Overcoming Deployment Delays in Java EE 7 with Docker
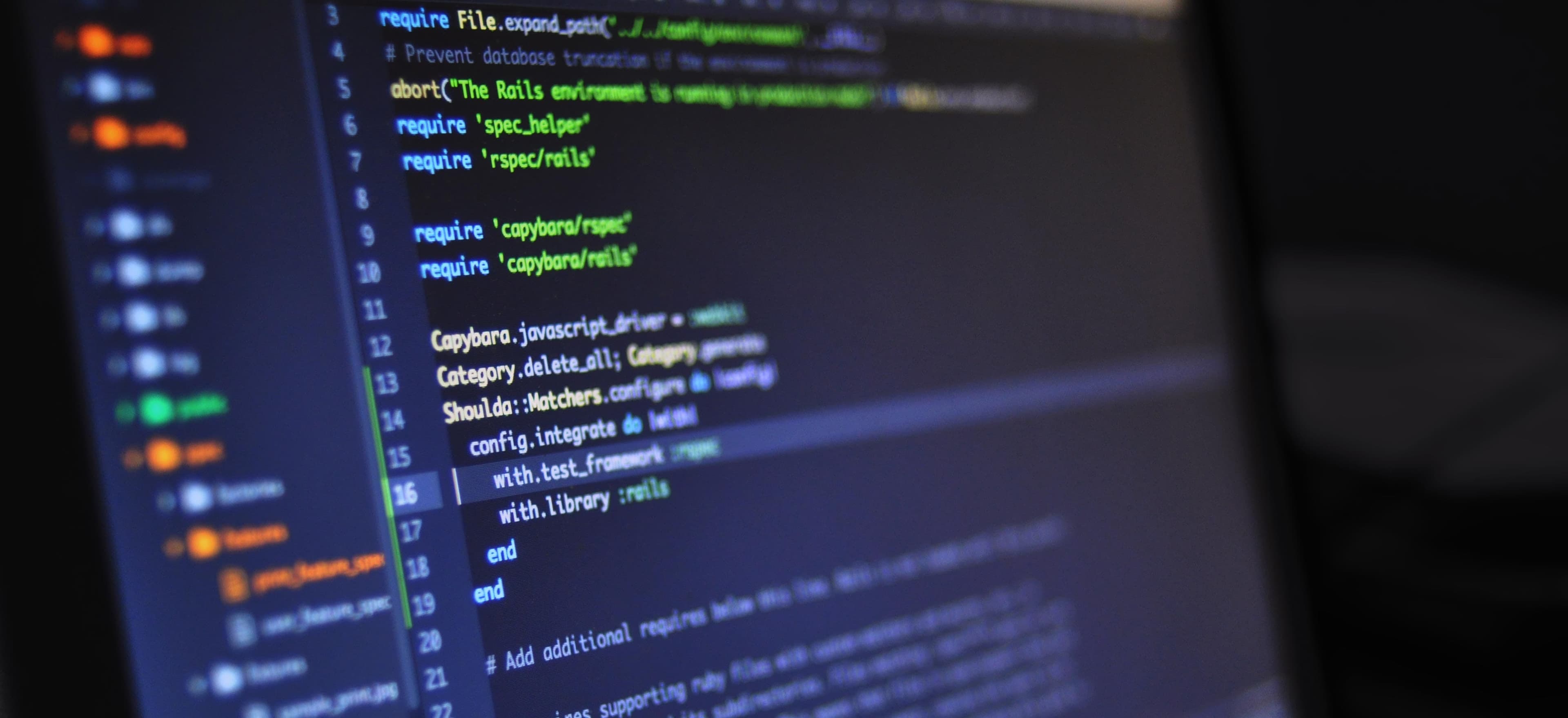
- Published on
Overcoming Deployment Delays in Java EE 7 with Docker
In the fast-paced world of software development, deployment delays can be a bottleneck, often leading to increased frustration among teams and stakeholders. Java EE 7, while robust, can sometimes suffer from prolonged deployment cycles due to various environmental inconsistencies and dependencies. However, with the advent of containerization, particularly using Docker, these challenges can be significantly mitigated.
Understanding Docker and Java EE 7
Before we dive into solutions, let’s clarify the role of Docker in the deployment process. Docker is a platform that allows developers to automate the deployment of applications inside lightweight, portable containers. Java EE 7, on the other hand, provides enterprise-level features such as dependency injection, web services, and APIs for building scalable applications.
By combining these two technologies, developers can ensure that their applications behave consistently across different environments, reducing deployment delays.
