Mastering Java 8 SQL One-Liners: Tips for Joyful Coding
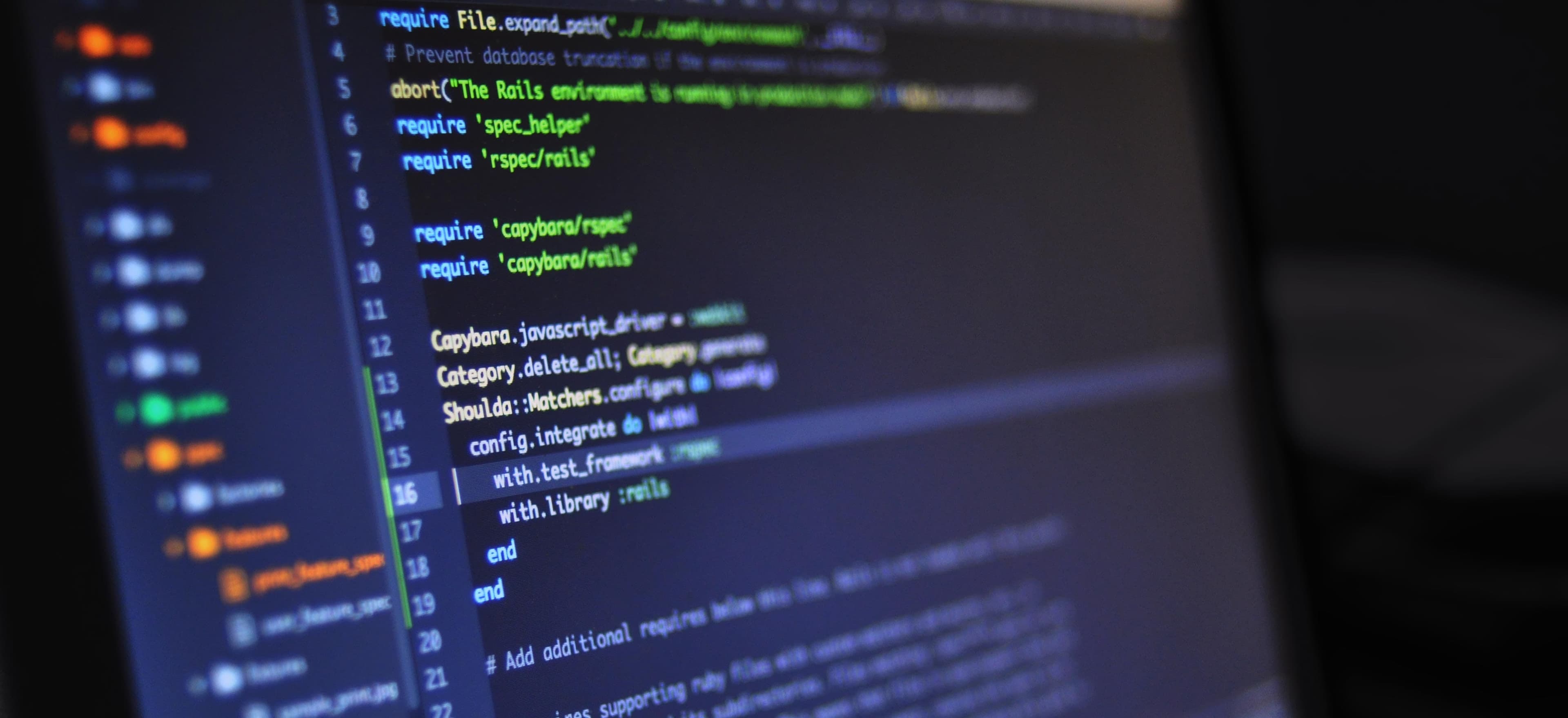
- Published on
Mastering Java 8 SQL One-Liners: Tips for Joyful Coding
Java has long been a powerhouse in the programming world, and with the introduction of Java 8, things got even more exciting, especially in terms of handling SQL data. The addition of the Stream API and lambda expressions has revolutionized how we work with collections and database results.
In this blog post, we will delve into some SQL one-liners using Java 8 features. These snippets will not only make your code cleaner but will also enhance your productivity. Let's roll up our sleeves and dive into joyful coding!
Understanding Java 8 Streams
Before we kick off, it’s essential to understand the Stream API. A stream is a sequence of data elements that can be processed in a functional style. Here's the official Java documentation for further reading.
The real magic happens when you combine streams with lambda expressions. This powerful duo allows for expressive code and real-time transformations.
Setting Up a Sample Database
For our examples, we will assume you have a database set up with a table called employees
. This table contains the following columns: id
, name
, department
, and salary
.
