Mastering Java 8: Common Issues When Reading Files
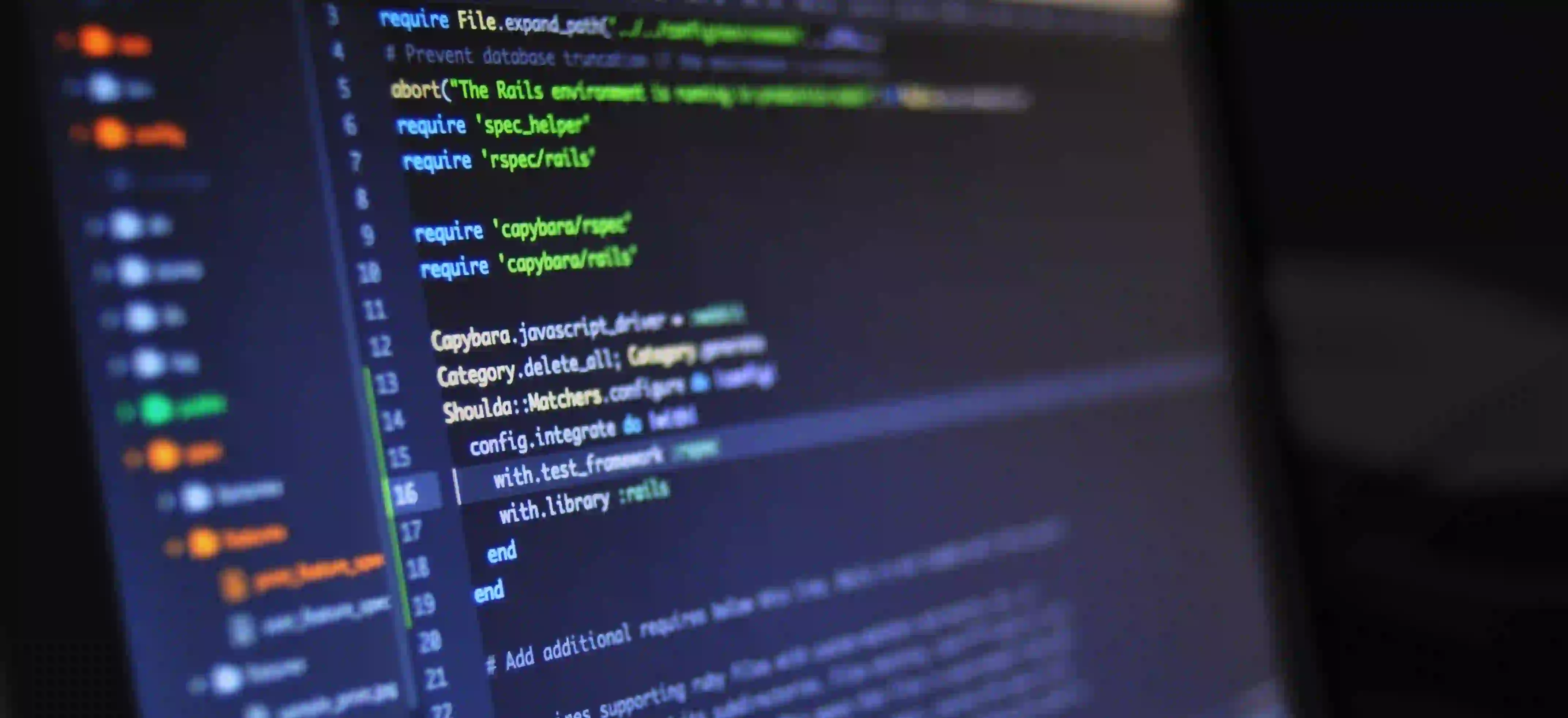
Mastering Java 8: Common Issues When Reading Files
Java 8 introduced several enhancements that significantly improved how we work with files. The introduction of the NIO.2 package brought the new java.nio.file
package, which provides a more comprehensive and flexible way to manage file I/O operations. However, even with these improvements, developers still encounter various common issues when handling files. In this blog post, we will explore these issues, supported by code snippets and practical examples, to ensure that you’re well-equipped for file reading endeavors in Java 8.
Table of Contents
- Overview of NIO.2
- Common File Reading Issues
- Issue 1: File Not Found
- Issue 2: Encoding Problems
- Issue 3: Handling Large Files Efficiently
- Issue 4: Resource Leaks
- Issue 5: Reading Non-Text Files
- Conclusion
- Additional Resources
1. Overview of NIO.2
The introduction of the NIO.2 APIs in Java 8 allowed developers to efficiently handle file operations without the verbosity and complexity of the earlier java.io
package. One key enhancement is the ability to use the Files
class, which provides numerous static methods for reading, writing, and manipulating files.
