Securing NoSQL Databases in Spring Boot Applications
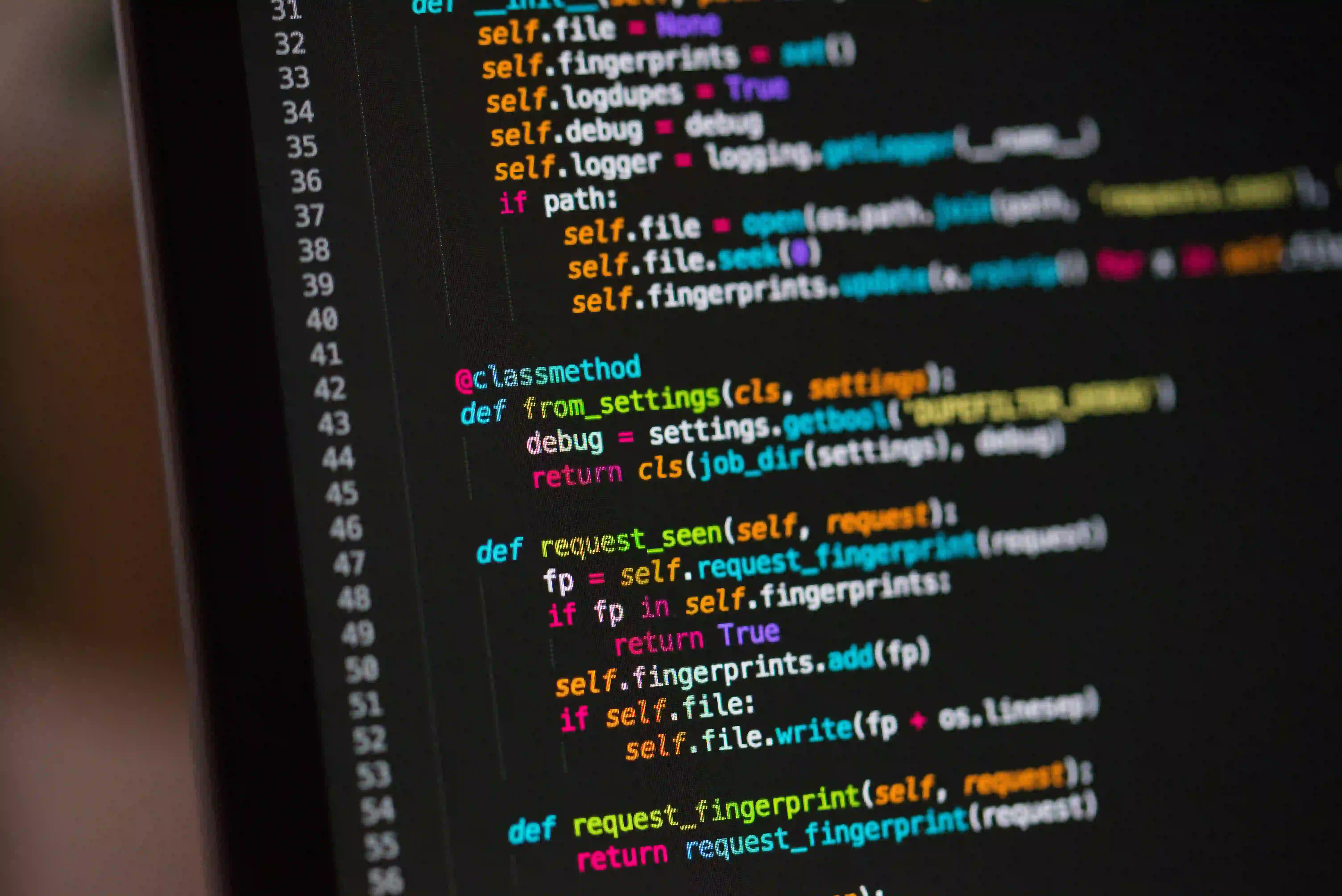
Securing NoSQL Databases in Spring Boot Applications
As the demand for scalable, flexible database solutions continues to rise, NoSQL databases like MongoDB, Cassandra, and DynamoDB have become mainstream choices for Spring Boot applications. However, with great power comes great responsibility—especially when it comes to security. In this blog post, we'll explore various strategies to secure NoSQL databases in Spring Boot applications, providing code snippets, best practices, and relevant resources.
Understanding NoSQL Databases Security Risks
Before we dive into the security measures, it's crucial to understand the unique risks associated with NoSQL databases:
- Data Exposure: NoSQL databases can expose sensitive information due to improper configurations or mismanagement.
- Injection Attacks: Similar to SQL injection, NoSQL databases can be susceptible to injection attacks.
- Insecure APIs: APIs that lack authentication can open the database to unauthorized access.
