Mastering Java for Machine Learning: Common Pitfalls to Avoid
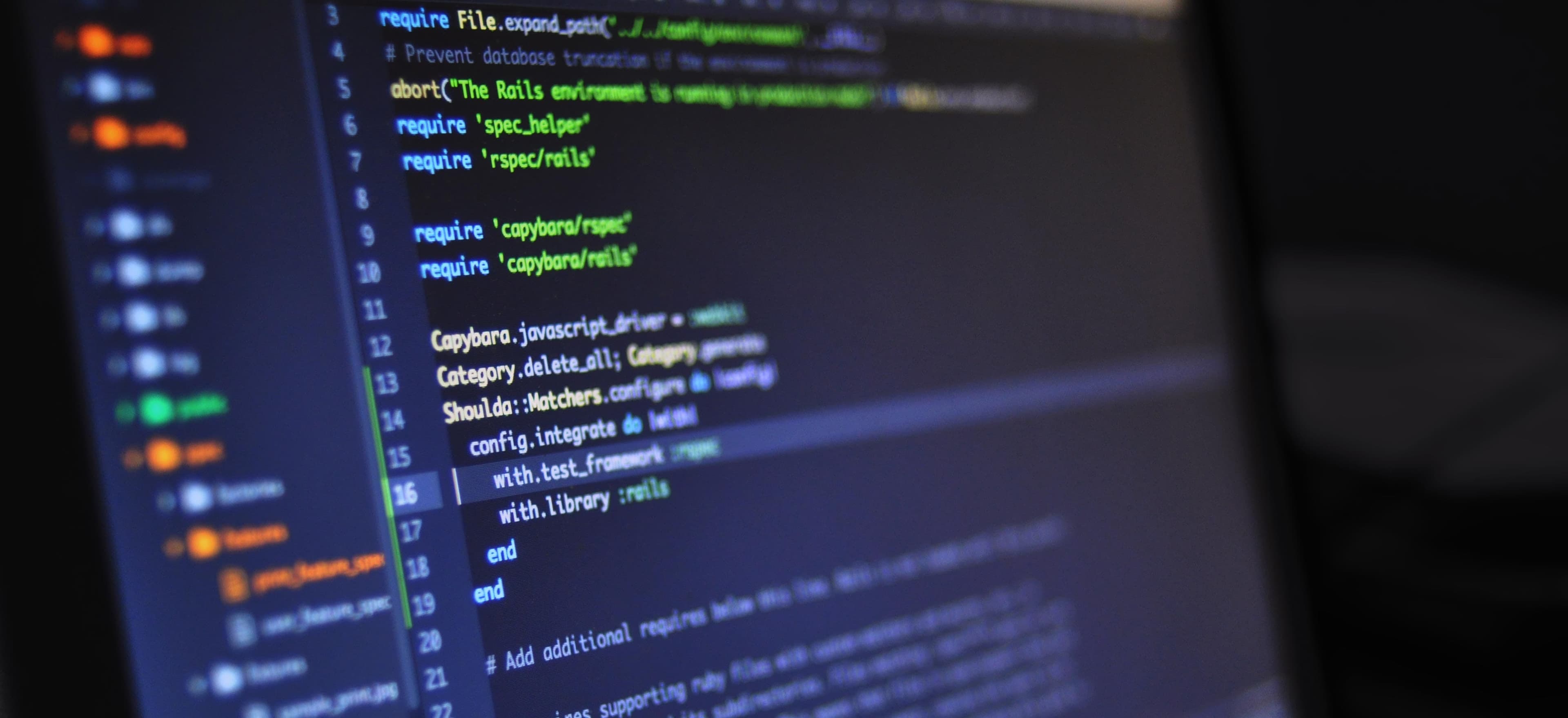
- Published on
Mastering Java for Machine Learning: Common Pitfalls to Avoid
Machine Learning (ML) is a revolutionary technology that influences various facets of our daily lives. While languages like Python and R are often the go-to for ML due to their rich ecosystem and libraries, Java remains an excellent choice thanks to its scalability, performance, and wide adoption in enterprise solutions. In this article, we will explore common pitfalls to avoid when mastering Java for machine learning, equipping you with insights that can elevate your ML projects.
Understanding Java's Role in Machine Learning
Java is a versatile programming language that provides several libraries geared toward machine learning. Libraries like Weka, Deeplearning4j, and MOA offer a robust environment conducive to machine learning development. However, as we dive deeper into these tools, the complexities of ML in Java can become overwhelming, leading us to make avoidable mistakes.
Below, we will outline common pitfalls and how to dodge them effectively.
