Common Pitfalls When Implementing Thread Pools in Java
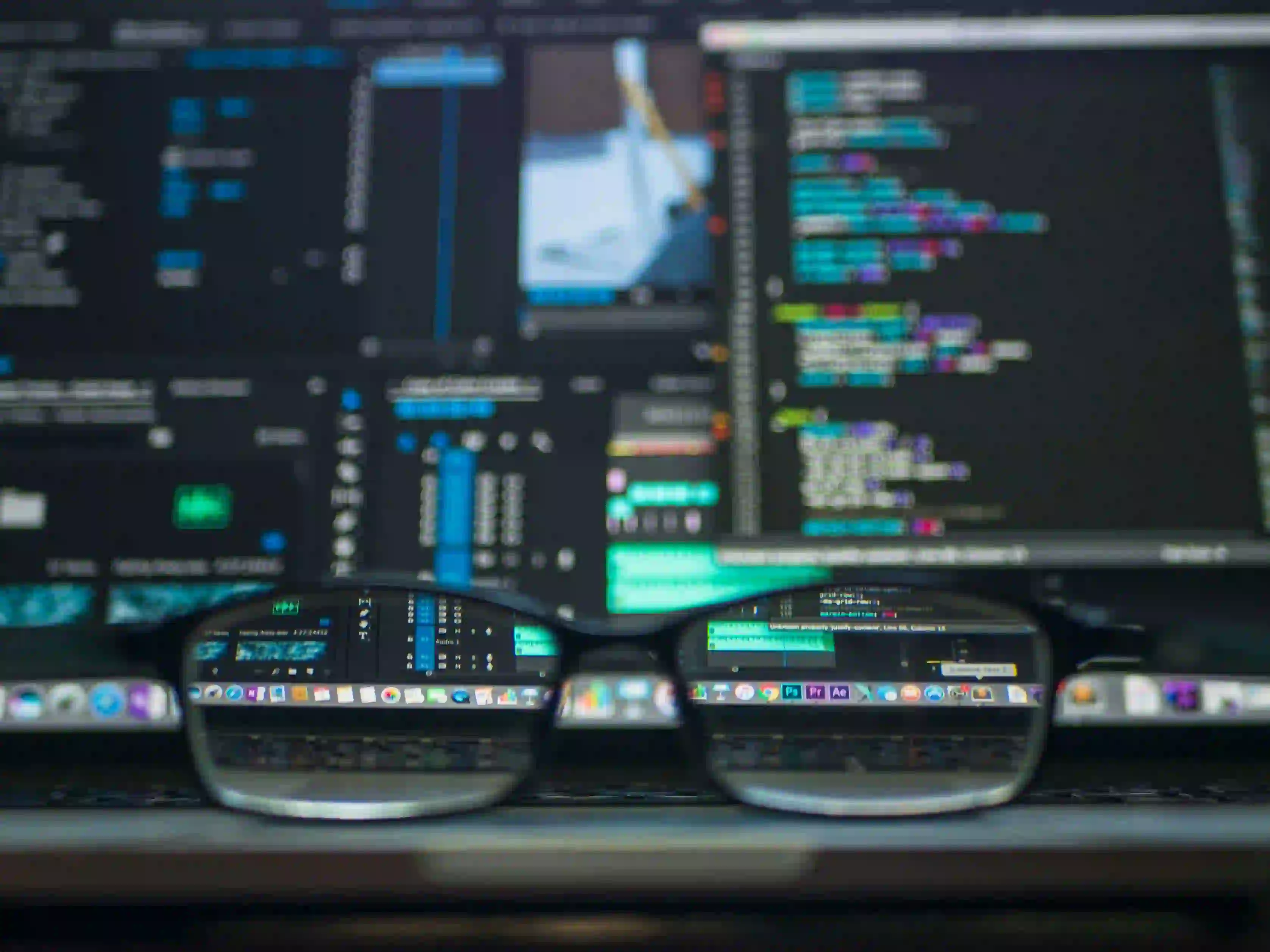
Common Pitfalls When Implementing Thread Pools in Java
In modern multithreaded programming, properly managing threads can significantly improve application performance, scalability, and responsiveness. Java provides robust tools for handling multithreaded operations, especially through the use of Thread Pools. However, despite their advantages, there are common pitfalls that developers can fall into when implementing thread pools in Java.
In this blog post, we will explore these pitfalls, backed by code examples and thorough discussions on why they matter. Whether you are a seasoned Java developer or new to concurrent programming, understanding these pitfalls will enhance your skill set.
