Overcoming JavaFX ScrollPane DropShadow Issues
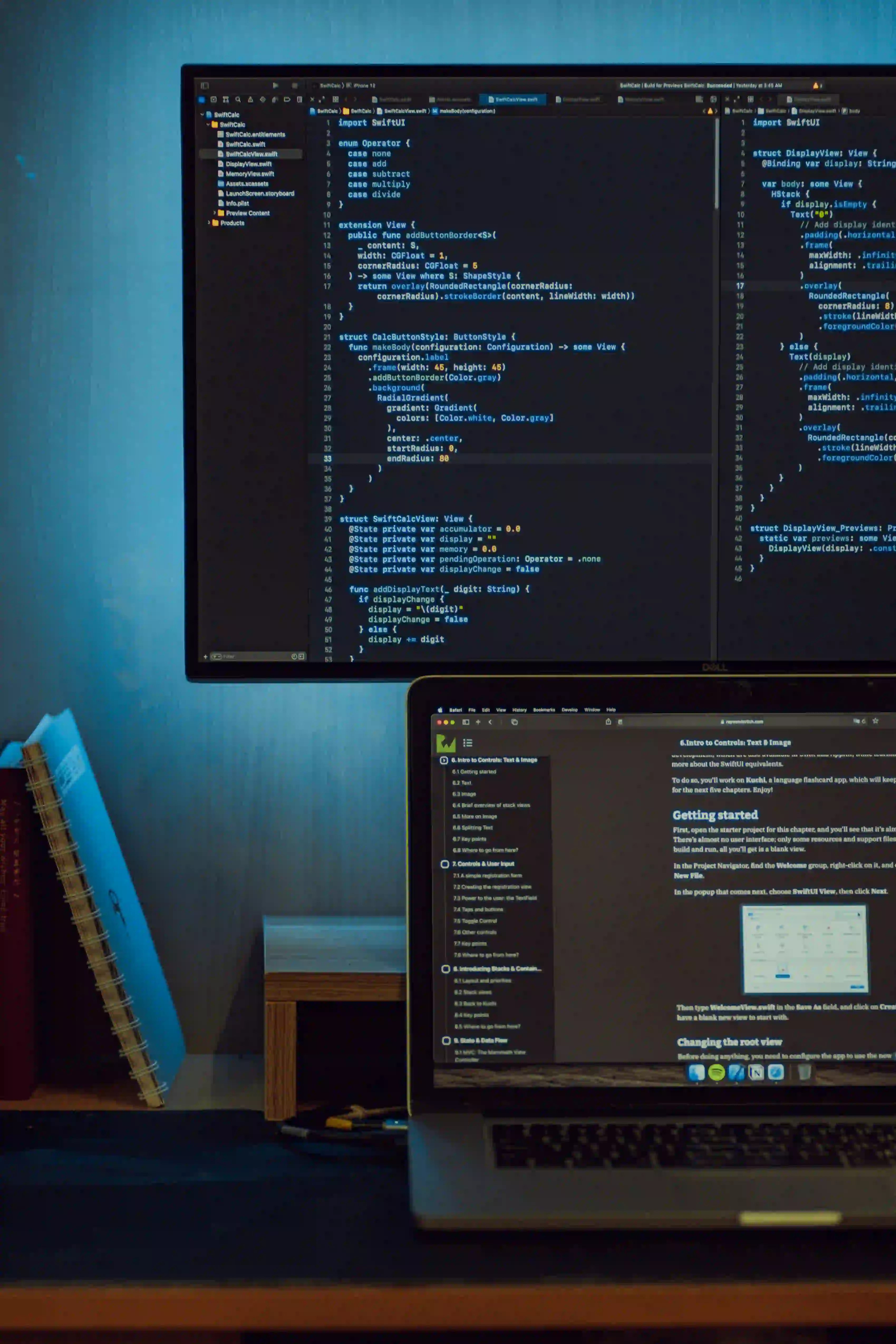
Overcoming JavaFX ScrollPane DropShadow Issues
In JavaFX development, creating engaging and interactive user interfaces is a common task. One useful component of JavaFX is the ScrollPane
, which enables smooth scrolling through a view that may exceed the dimensions of its container. However, developers often encounter issues with effects like DropShadow
not rendering as expected within a ScrollPane
. This blog post will delve into these challenges and present effective solutions, incorporating code snippets to illustrate best practices.
