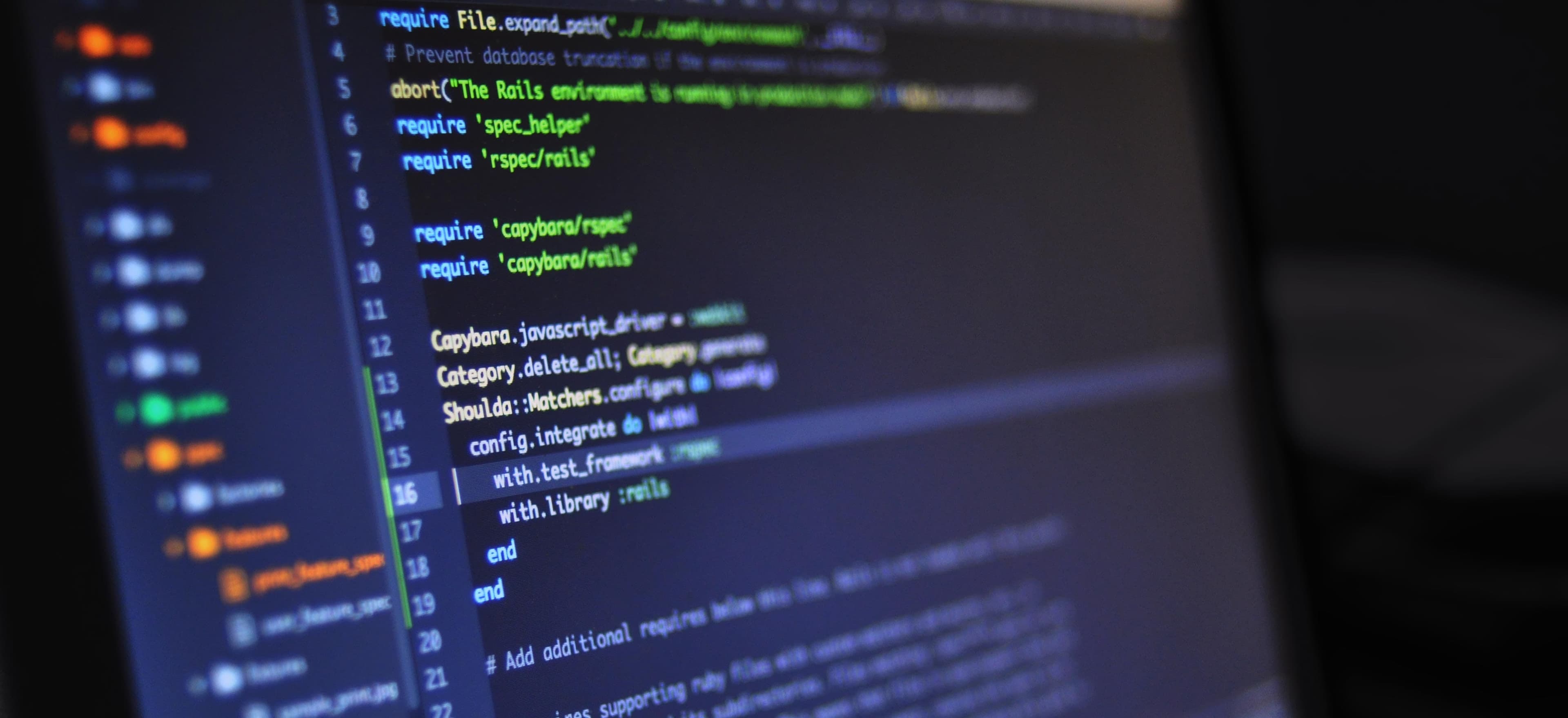
- Published on
Solving GWT Integration Issues with Spring and Hibernate
Google Web Toolkit (GWT) remains a powerful solution for building complex web applications. When combined with robust frameworks like Spring and Hibernate, the capabilities of GWT can be enhanced significantly, allowing developers to create scalable and maintainable applications.
However, integrating GWT with Spring and Hibernate can present a unique set of challenges. In this blog post, we will explore some of the common integration issues developers face and provide strategies for overcoming them. We will also include actual code snippets with explanations to enhance your understanding.
