Common Spring Boot Data JPA Mistakes Every Beginner Makes
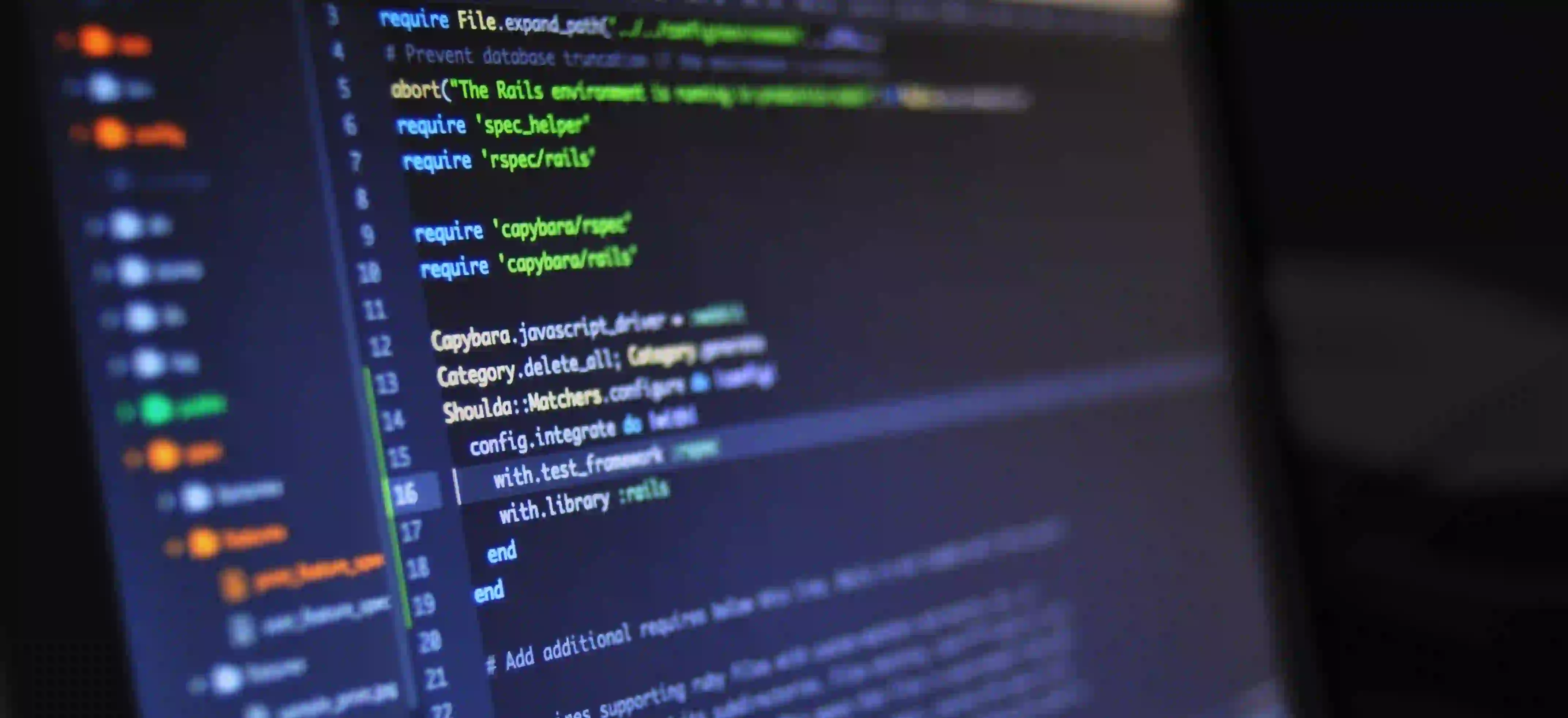
Common Spring Boot Data JPA Mistakes Every Beginner Makes
Spring Boot has revolutionized the way Java developers create applications. Data JPA makes it even easier by providing an abstraction layer over traditional database interactions. However, beginners often stumble upon common pitfalls that can lead to inefficiencies and bugs. This blog post delves into these common mistakes, providing insights to help you navigate the Spring Data JPA landscape effectively.
1. Ignoring Dependency Management
The Importance of Dependencies
One of the first steps to setting up a Spring Boot project is managing dependencies. Most beginners overlook the importance of including necessary libraries for Spring Data JPA and the database they wish to connect to.
Here’s an example of what you should include in your pom.xml
if you are using Maven:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>com.h2database</groupId>
<artifactId>h2</artifactId>
<scope>runtime</scope>
</dependency>
Why is this important? Missing dependencies can prevent your application from starting correctly or lead to exceptions during runtime. Always check that the required dependencies for both Spring Data JPA and your chosen database are included.
