Common Pitfalls in Thread Execution Mastery
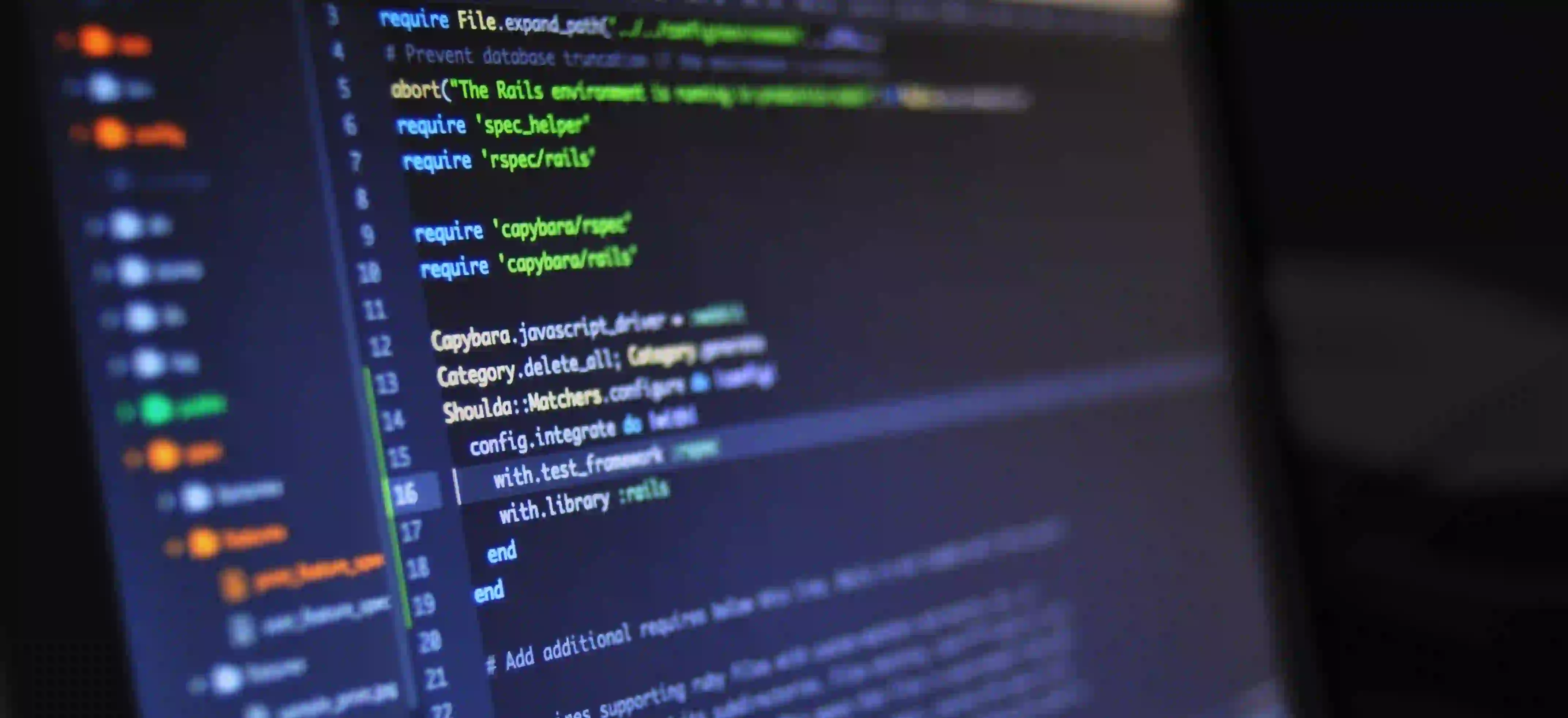
Common Pitfalls in Thread Execution Mastery
Thread execution is at the heart of modern programming, allowing developers to create efficient, responsive applications. Whether developing for desktop, web, or mobile, understanding threading and its challenges is critical for performance optimization. However, mastering thread execution often comes with its own set of pitfalls. In this blog post, we'll explore some of the most common pitfalls in thread execution, along with actionable insights on avoiding these traps.
Why Threads Are Important
Threads allow multiple tasks to run concurrently. This is particularly important for:
- Responsiveness: By handling multiple operations simultaneously, applications can remain responsive to user input.
- Resource Utilization: Modern processors have multiple cores, enabling better use of CPU resources.
- Performance: Properly managed threads can significantly reduce the overall execution time of applications.
However, as straightforward as threading may seem, it's fraught with challenges. Let’s delve into the common pitfalls and how to navigate them effectively.
