Common Spring Boot Fast MVC Pitfalls and How to Avoid Them
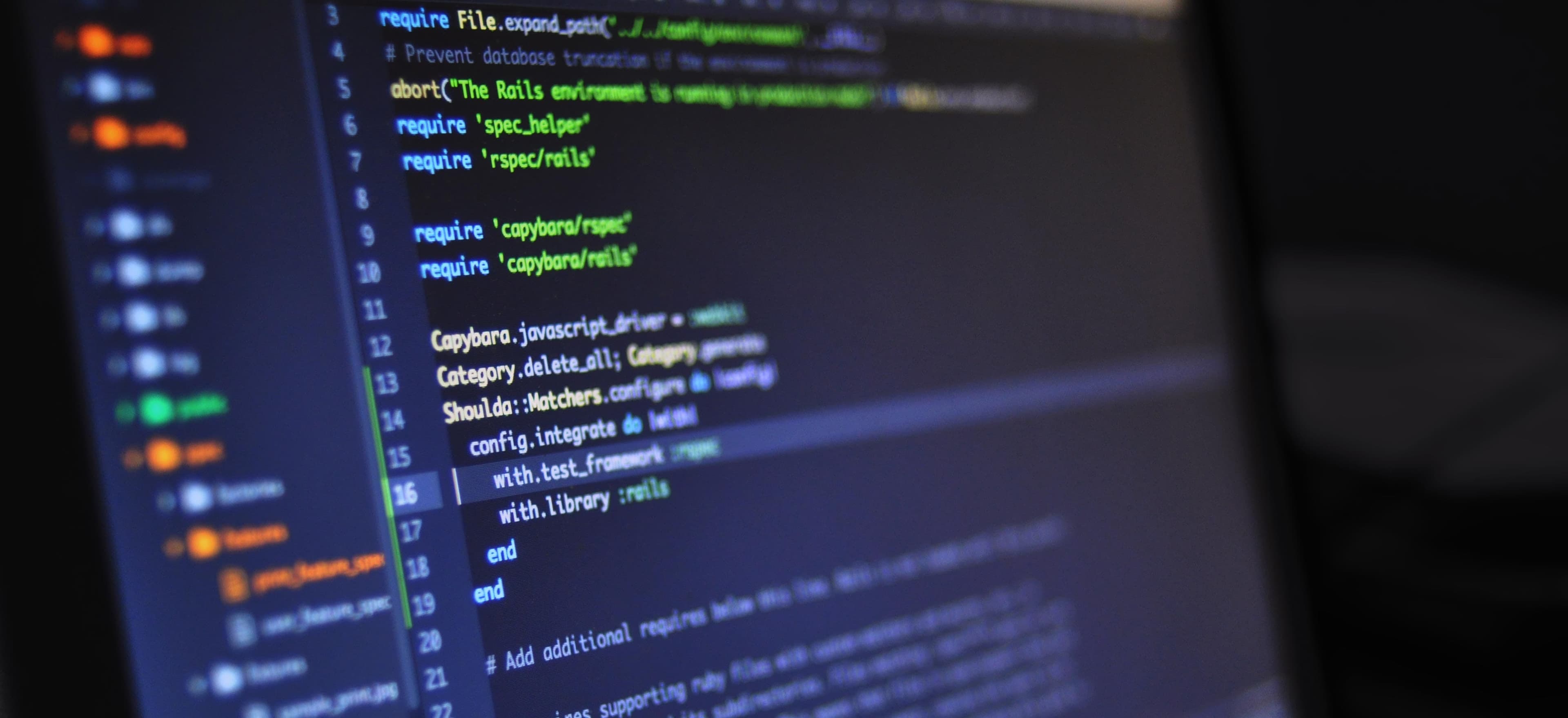
- Published on
Common Spring Boot Fast MVC Pitfalls and How to Avoid Them
Spring Boot is a powerful framework that simplifies the process of building Java applications. Fast MVC (Model-View-Controller) is a web framework that helps developers implement the MVC design pattern efficiently. However, even experienced developers can stumble upon common pitfalls when working with Spring Boot Fast MVC. This blog post discusses these pitfalls and offers practical solutions to avoid them.
Understanding Spring Boot and Fast MVC
Before delving into the pitfalls, let's briefly recap what Spring Boot and Fast MVC entail.
Spring Boot is designed to make it easy to create stand-alone, production-grade Spring-based applications. It consolidates various Spring features and is equipped with default configurations, minimizing the time developers spend on setup.
Fast MVC is an abstraction over Spring MVC, providing a more streamlined approach to building web applications. This allows developers to focus on application logic rather than boilerplate code.
Pitfall #1: Not Leveraging Spring Boot Starter Dependencies
One of the biggest advantages of Spring Boot is its starter dependencies, particularly for web applications. Many developers fall into the trap of manually adding dependencies instead of using the convenience of starters.
Why This is a Pitfall?
