Overcoming Challenges in Java Temporary Caching API Testing
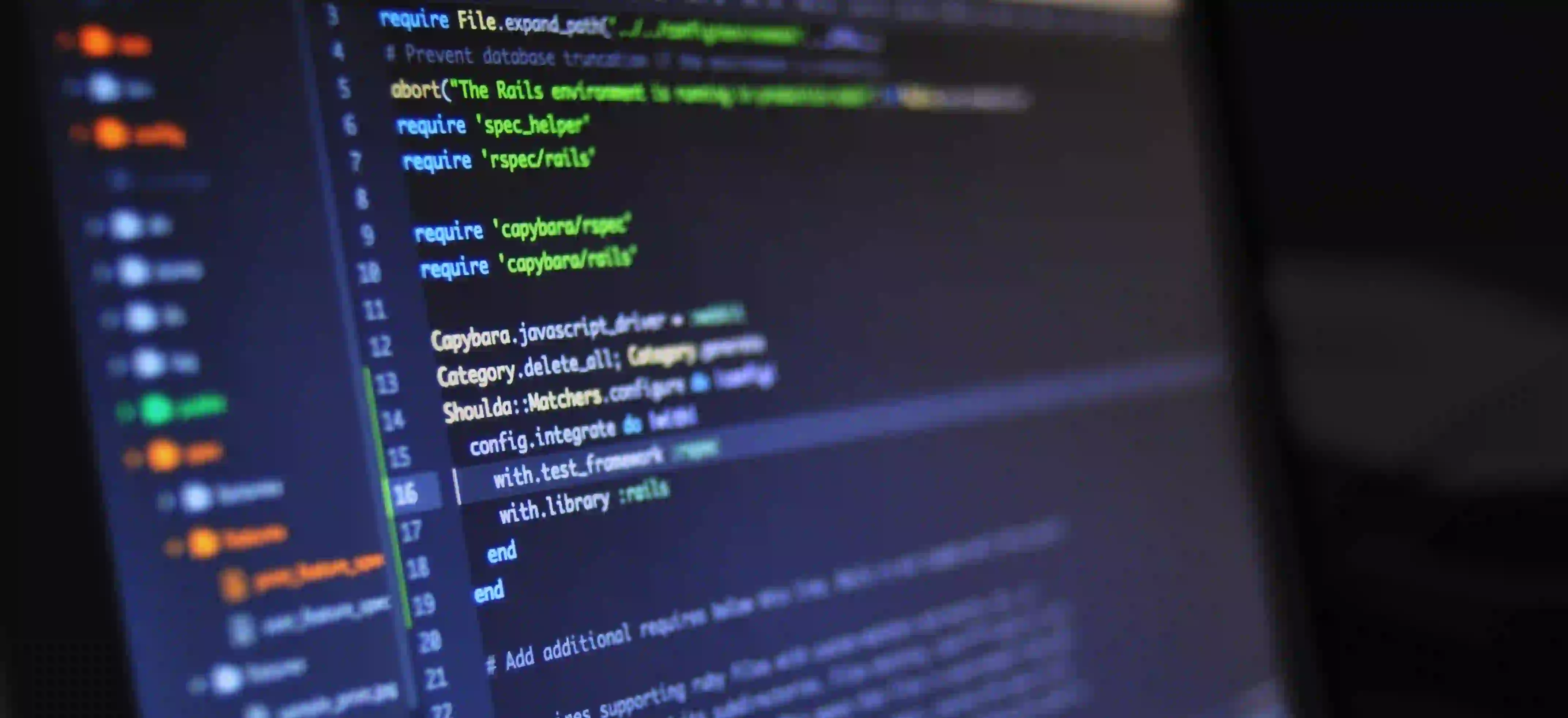
Overcoming Challenges in Java Temporary Caching API Testing
Caching has become a crucial aspect of performance optimization in web applications. To ensure that the APIs leveraging caches perform as expected, testing these temporary caching mechanisms becomes vital. In this blog post, we will dive into the challenges associated with Java temporary caching API testing and explore effective strategies to overcome them.
Understanding Temporary Caching
Temporary caching refers to the short-lived storage of frequently accessed data in memory to reduce latency and improve response times in applications. Java developers often use frameworks and libraries such as Ehcache or Caffeine for caching in their applications. However, when it comes to testing these caching mechanisms, several challenges may arise.
Common Challenges in Temporary Caching API Testing
-
Data Consistency and Staleness: Temporary caches may not reflect the most recent state of underlying data, leading to potential staleness issues during testing.
