How GC Affects Throughput and Latency in Your Applications
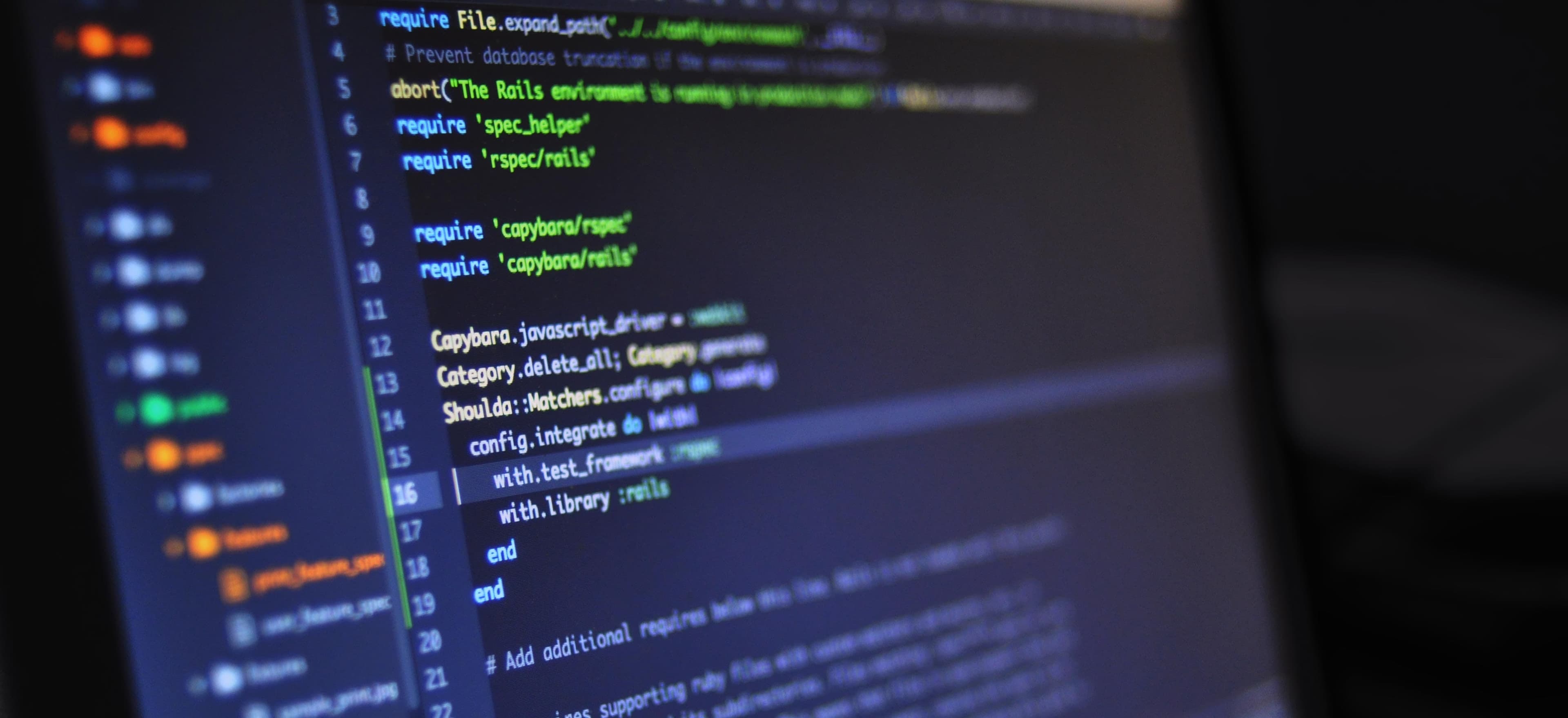
- Published on
How GC Affects Throughput and Latency in Your Applications
In today's fast-paced software world, application performance is critical for user satisfaction and business success. One of the most significant factors affecting performance in Java applications is garbage collection (GC). In this blog, we will explore how GC affects throughput and latency, and we'll look at strategies you can employ to optimize your Java applications.
Understanding Garbage Collection
Garbage collection is Java's automatic memory management mechanism, responsible for reclaiming memory allocated to objects that are no longer needed. This process helps avoid memory leaks and overconsumption of memory resources, ensuring that your applications run smoothly.
While GC is essential for memory management, it introduces two primary concerns:
-
Throughput: This is the amount of work done by the application in a given time, usually expressed as requests per second. Essentially, higher throughput indicates that the system is processing more transactions.
-
Latency: This is the time taken to process a single request. In contexts where user experience is crucial, such as web applications, lower latency is more desirable.
Understanding the trade-off between throughput and latency is paramount for optimal performance.
The Garbage Collection Process
Garbage collection in Java primarily follows these stages:
- Marking: The GC identifies which objects are still in use.
