Choosing Between Spring RestTemplate and WebClient: A Guide
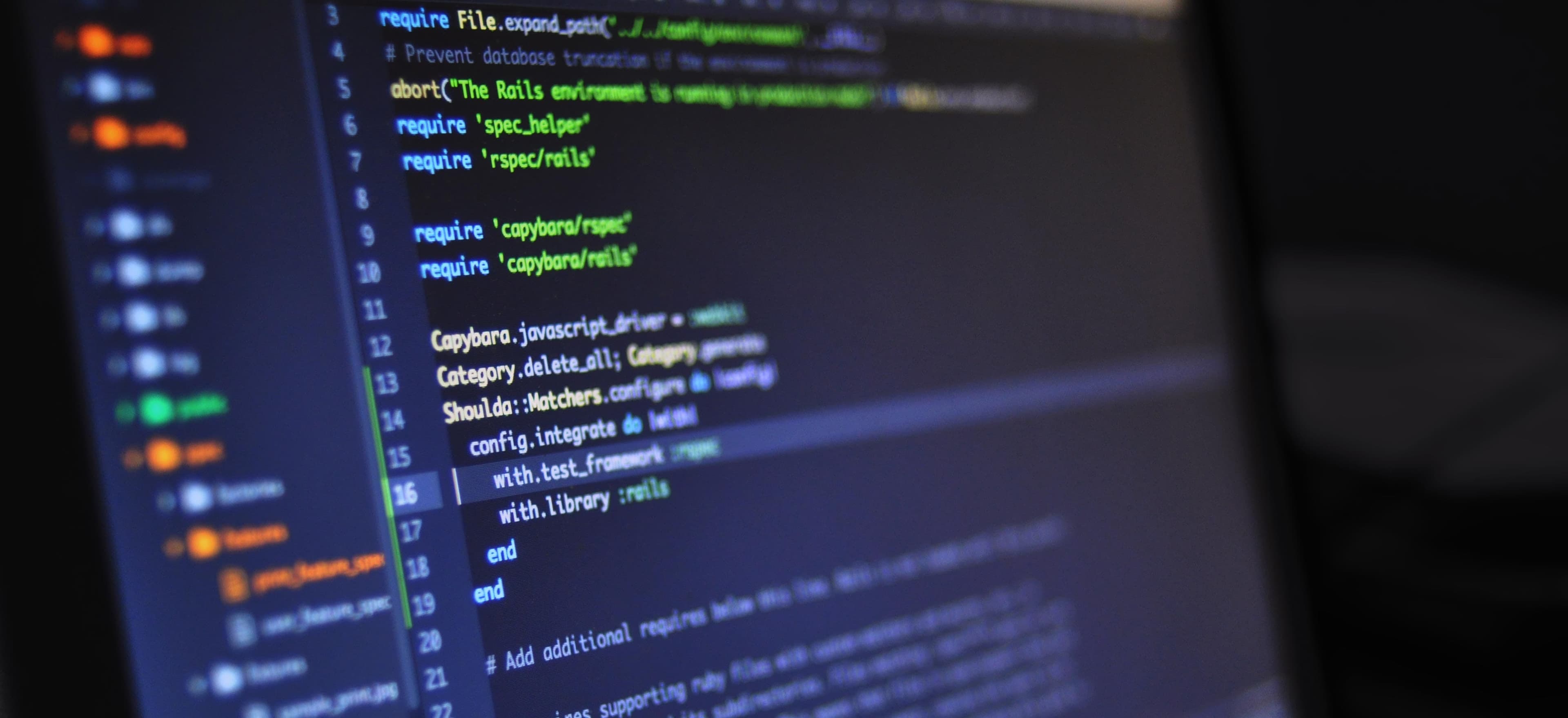
- Published on
Choosing Between Spring RestTemplate and WebClient: A Guide
In the world of Spring Framework, developers often find themselves in need of performing HTTP requests. Whether you are interacting with RESTful services or consuming APIs, the choice of HTTP client is pivotal. Two popular options provided by Spring are RestTemplate and WebClient. This guide will explore both, highlight their differences, and help you make an informed decision based on your project needs.
Overview of RestTemplate
RestTemplate is an older, synchronous client that simplifies communication with HTTP servers. It provides methods that facilitate CRUD operations: Create, Read, Update, and Delete.
