Understanding Java's Volatile Modifier: Common Pitfalls
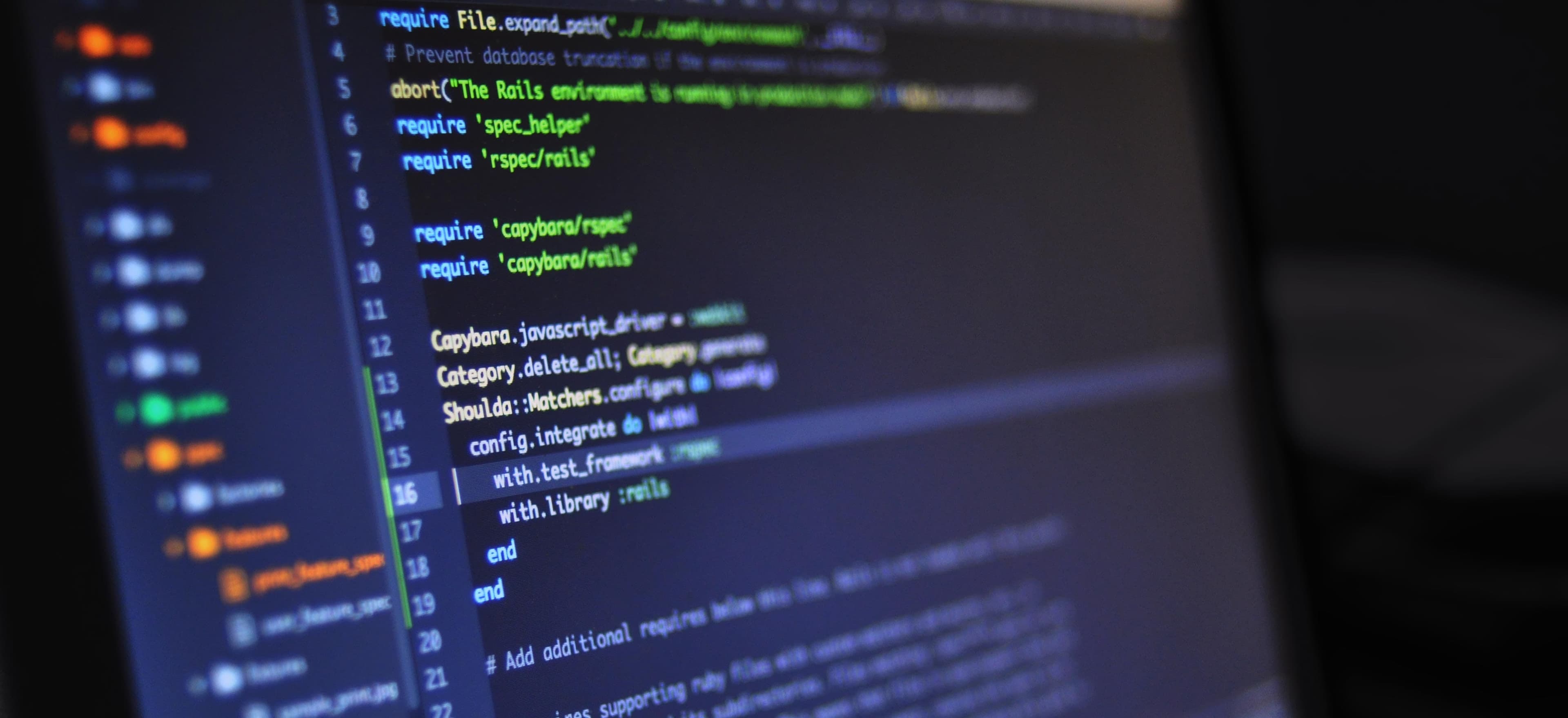
- Published on
Understanding Java's Volatile Modifier: Common Pitfalls
Java is a powerful programming language that allows developers to write efficient, multi-threaded applications. However, when it comes to shared mutable data between threads, there are certain complexities. One of the keywords that often comes up in discussions about concurrency is the volatile
modifier.
In this post, we'll dive deep into the volatile
modifier in Java, exploring how it works, common pitfalls developers face, and best practices for utilizing it effectively.
What is the Volatile Modifier?
The volatile
modifier is used in Java to indicate that a particular variable's value will be modified by different threads. When a variable is declared as volatile
, it tells the Java Virtual Machine (JVM) to always read the latest value from main memory instead of from the thread's local cache.
Here’s a simple example:
public class Example {
private volatile boolean flag = false;
public void writer() {
flag = true; // Set flag to true
}
public void reader() {
if (flag) {
// Do something
}
}
}
Why Use Volatile?
