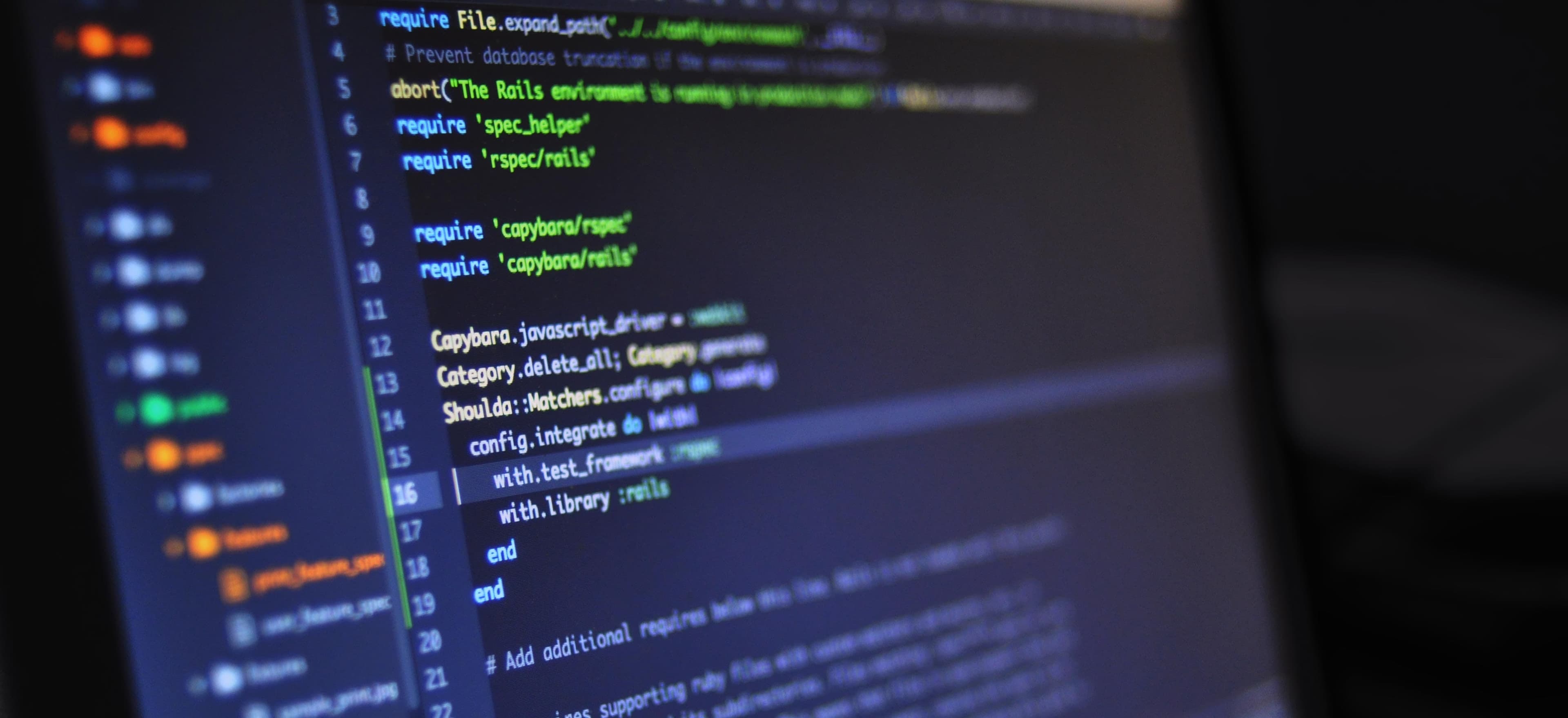
- Published on
Top 5 Android Programming Pitfalls and How to Avoid Them
Android is a powerful platform for creating mobile applications, but it comes with its complexities. As developers, we must navigate potential pitfalls that could hinder performance, maintainability, and user experience. In this blog post, we'll discuss the top five common pitfalls in Android programming and provide solutions to avoid them. This will boost your efficiency and help you create apps that stand out.
1. Memory Leaks
What is a Memory Leak?
A memory leak occurs when your app retains references to objects that are no longer needed, leading to increased memory usage and potential crashes. This often happens with Activity
and Context
references when using non-static inner classes, AsyncTask
, or listeners.
