Handling Event Duplication with Google Guava EventBus
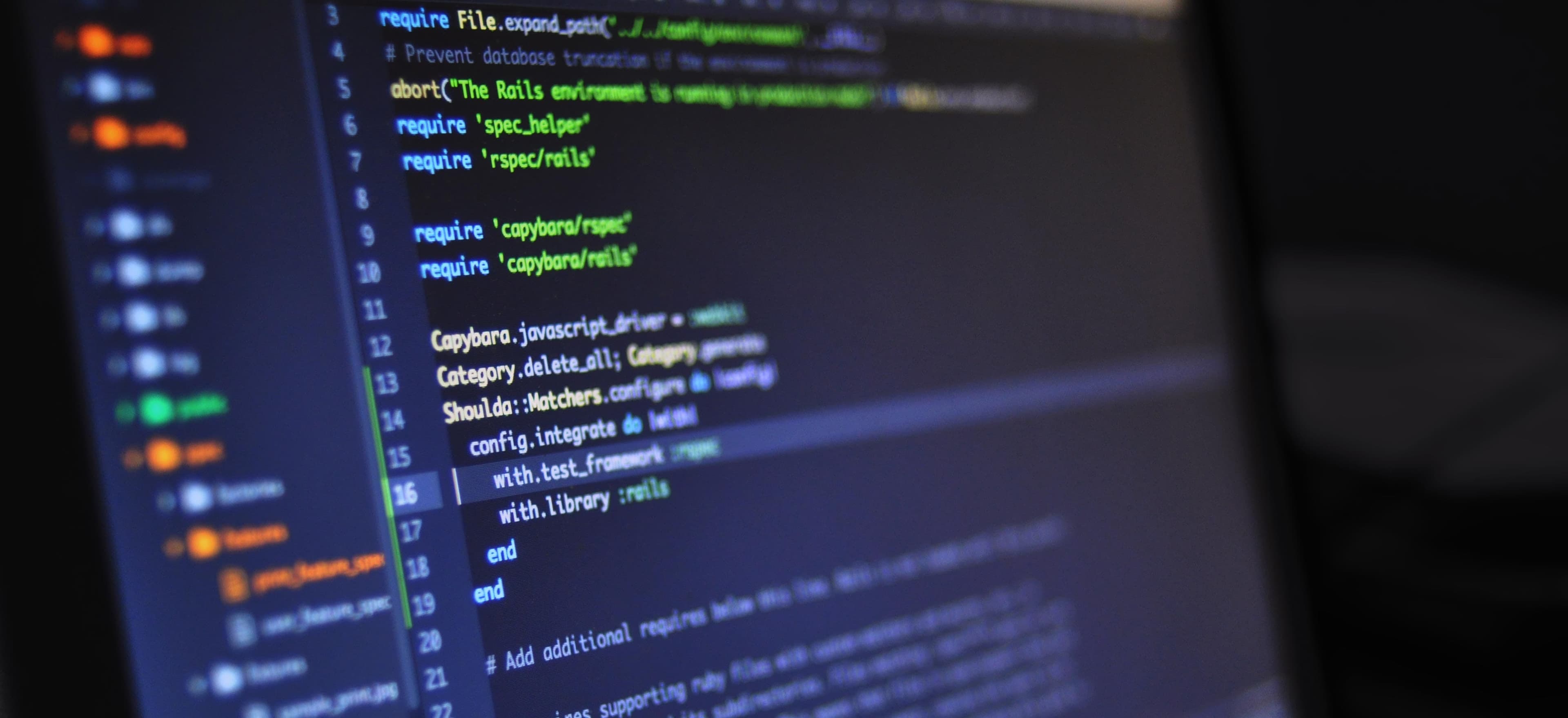
- Published on
Handling Event Duplication with Google Guava EventBus
Events are a central aspect of many Java applications, from user interactions in a GUI to responding to system-level changes. A common challenge in event-driven programming is event duplication, where the same event might be fired multiple times, leading to inconsistent states or unintended behaviors in your application. Google Guava provides a powerful tool for managing events through its EventBus system, which helps in decoupling the sender and receiver of events.
In this blog post, we'll explore how to handle event duplication using Google Guava's EventBus. We'll discuss the basics of EventBus, why event duplication can be problematic, and how to prevent it with practical examples.
Understanding Google Guava EventBus
Google Guava's EventBus is a simple and flexible publish-subscribe framework that allows for communication between components without tight coupling. It promotes a clear separation of concerns in your application architecture.
Basic Concepts of EventBus
- Event: An object that represents some occurrence in your application.
- Subscriber: An object that listens for specific events.
- Publisher: An object that sends events to the EventBus.
