Overcoming Integration Testing Challenges in Software Development
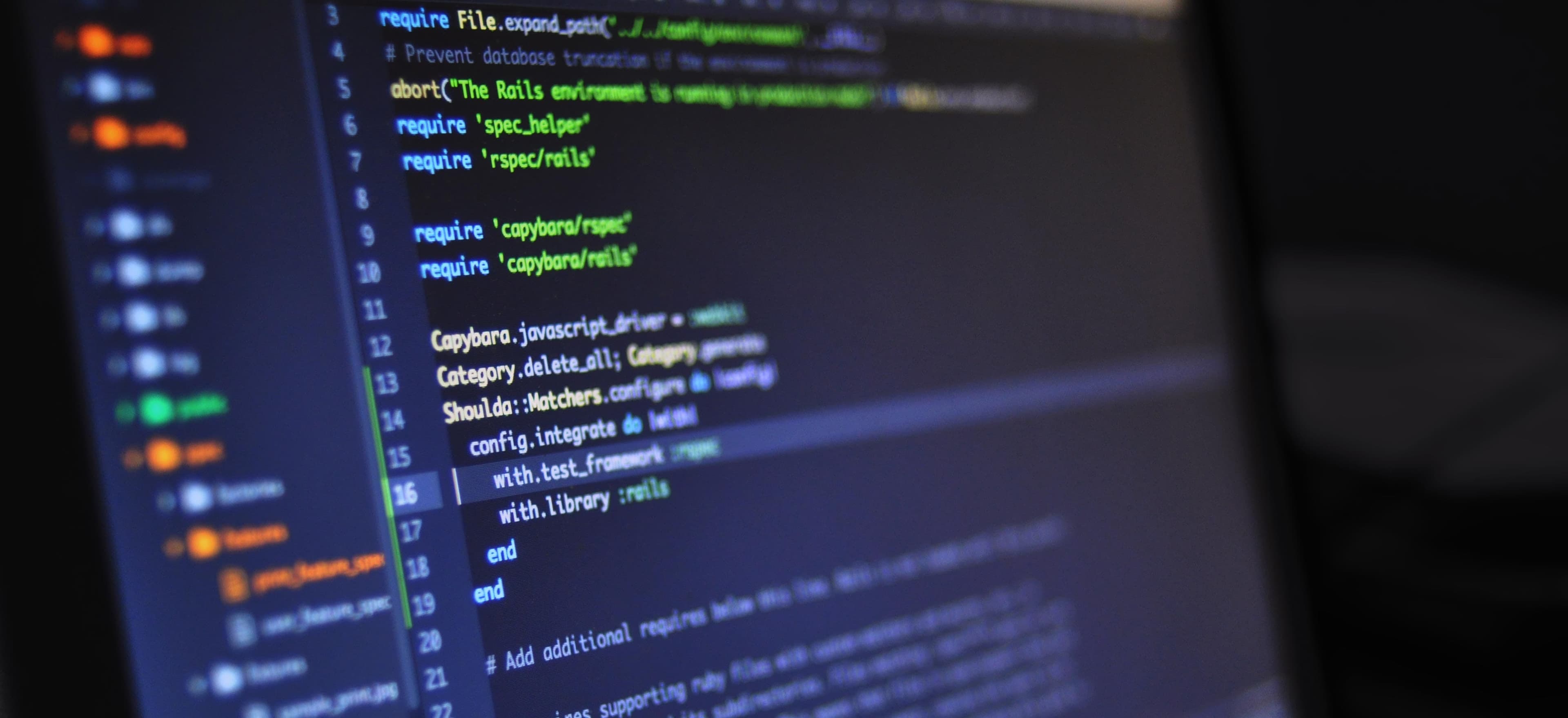
- Published on
Overcoming Integration Testing Challenges in Software Development
In the realm of software development, integration testing plays a crucial role in ensuring that multiple components of a system operate effectively together. While the significance of integration testing is well-recognized, the challenges associated with it can often lead to frustration and setbacks. In this blog post, we will explore common integration testing challenges and practical strategies to overcome them, all while embedding Java examples that showcase effective approaches.
Table of Contents
- Understanding Integration Testing
- Common Integration Testing Challenges
- Solutions to Integration Testing Challenges
- Java Code Snippets for Integration Testing
- Best Practices for Integration Testing
- Conclusion
Understanding Integration Testing
Integration testing is primarily concerned with the interactions between different modules or services in a software application. It aims to identify issues that may not be observable when components are tested in isolation during unit testing. According to the Agile Alliance, integration testing is vital for verifying data exchange between modules and ensuring overall system integrity.
Why Integration Testing Matters
- Detecting Interface Issues: Often, the way two components interact can expose bugs that unit tests fail to catch.
