Efficiently Split Strings in Java: Overcoming Nth Character Issues
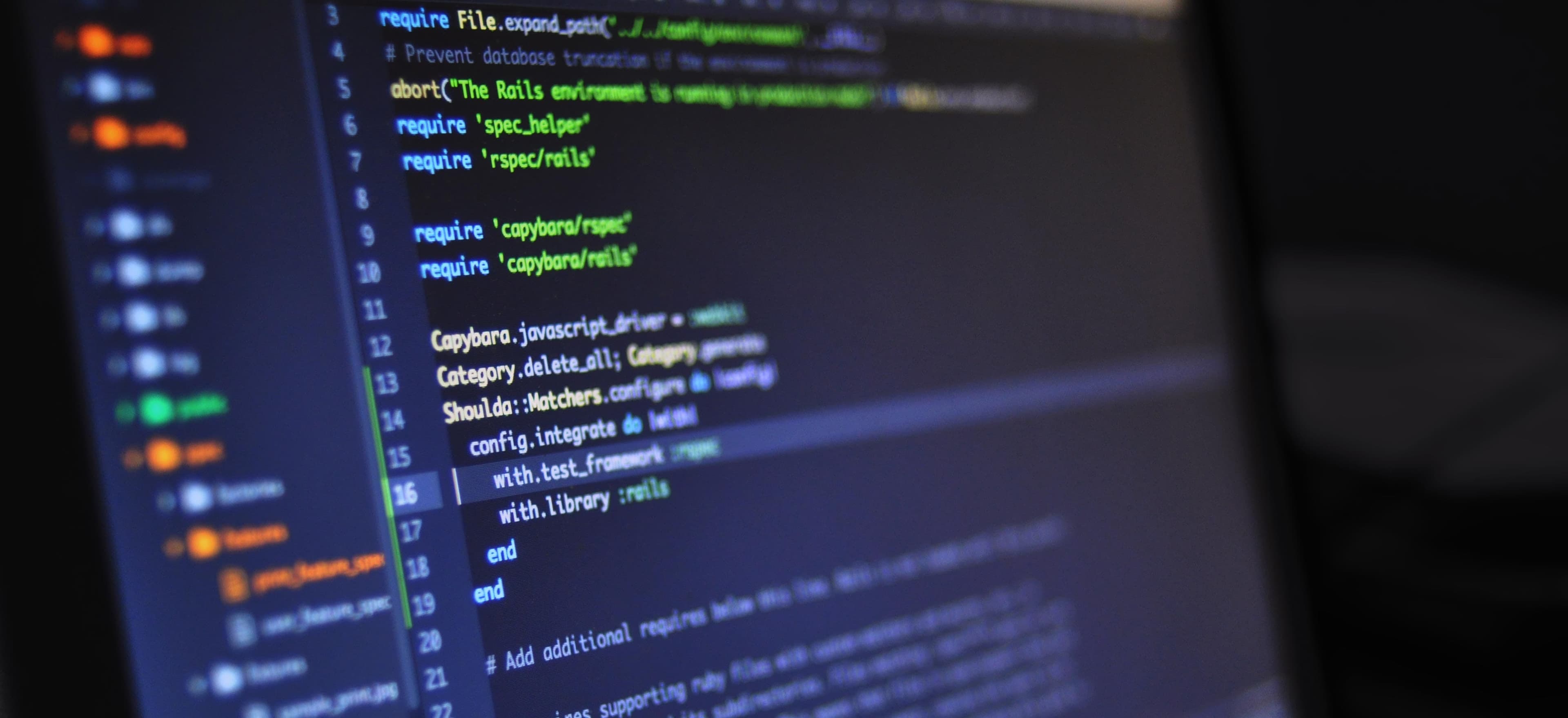
- Published on
Efficiently Split Strings in Java: Overcoming Nth Character Issues
In the world of programming, strings are a fundamental data type used in nearly every application. However, manipulating strings can sometimes be a challenging task, especially when it comes to splitting them. This article delves into effective methods for splitting strings in Java, particularly how to tackle issues related to the Nth character.
