Unlocking the Complexity of Spring Integration: A Guide
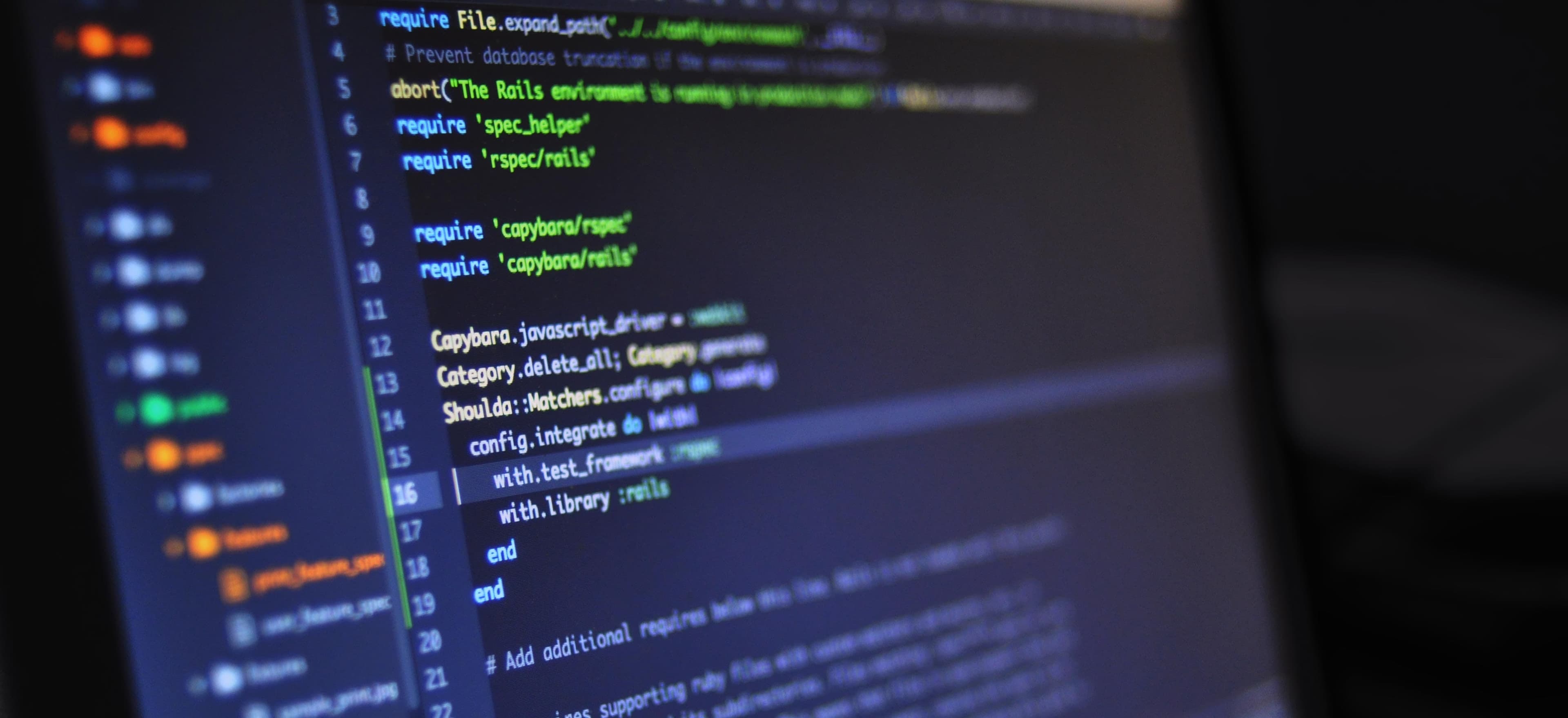
- Published on
Unlocking the Complexity of Spring Integration: A Guide
Spring Integration is a powerful framework that provides support for building enterprise integration solutions. It allows Java developers to implement complex integration patterns and create applications that can connect various systems through various protocols. In this blog post, we will unravel the complexity of Spring Integration, explore its core components, and provide practical examples with code snippets to illustrate how you can leverage it in your applications.
What Is Spring Integration?
Spring Integration is part of the Spring Framework suite. Its primary goal is to provide a lightweight framework for building integration solutions in a Spring context. It extends the Spring programming model to support the application of enterprise integration patterns (EIPs). By using Spring Integration, developers can easily orchestrate messaging, routing, and processing of data across different systems and formats.
