Struggling with Spring Security? Custom Password Encoding Made Easy!
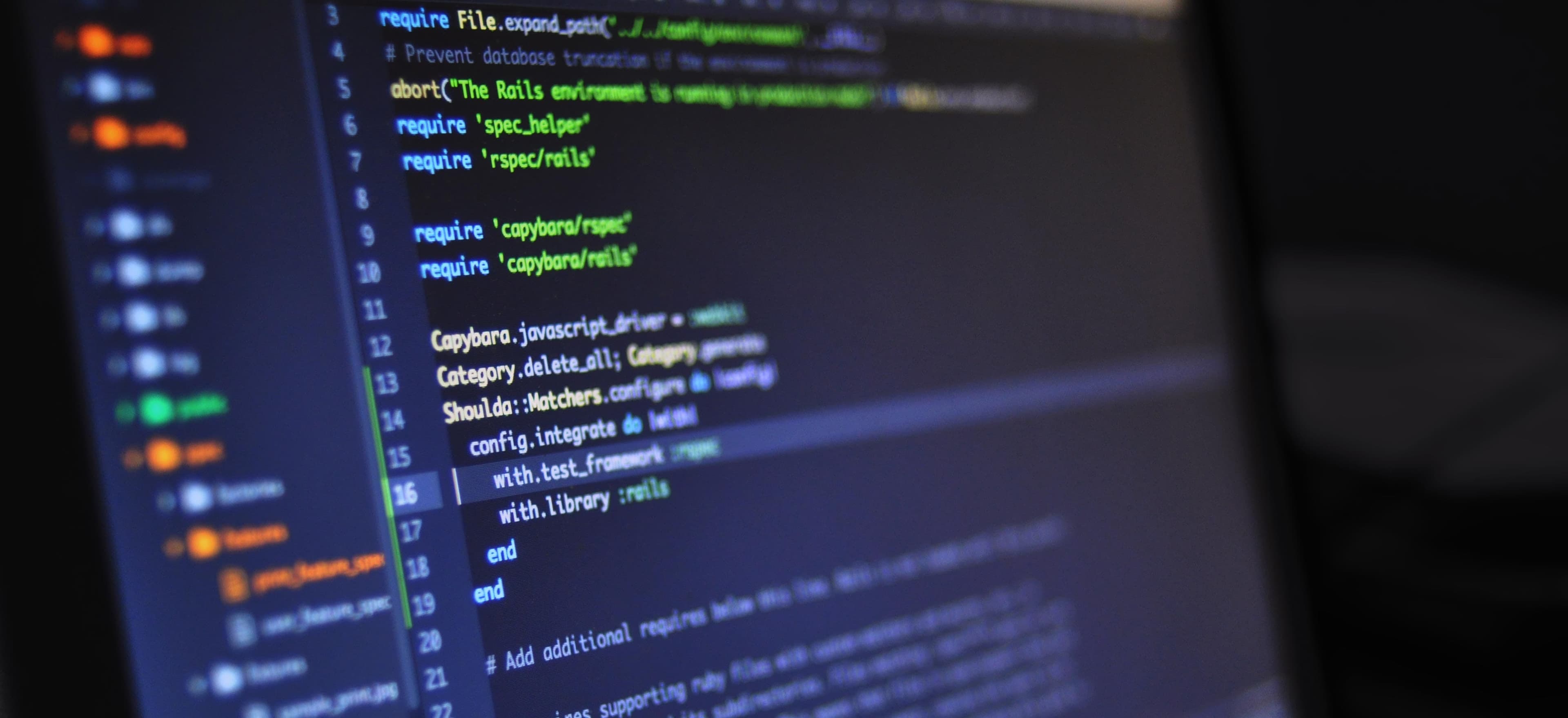
- Published on
Struggling with Spring Security? Custom Password Encoding Made Easy!
When it comes to securing applications in Java, Spring Security is one of the go-to frameworks. However, one common struggle developers face is configuring custom password encoding strategies. In this post, we’ll simplify the process of custom password encoding in Spring Security, ensuring that you can implement secure user authentication with confidence.
Why Password Encoding Matters
Before diving into the code, let's first discuss why password encoding is essential. Storing passwords as plain text is a security nightmare. If an unauthorized entity gains access to your database, they could easily compromise user accounts.
Password encoding transforms a password into a hash, meaning that the original password cannot be easily retrieved from the hash, even if the hash itself is exposed. Spring Security offers multiple ways to handle password encoding, allowing you to choose the scheme that best fits your application's needs.