Understanding String Splitting
String splitting is a common operation in Java. The String
class includes a method called split(String regex)
, which allows developers to partition a string based on a specified regular expression. Here's a simple illustration:
String text = "apple,banana,cherry";
String[] fruits = text.split(",");
// Outputting the result
for (String fruit : fruits) {
System.out.println(fruit);
}
Why Split Strings?
Splitting strings can help you handle data more effectively. Whether you are parsing CSV data or extracting tokens from a sentence, mastering string manipulation is essential. When it comes to intricate patterns or unique character positions, the challenge increases. That's where understanding the Nth character concept comes into play.
Overview of the Nth Character Challenge
Many Java developers encounter difficulties when they need to manipulate strings with a focus on specific positions—specifically, when they want to split strings based on the Nth occurrence of a delimiter. This concept is essential when your data structure does not conform to a predictable pattern, requiring more advanced techniques.
For instance, if you want to split the example string after the second comma, you cannot achieve this with basic split()
functionality alone. Instead, you would need a more refined approach.
For a detailed exploration of string splitting challenges in Java and strategies to overcome them, check out our previous article: Mastering String Splitting: Tackle the Nth Character Challenge!.
Custom Split Method to Handle Nth Character
To effectively tackle Nth character splits, we can create a custom method. Here's an example to illustrate this concept:
public class StringSplitUtil {
public static String[] splitAtNth(String str, String delimiter, int n) {
String[] parts = new String[n + 1];
int index = 0;
int pos = 0;
while (n > 0) {
pos = str.indexOf(delimiter);
if (pos == -1) break;
parts[index++] = str.substring(0, pos);
str = str.substring(pos + delimiter.length());
n--;
}
parts[index] = str; // Add the rest of the string
return java.util.Arrays.copyOf(parts, index + 1); // Resize array
}
public static void main(String[] args) {
String text = "one,two,three,four,five";
String[] result = splitAtNth(text, ",", 2);
for (String part : result) {
System.out.println(part);
}
}
}
Code Breakdown
- Method Definition: The method
splitAtNth
takes a string, a delimiter, and the Nth value. - Loop and Split: We loop until the Nth occurrence is reached:
- Use
indexOf()
to find the delimiter. - Use
substring()
to extract parts of the string.
- Use
- Handling Remaining String: After reaching the last split, store the remaining string.
- Dynamic Array: Finally, we return a copy of the array to ensure it has the correct size.
This approach provides greater control and allows developers to specify exactly where the splits should occur.
Alternatives to String Splitting
While the standard split()
method is convenient, it lacks flexibility for complex string manipulations. Here are a couple of additional alternatives you can consider:
-
Using Regular Expressions: Regular expressions can be powerful for intricate patterns, but they may come with their performance costs for highly repetitive tasks.
Example:
String text = "one,two,three,four,five"; String[] parts = text.split(",(?=([^,]*,){2})"); for (String part : parts) { System.out.println(part); }
-
Java Streams API: If you are leveraging Java 8 or above, you can utilize streams for efficient processing.
Example:
String text = "one,two,three,four,five"; List<String> parts = Arrays.stream(text.split(",")) .limit(2) .collect(Collectors.toList()); parts.forEach(System.out::println);
Performance Considerations
When repeatedly splitting strings in tight loops or handling large texts, consider the performance implications of your choice. Custom methods tend to yield better performance over time, as they can be optimized for specific requirements.
Bringing It All Together
String manipulation is a vital skill in Java, especially when faced with challenges like Nth character splitting. By adopting custom split methods and understanding the intricacies of string processing, developers can enhance their applications significantly.
Exploring advanced string manipulation techniques, such as those discussed in this article and the more extensive Mastering String Splitting: Tackle the Nth Character Challenge! article, will undoubtedly amplify your toolkit as a Java developer.
Remember, mastering string manipulation can lead to cleaner, more efficient code and ultimately, a better experience for the end-users you serve. Happy coding!
Checkout our other articles