Key Components of Spring Integration
Spring Integration is comprised of several key components. Understanding these components will help you develop and maintain integration solutions more efficiently.
1. Channels
Channels are the conduits through which messages travel. Spring Integration supports several types of channels, including:
- Direct Channel: A simple channel that sends messages directly from one component to another.
- Publish-Subscribe Channel: Allows multiple subscribers to receive messages from one publisher.
Here's a simple example of a direct channel configuration:
@Bean
public DirectChannel directChannel() {
return new DirectChannel();
}
In the example above, we create a direct channel named directChannel
. The 'DirectChannel' is synchronous, meaning the sender will wait until the receiver processes the message.
2. Messages
In Spring Integration, everything revolves around messages. A message is essentially a data payload combined with metadata (headers). Creating and sending messages is fundamental to the functionality of Spring Integration.
Here's a basic example of a message:
Message<String> message = MessageBuilder.withPayload("Hello, Spring Integration!")
.setHeader("Source", "Example")
.build();
This code snippet demonstrates how to build a message with a payload of type String along with a header. Here we see the combination of data and metadata, ensuring proper handling of the message.
3. Endpoints
Endpoints are the components that interact with channels. They can perform various actions based on the messages they receive or send. There are several types of endpoints, including:
- Service Activators: Components that process messages.
- Message Gateways: Interfaces for sending and receiving messages from external systems.
Here is a simple example of a service activator:
@ServiceActivator(inputChannel = "directChannel")
public void processMessage(String message) {
System.out.println("Received message: " + message);
}
In this example, the service activator listens for messages on the directChannel
and prints the received message. This encapsulates the process of message handling clearly and concisely.
4. Routers
Routers are used to route messages to different channels based on certain conditions. This is beneficial for handling various workflows within your application.
Here is an example of a simple router:
@Router(inputChannel = "directChannel")
public MessageChannel route(Message<?> message) {
// Logic to route messages to different channels based on conditions
if (message.getPayload().equals("Important")) {
return importantChannel();
}
return regularChannel();
}
In this code, we evaluate the message payload to determine the next channel for routing. Conditional logic plays a crucial role in directing the flow of messages.
Integrating with External Systems
Spring Integration shines when it comes to connecting with external systems. It supports various protocols such as HTTP, JMS, FTP, and even WebSockets. Below, we will cover how to set up HTTP integration.
Example: HTTP Integration
First, ensure that you have the necessary dependencies in your pom.xml
or build.gradle
file for HTTP support:
<dependency>
<groupId>org.springframework.integration</groupId>
<artifactId>spring-integration-http</artifactId>
</dependency>
Next, configure an HTTP inbound gateway to listen for requests:
@Bean
public HttpRequestHandlingMessagingGateway httpGateway() {
HttpRequestHandlingMessagingGateway gateway =
new HttpRequestHandlingMessagingGateway();
gateway.setRequestChannel(directChannel());
// additional configurations if needed
return gateway;
}
By defining an HTTP request handling gateway, we can receive HTTP requests and handle them via our established directChannel
.
Example: Sending HTTP Requests
You can also send HTTP requests using Spring Integration. Here’s a simple implementation using the HTTP outbound gateway:
@Bean
public IntegrationFlow httpOutboundFlow() {
return IntegrationFlows.from("httpOutboundChannel")
.handle(Http.outboundGateway("http://example.com/api")
.httpMethod(HttpMethod.POST)
.expectedResponseType(String.class))
.get();
}
In this example, messages sent to httpOutboundChannel
are processed by the HTTP outbound gateway, making an HTTP POST request to the specified URL. The expected response type is defined to handle responses properly.
Final Considerations
Spring Integration provides a flexible and powerful way to manage complex integrations in Java applications. With its various components, developers can implement messaging, routing, and transformation capabilities fluidly.
By breaking down the concepts into manageable pieces, we explored channels, messages, endpoints, and routers while providing code snippets to illustrate their functionality. You can also connect to different systems via various protocols, making Spring Integration an indispensable tool for modern enterprise applications.
To dive deeper into Spring Integration, consider reviewing the official documentation and exploring other tutorials online. Embrace the power of integration and unlock new capabilities for your Java applications!
Now that you've learned the basics, it's time to experiment with Spring Integration in your projects. Happy coding!
Checkout our other articles