Mastering Java: How to Style Your App with Effective CSS
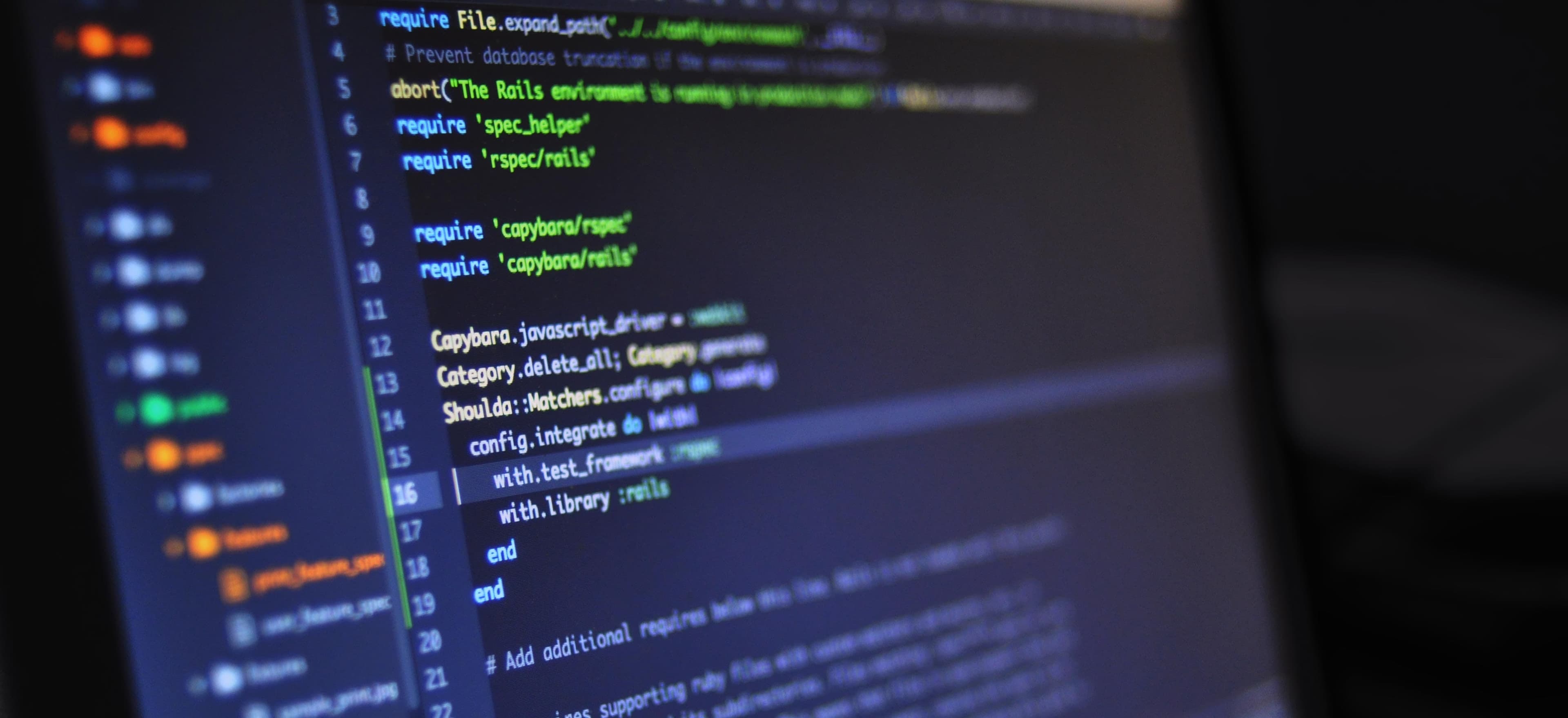
- Published on
Mastering Java: How to Style Your App with Effective CSS
When developing applications, Java stands as a strong pillar in the world of programming. It boasts resilience, versatility, and a robust framework for backend development. However, even with excellent backend coding, a poorly styled front end can compromise user engagement. CSS (Cascading Style Sheets) comes into play, allowing you to elevate the visual aspect of your applications significantly.
In this guide, you'll learn how to leverage CSS in your Java applications to create appealing interfaces while keeping performance in focus. This article pulls together essential principles from both Java coding and effective CSS design strategies. Our goal? To sharpen your technical skills and enhance your application experience.