When you add dependencies manually, you risk version conflicts and missing transitive dependencies that come bundled with the starters. This can lead to unexpected bugs and errors.
How to Avoid It
Instead of adding individual dependencies one by one, use Spring Boot starters. Here's an example of how to utilize the Spring Boot starter for web applications.
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
By using spring-boot-starter-web
, you automatically include important dependencies like Spring MVC, Jackson for JSON serialization, and more.
Pitfall #2: Ignoring Properties Configuration
Another common mistake is neglecting the application properties configuration. Spring Boot applications come with a default application.properties
file, but many developers do not customize it.
Why This is a Pitfall?
Not configuring properties can lead to performance issues, security vulnerabilities, and application misbehavior.
How to Avoid It
Make sure to configure properties to suit your application needs. Here’s an example of configuring port and context path in application.properties
:
server.port=8081
server.servlet.context-path=/api
Setting your desired server port and context path helps in organizing your application endpoints systematically and reduces conflicts with other applications.
Pitfall #3: Not Using Clear Endpoint Mappings
When defining REST controllers, some developers overlook the importance of clear and concise endpoint mappings. This leads to confusion about API endpoints, making it difficult for other developers to consume your API.
Why This is a Pitfall?
Unclear or inconsistent endpoint mappings can lead to increased learning curves, bugs, and miscommunication among team members.
How to Avoid It
Follow a RESTful design for your endpoint mappings. Here's a simple example:
@RestController
@RequestMapping("/users")
public class UserController {
@GetMapping("/{id}")
public User getUserById(@PathVariable Long id) {
// Method implementation
}
@PostMapping
public User createUser(@RequestBody User user) {
// Method implementation
}
}
By structuring your endpoints clearly, you make your API intuitive and easier to use, which is essential for both development and maintenance.
For a deeper dive into Spring MVC best practices, consider checking out Spring's official documentation.
Pitfall #4: Ignoring Exception Handling
Many developers either ignore or poorly implement error handling in their Fast MVC applications. This can lead to a frustrating user experience and vague error messages.
Why This is a Pitfall?
Failing to handle exceptions gracefully can expose internal errors to users or make it difficult to debug issues later.
How to Avoid It
Utilize @ControllerAdvice
to create a centralized exception handling mechanism. Here’s an example:
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(UserNotFoundException.class)
public ResponseEntity<ErrorResponse> handleUserNotFound(UserNotFoundException ex) {
ErrorResponse errorResponse = new ErrorResponse("User not found", ex.getMessage());
return new ResponseEntity<>(errorResponse, HttpStatus.NOT_FOUND);
}
}
In this example, a general exception handler addresses the UserNotFoundException
, providing a clear response format via a standardized ErrorResponse
object. This makes debugging and user experience more manageable.
Pitfall #5: Overlooking CORS Configuration
With modern web applications, CORS (Cross-Origin Resource Sharing) configuration has become crucial. New developers often overlook this, leading to security service issues or blocked requests.
Why This is a Pitfall?
If CORS is not configured, your application may reject requests from desired origins, leading to functionality limitations.
How to Avoid It
You can easily configure CORS for your Spring Boot application. Here's how:
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.CorsRegistry;
import org.springframework.web.servlet.config.annotation.WebMvcConfigurer;
@Configuration
public class WebConfig implements WebMvcConfigurer {
@Override
public void addCorsMappings(CorsRegistry registry) {
registry.addMapping("/**")
.allowedOrigins("http://localhost:3000")
.allowedMethods("GET", "POST", "PUT", "DELETE");
}
}
This example allows requests from http://localhost:3000
, covering all endpoints in the application. It is crucial for maintaining secure yet flexible application architecture.
Pitfall #6: Unused Annotations
Spring Boot offers many powerful annotations, but developers sometimes misuse or overuse them without fully understanding their implications.
Why This is a Pitfall?
Using annotations unnecessarily can lead to bloated code, complicating the logic and reducing maintainability.
How to Avoid It
Be judicious when applying annotations. Before you use an annotation, verify that it provides additional functionality relevant to your specific context. Always comment on why you need the annotation.
For example, use @Transactional
judiciously for methods that alter database states. This should encapsulate transactional boundaries explicitly.
@Service
public class UserService {
@Transactional
public User addUser(User user) {
// Method implementation
}
}
A simple comment explaining the rationale for this annotation can help future developers understand its necessity.
Closing the Chapter
Spring Boot Fast MVC is a robust framework, but it does come with its set of pitfalls. By leveraging the framework's features — such as starters, clear properties, structured endpoints, robust exception handling, appropriate CORS setup, and thoughtful annotation usage — you can build smoother applications with improved maintainability.
Remember, staying informed and continuously learning about best practices and new features is critical in a rapidly evolving technology landscape. For a more in-depth resource, explore the comprehensive Spring Boot guides available on Baeldung.
By addressing these common pitfalls head-on, you set your Spring Boot Fast MVC applications up for success, ensuring a seamless experience for both developers and users alike.