Maximize Java Framework Performance: CSS Integration Tips
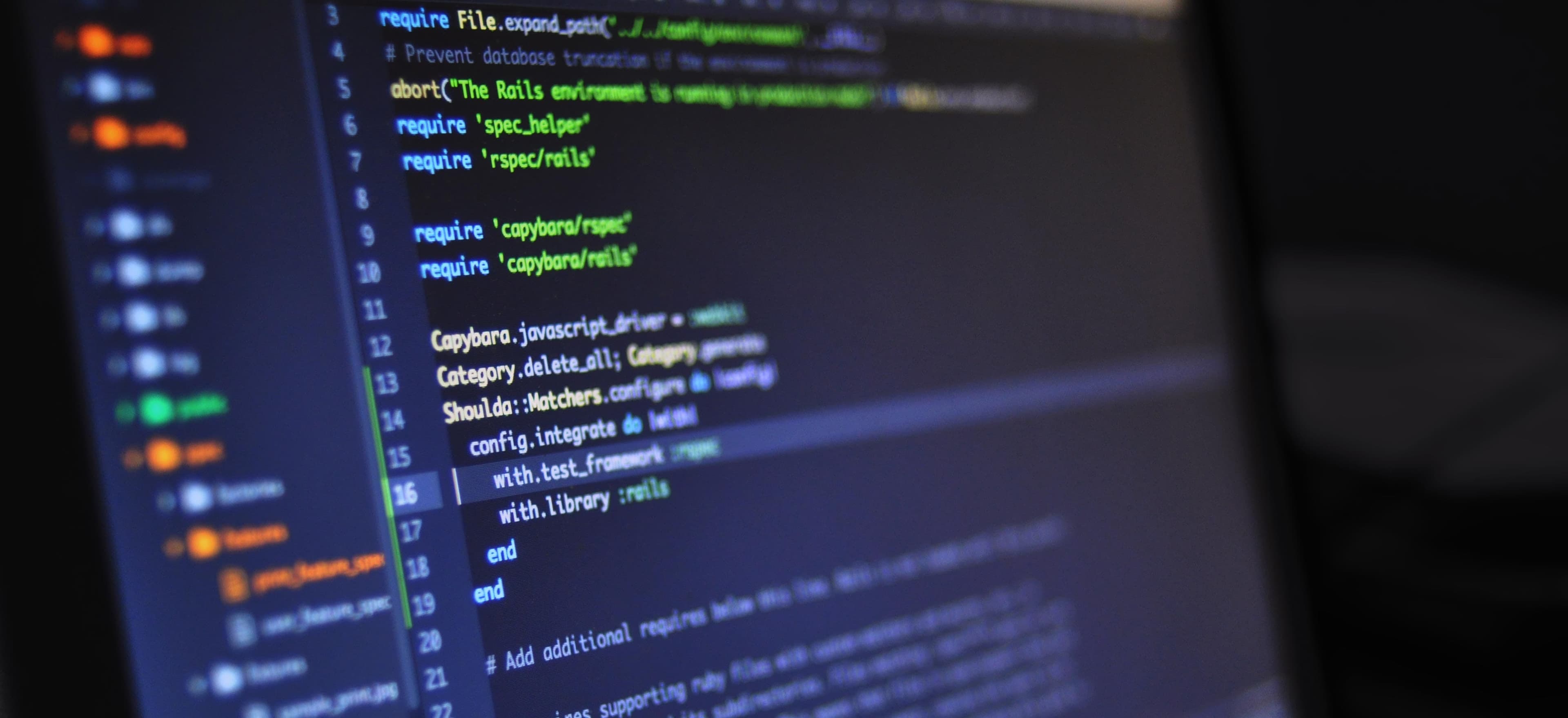
- Published on
Maximize Java Framework Performance: CSS Integration Tips
Java frameworks have revolutionized the way developers create web applications. They offer structure, reusable components, and improved developer productivity. However, maximizing the performance of your Java application is an ongoing concern that often intersects with how well the application integrates with cascading style sheets (CSS). In this article, we will explore effective strategies to seamlessly blend Java frameworks with CSS, enhancing your application's overall performance.