-
Cache Eviction: The caching strategy might result in different data being returned at different times, especially after cache eviction events.
-
Environment Configuration: Testing caches often requires a specific environment setup, which can be cumbersome.
-
Multi-threaded Access: Simultaneous access to cached data can lead to inconsistent results if not handled properly.
-
Performance Metrics: Measuring the performance impact of caching during tests can be complex.
-
Mocking External Services: When caches depend on external services, mocking these services can be challenging.
To tackle these issues, it is essential to explore structured approaches and best practices.
Strategies for Effective Testing of Temporary Caching
1. Establishing a Testing Framework
A comprehensive testing framework is the foundation of reliable caching tests. Utilize libraries such as JUnit and Mockito.
Example Setup using JUnit
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import static org.mockito.Mockito.*;
class CacheServiceTest {
private CacheService cacheService;
private ExternalService externalService;
@BeforeEach
void setUp() {
externalService = mock(ExternalService.class);
cacheService = new CacheService(externalService);
}
@Test
void testCacheIsPopulated() {
when(externalService.getData()).thenReturn("Expected Data");
String cachedData = cacheService.getCachedData();
assertEquals("Expected Data", cachedData);
}
}
Why Use This Code? Mocking the external service allows you to isolate tests and focus solely on the caching logic, ensuring clarity and reliability in your tests.
2. Managing Data Consistency
To deal with data staleness, consider implementing mechanisms that validate cache freshness. Define a strategy for when to refresh or invalidate cached data.
Example Strategy to Refresh Cache
public class CacheService {
private Cache<String, String> cache;
public CacheService() {
this.cache = Caffeine.newBuilder()
.expireAfterWrite(5, TimeUnit.MINUTES)
.build();
}
public String getCachedData() {
String key = "importantData";
return cache.get(key, k -> fetchDataFromExternalService());
}
private String fetchDataFromExternalService() {
// Logic to fetch data
return ...;
}
}
Why Implement This? This code snippet uses Caffeine's expiration features to ensure that the data in the cache remains relevant, thereby helping to mitigate data staleness.
3. Simulating Eviction Scenarios
To thoroughly test how your cache responds to eviction, configure tests to fill the cache to its limits. Use various eviction strategies to simulate pressure on the cache.
Example Cache Eviction Test
@Test
void testCacheEviction() {
for (int i = 0; i < 10; i++) {
cacheService.setData("key" + i, "value" + i);
}
// Assume that the cache can only hold 5 items
assertNull(cacheService.getData("key0")); // This might be evicted
}
Why Use This Approach? Testing cache eviction gives insights into how the cache handles pressure and if stale data can lead to performance issues.
4. Multi-threaded Access Testing
Ensure your test cases account for concurrent access to caches. Java provides the ExecutorService
to simulate concurrent requests.
Example Concurrent Access Test
@Test
void testConcurrentCacheAccess() throws InterruptedException {
ExecutorService executor = Executors.newFixedThreadPool(10);
String key = "sharedData";
for (int i = 0; i < 10; i++) {
executor.submit(() -> {
String result = cacheService.getCachedData(key);
// Assert cache behavior, e.g., check if expected data is returned
});
}
executor.shutdown();
executor.awaitTermination(1, TimeUnit.MINUTES);
}
Why Implement This? This code showcases the importance of thread safety and how to assess cache reliability under multi-threaded conditions, ensuring robustness in concurrent scenarios.
5. Performance Monitoring and Optimization
Use profiling tools like Java Flight Recorder or Java VisualVM to measure the performance impact of caching. These tools allow you to understand memory usage and response times.
6. Mocking External Dependencies
Utilize Mockito to create mocks for any external services relied upon by your caching mechanism. This enables developers to create isolated tests without relying on external systems.
The Last Word
Testing temporary caching mechanisms in Java APIs presents various challenges, from data consistency to multi-threaded access. By establishing a robust testing framework, simulating eviction and concurrent access, and ensuring performance monitoring, these challenges can be effectively addressed. Utilizing libraries such as JUnit, Mockito, and caching frameworks like Caffeine creates an environment conducive to reliable testing.
For deeper insights and additional resources on Java caching and testing methods, check out the official documentation for Ehcache and Caffeine.
By following these outlined strategies, Java developers can ensure their caching implementations are tested rigorously and held to high performance standards. Ready to take your Java caching API testing to the next level? Start coding today!