Understanding GWT, Spring, and Hibernate
Before diving into the issues and solutions, it is essential to have a brief understanding of each framework.
-
GWT: GWT allows developers to write client-side applications using Java, which is then compiled into JavaScript. This eliminates the need for developers to work with JavaScript directly.
-
Spring: Spring is a powerful framework for building Java applications. It provides comprehensive infrastructure support and allows for a modular architecture.
-
Hibernate: Hibernate is an ORM (Object-Relational Mapping) tool that simplifies database interactions in Java applications. It enables developers to manage database operations through Java objects rather than SQL queries directly.
Common Integration Issues
1. Handling GWT RPC Calls
GWT uses Remote Procedure Calls (RPC) to communicate between the client-side and server-side code. Integrating Spring with GWT can sometimes break this communication.
Solution: Use Spring's @RequestMapping
to define endpoint URLs for your RPC services.
@RestController
public class MyServiceImpl implements MyService {
@Autowired
private MyService myService;
@Override
@RequestMapping(value = "/myService", method = RequestMethod.POST)
public MyResponse myMethod(MyRequest request) {
// Your business logic here
return myService.processRequest(request);
}
}
Why?: This approach keeps your service methods consistent with Spring MVC, enabling better integration between GWT's RPC mechanism and Spring's request handling.
2. Sharing JVM Classes Between Client and Server
One of the challenges in GWT applications is ensuring that classes used in RPC are serializable and available on both the client and server sides.
Solution: Code GWT's shared classes in a shared module.
// In common library
public class MySharedObject implements Serializable {
private String data;
public MySharedObject() {}
public MySharedObject(String data) {
this.data = data;
}
public String getData() {
return data;
}
public void setData(String data) {
this.data = data;
}
}
Why?: By placing your shared classes in a separate module, you can manage them more effectively and ensure that they are included in both the client and server code.
3. Configuration of Hibernate Sessions
Another common problem arises when managing Hibernate sessions. A common pattern is to open a session for every request and close it afterward. However, in a GWT application, session management often becomes tricky due to the asynchronous nature of RPC calls.
Solution: Use Spring's @Transactional
annotation to manage Hibernate sessions automatically.
@Service
public class MyEntityService {
@Autowired
private MyEntityRepository repository;
@Transactional
public MyEntity save(MyEntity entity) {
return repository.save(entity);
}
}
Why?: The @Transactional
annotation ensures that the session is properly opened and closed for each method execution, saving you from manual session management and potential memory leaks.
Best Practices for GWT with Spring and Hibernate
Reusing Form Objects
Instead of creating separate objects for GWT and server-side processing, use the same objects as much as possible. This promotes DRY (Don't Repeat Yourself) principles and reduces maintenance overhead.
Use DTOs (Data Transfer Objects)
For complex data, consider using DTOs to simplify the data structure passed between GWT and Spring. DTOs can help minimize the data exposed to the client and improve performance.
public class MyEntityDTO {
private Long id;
private String name;
// getters and setters
}
Why: This separation makes it easier to manage changes to the server-side model without breaking the client-side application.
Exception Handling
Proper exception handling is critical in ensuring that the client understands server responses. Use Spring's @ControllerAdvice
for a global exception handling strategy.
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
public ResponseEntity<ErrorResponse> handleException(Exception ex) {
// Log exception
ErrorResponse errorResponse = new ErrorResponse("Internal Server Error", ex.getMessage());
return new ResponseEntity<>(errorResponse, HttpStatus.INTERNAL_SERVER_ERROR);
}
}
Why?: This approach centralizes error handling, making your application more robust and easier to maintain.
The Closing Argument
Integrating GWT with Spring and Hibernate can be expected to bring complexity, but with a systematic approach to managing RPC calls, session handling, and reliable error management, these challenges can be addressed effectively.
By following the best practices outlined above and utilizing the strategies provided, you can ensure that your GWT application is not just functional but also elegant and easy to maintain.
For further reading and examples on GWT, Spring, and Hibernate, consider checking the respective official documentation:
- GWT Documentation
- Spring Framework Documentation
- Hibernate Documentation
By adopting these best practices, you can set a solid foundation for your GWT applications, ensuring scalability and maintainability as your application evolves. Happy coding!
Checkout our other articles