Overcoming Deployment Challenges in Cloud-Native Apps
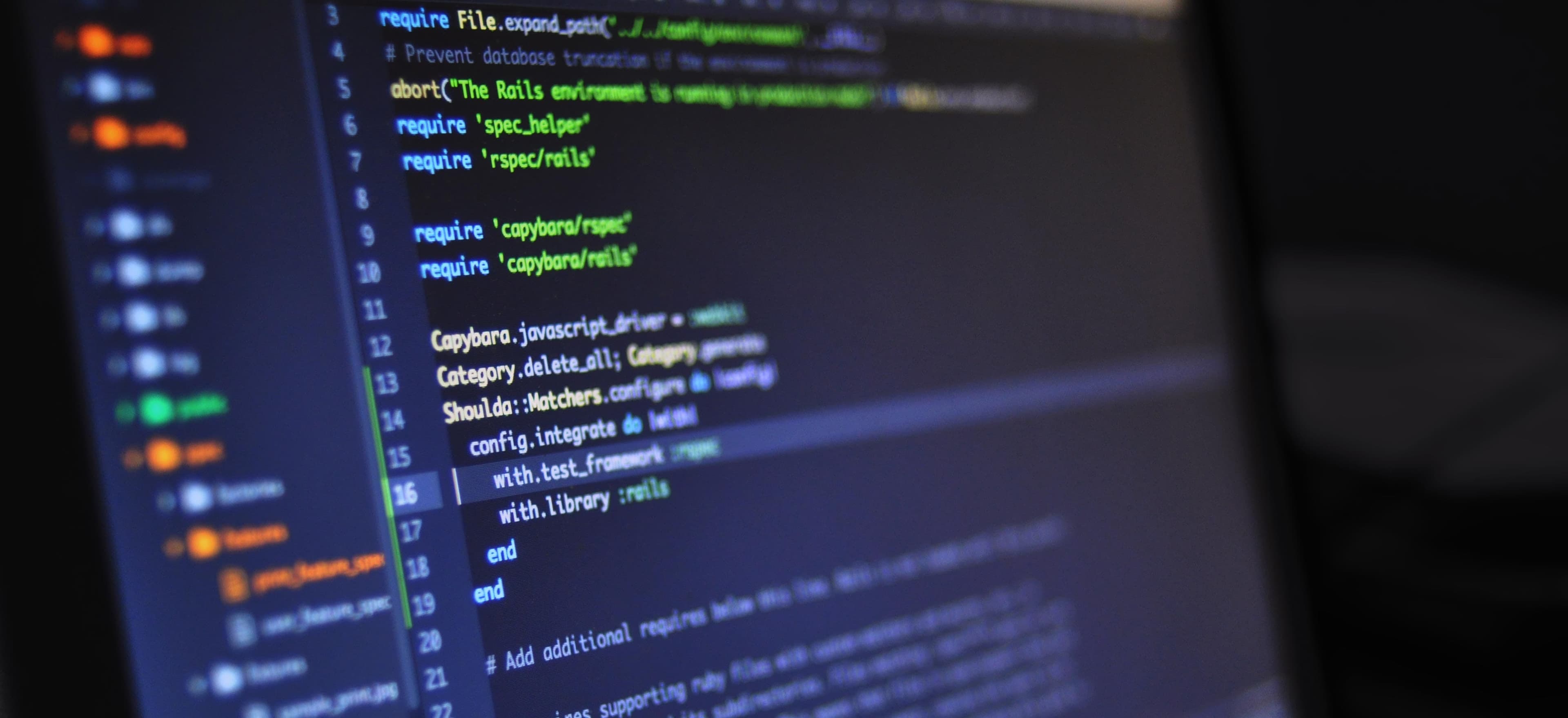
- Published on
Overcoming Deployment Challenges in Cloud-Native Apps
The advent of cloud computing has transformed the way businesses deploy applications. With the possibility to enhance scalability, availability, and resilience, cloud-native architectures are rapidly becoming the go-to choice for modern developers. However, deploying cloud-native applications is not without its challenges. In this post, we will explore these challenges in depth, discuss some common obstacles, and present effective solutions to overcome them.
Understanding Cloud-Native Architecture
Before diving into the deployment challenges, let’s clarify what cloud-native means. Cloud-native applications are built to leverage the advantages of cloud computing delivery models. These applications often consist of microservices, are designed for elasticity, and can harness container technologies. This adaptability is the reason why they have gained immense popularity.