Here’s what the structure might look like:
| id | name | department | salary | |-----|--------------|------------|---------| | 1 | Alice | HR | 70000 | | 2 | Bob | IT | 80000 | | 3 | Charlie | IT | 60000 | | 4 | David | Marketing | 60000 |
To connect Java to a SQL database, we typically use JDBC. Here’s how to set up a connection:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.SQLException;
public class DatabaseConnector {
public static Connection connect() {
try {
return DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
} catch (SQLException e) {
throw new RuntimeException("Failed to connect to database", e);
}
}
}
Explanation
This snippet connects to a MySQL database. Make sure to replace "mydatabase"
, "username"
, and "password"
with your actual database credentials. The connection object will be used for executing SQL queries.
SQL One-Liners: Fetching Employees
Now, let’s get into the SQL one-liners using the Stream API. We will retrieve employee data and perform some operations.
To fetch all employees from the database and collect them into a list, we can write the following one-liner:
import java.sql.*;
import java.util.List;
import java.util.stream.Collectors;
public class EmployeeFetcher {
public static List<Employee> fetchAllEmployees() {
try (Connection conn = DatabaseConnector.connect();
Statement stmt = conn.createStatement()) {
ResultSet rs = stmt.executeQuery("SELECT * FROM employees");
return mapResultSetToList(rs);
} catch (SQLException e) {
throw new RuntimeException("Failed to fetch employees", e);
}
}
private static List<Employee> mapResultSetToList(ResultSet rs) throws SQLException {
return (List<Employee>) ((Iterable<Employee>) () -> new ResultSetIterator(rs));
}
}
Explanation
In the above code:
try-with-resources
automatically closes resources when done.mapResultSetToList
uses a custom iterator (ResultSetIterator
) that convertsResultSet
into a streamable sequence of elements.
Now, let’s implement the ResultSetIterator
:
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Iterator;
public class ResultSetIterator implements Iterator<Employee> {
private final ResultSet rs;
private boolean hasNext;
public ResultSetIterator(ResultSet rs) throws SQLException {
this.rs = rs;
hasNext = rs.next(); // move to the first row
}
@Override
public boolean hasNext() {
return hasNext;
}
@Override
public Employee next() {
try {
Employee employee = new Employee(rs.getInt("id"), rs.getString("name"), rs.getString("department"), rs.getDouble("salary"));
hasNext = rs.next(); // move to the next row
return employee;
} catch (SQLException e) {
throw new RuntimeException("Failed to access row", e);
}
}
}
Explanation
In this custom iterator:
- It implements
Iterator<Employee>
, allowing it to return instances of the Employee class. - The
next
method moves the cursor forward and fetches the data while maintaining the internal pointer.
Filtering Employees
Let’s filter employees by salary. Assuming we want all employees earning more than 65000:
import java.util.stream.Stream;
public class EmployeeFilter {
public static List<Employee> getHighEarners(List<Employee> employees) {
return employees.stream()
.filter(e -> e.getSalary() > 65000)
.collect(Collectors.toList());
}
}
Explanation
- The
filter
method uses a lambda expression to specify our condition. collect(Collectors.toList())
gathers the filtered results back into a list.
Aggregating Data: Average Salary
Calculating the average salary for employees in our database can be accomplished in one concise line:
import java.util.OptionalDouble;
public class EmployeeAggregator {
public static OptionalDouble getAverageSalary(List<Employee> employees) {
return employees.stream()
.mapToDouble(Employee::getSalary)
.average();
}
}
Explanation
Here’s what’s happening:
mapToDouble(Employee::getSalary)
transforms our Employee objects into a double stream representing their salary.average()
calculates the mean of these salaries. The return type isOptionalDouble
, encapsulating the possibility that there may be no values to average.
Putting It All Together
Now, let's integrate everything into our main application:
public class MainApp {
public static void main(String[] args) {
List<Employee> employees = EmployeeFetcher.fetchAllEmployees();
List<Employee> highEarners = EmployeeFilter.getHighEarners(employees);
highEarners.forEach(System.out::println);
OptionalDouble averageSalary = EmployeeAggregator.getAverageSalary(employees);
averageSalary.ifPresent(avg -> System.out.println("Average Salary: " + avg));
}
}
Explanation
In MainApp
, we first fetch all employees, then filter and print high earners, and finally calculate and print the average salary. This architecture aids in keeping your code modular, tests easier, and upgrades smoother.
The Closing Argument
Mastering Java 8 SQL one-liners can significantly enhance your coding joy and productivity. By leveraging streams and lambda expressions, your SQL-related code can become concise and more readable.
For additional learning, explore the official Java tutorials on Lambda Expressions and Streams.
Remember, the real power of Java 8 emerges when you create expressive, functional-style solutions. Happy coding!
Checkout our other articles