Understanding the Role of CSS in Java Applications
Java is predominantly used for backend development, but the user interface (UI) does not come from Java alone. For Java desktop applications, CSS has found its way into JavaFX, a powerful framework used for designing rich client applications.
What is CSS?
CSS is a style sheet language that dictates how HTML elements are displayed. It is crucial for controlling layout, colors, fonts, and other visual elements in web applications. In JavaFX, you can style UI components using CSS files, enabling you to separate design from functionality.
Why Style Your Application?
- User Experience: An attractive UI promotes user retention.
- Branding: Consistency in styling develops brand recognition.
- Maintainability: A well-styled application with separated concerns (CSS for styling, Java for logic) is easier to maintain.
Introducing JavaFX and CSS
JavaFX applications can leverage CSS to improve their UI. Consider a basic JavaFX application that displays a button. Here’s how you can style that button using CSS.
Simple JavaFX Example
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class SimpleButtonApp extends Application {
@Override
public void start(Stage primaryStage) {
Button btn = new Button();
btn.setText("Click Me!");
btn.setStyle("-fx-background-color: lightblue;"); // Inline CSS for quick styling
StackPane root = new StackPane();
root.getChildren().add(btn);
Scene scene = new Scene(root, 300, 250);
primaryStage.setTitle("JavaFX Button Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why Inline CSS?
In the code above, we use inline CSS to modify the background color of the button. However, while inline styling is effective for quick prototyping, it is not ideal for production-level applications.
Moving to External CSS
To maintain scalability and organization, it's recommended to use external CSS files. Here’s how:
Create a styles.css
file:
.button {
-fx-background-color: lightblue;
-fx-text-fill: black;
-fx-font-size: 16px;
-fx-padding: 10px 20px; /* Create spacing */
}
Modify your Java code to utilize the external CSS:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class StyledButtonApp extends Application {
@Override
public void start(Stage primaryStage) {
Button btn = new Button();
btn.setText("Click Me!");
btn.getStyleClass().add("button"); // Adding CSS class
StackPane root = new StackPane();
root.getChildren().add(btn);
Scene scene = new Scene(root, 300, 250);
scene.getStylesheets().add("styles.css"); // Link the CSS file
primaryStage.setTitle("JavaFX Styled Button Example");
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
Why Use External CSS?
Using external CSS keeps your styling organized and allows for alterations without modifying the Java code directly. This method supports maintainability and ease of updates.
Advanced CSS Techniques
Responsive Design
Responsive design is crucial for creating a user-friendly interface. JavaFX allows for responsive layouts through the use of layout panes like VBox
, HBox
, and GridPane
.
.button {
-fx-background-color: lightblue;
-fx-padding: 10px 20px;
}
.button:hover {
-fx-background-color: darkblue; /* Darker shade on hover */
}
Combining this with JavaFX’s layout capabilities, you can create applications that adapt to various screen sizes.
Hover Effects
Creating hover effects boosts interactivity. The above CSS snippet illustrates this by changing the button color when hovered. Such feedback is essential for enhancing user experience.
Complementing Strategies
While you focus on Java and CSS, remember that other techniques can augment your development process. An article like Boost Your CSS Battle Scores: 4 Proven Strategies offers an excellent framework for enhancing your CSS skill set. These strategies will refine your methods, resulting in finer styling and layout designs.
Performance Considerations
While CSS enhances appearance, it can also affect application performance. Here are quick tips to enhance speed while using CSS in JavaFX:
- Minimize CSS File Size: Use tools to reduce file sizes and remove unused styles.
- Use Shorthands: For example, combine margins or padding into a single declaration.
- Limit Use of Animation: Overuse may lead to performance lag; keep effects subtle and purposeful.
Wrapping Up
Styling Java applications with CSS is not only rewarding but necessary for crafting beautiful and engaging user experiences. By using JavaFX and CSS, you create a dynamic duo that ensures your app appeals visually while providing excellent functionality.
Your journey towards mastering Java with effective CSS is just beginning. By abiding by the principles and practices discussed in this article, you can achieve a high level of proficiency in styling your applications.
Explore the fundamentals, stay updated on CSS strategies, and always look out for new CSS and JavaFX advancements to help bolster your project’s appeal. Interested in further enhancing your CSS skills? Don't forget to check out articles like Boost Your CSS Battle Scores: 4 Proven Strategies to continually refine your approach.
Additional Resources
By implementing the strategies discussed herein, you not only set a solid foundation for your Java applications but also boost user engagement and satisfaction—crucial elements in today’s competitive landscape. Happy coding!