Key Features of RestTemplate
- Simplicity: APIs are straightforward and easy to use.
- Synchronous: Operates in a blocking manner, where a thread waits for the response.
- Support for Various HTTP Methods: Easily handle GET, POST, PUT, DELETE requests and more.
- Exception Handling: Built-in support for handling HTTP errors.
Example of Using RestTemplate
Here's a basic example of how to consume a REST API using RestTemplate.
import org.springframework.web.client.RestTemplate;
import org.springframework.http.ResponseEntity;
public class RestTemplateExample {
public static void main(String[] args) {
RestTemplate restTemplate = new RestTemplate();
String url = "https://api.example.com/products/1";
// Making a GET request
Product product = restTemplate.getForObject(url, Product.class);
System.out.println("Product Name: " + product.getName());
// Making a POST request
Product newProduct = new Product("New Product", 99.99);
ResponseEntity<Product> response =
restTemplate.postForEntity(url, newProduct, Product.class);
System.out.println("Created Product ID: " + response.getBody().getId());
}
}
In this snippet, we define a RestTemplateExample
class where we create an instance of RestTemplate
. We make GET and POST requests with minimal code. Its simplicity is one of the main advantages of using RestTemplate.
Overview of WebClient
WebClient, on the other hand, is a part of the Spring WebFlux framework and is designed for non-blocking, reactive programming. It is an advanced and modern alternative to RestTemplate.
Key Features of WebClient
- Asynchronous and Non-Blocking: Offers a non-blocking way to handle requests.
- Reactive Support: Integrates seamlessly with the reactive programming model.
- Flexibility: Supports more complex scenarios with diverse response types.
- Enhanced Error Handling: Provides a more robust mechanism for error handling.
Example of Using WebClient
Here's an example demonstrating how to use WebClient to fetch data asynchronously.
import org.springframework.web.reactive.function.client.WebClient;
import reactor.core.publisher.Mono;
public class WebClientExample {
public static void main(String[] args) {
WebClient webClient = WebClient.create("https://api.example.com");
// Making a GET request
Mono<Product> productMono = webClient.get()
.uri("/products/1")
.retrieve()
.bodyToMono(Product.class);
// Subscribe to the Mono to perform actions when the response is received
productMono.subscribe(product -> {
System.out.println("Product Name: " + product.getName());
});
// Making a POST request
Product newProduct = new Product("New Product", 99.99);
webClient.post()
.uri("/products")
.body(Mono.just(newProduct), Product.class)
.retrieve()
.bodyToMono(Product.class)
.subscribe(responseProduct -> {
System.out.println("Created Product ID: " + responseProduct.getId());
});
}
}
In this example, we create a WebClient
instance and perform both GET and POST requests. The use of Mono
enables us to handle the response asynchronously, making it powerful for modern applications.
Comparing RestTemplate and WebClient
Performance
- RestTemplate: It can be easier to use for simpler applications but can lead to resource blocking under high loads.
- WebClient: Offers better performance in reactive applications and is more suitable for applications that require high concurrency.
API Complexity
- RestTemplate: Easy to grasp, especially for developers familiar with synchronous programming.
- WebClient: Has a steeper learning curve, but offers more flexibility and control over the responses.
Use Cases
- RestTemplate: Best for legacy systems or smaller applications that do not require concurrency.
- WebClient: Ideal for reactive applications and services under Spring WebFlux that need non-blocking calls.
When to Use Each Client
When deciding between RestTemplate and WebClient, consider the following questions:
- Is your application synchronous or asynchronous? If you are building a modern, asynchronous application, prefer WebClient.
- Do you require compatibility with a reactive-stack? Choose WebClient if you aim to integrate with Project Reactor and utilize reactive streams.
- Are you maintaining an existing application? Stick to RestTemplate if the application uses it and there is no immediate need for upgrade.
Wrapping Up
The choice between RestTemplate and WebClient largely depends on your application's architecture, performance, and complexity requirements. While RestTemplate serves well in simpler, synchronous applications, WebClient proves its worth in modern, reactive programming contexts.
For added depth on Spring's HTTP client capabilities, consider exploring the Spring Documentation or delve into reactive programming concepts to leverage these technologies effectively.
Ultimately, understanding the nuances of both clients will enable you to select the one that aligns best with your development goals. Happy coding!