Unlocking Mapped Diagnostic Context for Java EE Logging
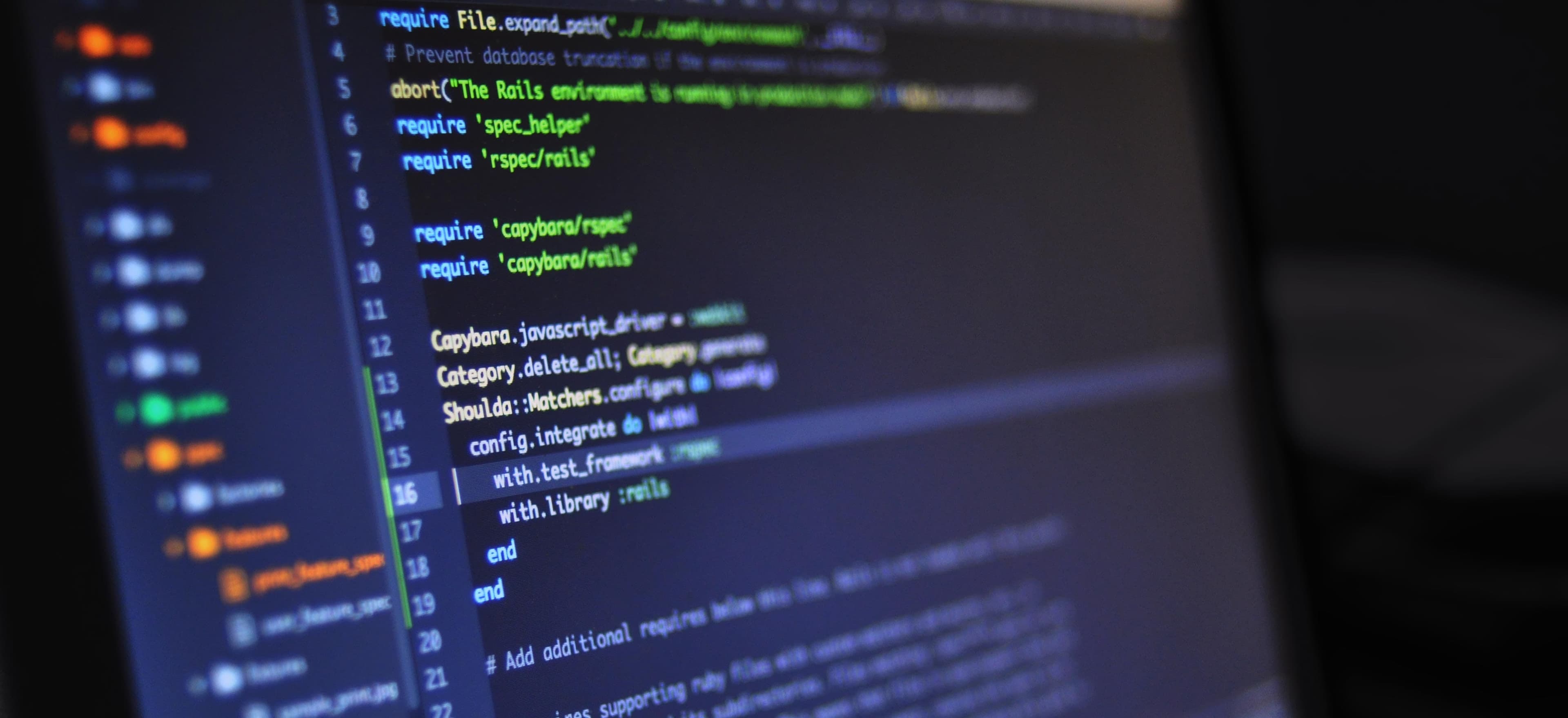
- Published on
Unlocking Mapped Diagnostic Context for Java EE Logging
In the realm of Java Enterprise Edition (Java EE), logging might appear straightforward, but it often harbors complexities that can lead to inefficiencies in troubleshooting and monitoring. One of the most effective tools in a Java developer's logging arsenal is the concept of Mapped Diagnostic Context (MDC). This blog post aims to demystify MDC and illustrate its powerful capabilities through engaging discussions and practical code examples.
What is Mapped Diagnostic Context (MDC)?
The Mapped Diagnostic Context is a feature provided by logging frameworks, most notably Log4j and SLF4J, that allows developers to enrich log messages with contextual information. This additional context can include user identifiers, session IDs, request paths, and more, permitting developers to trace the flow of execution more effectively within their applications.