- Sweeping: Memory occupied by unreferenced objects is reclaimed.
- Compacting: The remaining objects are moved closer together to free up contiguous memory.
While this process is essential, it can also be resource-intensive, leading to potential performance degradation. When the GC runs, it can pause the application, causing latency spikes.
Example Code Snippet
Here is a basic example showcasing object creation and how it might impact GC:
import java.util.ArrayList;
public class GarbageCollectionExample {
public static void main(String[] args) {
ArrayList<String> items = new ArrayList<>();
for (int i = 0; i < 1_000_000; i++) {
items.add("Item " + i);
}
System.out.println("Items created.");
// Explicitly clearing the list to help the GC
items.clear();
// Request the JVM to run the garbage collector
System.gc();
System.out.println("Garbage collection requested.");
}
}
Commentary on the Example
- ArrayList Creation: We create a large number of objects in the loop. This increases the workload on the GC, as it needs to track and eventually clean up these objects.
- Clear Method: By calling
items.clear()
, we ensure that we no longer reference the items, allowing them to be eligible for garbage collection. - Manual GC Request: The call to
System.gc()
is an explicit request for garbage collection. Though it is not guaranteed that the GC will run, it signals the JVM that there is memory to be collected.
GC Algorithms and Their Impact
Java offers several garbage collection algorithms, each with unique characteristics that affect throughput and latency differently:
-
Serial GC: This is the simplest GC algorithm, using a single thread for garbage collection. It is best for small applications with low memory footprints.
- Throughput: Good, as it minimizes overhead during collection.
- Latency: Poor, as application pauses can be significant when the GC runs.
-
Parallel GC: Employing multiple threads for garbage collection, this algorithm is suitable for multi-core processors.
- Throughput: Improved over Serial because it can reclaim memory faster.
- Latency: Better than Serial but still can lead to pauses during collection.
-
Concurrent Mark-Sweep (CMS): This algorithm minimizes pauses by performing the marking and sweeping phases concurrently with application threads.
- Throughput: Moderate, as it attempts to strike a balance between performance and pause times.
- Latency: Superior to Serial and Parallel, making it a good fit for latency-sensitive applications.
-
G1 Garbage Collector: Redesigning the garbage collection process, G1 efficiently handles larger heaps and aims for predictable latency.
- Throughput: Generally excellent in larger environments.
- Latency: Configurable, allowing for specific pause time goals to be set, making it ideal for real-time applications.
Example Usage of G1 GC
To utilize the G1 Garbage Collector, you can configure the JVM as follows:
java -XX:+UseG1GC -Xms1G -Xmx4G -jar YourJavaApp.jar
- -XX:+UseG1GC: This flag enables the G1 Garbage Collector.
- -Xms and -Xmx: These flags define the initial and maximum heap sizes, which can affect GC behavior.
Impact on Throughput and Latency
Throughput vs. Latency - A Balancing Act
The choice of the garbage collection algorithm has a direct impact on both throughput and latency.
-
In scenarios where high throughput is essential, you might lean towards Parallel GC. This algorithm quickly processes bulk loads but can have higher pause times.
-
Conversely, if latency is a significant concern, G1 or CMS is likely the better choice, providing lower pause times but possibly at the cost of throughput.
Best Practices to Optimize GC Performance
-
Tune JVM Parameters: Adjusting settings like heap size and GC algorithm can yield significant improvements. Experiment with various parameters to find the best fit for your application’s workload.
-
Avoid Rapid Object Creation: Reduce the creation of unnecessary objects to lower GC overhead. For frequently used objects, use a pool.
-
Monitor GC Activity: Utilize tools like VisualVM or Java Mission Control to monitor GC activity and application performance in real-time.
-
Profiling: Regularly profile your application with tools like YourKit or Eclipse Memory Analyzer to understand memory allocation patterns better.
-
Analyze GC Logs: Enable GC logging to get insights into the frequency and duration of GC pauses. Monitoring tools can use these logs to provide actionable insights.
Example Code: Enabling GC Logging
To enable GC logging, run your application with these JVM flags:
java -Xlog:gc*:file=gc.log -jar YourJavaApp.jar
This will output detailed GC information to gc.log
, which you can analyze later.
Final Thoughts
Garbage collection is a critical aspect of memory management in Java applications. Understanding its impact on throughput and latency is vital for developing high-performing applications.
By choosing the right GC algorithm, tuning the JVM appropriately, and employing best practices, you can significantly enhance your application's performance. Remember, performance optimization is an ongoing process, and regular monitoring and adjustments can lead to substantial improvements.
For further reading on garbage collection tuning and performance optimization strategies, consider exploring the Oracle Java Documentation.
By following the guidelines outlined in this article, you will be well on your way to mastering the complexities of garbage collection and optimizing your Java applications for the best possible performance.
Checkout our other articles