Understanding the Importance of CSS in Java Applications
CSS is not just a tool for styling; it significantly impacts the performance and user experience of your web application. Proper integration and optimization of CSS can lead to faster load times, improved rendering speed, and enhanced responsiveness. For example, studies indicate that slow rendering can lead to user frustration and increased bounce rates.
As such, having a well thought out CSS integration strategy within your Java application framework is crucial. Below are several tips to maximize performance through effective CSS usage.
1. Minimize and Optimize CSS Files
Why Minimize?
Larger CSS files can slow down your application's loading time. Minimizing your CSS files decreases their size, allowing your web pages to load faster. This is particularly vital when using Java frameworks, as heavy CSS can offset the performance benefits of Java's efficient backend.
Example of How to Minimize CSS
You can use tools like CSSNano or CleanCSS to minimize your files. Here's a simple example of how to do this using Node.js and npm:
npm install --save-dev cssnano
Then in your build configuration:
const cssnano = require('cssnano');
const minimizeCSS = (css) => {
return cssnano.process(css, { from: undefined }).then(result => result.css);
};
This function will automatically minimize your CSS, reducing file size without losing style definitions.
2. Leverage CSS Preprocessors
What Are CSS Preprocessors?
CSS preprocessors like SASS or LESS extend the capabilities of CSS by adding features such as variables, nesting, and mixins. These features promote cleaner, more maintainable code. Using preprocessors allows you to maintain a cleaner codebase when integrating CSS into your Java framework.
Getting Started with SASS
First, install SASS:
npm install -g sass
Then create a .scss
file:
$primary-color: #333;
body {
font-family: Arial, sans-serif;
color: $primary-color;
h1 {
font-size: 2em;
}
}
Compile to CSS:
sass styles.scss styles.css
This compiled CSS is lighter and easier to maintain.
3. Use CSS Modules or Scoped CSS
What Are CSS Modules?
CSS Modules help in avoiding global namespace issues by encapsulating styles to specific components. This is particularly useful in Java frameworks like Spring Boot when modularizing your frontend with React or Angular.
Implementing CSS Modules with React
Install necessary dependencies:
npm install --save-dev react-css-modules
Use your styles as follows:
import styles from './Component.module.css';
const MyComponent = () => {
return <div className={styles.container}>Hello, World!</div>;
};
CSS Modules ensure that the styles are scoped to the component, preventing unwanted cascades, which can bloat the final CSS file size.
4. Load CSS Asynchronously
Why Load Asynchronously?
Loading CSS asynchronously can improve page loading time by allowing HTML content to render before the CSS is completely downloaded. This can be done using the link
tag with the rel="preload"
attribute.
Example Implementation
Add the following to your HTML header:
<link rel="preload" href="styles.css" as="style" onload="this.onload=null;this.rel='stylesheet'">
<noscript><link rel="stylesheet" href="styles.css"></noscript>
This code snippet starts the CSS loading in the background, streamlining the user experience.
Additional Tips for Effective CSS Integration
5. Avoid Inline Styles
Inline styles can be hard to maintain and override. Modular CSS and class-based styling are usually preferable. They provide a clean structure and ease future updates.
6. Use CSS Grid and Flexbox
Modern CSS layout techniques like Grid and Flexbox are performance-optimized and reduce the need for JavaScript-driven layouts. This minimizes CSS overrides and enhances application efficiency.
7. Keep CSS Selectors Simple
Use fewer and simpler selectors. Complex selectors can slow down rendering, affecting performance. For example, prefer classes over IDs as they often result in better performance in CSS engines.
To Wrap Things Up
Integrating CSS efficiently within your Java framework is essential for maximizing performance. The techniques discussed in this article—optimizing file sizes, leveraging preprocessors, using CSS Modules, loading styles asynchronously, avoiding inline styles, and simplifying selectors—can significantly enhance your application's user experience.
Want to Boost Your CSS Battle Scores?
For further reading on CSS optimization strategies, refer to the article Boost Your CSS Battle Scores: 4 Proven Strategies.
Implementing these strategies in your Java applications not only improves performance but also ensures a smooth user experience. Remember, a well-integrated and optimized frontend can significantly improve the reputation and usability of your application.