Mastering Java: Efficiently Handling Data Transformation
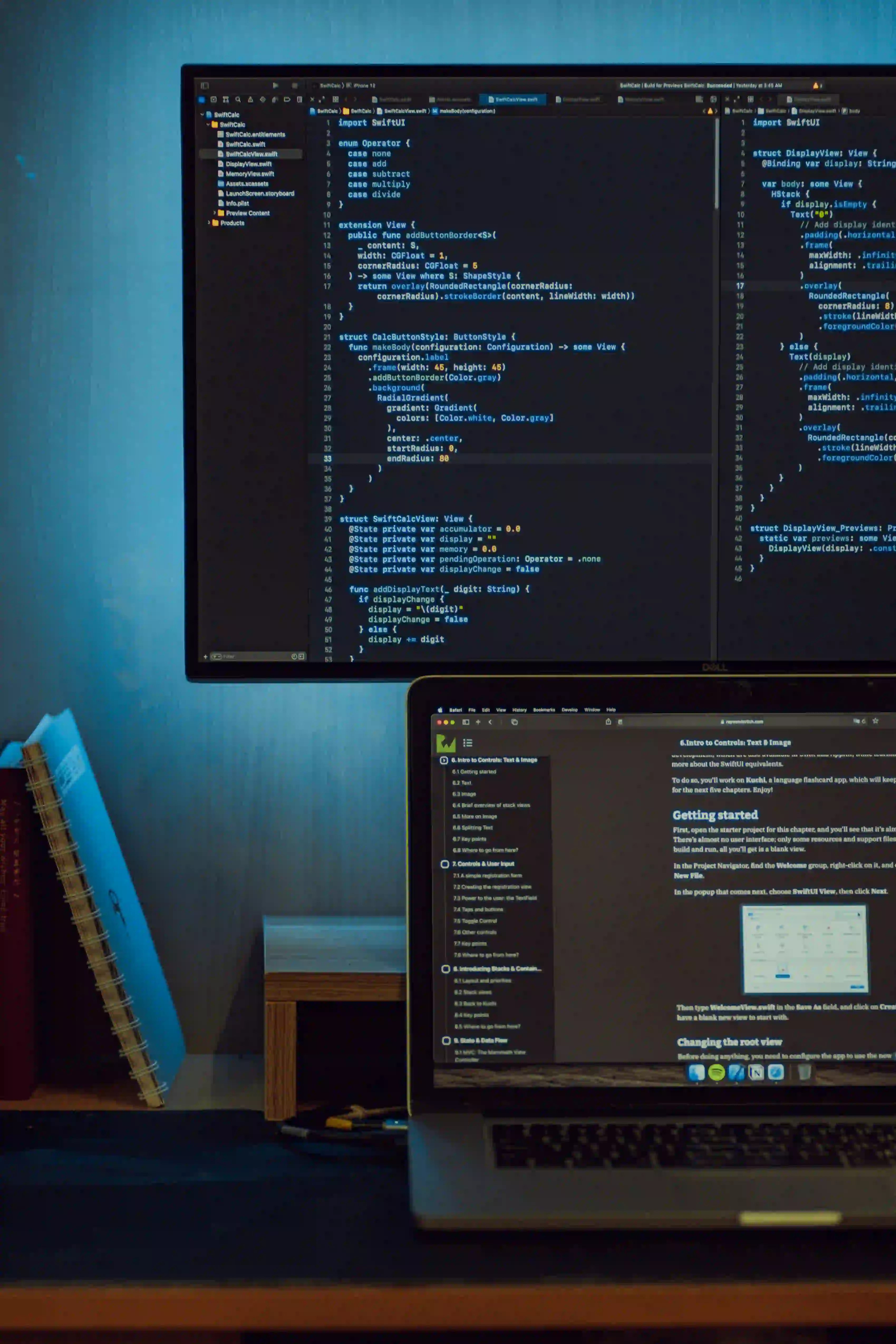
Mastering Java: Efficiently Handling Data Transformation
Data transformation is a critical skill in software development. Whether you're working with databases, user input, or complex data structures, understanding how to manipulate and format that data is paramount. In this blog post, we will explore how Java can be utilized to efficiently handle data transformation, particularly focusing on converting vertical data into horizontal formats, a task that is commonly required in data processing and reporting.
Understanding Data Transformation
Data transformation refers to the process of converting data from one format or structure into another. The most common scenarios include:
- Changing Data Formats: This includes converting raw strings into structured objects, such as transforming JSON strings into Java objects.
- Restructuring Data: This involves altering the orientation of datasets, like changing vertical data representation to horizontal.
- Data Cleaning: This includes removing unnecessary data, filling in missing values, and ensuring data integrity.