Key Principles of Cloud-Native Applications:
- Microservices: Breaking down applications into smaller, independent services.
- Containers: Packaging software into standardized units called containers to simplify deployment and scaling.
- Dynamic Orchestration: Managing microservices effectively, often with tools like Kubernetes.
For more details on cloud-native principles, you can refer to 12-Factor App.
Common Deployment Challenges
1. Complexity in Management
Deploying multiple microservices can introduce significant complexity. Each service may have its own deployment cycle, dependencies, and configurations. Managing these can become chaotic.
Solution
Employ techniques like Kubernetes or Docker Swarm for orchestration. These tools help in managing containerized applications smoothly by automating deployment, scaling, and operations of application containers.
# Simple Kubernetes Deployment Manifest
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-app
spec:
replicas: 3 # Scale to 3 instances
selector:
matchLabels:
app: my-app
template:
metadata:
labels:
app: my-app
spec:
containers:
- name: my-app-container
image: my-app:latest # Use the latest image of our app
ports:
- containerPort: 80
Why? This configuration allows you to scale your application seamlessly. By wishing for a specific number of replicas, Kubernetes takes care of managing the desired instances.
2. Configuration Management
With numerous microservices, ensuring correct configurations across different environments can become tedious. Misconfigurations can lead to runtime failures.
Solution
Utilize configuration management tools such as HashiCorp's Consul or Kubernetes ConfigMaps and Secrets to handle configuration parameters efficiently.
# Example of a ConfigMap in Kubernetes
apiVersion: v1
kind: ConfigMap
metadata:
name: my-app-config
data:
DATABASE_URL: "db.example.com"
API_KEY: "your-api-key"
Why? Using ConfigMaps allows your microservices to access configurations without hardcoding them into your application. This promotes security and maintainability.
3. Continuous Integration/Continuous Delivery (CI/CD)
Setting up effective CI/CD pipelines that cater to microservices' needs can be challenging. You want to ensure that automated tests are integrated into your workflow without excessive overhead.
Solution
Incorporate CI/CD tools like Jenkins, GitLab CI, or GitHub Actions in conjunction with testing frameworks to facilitate efficient deployments.
# Sample GitHub Actions workflow
name: CI/CD Pipeline
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Build Docker image
run: |
docker build -t my-app:latest .
- name: Run tests
run: |
docker run my-app:latest test
Why? This GitHub Actions workflow automates the testing process every time there's a push to the main branch, thus facilitating a safer deployment practice.
4. Security Concerns
Deploying cloud-native applications increases the attack surface. Microservices communicating over APIs need to be secured, and vulnerabilities must be handled proactively.
Solution
Adopt security practices like zero-trust architecture, API gateways, and service meshes (like Istio) to ensure secure communication between services.
# Example of securing a service with Istio
apiVersion: authentication.istio.io/v1alpha1
kind: Policy
metadata:
name: my-app-secure
spec:
targets:
- name: my-app
peerauthentication:
mtls:
mode: STRICT
Why? By enforcing mutual TLS, we ensure that communication between services is encrypted and authenticated, thus enhancing security.
5. Handling State
In a cloud-native world, many developers shy away from state management, leading to what can feel like a loss of control and data inconsistency.
Solution
Implement external storage solutions such as Amazon RDS or Azure Cosmos DB. Use database patterns like event sourcing or Command Query Responsibility Segregation (CQRS) for state management.
// Example of using Event Sourcing in Java
public class Account {
private List<Event> changes = new ArrayList<>();
public void deposit(Money amount) {
applyChange(new MoneyDeposited(amount));
}
private void applyChange(Event event) {
changes.add(event);
// Update account state based on event
}
}
Why? By utilizing the event sourcing pattern, you're capturing all state changes as a sequence of events, making it easier to reproduce the state at any point in time.
Final Considerations
Deploying cloud-native applications presents unique challenges, but they are surmountable with the right approaches and tools. By embracing container orchestration, configuration management, CI/CD practices, robust security methods, and managing state effectively, developers can mitigate these challenges and enjoy the full benefits of cloud-native architectures.
As you venture into deploying cloud-native apps, remember that each application has its distinct needs. It's pivotal to evaluate your application’s architecture and select solutions that best align with your operational strategies and objectives. By doing so, you not only overcome challenges but also harness the full potential of modern cloud infrastructure.
For further reading, you might find these resources helpful:
Happy deploying!
Checkout our other articles