Overcoming Design Challenges in Android Game Development
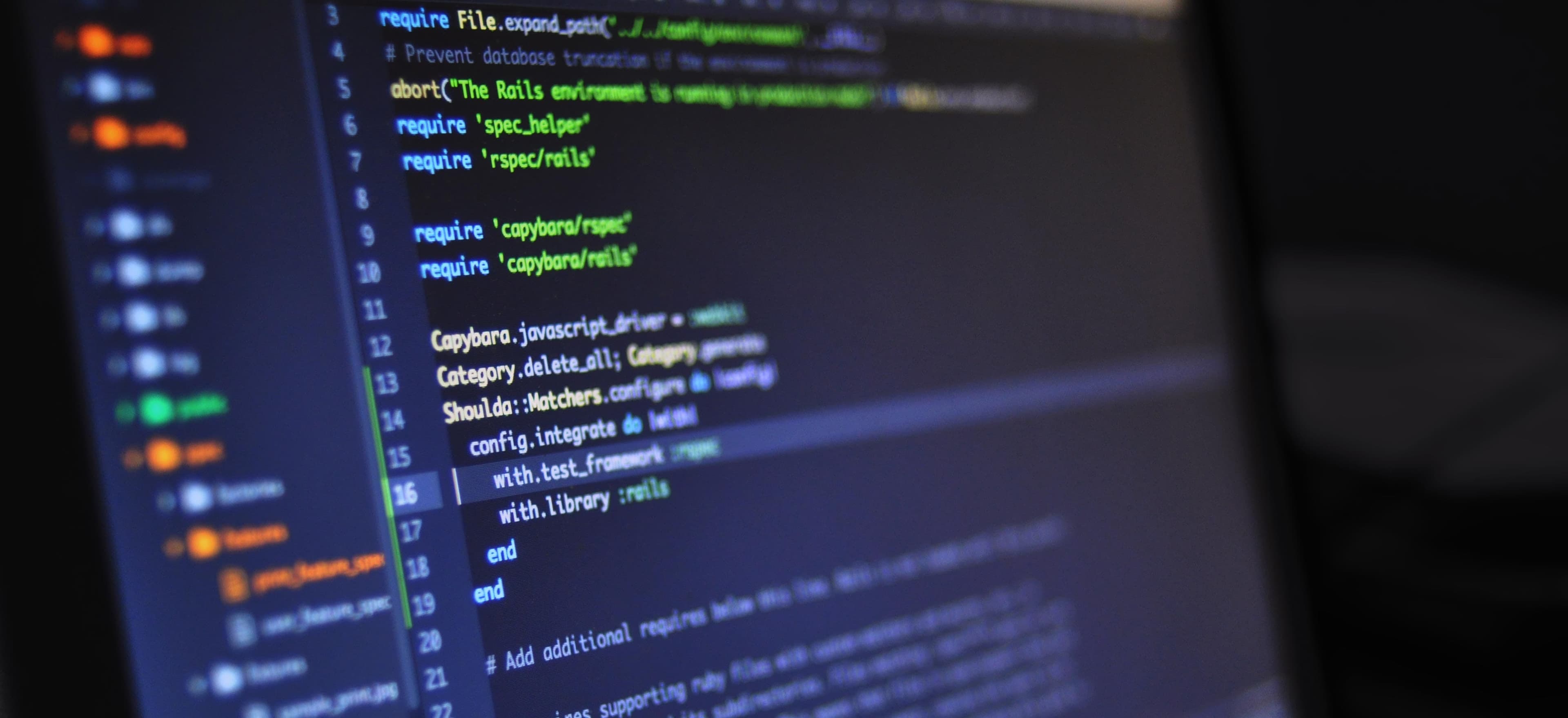
- Published on
Overcoming Design Challenges in Android Game Development
Game development on the Android platform brings unique challenges. From performance constraints to screen sizes, developers must navigate various hurdles to create successful games. In this blog post, we’ll explore common design challenges in Android game development and offer practical solutions.
Understanding the Android Ecosystem
Before we dive into specific challenges, it’s important to understand the Android ecosystem. Android is an open-source operating system widely used on many devices, which means:
- Diverse Screen Sizes and Resolutions: From watches to tablets, devices vary greatly.
- Multiple Hardware Configurations: Different manufacturers implement varying hardware capabilities.
- Fragmentation: Numerous versions of the OS are in use, complicating support.
The above factors can significantly impact game design and development. Let’s break down some common challenges.