The primary parameters for using the volatile
modifier include:
- Visibility: Changes made by one thread to a
volatile
variable will be visible to other threads immediately. - Atomicity of Reads/Writes: All reads and writes to a
volatile
variable are atomic, meaning they cannot be interrupted.
While volatile
provides some guarantees, it’s not a one-size-fits-all solution for thread safety.
Common Pitfalls of Using Volatile
Now let's explore some of the most common pitfalls developers encounter when using the volatile
modifier.
1. Misunderstanding Volatile's Guarantees
Many developers mistakenly believe that declaring a variable as volatile
guarantees that compound actions are atomic. For example, consider the following code snippet:
public class Counter {
private volatile int count = 0;
public void increment() {
count++; // Not atomic
}
public int getCount() {
return count;
}
}
Despite count
being volatile, the operation count++
is not atomic. It involves reading the current value, incrementing it, and writing it back — a sequence of operations that can be interrupted by other threads.
Solution: Use AtomicInteger
for thread-safe increments.
import java.util.concurrent.atomic.AtomicInteger;
public class AtomicCounter {
private AtomicInteger count = new AtomicInteger(0);
public void increment() {
count.incrementAndGet(); // Atomic operation
}
public int getCount() {
return count.get();
}
}
2. Volatile Does Not Provide Mutual Exclusion
Another common misconception is that volatile
provides mutual exclusion. In multi-threaded applications, it’s crucial to ensure that only one thread accesses a critical section at a time. The volatile
keyword does not enforce this constraint.
For example:
public class SharedResource {
private volatile boolean flag = false;
public void changeFlag() {
flag = true; // A thread may set the flag
}
public void useResource() {
if (!flag) {
// Use the resource
}
}
}
If multiple threads execute useResource
, it could lead to inconsistent states since there is no mechanism to prevent simultaneous access.
Solution: Use synchronized blocks or locks for accessing shared resources.
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class SafeSharedResource {
private boolean flag = false;
private final Lock lock = new ReentrantLock();
public void changeFlag() {
try {
lock.lock();
flag = true; // Safe from concurrent access
} finally {
lock.unlock();
}
}
public void useResource() {
try {
lock.lock();
if (!flag) {
// Use the resource
}
} finally {
lock.unlock();
}
}
}
3. Overuse of Volatile Variables
While volatile
can solve specific visibility problems, overusing it or relying on it for thread safety can lead to unmaintainable code. In many scenarios, using volatile
might be overkill when simple local variables or thread-local storage can accomplish the task.
For instance, use volatile
only when:
- You need a flag with very little contention.
- You need to ensure visibility for a variable shared across multiple threads.
Suggestion: Aim for a balance between simplicity and performance. Use constructs designed for concurrency, such as Locks
, Executors
, or CompletableFutures
.
4. Dependency on Volatile Serialization Behavior
The volatile
keyword guarantees visibility but does not guarantee order of operations. If a particular sequence of operations is dependent on the state of a volatile
variable, the order can lead to unexpected outcomes.
class Data {
private int a = 0;
private volatile int flag = 0;
public void writer() {
a = 42; // Write a value
flag = 1; // Write to volatile
}
public void reader() {
if (flag == 1) {
// The value of 'a' might still be 0 here
System.out.println(a); // Last written value could be uninitialized
}
}
}
Solution: To ensure orderings, consider using synchronized
or other concurrency controls.
Best Practices
Prefer High-Level Abstractions
Many modern Java applications can benefit from using high-level abstractions available in the java.util.concurrent
package rather than manually managing volatile
variables. These constructs are designed to provide better scalability, ease of use, and clarity.
Understand Thread Safety Patterns
Familiarize yourself with common concurrent programming patterns and make use of them, such as:
- Producer-Consumer
- Read-Write Locks
- Thread Pools
Measure and Analyze
Always measure the performance impacts of your concurrency solutions. What works well in one scenario may not be optimal in another. Use profiling tools to understand the bottlenecks in your application.
To Wrap Things Up
The volatile
modifier is a crucial tool in the Java programmer's toolkit, but it is essential to use it wisely. Misunderstandings around its guarantees and appropriate use can lead to fragile and error-prone code.
By understanding the common pitfalls associated with volatile
, along with the best practices outlined in this post, developers can make more informed decisions about thread safety in their Java applications. For more information on concurrency in Java, checking out the Java Concurrency documentation is a great place to start.
If you have any experiences or questions about using the volatile
keyword, feel free to share in the comments! Happy coding!
Checkout our other articles