How to Effectively Suppress FindBugs Warnings in Spring Boot
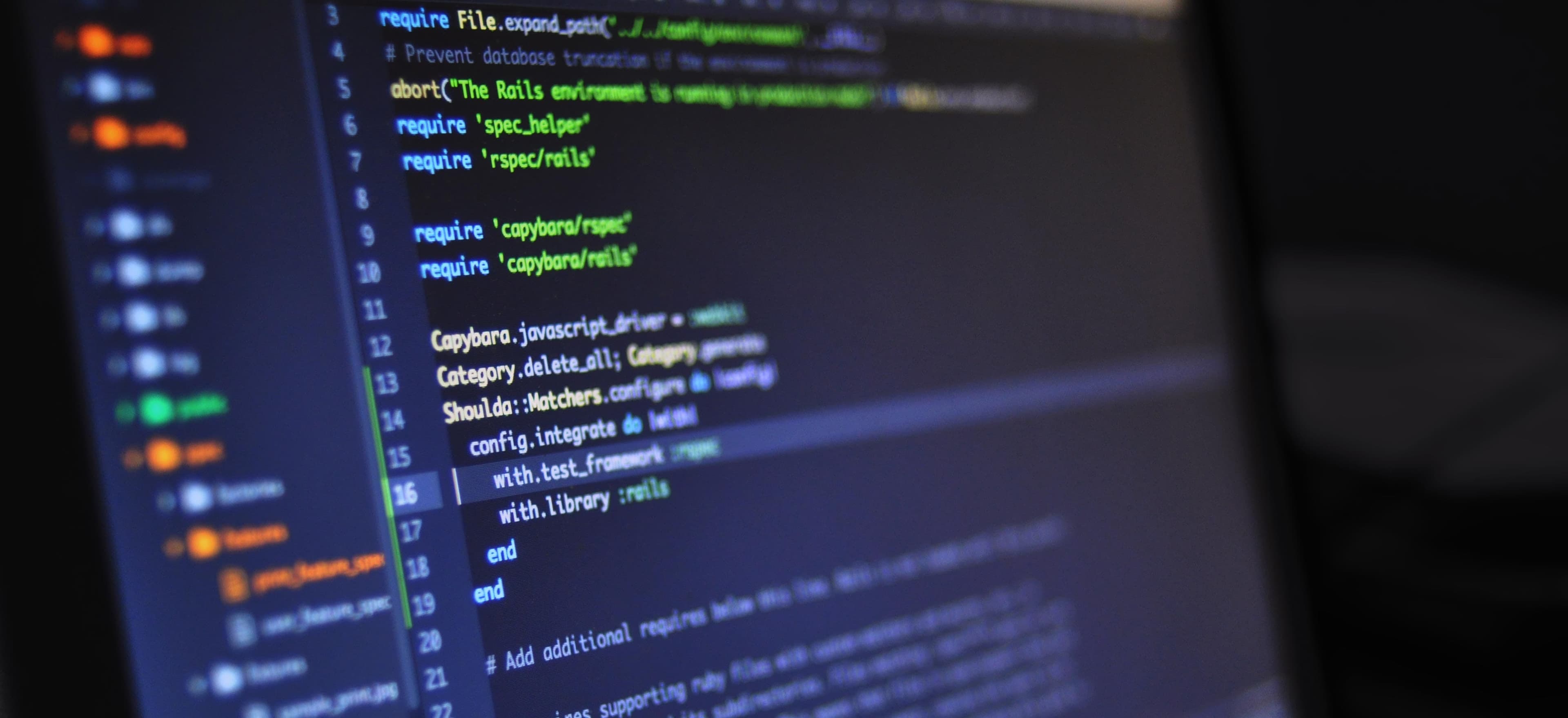
- Published on
How to Effectively Suppress FindBugs Warnings in Spring Boot
Finding and suppressing warnings in your Spring Boot application is crucial for maintaining clean code and optimal performance. One popular tool for static analysis in Java projects is FindBugs, which identifies potential bugs in your codebase. However, as projects evolve, some warnings may become irrelevant or overly sensitive. This blog post will guide you through effectively managing and suppressing FindBugs warnings in your Spring Boot application while adhering to best practices.