Pitfall #1: Ignoring Object-Oriented Principles
Java is an object-oriented language, and failing to leverage its principles can render your codebase inefficient and unmanageable. Java promotes modularity, code reusability, and clean architecture which are pivotal in ML projects.
Example:
Consider the following non-object-oriented approach:
public class SimpleML {
public static void main(String[] args) {
double[] data1 = {1.0, 2.0, 3.0};
double[] data2 = {4.0, 5.0, 6.0};
double mean1 = calculateMean(data1);
double mean2 = calculateMean(data2);
// Perform further ML operations...
}
public static double calculateMean(double[] data) {
double sum = 0;
for (double value : data) {
sum += value;
}
return sum / data.length;
}
}
Why This Approach Is Limiting
This code lacks extensibility and maintainability. When you add new functionalities or algorithms, you might end up with a messy codebase. To boost your project's structure, utilize classes and objects to encapsulate functionalities.
Improved Version:
public class DataSet {
private double[] data;
public DataSet(double[] data) {
this.data = data;
}
public double calculateMean() {
double sum = 0;
for (double value : data) {
sum += value;
}
return sum / data.length;
}
}
public class MLApp {
public static void main(String[] args) {
DataSet dataset1 = new DataSet(new double[]{1.0, 2.0, 3.0});
DataSet dataset2 = new DataSet(new double[]{4.0, 5.0, 6.0});
double mean1 = dataset1.calculateMean();
double mean2 = dataset2.calculateMean();
// Perform further ML operations...
}
}
This modularizes your code, improves readability, and allows for flexibility with future data handling tools.
Pitfall #2: Overlooking the Importance of Data Preprocessing
Machine Learning thrives on quality data, and preprocessing is often the cornerstone of successful model training. However, beginners sometimes neglect this step or inadequately handle it.
Points to Consider:
- Normalization: Scale your data to ensure all features contribute equally to the model.
- Handling Missing Values: Decide whether to impute or remove missing entries. Ignoring them can skew results.
Java Snippet for Normalization:
public static double[] normalizeData(double[] data) {
double max = Arrays.stream(data).max().orElse(1);
double min = Arrays.stream(data).min().orElse(0);
double[] normalizedData = new double[data.length];
for (int i = 0; i < data.length; i++) {
normalizedData[i] = (data[i] - min) / (max - min);
}
return normalizedData;
}
Why Normalize?
Normalizing data enhances convergence rates when training algorithms, particularly when using gradient descent-based techniques.
Pitfall #3: Forgetting to Evaluate Model Performance
Model evaluation is a critical part of machine learning. Often, learners may skip proper testing, potentially leading to overfitting or underfitting.
Key Evaluation Techniques:
- Train-Test Split: Use a portion of your data to validate the model's performance.
- Cross-Validation: In cases with limited data, cross-validation provides more robust performance metrics.
Example of Train-Test Split:
import java.util.Arrays;
public class TrainTestSplit {
public static void main(String[] args) {
int totalDataPoints = 100;
double[][] data = new double[totalDataPoints][2]; // Sample data: replace with real data
// Fill the data array ...
int trainSize = (int) (0.8 * totalDataPoints);
double[][] trainSet = Arrays.copyOfRange(data, 0, trainSize);
double[][] testSet = Arrays.copyOfRange(data, trainSize, totalDataPoints);
System.out.println("Train set size: " + trainSet.length);
System.out.println("Test set size: " + testSet.length);
}
}
Why Split Data?
Using a train-test split allows you to evaluate how well the model generalizes to unseen data. This is paramount in avoiding overfitting.
Pitfall #4: Neglecting to Utilize Libraries
In the realm of machine learning, libraries equipped with pre-built algorithms save time and minimize errors. Novices often build from scratch, disregarding the capabilities of established libraries.
Recommended Libraries:
- Weka: Ideal for beginners, Weka provides easy-to-use data mining and machine learning algorithms.
- Deeplearning4j: This library is perfect for deep learning applications within the Java ecosystem.
You can find more information about Weka and Deeplearning4j to explore how you can leverage them in your projects.
Why Use Libraries?
They encapsulate complex algorithms, so you can focus on modeling instead of coding low-level details. This can significantly accelerate the development process and reduce bugs.
Bringing It All Together
Mastering Java for machine learning involves an understanding of both the language's core features and best practices in machine learning. Avoiding common pitfalls - like neglecting object-oriented principles, overlooking the importance of data preprocessing, failing to evaluate model performance, and ignoring beneficial libraries - can lead to cleaner, more effective, and scalable machine learning applications.
Embark on your journey in Java-based machine learning equipped with these insights, and remember, continuous learning and practice are vital as the field of machine learning continues to evolve. Happy coding!