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class FileReaderExample {
public static void main(String[] args) {
Path path = Paths.get("example.txt");
try {
String content = Files.readString(path);
System.out.println(content);
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this example, the Files.readString()
method is a clean and simple way to read the entire content of a file. This API abstracts many low-level operations, which can drastically improve your productivity. However, as with any powerful tool, common issues can arise.
2. Common File Reading Issues
Issue 1: File Not Found
One of the most common issues developers encounter is the "file not found" error. This usually occurs due to an incorrect file path or permissions.
Solution: Verify the File Path
Always check your file path. Paths can be absolute or relative. Here’s an example of how to handle this:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class FileNotFoundExample {
public static void main(String[] args) {
Path path = Paths.get("nonexistent.txt");
if (Files.notExists(path)) {
System.out.println("File does not exist: " + path.toAbsolutePath());
return;
}
try {
String content = Files.readString(path);
System.out.println(content);
} catch (IOException e) {
e.printStackTrace();
}
}
}
Here, we check if the file exists before attempting to read it. This preventive measure helps avoid runtime exceptions.
Issue 2: Encoding Problems
Encoding issues often surface when reading text files with unexpected character sets. Java, by default, uses UTF-8 encoding, but if your file is in a different encoding (like ISO-8859-1), it may lead to garbled output.
Solution: Specify Encoding
You can specify the desired encoding using an InputStreamReader
. Here’s how:
import java.nio.charset.StandardCharsets;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.Reader;
public class FileEncodingExample {
public static void main(String[] args) {
Path path = Paths.get("example.txt");
try (Reader reader = new InputStreamReader(Files.newInputStream(path), StandardCharsets.ISO_8859_1)) {
char[] buffer = new char[1024];
int bytesRead;
while ((bytesRead = reader.read(buffer)) != -1) {
System.out.print(new String(buffer, 0, bytesRead));
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
By specifying StandardCharsets.ISO_8859_1
, we ensure accurate reading of the file content. Always be aware of your file’s encoding to avoid misinterpretation.
Issue 3: Handling Large Files Efficiently
Reading large files entirely into memory can lead to performance bottlenecks. Instead, it's best practice to read files in smaller chunks.
Solution: Use BufferedReader
Using BufferedReader
allows you to read large files line by line, which is more efficient. See the code snippet below:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.BufferedReader;
import java.io.IOException;
public class LargeFileReaderExample {
public static void main(String[] args) {
Path path = Paths.get("largefile.txt");
try (BufferedReader br = Files.newBufferedReader(path)) {
String line;
while ((line = br.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
This approach minimizes memory consumption and handles even huge files gracefully. For further optimization, consider using parallel processing techniques for large files.
Issue 4: Resource Leaks
Neglecting to close file resources can lead to resource leaks, which, over time, can degrade the performance of your application.
Solution: Try-With-Resources
Java 7+ offers the try-with-resources statement which ensures that each resource is closed after use:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class ResourceLeakExample {
public static void main(String[] args) {
Path path = Paths.get("example.txt");
try {
String content = Files.readString(path);
System.out.println(content);
} catch (IOException e) {
e.printStackTrace();
}
}
}
In this example, the Files.readString
is being used within a try block, and resources will be closed automatically. This mitigates the risk of resource leaks.
Issue 5: Reading Non-Text Files
Sometimes, you may encounter binary files or files with non-text content. Attempting to read them as text will lead to unexpected results.
Solution: Use Appropriate Methods
For binary files (like images or PDFs), use:
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.io.IOException;
public class BinaryFileReaderExample {
public static void main(String[] args) {
Path path = Paths.get("image.png");
try {
byte[] fileBytes = Files.readAllBytes(path);
System.out.println("Number of bytes read: " + fileBytes.length);
} catch (IOException e) {
e.printStackTrace();
}
}
}
This example reads the binary data into a byte array. Understanding the type of file you're reading helps you select the correct approach.
3. Conclusion
Through the exploration of common issues associated with reading files in Java 8, we’ve uncovered solutions that will streamline your file handling. Embracing the power of the NIO.2 API while being cautious of potential pitfalls will enhance your Java programming experience.
For further reading and resources, check out the official Java documentation on NIO.2 and a dedicated overview for file handling utilities.
4. Additional Resources
- Java SE 8 Documentation
- Java File I/O (NIO.2) Tutorial
With the right knowledge and tools, mastering file operations in Java will become a seamless part of your development workflow!