Understanding JavaFX DropShadow
The DropShadow
effect adds depth to user interface components, enhancing the aesthetic appeal of applications. This effect is applied as a filter to nodes, creating a shadow that appears behind the node. Here's a simple example of applying a DropShadow
to a Label
:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.effect.DropShadow;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class DropShadowExample extends Application {
@Override
public void start(Stage primaryStage) {
Label label = new Label("Hello, JavaFX!");
// Apply DropShadow effect
DropShadow dropShadow = new DropShadow();
dropShadow.setRadius(10);
dropShadow.setOffsetX(5);
dropShadow.setOffsetY(5);
label.setEffect(dropShadow);
StackPane root = new StackPane(label);
Scene scene = new Scene(root, 300, 200);
primaryStage.setTitle("DropShadow Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why Use DropShadow?
Adding a DropShadow
effect can enhance usability by improving visibility and focusing the user's attention on important items. However, when combined with a ScrollPane
, this effect can become problematic.
The ScrollPane Challenge
When adding nodes with effects like DropShadow
to a ScrollPane
, developers may notice that the shadow appears cut off or rendered incorrectly at the edges. This often happens because of how the ScrollPane
renders nodes.
The Cause of the Problem
The primary issue is that ScrollPane
clips content when it exceeds its predefined viewport boundaries. This clipping can unintentionally hide parts of the DropShadow
, causing it to look visually incomplete.
Example of the Issue
Try running the following code, which adds a label with a DropShadow
effect into a ScrollPane
. You'll see how the shadow is cut off:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.effect.DropShadow;
import javafx.scene.layout.VBox;
import javafx.scene.layout.StackPane;
import javafx.scene.control.ScrollPane;
import javafx.stage.Stage;
public class ScrollPaneDropShadowIssue extends Application {
@Override
public void start(Stage primaryStage) {
ScrollPane scrollPane = new ScrollPane();
VBox vBox = new VBox(10);
for (int i = 0; i < 20; i++) {
Label label = new Label("Label " + (i + 1));
DropShadow dropShadow = new DropShadow(10, 5, 5, javafx.scene.paint.Color.GRAY);
label.setEffect(dropShadow);
vBox.getChildren().add(label);
}
scrollPane.setContent(vBox);
Scene scene = new Scene(scrollPane, 300, 200);
primaryStage.setTitle("ScrollPane DropShadow Issue");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Solutions to the DropShadow Issue
1. Adjust the Clip Property
One of the simplest solutions is to adjust the clipping behavior of the ScrollPane
. By setting the clip property to null
, we disable clipping entirely, allowing the DropShadow
to display correctly:
scrollPane.setClip(null);
2. Use a StackPane
Another effective approach is to wrap the content of the ScrollPane
in a StackPane
. The StackPane
allows children to overlap, which can help in displaying shadows correctly since it doesn't clip its children by default:
StackPane stackPane = new StackPane();
stackPane.getChildren().add(vBox);
scrollPane.setContent(stackPane);
Implementation Example
Here’s an improved version of our previous example, incorporating these solutions:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label;
import javafx.scene.effect.DropShadow;
import javafx.scene.layout.VBox;
import javafx.scene.layout.StackPane;
import javafx.scene.control.ScrollPane;
import javafx.stage.Stage;
public class CorrectedScrollPaneExample extends Application {
@Override
public void start(Stage primaryStage) {
ScrollPane scrollPane = new ScrollPane();
VBox vBox = new VBox(10);
for (int i = 0; i < 20; i++) {
Label label = new Label("Label " + (i + 1));
DropShadow dropShadow = new DropShadow(10, 5, 5, javafx.scene.paint.Color.GRAY);
label.setEffect(dropShadow);
vBox.getChildren().add(label);
}
StackPane stackPane = new StackPane(vBox);
scrollPane.setContent(stackPane);
scrollPane.setFitToWidth(true); // Optional: Fit content to the width of the ScrollPane
scrollPane.setClip(null); // Disable clipping
Scene scene = new Scene(scrollPane, 300, 200);
primaryStage.setTitle("Corrected ScrollPane Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Closing the Chapter
Integrating effects such as DropShadow
with a ScrollPane
in JavaFX can be challenging due to unexpected clipping issues. By employing strategies like adjusting the clip property and utilizing a StackPane
, we can create a more visually appealing and user-friendly interface.
For further reading on JavaFX components and effects, consider exploring the official JavaFX Documentation. With these insights and adjustments, you're equipped to enhance your JavaFX applications. Happy coding!