Example of a Simple EventBus Setup
To illustrate the basic setup of EventBus, let's first create a simple event and subscriber.
import com.google.common.eventbus.EventBus;
import com.google.common.eventbus.Subscribe;
class MyEvent {
private final String message;
MyEvent(String message) {
this.message = message;
}
String getMessage() {
return message;
}
}
class MyEventListener {
@Subscribe
public void handleEvent(MyEvent event) {
System.out.println("Event received: " + event.getMessage());
}
}
public class EventBusExample {
public static void main(String[] args) {
EventBus eventBus = new EventBus();
MyEventListener listener = new MyEventListener();
// Register the event listener with the EventBus
eventBus.register(listener);
// Publish an event
eventBus.post(new MyEvent("Hello, EventBus!"));
}
}
Why Use EventBus?
Using EventBus decouples the logic of event production and event handling. It allows for:
- Improved maintainability of code
- Easier testing of components
- Dynamic registration of subscribers
However, even with these advantages, event duplication can lead to unexpected behavior, making it essential to implement safeguards against it.
The Problem of Event Duplication
What Causes Event Duplication?
Event duplication can occur for various reasons:
- Multiple Event Listeners: If multiple subscribers are registered to listen for the same event without duplicate checks, each listener will react to the event.
- Retried Events: In scenarios where an event's processing fails, it may be retried, leading to the same event being handled multiple times.
- Concurrent Executions: In multi-threaded applications, a single event may be posted multiple times due to threading issues.
Consequences of Event Duplication
- Inconsistent Data States: Repeatedly processing the same event can lead to state corruption where the system is left in an undefined or incorrect state.
- Performance Issues: Handling the same event multiple times can lead to unnecessary resource consumption and reduce application performance.
Strategies for Handling Event Duplication
Here, we will discuss some strategies to effectively manage event duplication using Google Guava's EventBus.
1. Using a Unique Event ID
One effective method to handle event duplication is by assigning a unique identifier to each event. By maintaining a record of processed event IDs, you can ignore duplicates.
import com.google.common.eventbus.EventBus;
import com.google.common.eventbus.Subscribe;
import java.util.HashSet;
import java.util.Set;
class UniqueEvent {
private final String id;
private final String message;
UniqueEvent(String id, String message) {
this.id = id;
this.message = message;
}
String getId() {
return id;
}
String getMessage() {
return message;
}
}
class UniqueEventListener {
private final Set<String> processedEventIds = new HashSet<>();
@Subscribe
public void handleUniqueEvent(UniqueEvent event) {
if (!processedEventIds.contains(event.getId())) {
processedEventIds.add(event.getId());
System.out.println("Processing unique event: " + event.getMessage());
} else {
System.out.println("Duplicate event ignored: " + event.getId());
}
}
}
public class UniqueEventBusExample {
public static void main(String[] args) {
EventBus eventBus = new EventBus();
UniqueEventListener listener = new UniqueEventListener();
eventBus.register(listener);
// Post unique event
eventBus.post(new UniqueEvent("1", "First event"));
eventBus.post(new UniqueEvent("1", "First event again")); // Duplicate
eventBus.post(new UniqueEvent("2", "Second event"));
}
}
Why This Works
In the above code:
- We define an event class,
UniqueEvent
, that carries an ID. - The listener maintains a
Set
of processed event IDs. - During event handling, it checks if the event ID is already present, thus preventing duplicate processing.
This approach works well in scenarios where events can be uniquely identified.
2. Event Throttling
Another strategy is event throttling, which limits the rate at which events are processed. This ensures your system doesn't get overwhelmed by multiple rapid-fire events.
import com.google.common.eventbus.EventBus;
import com.google.common.eventbus.Subscribe;
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
class ThrottleEvent {
private final String message;
ThrottleEvent(String message) {
this.message = message;
}
String getMessage() {
return message;
}
}
class ThrottleEventListener {
private final ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
private volatile boolean processing = false;
@Subscribe
public void handleThrottleEvent(ThrottleEvent event) {
if (!processing) {
processing = true;
System.out.println("Processing: " + event.getMessage());
// Reset processing flag after a delay
scheduler.schedule(() -> processing = false, 1, TimeUnit.SECONDS);
} else {
System.out.println("Event throttled: " + event.getMessage());
}
}
}
public class ThrottleEventBusExample {
public static void main(String[] args) {
EventBus eventBus = new EventBus();
ThrottleEventListener listener = new ThrottleEventListener();
eventBus.register(listener);
// Post multiple events in quick succession
eventBus.post(new ThrottleEvent("Event 1"));
eventBus.post(new ThrottleEvent("Event 2"));
eventBus.post(new ThrottleEvent("Event 3"));
}
}
Why Throttling is Effective
In this example:
- We use a
ScheduledExecutorService
to introduce a processing delay. - If another event arrives within the throttle period, it is ignored.
This technique is useful in scenarios where excessive event processing can lead to poor performance.
Key Takeaways
Handling event duplication is crucial for maintaining the integrity of your Java applications, especially when leveraging powerful tools like Google Guava's EventBus. By implementing strategies such as unique event IDs and event throttling, you can ensure robust event handling that anticipates and mitigates the potential pitfalls of event-driven programming.
For more information on Google Guava, check out the official Guava documentation. These safeguards can significantly improve your application's reliability and performance by streamlining event handling.
If you have any further questions or want to share your experiences with event handling in Java, feel free to leave a comment below!