1. Race Conditions
The Problem
Race conditions occur when multiple threads access shared resources simultaneously. This can lead to unpredictable results, making your application behave inconsistently.
Example
Let's take a look at a simple example:
class Counter {
private int count = 0;
public void increment() {
count++;
}
public int getCount() {
return count;
}
}
In this example, if multiple threads access the increment
method concurrently, you might not get the expected value in getCount()
.
The Solution
To avoid race conditions, we can synchronize access to the critical section of the code:
class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public synchronized int getCount() {
return count;
}
}
Using the synchronized
keyword ensures that only one thread can execute a method at a time, maintaining consistent state across threads. Read more about Java synchronization here.
2. Deadlocks
The Problem
A deadlock occurs when two or more threads are blocked forever, waiting for each other to release resources. This can bring your application to a standstill.
Example
Imagine the scenario below where two threads are trying to acquire locks on two resources:
class Resource {
public synchronized void lock1(Resource resource) {
System.out.println(Thread.currentThread().getName() + " acquired lock on resource 1.");
resource.lock2();
}
public synchronized void lock2() {
System.out.println(Thread.currentThread().getName() + " acquired lock on resource 2.");
}
}
If two threads call lock1
on two separate Resource
instances, they could end up in a deadlock situation.
The Solution
- Lock Ordering: Always acquire locks in a consistent order.
- Timeouts: Implementing timeouts can help in avoiding deadlocks.
Example of lock ordering:
class Resource {
public synchronized void lock1(Resource resource) {
System.out.println(Thread.currentThread().getName() + " acquired lock on resource 1.");
resource.lock2(this); // Always sends 'this' to prevent deadlock
}
public synchronized void lock2(Resource resource) {
System.out.println(Thread.currentThread().getName() + " acquired lock on resource 2.");
}
}
For further details on managing deadlocks, explore this resource.
3. Resource Starvation
The Problem
Resource starvation occurs when a thread is perpetually denied access to resources it needs to proceed. This can result in unresponsive applications where critical processes are stalled indefinitely.
Example
If higher-priority threads continuously preempt lower-priority ones, the latter may never get the chance to run.
The Solution
- Thread Priority: Ensure that thread priority is set sensibly.
- Fair Queuing: Implement fair scheduling techniques.
Utilizing Fair Locks
You can create and use a fair lock with Java's ReentrantLock
:
import java.util.concurrent.locks.ReentrantLock;
class FairLock {
private final ReentrantLock lock = new ReentrantLock(true); // Fair lock
public void doSomething() {
lock.lock();
try {
// Protected code
} finally {
lock.unlock();
}
}
}
Learn more about ReentrantLock
here.
4. Thread Leaks
The Problem
Thread leaks occur when threads are not properly terminated or reused. Over time, this can consume considerable CPU and memory resources, negatively impacting the application’s performance.
The Solution
- Thread Pools: Instead of creating new threads, use a Thread Pool.
- Proper Shutdown: Ensure that threads are properly interrupted or terminated upon application completion.
Example Using ExecutorService
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
private final ExecutorService executorService = Executors.newFixedThreadPool(5);
public void executeTask(Runnable task) {
executorService.submit(task);
}
public void shutdown() {
executorService.shutdown();
}
}
Using ExecutorService
helps manage thread lifecycles elegantly. For more on thread management, consider this article.
5. Lack of Thread Safety in Collections
The Problem
Some Java collections are not thread-safe. Using them across multiple threads without proper synchronization can lead to inconsistencies and exceptions.
Solution
Use concurrent collections such as ConcurrentHashMap
or CopyOnWriteArrayList
to ensure thread safety.
Example
import java.util.concurrent.ConcurrentHashMap;
class ConcurrentExample {
private final ConcurrentHashMap<String, Integer> map = new ConcurrentHashMap<>();
public void update(String key, Integer value) {
map.put(key, value);
}
public Integer get(String key) {
return map.get(key);
}
}
For more information about Java Collections and thread safety, check out the Javadoc for Collections.
To Wrap Things Up
Threading is essential, but it comes with its own challenges. Awareness of the common pitfalls—race conditions, deadlocks, resource starvation, thread leaks, and insensitivity in collections—will empower you to write better, more reliable concurrent Java applications.
Through proper techniques such as synchronization, fair locking, using thread pools, and leveraging concurrent collections, developers can avoid these pitfalls and enhance performance.
For further learning, consider exploring the Java Concurrency tutorial on the official Oracle website.
By understanding and mastering thread execution, you position yourself for success in the fast-paced world of programming. Happy coding!