Common Password Encoding Schemes
While Spring Security provides built-in encoders like BCryptPasswordEncoder
, NoOpPasswordEncoder
, and SCryptPasswordEncoder
, you might want to implement a custom encoder for more flexibility or unique requirements.
Built-In Encoders
- BCryptPasswordEncoder: Uses the BCrypt hashing function, offering strong security against brute-force attacks.
- NoOpPasswordEncoder: Useful for testing and development, but not recommended for production due to lack of security.
- SCryptPasswordEncoder: Useful when you need a configurable memory and CPU cost, offering more security at the cost of increased resource usage.
Implementing a Custom Password Encoder
Let’s break down the steps to create your custom password encoder. We will be implementing a simple CustomPasswordEncoder
that utilizes SHA-256 hashing.
Step 1: Creating the Custom Password Encoder
First, create a Java class for your custom password encoder.
import org.springframework.security.crypto.password.PasswordEncoder;
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class CustomPasswordEncoder implements PasswordEncoder {
@Override
public String encode(CharSequence rawPassword) {
return hashPassword(rawPassword.toString());
}
@Override
public boolean matches(CharSequence rawPassword, String encodedPassword) {
return encode(rawPassword).equals(encodedPassword);
}
private String hashPassword(String password) {
try {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] hashedBytes = digest.digest(password.getBytes());
StringBuilder hexString = new StringBuilder();
for (byte b : hashedBytes) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) {
hexString.append('0');
}
hexString.append(hex);
}
return hexString.toString();
} catch (NoSuchAlgorithmException e) {
throw new RuntimeException("Error hashing password", e);
}
}
}
Explanation of the Code
- PasswordEncoder Interface: We implement
PasswordEncoder
, which requires defining two methods:encode()
andmatches()
. - Hashing Method: The
hashPassword()
uses the SHA-256 algorithm to encode the password. This is a secure one-way hashing approach, although you might want to consider using salts for added security. - Exception Handling: We catch
NoSuchAlgorithmException
to handle cases where the algorithm is unavailable.
Step 2: Configuring Spring Security
Once you have implemented your custom password encoder, you need to configure it in your Spring Security configuration.
import org.springframework.context.annotation.Bean;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
import org.springframework.security.crypto.password.PasswordEncoder;
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.userDetailsService(userDetailsService())
.passwordEncoder(passwordEncoder());
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated()
.and()
.formLogin();
}
@Bean
public PasswordEncoder passwordEncoder() {
return new CustomPasswordEncoder();
}
}
Explanation of the Configuration
- AuthenticationManagerBuilder: We configure the authentication manager to use our
CustomPasswordEncoder
. - HttpSecurity: Here, we define which endpoints require authentication and how users will authenticate (via form login).
- Bean Configuration: Our custom password encoder is defined as a Spring bean, making it accessible throughout the application.
Step 3: Testing the Custom Password Encoder
After integrating your custom password encoder, it's important to test it out. You can set up unit tests to verify both the encoding and matching functionalities.
import static org.junit.jupiter.api.Assertions.*;
import org.junit.jupiter.api.Test;
public class CustomPasswordEncoderTest {
private final CustomPasswordEncoder encoder = new CustomPasswordEncoder();
@Test
public void testEncoding() {
String rawPassword = "mySecurePassword";
String encodedPassword = encoder.encode(rawPassword);
assertNotNull(encodedPassword);
assertNotEquals(rawPassword, encodedPassword);
}
@Test
public void testMatching() {
String rawPassword = "mySecurePassword";
String encodedPassword = encoder.encode(rawPassword);
assertTrue(encoder.matches(rawPassword, encodedPassword));
assertFalse(encoder.matches("wrongPassword", encodedPassword));
}
}
Explanation of the Tests
- The first test checks that the encoded password is not null or equal to the raw password.
- The second verifies that the
matches()
method returns true for the correct password and false for an incorrect one.
The Bottom Line
Custom password encoding in Spring Security might seem daunting at first, but it can be simplified with the right approach and understanding. By implementing a CustomPasswordEncoder
, you can cater to the specific security needs of your application while maintaining a clear and straightforward code structure.
By following this guide, you can now confidently secure user passwords and enhance the overall security of your Java applications using Spring Security. Always remember, the key to secure authentication is not just a strong algorithm but also ensuring that sensitive data is never stored or transmitted in a vulnerable state.
For more information on Spring Security, check out the official Spring Security documentation to explore advanced topics and best practices. Happy coding!