How to Avoid Memory Leaks
Use Weak References
Weak references allow the garbage collector to reclaim an object, helping prevent memory leaks. Here’s an example of using a WeakReference
for an AsyncTask
.
public class MyAsyncTask extends AsyncTask<Void, Void, Void> {
private WeakReference<MainActivity> activityReference;
MyAsyncTask(MainActivity context) {
activityReference = new WeakReference<>(context);
}
@Override
protected Void doInBackground(Void... voids) {
// Background work
return null;
}
@Override
protected void onPostExecute(Void result) {
MainActivity activity = activityReference.get();
if (activity == null || activity.isFinishing()) return;
// Update UI
}
}
In this code snippet, MainActivity
is passed as a weak reference, preventing it from being held in memory unnecessarily after it is destroyed.
Additional Techniques
Consider following these best practices:
- Use
ViewModel
andLiveData
from Android's Architecture Components to manage UI-related data in a lifecycle-conscious way. - Utilize the
onDestroy()
method judiciously to nullify references to prevent leaks.
2. Ignoring the Lifecycle
The Importance of Lifecycle Awareness
The Android system manages the lifecycle of components like Activities
and Fragments
. Ignoring lifecycle states can lead to crashes or undesirable behavior, as resources may not be correctly released or acquired based on the lifecycle state.
How to Avoid Lifecycle Issues
Utilize Lifecycle-Aware Components
The Android Architecture Components include LifecycleOwner
and LifecycleObserver
, allowing you to respond to lifecycle changes appropriately.
public class MyActivity extends AppCompatActivity implements LifecycleObserver {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
getLifecycle().addObserver(this);
}
@OnLifecycleEvent(Lifecycle.Event.ON_START)
public void connect() {
// Connect to resources
}
@OnLifecycleEvent(Lifecycle.Event.ON_STOP)
public void disconnect() {
// Disconnect from resources
}
}
By adding lifecycle observers, you can control resource connection and disconnection more reliably, ensuring your app behaves predictably through various state changes.
Emphasizing Best Practices
- Always unregister listeners or observers in
onStop()
oronDestroy()
. - Use
lifecycle-aware
components instead of manually managing lifecycles.
3. Poor UI Thread Management
Why UI Thread Management Matters
Android enforces a single-threaded architecture for the UI. Running long operations on the UI thread can lead to Application Not Responding (ANR) errors, causing a poor user experience.
How to Properly Manage the UI Thread
Use Background Threads
Always perform heavy tasks, such as network calls or database operations, off the UI thread.
new Thread(new Runnable() {
@Override
public void run() {
// Simulate long task
// After task completion, update UI on the main thread
runOnUiThread(new Runnable() {
@Override
public void run() {
// Update UI elements
}
});
}
}).start();
Using a separate thread, as shown above, ensures that the UI remains responsive.
Consider Using AsyncTask or Executors
For less complex tasks, AsyncTask
or Executors
can manage threading more efficiently.
ExecutorService executorService = Executors.newSingleThreadExecutor();
executorService.execute(new Runnable() {
@Override
public void run() {
// Perform background work
// Update UI on the main thread if necessary
}
});
Summary of Best Practices
- Use
AsyncTask
,HandlerThread
, orExecutors
for background tasks. - Always update UI elements on the main thread.
4. Hardcoding Strings and Resources
The Risks of Hardcoding
Hardcoding strings or resource identifiers makes your code unmanageable and difficult to translate into other languages. It can lead to errors when modifying your app or adding features.
How to Properly Manage Strings and Resources
Use Resources Instead of Hardcoding
Leverage the strings.xml
file to define string literals and other resources:
<resources>
<string name="welcome_message">Welcome to My App!</string>
</resources>
Access it programmatically:
String welcomeMessage = getString(R.string.welcome_message);
Emphasizing Best Practices
- Use string resources for all user-facing text.
- Maintain structured resource organization for easy localization.
5. Overlooking Gradle Build Optimizations
Why Gradle Configuration Matters
Inefficient or wrong Gradle configuration can lead to long build times and bloated application sizes, affecting development speed and user experience.
How to Optimize Gradle Builds
Enable ProGuard or R8
Gradle’s ProGuard or R8 can help shrink the size of your APK by removing unused resources and code. This can drastically decrease loading times.
buildTypes {
release {
minifyEnabled true
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
Enabling minifyEnabled
in your build.gradle
file ensures that ProGuard or R8 runs during the release build.
Additional Optimizations
- Avoid unnecessary dependencies by regularly cleaning up your
build.gradle
file. - Use
build types
wisely to control different configurations (like debug vs. release) effectively.
The Closing Argument
Navigating the Android programming landscape can be challenging, fraught with many pitfalls ranging from memory leaks to poor resource management. By implementing the strategies outlined above, you can build more robust, maintainable, and user-friendly applications.
To deepen your understanding, consider exploring the following resources:
- Android Developer Documentation
- Android Architecture Components
Avoiding these common pitfalls will set you on a path to success in Android programming. Happy coding!
Checkout our other articles