The Problem with Traditional Deployment
Typical application deployment in Java EE involves multiple steps, which may include:
- Building the application.
- Packaging it as a WAR/JAR file.
- Deploying it to a server (like Glassfish, WildFly, or TomEE).
- Configuring the server environment to match development settings.
Each of these steps can introduce delays. Let's consider a real-world example: a simple Java EE application that takes a few minutes to deploy. If there are discrepancies in the server environment or configuration, the deployment can fail, forcing teams to troubleshoot and start the process all over again.
Introducing Docker into the Workflow
Why Use Docker for Java EE 7 Deployment?
-
Consistency Across Environments: Docker provides a consistent environment by allowing developers to encapsulate their application and its dependencies within a container. This means every team member, from developer to QA to production, interacts with the exact same environment.
-
Speed: Docker containers can be started, stopped, and destroyed quickly. This significantly reduces the time spent on managing resources.
-
Isolation: Docker allows multiple applications to run on the same hardware but keeps them isolated from one another. This means you can deploy multiple versions of your Java EE application without conflicts.
Setting Up Docker with a Java EE 7 Application
Let’s walk through creating a basic Docker setup for a Java EE 7 application. For this example, assume you have already built a simple RESTful service using Java EE 7.
Step 1: Create a Dockerfile
A Dockerfile
is a script that contains a series of instructions to assemble the container image. Here is a sample Dockerfile for a Java EE application.
# Use the official Open Liberty image from the Docker Hub
FROM openliberty/open-liberty:full-java8-openj9
# Copy your application WAR file into the server's apps directory
COPY target/myapp.war /config/apps/
# Expose the server port
EXPOSE 9080
# Start Open Liberty
CMD ["/opt/ol/wlp/bin/server", "run", "defaultServer"]
Explanation
- FROM: Specifies the base image for the container. Here, we use the official Open Liberty image.
- COPY: Copies the compiled WAR file from the local system into the container.
- EXPOSE: Defines the port on which the application will run.
- CMD: Provides the command to run once the container starts, launching the server.
Step 2: Build the Docker Image
To create the Docker image from your Dockerfile, run the following command in the directory containing the Dockerfile.
docker build -t myapp-image .
Explanation
The -t
flag tags the image for easier reference. The dot (.
) at the end specifies the context directory for Docker to find the Dockerfile.
Step 3: Run the Container
After building the image, run the container with this command:
docker run -d -p 9080:9080 --name myapp-container myapp-image
Explanation
- -d: Runs the container in detached mode (in the background).
- -p: Maps port 9080 from the container to port 9080 on the host.
- --name: Assigns a name to the container for easy reference.
Validating the Deployment
To check if your application is running correctly, open your web browser or use a tool like curl
to access:
http://localhost:9080/myapp/api
Replace /myapp/api
with the actual path to your REST endpoint. If everything is working correctly, you should receive the expected JSON response.
Overcoming Post-Deployment Challenges
While using Docker improves deployment speed, challenges can still arise post-deployment, such as managing configurations, scaling, or logging.
Configuration Management with Docker
Using environment variables inside Docker can help manage various deployment configurations without hardcoding values.
To pass an environment variable to your Docker container, include the -e
flag:
docker run -d -p 9080:9080 --name myapp-container -e MY_ENV_VAR=value myapp-image
Individual environments—be it Development, Staging, or Production—can have separate configurations without altering the codebase.
Scaling Applications
Docker's inherent capabilities for scaling applications also allow you to easily deploy multiple instances of your Java EE application with minimal effort, using orchestration tools like Docker Compose and Kubernetes.
For example, using Docker Compose, you can define multiple replicas of your application in a straightforward YAML configuration.
version: '3'
services:
myapp:
image: myapp-image
ports:
- "9080:9080"
deploy:
replicas: 3
Enhanced Logging and Monitoring
To manage logs efficiently, consider using centralized logging solutions like ELK Stack (Elasticsearch, Logstash, and Kibana), or integrated Docker logging drivers. This centralization makes it easier to troubleshoot issues without hunting through multiple log files.
The Closing Argument
By leveraging Docker for deploying Java EE 7 applications, you can efficiently streamline your deployment process, reduce delays, and ensure consistency across environments. This ultimately fosters better collaboration among development, testing, and operations teams.
Further Reading
For those wanting to explore this topic in greater depth, consider looking at:
By embracing containerization and automation, you can unlock a smoother workflow for deploying your Java EE applications and turn deployment delays into rapid rollouts.
Checkout our other articles