2. Misusing Entity Annotations
Understanding Entity Annotations
In Spring Data JPA, you define your model classes with various annotations. One of the most common mistakes is either forgetting to include the @Entity
annotation or misunderstanding how it works.
Consider the following code snippet:
import javax.persistence.Entity;
import javax.persistence.Id;
@Entity
public class User {
@Id
private Long id;
private String name;
// Getters and Setters
}
The @Entity
annotation tells Spring JPA that this class should be mapped to a database table. Without it, JPA won’t manage this class as an entity, and your data won’t be persisted as intended.
3. Not Defining a Repository Interface
The Repository Pattern
A common misconception is that developers can directly interact with their entity classes without defining a repository interface. This can lead to inefficient and verbose code.
Here's how you should define a repository for your User
entity:
import org.springframework.data.jpa.repository.JpaRepository;
public interface UserRepository extends JpaRepository<User, Long> {
User findByName(String name);
}
Using JpaRepository
provides built-in CRUD operations. Additionally, you can define custom queries with minimal effort, enhancing your code's maintainability and readability.
4. Excessive Fetching of Data
Understanding Fetch Types
Another typical mistake is not understanding the concept of fetching strategies. By default, JPA uses a Lazy Loading strategy. However, if you aren't aware of this and choose to force Eager Loading indiscriminately, it can lead to performance bottlenecks.
Here's a clear example:
@Entity
public class User {
@Id
private Long id;
@OneToMany(fetch = FetchType.EAGER)
private List<Order> orders;
}
In this example, every time a User
is loaded, all their associated orders
will also be loaded. Instead, consider using FETCH_TYPE.LAZY
and only initialize your collections when necessary to optimize your application's performance.
5. Failing to Manage Transactions Properly
The Role of Transactions
In Spring Data JPA, transactions ensure that a set of operations is executed reliably. A common oversight among beginners is not annotating service methods appropriately for transaction management.
Here's how you can define a service method with transactions:
import org.springframework.stereotype.Service;
import org.springframework.transaction.annotation.Transactional;
@Service
public class UserService {
private final UserRepository userRepository;
public UserService(UserRepository userRepository) {
this.userRepository = userRepository;
}
@Transactional
public void createUser(User user) {
userRepository.save(user);
}
}
The @Transactional
annotation ensures that the method runs in a transactional context, which is crucial for maintaining data integrity.
6. Using @Transactional
at the Wrong Level
Where to Place @Transactional
Another common mistake is misunderstanding where to place the @Transactional
annotation. Many beginners place it on their repository interfaces instead of service methods.
Consider this:
@Transactional
public interface UserRepository extends JpaRepository<User, Long> {
// repository methods
}
Why is this incorrect? Placing @Transactional
on the repository layer makes it harder to manage transactions effectively. Always place it on service methods where the business logic executes.
7. Not Handling Exceptions Properly
Exception Handling Strategies
When working with JPA, exceptions such as EntityNotFoundException
or DataIntegrityViolationException
can occur. A typical oversight is failing to handle these exceptions, resulting in application crashes.
Here's how you can add exception handling to your service layer:
import org.springframework.dao.DataIntegrityViolationException;
@Service
public class UserService {
public void createUser(User user) {
try {
userRepository.save(user);
} catch (DataIntegrityViolationException e) {
// Handle data integrity violation
throw new CustomException("User already exists!");
}
}
}
Effective exception handling allows you to gracefully respond to issues, maintaining a robust application.
8. Not Optimizing Queries
The Need for Query Optimization
When beginners rely solely on auto-generated queries while ignoring optimization techniques, performance can suffer. Using the derived query method provided by Spring Data JPA can lead to unoptimized SQL.
To create explicit queries, consider using @Query
:
import org.springframework.data.jpa.repository.Query;
public interface UserRepository extends JpaRepository<User, Long> {
@Query("SELECT u FROM User u WHERE u.name = ?1")
User findUserByName(String name);
}
By creating customized queries, you can control what operations are performed, potentially improving performance.
My Closing Thoughts on the Matter
Spring Data JPA simplifies database interactions, but making common mistakes can introduce complications. Understanding how to handle dependencies, entity annotations, repository patterns, fetching strategies, transactions, exceptions, and query optimizations is vital for leveraging the full power of Spring Data JPA.
For further reading on enhancing your knowledge of Spring Boot and JPA, check out Spring Data JPA Documentation and Baeldung's Spring Boot Guide.
By avoiding these common pitfalls and understanding the 'why' behind each aspect, you can build efficient, performant, and maintainable applications with Spring Boot Data JPA. Happy coding!