For reference and further reading, you can check out the article "Transforming Vertical Data to Horizontal in SQL" for detailed SQL transformations at infinitejs.com/posts/transforming-vertical-data-to-horizontal-in-sql.
The Need for Vertical-to-Horizontal Transformation
In database terms, vertical data often arises from normalization, where data is stored in tables for flexibility. However, for reporting or visualization, this vertical format must often be transformed to a horizontal layout, or a denormalized format.
Example Scenario: Employee Data
Consider a scenario where we have employee data in a vertical format as follows:
| Employee ID | Attribute | Value | |-------------|----------------|-------------| | 1 | Name | John Smith | | 1 | Department | Engineering | | 1 | Salary | 70000 | | 2 | Name | Jane Doe | | 2 | Department | Marketing | | 2 | Salary | 80000 |
The goal is to transform this data to a horizontal format where each employee’s details are laid out in a single row:
| Employee ID | Name | Department | Salary | |-------------|------------|-------------|--------| | 1 | John Smith | Engineering | 70000 | | 2 | Jane Doe | Marketing | 80000 |
Implementing Data Transformation in Java
Java provides various data structures and methods that can help us achieve this transformation effectively.
Step 1: Define the Data Structure
First, we will need to define a class to represent our data for easy manipulation and access.
public class Employee {
private int id;
private String name;
private String department;
private double salary;
// Constructor
public Employee(int id, String name, String department, double salary) {
this.id = id;
this.name = name;
this.department = department;
this.salary = salary;
}
// Getters
public int getId() { return id; }
public String getName() { return name; }
public String getDepartment() { return department; }
public double getSalary() { return salary; }
@Override
public String toString() {
return "Employee{" +
"id=" + id +
", name='" + name + '\'' +
", department='" + department + '\'' +
", salary=" + salary +
'}';
}
}
Why use a Class?
By defining an Employee
class, we encapsulate the data associated with each employee, allowing for more organized code and easier manipulation later in the transformation process.
Step 2: Reading and Transforming Vertical Data
Next, we'll simulate the reading of vertical data. For simplicity, assume we have this data in an array or a list. We will iterate through it and populate a map to convert from vertical to horizontal format.
import java.util.*;
public class DataTransformation {
public static void main(String[] args) {
List<Map<String, String>> verticalData = Arrays.asList(
Map.of("Employee ID", "1", "Attribute", "Name", "Value", "John Smith"),
Map.of("Employee ID", "1", "Attribute", "Department", "Engineering"),
Map.of("Employee ID", "1", "Attribute", "Salary", "70000"),
Map.of("Employee ID", "2", "Attribute", "Name", "Jane Doe"),
Map.of("Employee ID", "2", "Attribute", "Department", "Marketing"),
Map.of("Employee ID", "2", "Attribute", "Salary", "80000")
);
List<Employee> employees = transformData(verticalData);
for (Employee employee : employees) {
System.out.println(employee);
}
}
public static List<Employee> transformData(List<Map<String, String>> verticalData) {
Map<Integer, Employee> employeeMap = new HashMap<>();
for (Map<String, String> entry : verticalData) {
int id = Integer.parseInt(entry.get("Employee ID"));
String attribute = entry.get("Attribute");
String value = entry.get("Value");
employeeMap.putIfAbsent(id, new Employee(id, "", "", 0));
Employee employee = employeeMap.get(id);
switch(attribute) {
case "Name":
employeeMap.put(id, new Employee(id, value, employee.getDepartment(), employee.getSalary()));
break;
case "Department":
employeeMap.put(id, new Employee(id, employee.getName(), value, employee.getSalary()));
break;
case "Salary":
employeeMap.put(id, new Employee(id, employee.getName(), employee.getDepartment(), Double.parseDouble(value)));
break;
}
}
return new ArrayList<>(employeeMap.values());
}
}
Why Use a Map?
Using a HashMap
allows us to group attributes associated with the same employee together, enabling efficient lookups and updates as we read each piece of data. The putIfAbsent
method ensures that we only create a new Employee
instance if it doesn't already exist.
Step 3: Output the Results
When you run the above main
method, you will see the transformed output, showing each employee’s details structured horizontally.
The Bottom Line
In this blog post, we examined how to transform vertical data into a horizontal representation using Java. By using collections effectively, leveraging objects for encapsulation, and applying straightforward logic, we can achieve efficient data transformation.
Efficient data handling is a key component in software development, especially as your applications scale. Mastering these techniques not only enhances your coding repertoire but also prepares you for real-world data challenges encountered in various fields, from finance to web analytics.
For more complex data manipulations and transformation insights, explore other resources or articles related to data processing, such as Transforming Vertical Data to Horizontal in SQL.
Keep coding, and embrace the power of data in your applications!