Choosing the Wrong Hashing Strategy: Common Pitfalls Explained
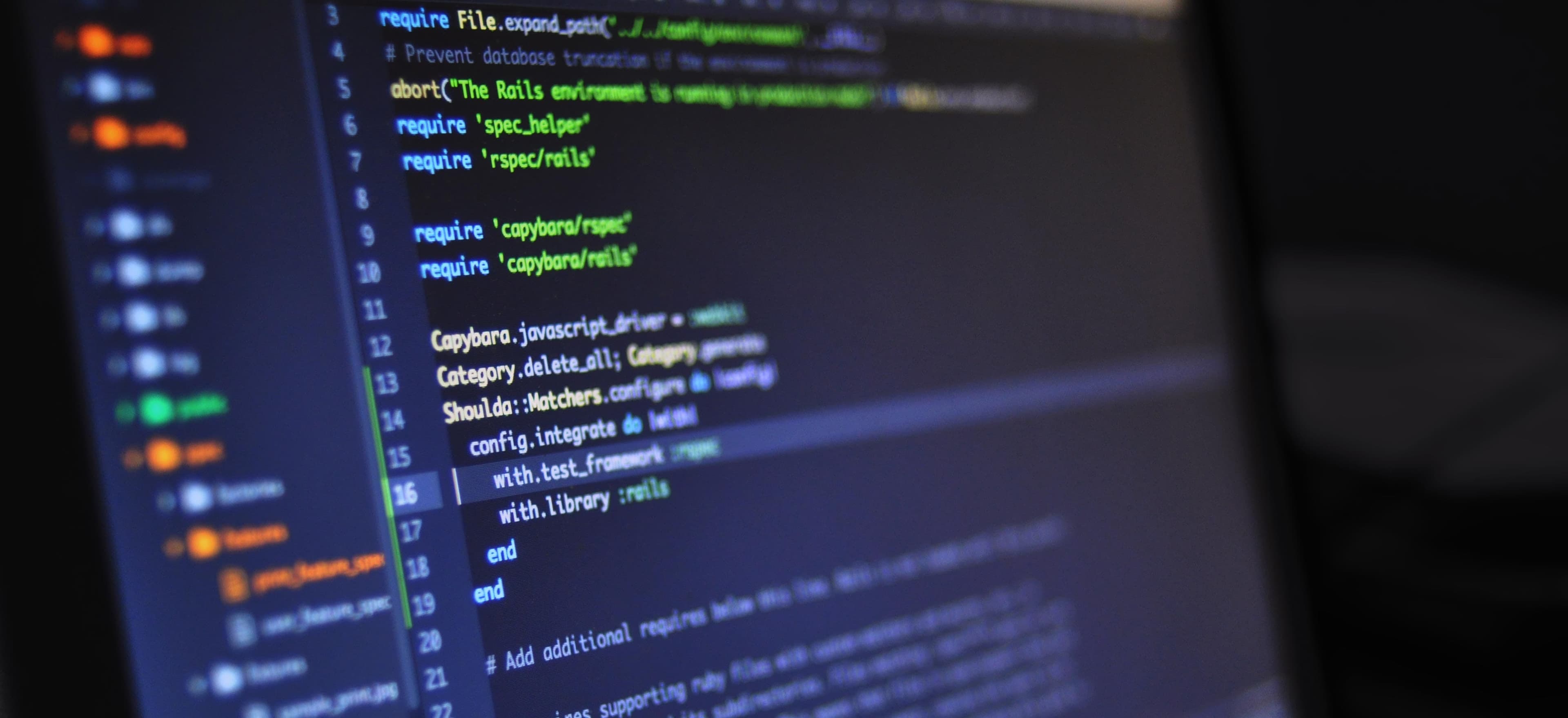
- Published on
Choosing the Wrong Hashing Strategy: Common Pitfalls Explained
As developers, we often grapple with the complexities of data storage and retrieval in our applications. One of the cornerstones of efficient data management is hashing. Hashing improves data retrieval speeds and ensures data integrity, but choosing the right hashing strategy is crucial. In this blog post, we will explore common pitfalls associated with hashing strategies, provide illustrative Java examples, and highlight best practices for optimal implementation.
Understanding Hashing
Hashing is the process of transforming input data (or keys) into a fixed-size string of bytes. The output, called the hash value or hash code, is typically much shorter than the input data. This technique is widely employed in various applications, including data structures like hash tables, password storage, and digital signatures.