1. Managing Performance Constraints
The Challenge
Android devices range from high-end smartphones to older, budget devices. Games that are graphically intensive may run smoothly on high-end devices but can struggle on lower-end models. This inconsistency can lead to a poor user experience.
The Solution
To tackle performance issues, it's crucial to optimize your game's graphics and code. Here are some tips:
-
Use Efficient Graphics: Utilize 2D sprites instead of 3D models when possible. If you're incorporating 3D, consider using libraries like LibGDX, which simplify rendering.
-
Implement Lazy Loading: Load assets only when needed. For example, in a platformer game, do not load all levels at once; instead, load them as the player progresses.
// Load level only when needed
if (player.isNearNextLevel()) {
loadLevel(nextLevel);
}
- Profile Your Game: Use tools like Android Profiler to identify bottlenecks and improve game performance.
2. Optimizing User Interface for Various Screen Sizes
The Challenge
Android devices come in a range of screen sizes and resolutions. A UI designed for one device can look cluttered or improperly laid out on another.
The Solution
Designing responsive UIs is crucial in accommodating different devices. Key strategies include:
- Use ConstraintLayout: This layout manager helps create flexible UIs that adapt to various screen sizes.
<androidx.constraintlayout.widget.ConstraintLayout
android:layout_width="match_parent"
android:layout_height="match_parent">
<Button
android:id="@+id/startButton"
android:layout_width="0dp"
android:layout_height="wrap_content"
app:layout_constraintWidth_percent="0.5"
app:layout_constraintHeight_default="wrap"
app:layout_constraintTop_toTopOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
-
Flexible Design: Utilize
dp
instead ofpx
for dimensions. This helps scale interface elements appropriately on different devices. -
Multiple Layouts and Resources: Create different layout XML files for screen sizes (e.g.,
layout-small
,layout-large
). Android will pick the right layout at runtime.
3. Sound and Music Synchronization
The Challenge
Achieving proper synchronization of sound effects and background music can be tricky, particularly in fast-paced games. Poor synchronization may disrupt the immersive experience.
The Solution
Using an audio framework like OpenSL ES can help in precise sound management. Moreover, use asynchronous loading for sound assets:
AudioTrack audioTrack = new AudioTrack(
AudioManager.STREAM_MUSIC,
sampleRate,
channelConfig,
audioFormat,
bufferSize,
AudioTrack.MODE_STREAM
);
audioTrack.play(); // Start playback
Make sure to preload audio assets and carefully manage playback timing. For example, utilize SoundPool for short sound effects, which allows for quick loading and playing.
4. Handling Different Device Capabilities
The Challenge
Different devices come with varying processing power, screen resolutions, and sensor capabilities, which can lead to compatibility issues and a degraded user experience.
The Solution
Implement adaptive graphics settings that adjust based on device capabilities:
- Graphics Quality Settings: Allow users to select low, medium, or high graphics settings based on their device's capabilities.
if (deviceIsLowEnd()) {
setGraphicsQuality(GraphicsQuality.LOW);
} else {
setGraphicsQuality(GraphicsQuality.HIGH);
}
- Check Hardware Features: Use the PackageManager to detect if the device supports certain features before implementing them. This helps in avoiding game crashes on unsupported devices.
PackageManager pm = context.getPackageManager();
if(!pm.hasSystemFeature(PackageManager.FEATURE_SENSOR_ACCELEROMETER)) {
// Disable tilt controls
}
5. Testing Across Devices
The Challenge
Testing across a wide range of devices is time-consuming but essential before deploying your game.
The Solution
-
Use Cloud Testing Services: Platforms such as Firebase Test Lab allow you to test your app on different devices in the cloud.
-
Emulators and Virtual Devices: Android Studio offers emulators. However, ensure testing on real devices for better accuracy.
-
Automated Testing: Implement unit tests using JUnit or UI tests via Espresso to continually ensure the quality of your game throughout the development process.
In Conclusion, Here is What Matters
Developing games for Android can be challenging, but with understanding and preparation, you can overcome these hurdles. Focus on performance optimization, responsive UI design, synchronization of audio, managing device capabilities, and thorough testing.
By embracing these strategies, you can enhance your game's reliability and usability across the diverse Android ecosystem. Don’t forget to stay updated with the latest development practices and frameworks to continually improve your skills. Happy coding!
For more resources on Android game development, check out the following links:
With these insights at hand, your journey in Android game development will surely be a rewarding adventure!