Why Use FindBugs?
Before we dive deeper into the suppression methods, let’s briefly discuss why you should consider using FindBugs:
- Identify Hidden Issues: It can uncover common programming bugs that remain hidden during runtime.
- Improved Code Quality: By flagging potential problems, it encourages developers to write clean, maintainable code.
- Time-Saving: Fixing issues found early in the development cycle saves valuable time and resources.
For more information about FindBugs, check out the FindBugs overview.
Understanding FindBugs Warnings
FindBugs provides a comprehensive set of warnings, categorized into various types such as:
- Performance Warnings: Issues that can lead to slow performance.
- Security Vulnerabilities: Potential threats in your code.
- Bad Practice: Situations where standard Java practices are not followed.
Common Types of Warnings
Some common warnings you might encounter include:
- NP_NULL_ON_SOME_PATH_FROM_RETURN_VALUE: Indicates a possible null value being returned.
- BAD_PRACTICE: Highlights potential code smells.
- DM_DEFAULT_ENCODING: Signals the use of default character encoding.
Understanding the nature of these warnings is essential for deciding whether to address or suppress them.
How to Suppress Warnings
1. Suppress with Annotations
FindBugs allows you to suppress warnings directly in your Java code using annotations. This method is often the most straightforward approach. Below is an example:
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
public class MyService {
@SuppressFBWarnings(value = "NP_NULL_ON_SOME_PATH_FROM_RETURN_VALUE", justification = "Input is validated beforehand")
public String getUserNameById(Long id) {
// Logic to fetch the user
if (id == null) return "DefaultUser";
User user = userRepository.findById(id);
return user != null ? user.getName() : null;
}
}
Why Use Annotations?
- Local Suppression: This keeps the suppression contextual, only affecting the relevant code.
- Justifications: Adding justifications is excellent for code reviews, explaining why the warning is suppressed.
2. Suppress Findings in Configuration Files
If there are warnings that impact multiple files or the entire project, you may want to suppress them in your FindBugs configuration file. Here’s how to do it:
- Create or modify the
findbugs-annotations.xml
configuration file in your project. - Add the following entries for suppressing specific warnings:
<FindBugsFilter>
<Match>
<Class name="com.example.MyService" />
<Bug code="NP_NULL_ON_SOME_PATH_FROM_RETURN_VALUE" />
</Match>
</FindBugsFilter>
Benefits of Configuration Suppression:
- Project-Wide Control: Useful for larger projects where testing is required across many files.
- Consistent Application: Helps maintain uniformity across your application’s codebase.
3. Using Comments for Selective Suppression
If you want to handle temporary issues or want to suppress warnings more flexibly, consider using comments within your code, though this is less formal than annotations. You can achieve this with the following comment style:
public class MyController {
// Suppressing FindBugs warning for this method temporarily
@SuppressFBWarnings("DM_DEFAULT_ENCODING")
public void riskyMethod() {
String path = System.getProperty("user.home");
// Further processing
}
}
When to Use Comments:
- Temporary Workarounds: If you are planning to fix the issue in the near future.
- Clarity for Developers: Useful for adding context for developers who will read the code later.
4. Reviewing and Updating Suppressed Warnings
As a best practice, periodically review your suppression annotations. Over time, codebases change, and certain previously suppressed warnings may no longer apply. Regularly revisiting these can help maintain code quality.
5. Combining FindBugs with Other Tools
While FindBugs is a great tool, consider integrating it with other static analysis tools like PMD or SonarQube. Each tool can catch different sets of issues. For more details on integrating static analysis into your build process, refer to the SonarQube documentation.
To Wrap Things Up
Effectively suppressing FindBugs warnings in a Spring Boot application can significantly enhance your development workflow. By using a mix of annotations, configuration file changes, and comments, you can maintain a clean codebase while addressing only the most pertinent warnings.
Always remember to provide justification for suppressions, review them regularly, and combine the use of FindBugs with other tools for the best results. Following these practices ensures that your application remains robust and maintainable.
If you wish to dig deeper into static code analysis and its significance, consider exploring SonarQube’s guide. Happy coding!