When selecting a hashing strategy, one must understand the hashing algorithm's pros and cons. Common algorithms include MD5, SHA-1, and SHA-256. However, some may lead to significant pitfalls, which we will explore below.
Common Pitfalls in Hashing Strategies
1. Not Considering Collisions
When two distinct inputs produce the same hash value, it is termed a collision. Collisions can compromise the integrity of a data storage system and lead to unpredictable behavior.
Example:
import java.util.HashMap;
public class CollisionExample {
public static void main(String[] args) {
HashMap<String, String> map = new HashMap<>();
map.put("key1", "value1");
map.put("key2", "value2");
// Collision - two keys yielding the same hash value
System.out.println("Key1's Hash: " + map.get("key1").hashCode());
System.out.println("Key2's Hash: " + map.get("key2").hashCode());
}
}
When using a basic hash function like hashCode()
in Java, the chance of collision increases, especially with many elements in a collection. To mitigate this, employing a stronger hashing algorithm that distributes outputs more uniformly is important.
2. Using Insecure Hash Functions
Some hashing algorithms are no longer considered secure. For example, MD5 and SHA-1 have known vulnerabilities and should be avoided for cryptographic purposes.
Example:
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class InsecureHashExample {
public static void main(String[] args) {
String password = "mySecretPassword";
String hash = hashWithMD5(password);
System.out.println("MD5 Hash: " + hash);
}
public static String hashWithMD5(String input) {
try {
MessageDigest md = MessageDigest.getInstance("MD5");
byte[] messageDigest = md.digest(input.getBytes());
StringBuilder sb = new StringBuilder();
for (byte b : messageDigest) {
sb.append(String.format("%02x", b));
}
return sb.toString();
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException(e);
}
}
}
Using MD5 is a common pitfall for applications that require rigorous security, such as password storage. Instead, utilize a more secure hashing algorithm like SHA-256.
3. Overlooking Performance
Choosing the wrong hashing strategy can lead to poor performance. While security is critical, hashing speed should not be compromised, especially in applications requiring real-time data processing.
Example:
import java.util.HashMap;
public class PerformanceExample {
public static void main(String[] args) {
HashMap<Integer, String> inefficientMap = new HashMap<>(1000);
for (int i = 0; i < 100000; i++) {
inefficientMap.put(i, "value" + i);
}
System.out.println("Map Size: " + inefficientMap.size());
}
}
In this code, a HashMap is initialized without proper sizing that accounts for expected load, leading to increased hash collisions and degraded performance. Always estimate the number of entries to improve performance.
Best Practices for Hashing
Now that we've covered some common pitfalls, let's discuss best practices for effective hashing strategies.
1. Use Strong Hashing Algorithms
Prioritize secure hashing algorithms like SHA-256 or SHA-512 for applications dealing with sensitive data. For more robust implementations, consider using libraries like Bouncy Castle to enhance security.
Example: Secure Hashing
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class SecureHashExample {
public static void main(String[] args) {
String password = "mySecretPassword";
String hash = hashWithSHA256(password);
System.out.println("SHA-256 Hash: " + hash);
}
public static String hashWithSHA256(String input) {
try {
MessageDigest md = MessageDigest.getInstance("SHA-256");
byte[] messageDigest = md.digest(input.getBytes());
StringBuilder sb = new StringBuilder();
for (byte b : messageDigest) {
sb.append(String.format("%02x", b));
}
return sb.toString();
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException(e);
}
}
}
2. Implement Salting and Peppering
When storing passwords, always consider salting (adding a random value to a password before hashing) and optionally peppering (adding a secret value to the hash). This further mitigates the risk of rainbow table attacks.
3. Optimize Load Factors and Rehashing
Selecting an appropriate load factor for hash tables ensures performance is kept optimum. Generally, a load factor of 0.75 is recommended, blending balance between time complexity and space efficiency.
4. Test for Collisions
Regularly test your hashing strategy for collisions using statistical methods. Tools that analyze hashes can help in this regard, identifying weaknesses in the chosen algorithm.
A Final Look
Choosing the right hashing strategy is pivotal for maintaining data integrity and security. The common pitfalls discussed here illustrate the various dimensions—collisions, security vulnerabilities, and performance—that developers need to consider. Implementing the best practices outlined will guide you toward a robust hashing strategy that serves your application's needs effectively.
Remember, in the world of development, it is often the small details—like a well-chosen hashing algorithm—that can make a world of difference. For further reading, check out resources like OWASP's Password Storage Cheat Sheet to dive deeper into effective hashing practices.
If you have any questions or would like to share your experiences with hashing strategies, feel free to leave a comment below!