- Insufficient Access Controls: Inadequate user permissions can lead to unwanted data manipulation or leakage.
Understanding these risks is the first step toward a more secure application.
Fundamental Security Practices
Now that we've outlined the potential risks, let's discuss some fundamental security practices you can implement in Spring Boot applications using NoSQL databases.
1. Use Environment Variables for Configuration
Keep sensitive information, such as database credentials, out of your codebase by utilizing environment variables. Spring Boot enables easy access to environment properties using @Value
or Environment
.
Example:
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class MongoConfig {
@Value("${MONGODB_URI}")
private String mongoUri;
// This ensures credentials are not hardcoded
public String getMongoUri() {
return mongoUri;
}
}
In this example, the MongoDB URI is fetched from environment variables, keeping secrets secure and allowing easy updates without code changes.
2. Enable Authentication and Authorization
Most NoSQL databases, like MongoDB, come with built-in authentication. Always enable it and manage users and roles effectively. In Spring Boot, use Spring Security for managing user authentication and authorization.
Example: Integrating Spring Security
First, include the dependency in your pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-security</artifactId>
</dependency>
Then, configure basic security in your application:
import org.springframework.context.annotation.Configuration;
import org.springframework.security.config.annotation.authentication.builders.AuthenticationManagerBuilder;
import org.springframework.security.config.annotation.web.builders.HttpSecurity;
import org.springframework.security.config.annotation.web.configuration.EnableWebSecurity;
import org.springframework.security.config.annotation.web.configuration.WebSecurityConfigurerAdapter;
@Configuration
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("admin").password("{noop}password").roles("ADMIN");
}
@Override
protected void configure(HttpSecurity http) throws Exception {
http
.authorizeRequests()
.anyRequest().authenticated()
.and()
.httpBasic();
}
}
In the above code, we establish in-memory user authentication for demonstration purposes. Never use this in production! Implement a robust authentication mechanism such as OAuth2 or JWT in practice.
3. Secure Network Connections
Always use TLS/SSL for connections to your NoSQL database. This ensures that data is encrypted when in transit, minimizing the risk of man-in-the-middle attacks.
Example: Configuring TLS for MongoDB involves editing the mongod.conf
file:
net:
ssl:
mode: requireSSL
PEMKeyFile: /path/to/your/mongodb.pem
In Spring Boot, you can specify the SSL property in your application properties file:
spring.data.mongodb.uri=mongodb://<username>:<password>@localhost:27017/mydatabase?ssl=true
4. Limit Database Permissions
Creating roles with the principle of least privilege is vital. Only grant permission to resources strictly necessary for users and applications. In MongoDB, you can create roles with specific permissions.
Example: Define a custom user role:
db.createRole(
{
role: "readWriteRole",
privileges: [
{ resource: { db: "mydatabase", collection: "" }, actions: [ "find", "insert", "update" ] }
],
roles: []
}
)
Assign this role to your application user, ensuring that it only has the access it requires.
5. Monitor and Audit Access
Vigilance is crucial for maintaining security. Monitor access logs for unusual activity and audit database actions regularly. Spring Boot can integrate various monitoring tools to keep track of user access.
Example: Use Spring Boot Actuator for monitoring.
Add the following dependency in pom.xml
:
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
Then activate the endpoints in application.properties
:
management.endpoints.web.exposure.include=*
With this setup, you can monitor endpoint access through your application’s /actuator
endpoint.
Advanced Security Strategies
Once you have implemented fundamental security measures, you can delve into more advanced strategies.
1. Use Encryption
Data at rest must also be encrypted to protect it from unauthorized access. Many NoSQL databases offer built-in encryption options. For MongoDB, you can use MongoDB Encrypted Storage Engine to secure your data.
2. Implement API Gateway
Using an API gateway can provide an additional layer of security by acting as a single entry point for authentication and routing requests. Tools like Spring Cloud Gateway can help implement this architecture smoothly.
3. Regularly Update Dependencies
Regularly monitoring and updating your dependencies can help you mitigate vulnerabilities. Use tools like Maven’s dependency-check
plugin or OWASP Dependency-Check for this purpose.
4. Use Web Application Firewalls (WAF)
If your application is exposed to the internet, consider using a WAF for additional security. A WAF can help filter, monitor, and block HTTP traffic to and from your web application.
Final Considerations
Securing NoSQL databases in Spring Boot applications involves a continuous process of assessment and improvement. By following best practices, enabling vital security configurations, monitoring access, and incorporating advanced strategies, you can significantly reduce security risks.
For further reading, consider checking out:
In today's data-driven world, prioritizing security is not just essential; it is a necessity. By implementing these strategies, you can help ensure that your application remains secure while providing flexibility and performance.
Stay secure!