Benefits of Using MDC
- Contextual Logging: Helps in capturing the environment in which particular actions occurred, improving traceability.
- Debugging: Facilitates easier debugging as developers can correlate log entries with specific user actions or application states.
- Asynchronous Logging: Enables context propagation across asynchronous threads, providing cohesive logs even in concurrent execution.
Setting Up Logging with MDC
Before we dive into the implementation, ensure you have a logging framework set up in your Java EE application. For our example, we'll use SLF4J with Logback.
Maven Dependency
If you're using Maven for your project, include the following dependencies in your pom.xml
file:
<dependencies>
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.30</version>
</dependency>
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.3</version>
</dependency>
</dependencies>
Basic Configuration
Create a configuration file named logback.xml
in your resources folder. Here, you can specify how logs should be formatted and where they should be written.
<configuration>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{yyyy-MM-dd HH:mm:ss} [%thread] %-5level %logger{36} - %msg%n</pattern>
</encoder>
</appender>
<root level="debug">
<appender-ref ref="STDOUT" />
</root>
</configuration>
This configuration enables you to see logs on the console with a timestamp, thread name, log level, logger name, and message.
Getting Started with MDC
To utilize MDC, you need to import the MDC
class from SLF4J:
import org.slf4j.MDC;
Example: Using MDC
In this example, we will set up a simple user identification for our logging context.
public void processUserRequest(String userId) {
// Adding user ID to the Mapped Diagnostic Context
MDC.put("userId", userId);
try {
log.info("Processing request for user.");
// Business logic goes here
} catch (Exception e) {
log.error("Error processing request for user.", e);
} finally {
// Clearing MDC after the operation is complete to prevent memory leaks
MDC.clear();
}
}
Why Use MDC in This Example?
-
User Identifier: We store the
userId
in MDC, allowing any subsequent log messages within thetry
block to include user-specific context. -
Error Handling: In case of an exception, the logs produced will clearly indicate which user's request has failed.
-
Clear Context: Always clear the MDC after the operation. This practice avoids scenarios where old context information remains in the MDC, leading to unexpected log entries.
Log Output with MDC
When this method executes, log entries will include the userId
as part of their context:
2023-10-10 12:00:00 [main] INFO com.example.MyService - Processing request for user.
HTTP Request Context and MDC
In a web application, it’s common to use MDC to track requests and responses. This scenario typically arises with Java EE applications, where you need to log each HTTP request's context – like the request ID, user agent, and request URL.
Example: Logging HTTP Request Details
Imagine you have a servlet that processes web requests:
@WebServlet("/api/users")
public class UserServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// Adding request-specific information to MDC
MDC.put("requestId", request.getHeader("Request-ID"));
MDC.put("userAgent", request.getHeader("User-Agent"));
try {
log.info("Handling GET request for users.");
// Retrieve users and back to the client
} catch (Exception e) {
log.error("Error handling request.", e);
} finally {
// Always clear MDC
MDC.clear();
}
}
}
Why Capture HTTP Context?
-
Unique Identification: Each request can be uniquely identified using the
requestId
, making it easier to track down issues in asynchronous environments. -
User Experience Analysis: Including the
userAgent
can help developers understand which devices and browsers are most commonly used by your users.
Advanced Use: Propagating MDC Across Threads
One challenge in managing MDC is ensuring that context is preserved across asynchronous calls or multi-threaded executions. Using libraries like CompletableFuture, MDC context can be manually propagated for each task.
Example: Preserving MDC Context for Asynchronous Calls
ExecutorService executor = Executors.newFixedThreadPool(3);
public void handleRequest(String userId) {
MDC.put("userId", userId);
try {
executor.submit(() -> {
// Retaining MDC context in this lambda
try {
log.info("Processing user request in a separate thread.");
} finally {
// Clear MDC to avoid context leakage
MDC.clear();
}
});
} finally {
MDC.clear(); // Always clear MDC on the main thread too
}
}
Lessons Learned
The Mapped Diagnostic Context is a crucial feature for enhancing your Java EE application's logging capabilities. By providing contextual information in log entries, it simplifies debugging and improves performance monitoring.
Key Takeaways
- Contextual Enrichment: Ensure that logging in a multi-thread and web-context is enriched by contextualizing logs with MDC.
- Memory Management: Always remember to clear the MDC to prevent memory leaks and cross-thread contamination.
- Asynchronous Support: Use techniques such as propagating MDC context for asynchronous tasks to maintain clarity in your application's logging.
For further reading, consider exploring SLF4J Documentation and Logback Documentation. Happy logging!