- Ensuring Data Flow: Integration testing checks if data is accurately passed across different modules.
- Confidence in Deployment: A well-tested integration suite instills confidence before a production release.
Common Integration Testing Challenges
Despite its importance, integration testing faces several challenges:
1. Testing Environment Setup
Setting up an environment that closely mirrors production can be daunting. Differences in configurations can lead to inconsistent testing results.
2. Data Management
Properly seeding data for tests is challenging. The lack of a straightforward method to maintain and reset data can result in flaky tests.
3. Timing Issues
Asynchronous processes in modern applications can lead to race conditions, making it difficult to ensure tests run in a specific order.
4. Dependencies on Third-party Services
Testing components that rely on external services introduces variability. If those services are down or fail to respond correctly, it leads to false negatives.
5. Complexity in Test Cases
Identifying and writing test cases for every scenario can be overwhelming, especially in large applications.
Solutions to Integration Testing Challenges
1. Automate Environment Setup
Leveraging tools like Docker can help replicate production environments consistently. By using Docker containers, developers can create isolated environments for integration testing, ensuring all components communicate as expected.
2. Use Mocking and Stubbing
For dependencies on third-party services, libraries like Mockito in Java allow you to simulate responses. This approach circumvents the need for constant connectivity to external services during testing.
3. Implement Test Data Management
Database seeding and data management tools can help maintain the required data for running integration tests. Tools like Testcontainers can help ensure that each test starts with a clean slate.
4. Manage Asynchronous Calls
To effectively test asynchronous processes, consider using tools like Awaitility. It enables you to "wait" for specific conditions to be met before the test proceeds, thus reducing race condition issues.
5. Modularize Tests
Breaking integration tests into smaller, manageable test cases can simplify maintenance. Each test case should focus on a single scenario, making it easier to identify which specific case is failing.
Java Code Snippets for Integration Testing
Snippet 1: Using Mockito for Mocking Dependencies
First, let's look at how to use Mockito to mock a service dependency.
import static org.mockito.Mockito.*;
import org.junit.jupiter.api.Test;
public class UserServiceTest {
@Test
public void testGetUser() {
// Mocking the UserRepository
UserRepository mockRepo = mock(UserRepository.class);
UserService userService = new UserService(mockRepo);
User user = new User(1, "John Doe");
when(mockRepo.findById(1)).thenReturn(user);
// Execute the method to be tested
User result = userService.getUser(1);
// Validation
assertEquals("John Doe", result.getName());
}
}
Why Use Mockito? This approach simulates the repository without needing an actual database. Thus, we can focus on testing the UserService’s logic effectively.
Snippet 2: Using Docker for Environment Setup
Here is a simple example of a Dockerfile
you might use for a Java application testing setup.
FROM openjdk:11-jdk
COPY target/myapp.jar /usr/src/myapp/myapp.jar
WORKDIR /usr/src/myapp
CMD ["java", "-jar", "myapp.jar"]
Why Use Docker? By ensuring that your tests run on a consistent environment, you mitigate issues arising from differences between local and production setups. This leads to more reliable test outcomes.
Snippet 3: Using Awaitility for Asynchronous Tests
To test an asynchronous method, we can use Awaitility as follows:
import org.awaitility.Awaitility;
import static org.hamcrest.Matchers.is;
public class AsyncServiceTest {
@Test
public void testAsyncCall() {
AsyncService asyncService = new AsyncService();
asyncService.performAsyncOperation();
Awaitility.await()
.atMost(5, TimeUnit.SECONDS)
.until(() -> asyncService.getStatus(), is("COMPLETED"));
}
}
Why Use Awaitility? This allows us to specify conditions for an asynchronous operation, which helps in testing scenarios where timely responses are key.
Best Practices for Integration Testing
-
Version Control Testing Scripts: Always keep your test scripts under version control for better tracking.
-
Continuous Integration: Integrate tests in your CI/CD pipeline to ensure they run regularly, catching issues early.
-
Document Tests: Each test should have clear documentation explaining its purpose and scenarios covered.
-
Review Test Coverage: Regularly review test coverage to ensure that you're effectively testing all integration points.
-
Conduct Regular Cleanups: Ensure your test data and environments are regularly cleaned to avoid false positives or negatives.
Wrapping Up
Overcoming integration testing challenges is essential for developing high-quality software. By implementing the strategies discussed in this post and leveraging the power of tools like Mockito, Docker, and Awaitility, you can streamline your testing process, reduce errors, and enhance software reliability.
For developers venturing deeper into integration testing, consider exploring additional resources such as JUnit 5 User Guide and Mockito Documentation. As you tackle these challenges head-on, remember that effective integration testing is a journey towards superior software development practices. Happy Testing!