Understanding Thread Pools
Thread pools are a design pattern used to manage a pool of worker threads efficiently. Instead of creating a new thread for every task, a thread pool reuses a number of existing threads to perform ongoing work. This not only reduces the overhead of thread creation but also helps manage resources effectively.
Before diving into the pitfalls, let’s take a quick look at how to create a simple thread pool in Java.
Example: Creating a Simple Thread Pool
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
public class ThreadPoolExample {
public static void main(String[] args) {
// Create a thread pool with a fixed number of threads
ExecutorService executorService = Executors.newFixedThreadPool(5);
for (int i = 0; i < 10; i++) {
final int taskId = i;
executorService.submit(() -> {
System.out.println("Task " + taskId + " is running by " + Thread.currentThread().getName());
try {
Thread.sleep(1000); // Simulate doing work
} catch (InterruptedException e) {
Thread.currentThread().interrupt(); // Restore interrupt status
}
});
}
executorService.shutdown(); // Shutdown thread pool
System.out.println("All tasks submitted.");
}
}
In this example, we create a thread pool with a fixed size of 5 threads and submit 10 tasks to it. The tasks are processed by the threads in the pool, showcasing the basic execution scheme of thread pools.
Common Pitfalls
1. Not Shutting Down the Executor
One of the most common mistakes is neglecting to shut down the ExecutorService properly. If you fail to shut it down, it can lead to resource leaks, with the application potentially holding onto threads indefinitely.
Why it Matters: Not shutting down the thread pool can lead to performance degradation and eventually out-of-memory errors. Your application might continue to consume CPU and memory resources unnecessarily.
Solution:
Always call shutdown()
or shutdownNow()
after you have finished submitting tasks.
executorService.shutdown(); // Initiates an orderly shutdown
2. Blocking the Main Thread
When using thread pools, it’s crucial to remember that blocking the main thread while waiting for tasks can lead to bottlenecks. A common scenario is using the awaitTermination
method without caution.
Why it Matters: Blocking the main thread can negate the benefits of concurrency. Your application can become unresponsive, especially if the main thread is required for user interaction or processing.
Example:
try {
executorService.awaitTermination(5, TimeUnit.SECONDS); // May block indefinitely if tasks take long
} catch (InterruptedException e) {
e.printStackTrace();
}
Solution: Use the Future
class to check the status of your tasks or ensure that the main thread is not blocked.
3. Improper Handling of RejectedExecutionException
When submitting tasks to a thread pool, there’s a chance of encountering a RejectedExecutionException
. This occurs if the thread pool is already at maximum capacity and cannot accept more tasks.
Why it Matters: Ignoring this exception can lead to tasks being silently dropped, resulting in incomplete processing and unpredictable behavior.
Solution:
Always manage exceptions and consider using a custom RejectedExecutionHandler
.
executorService = Executors.newFixedThreadPool(5, new ThreadPoolExecutor.AbortPolicy());
// Handle rejected tasks here
4. Mismanaging Thread Pool Size
Choosing the right thread pool size is critical. Too few threads can lead to underutilization of resources, while too many may cause overhead from context switching.
Why it Matters: The ideal thread pool size depends on various factors, including CPU cores, I/O operations, and the specific workload of your application. Mismanagement can lead to performance issues.
Solution:
Use available utilities to determine optimal thread pool sizes. For example, a general rule of thumb for CPU-bound tasks is to set the thread pool size to the number of available cores, while for I/O-bound tasks, you may want a larger pool.
int cores = Runtime.getRuntime().availableProcessors();
ExecutorService executorService = Executors.newFixedThreadPool(cores);
5. Not Using Callable for Results
When using the Runnable
interface, you cannot return values from tasks. Opting for Callable
allows you to return results and handles exceptions more seamlessly.
Why it Matters: Ignoring the need for results can lead to incomplete workflows and inefficient debugging.
Solution:
Use Callable
when you need to get results or handle exceptions effectively.
Callable<Integer> task = () -> {
// Task logic
return result;
};
Future<Integer> futureResult = executorService.submit(task);
6. Ignoring Context and Synchronization
When threads share resources, ensure that access to those resources is synchronized. Failing to do so can lead to race conditions and corrupted data.
Why it Matters: Race conditions can cause unpredictable behavior, which can be particularly problematic in multi-threaded environments.
Solution:
Make use of synchronized methods, blocks, or appropriate concurrency utilities provided in the java.util.concurrent
package.
synchronized (sharedResource) {
// Perform operations on sharedResource
}
Bringing It All Together
Implementing thread pools in Java can lead to better application performance and improved resource management. However, it is crucial to avoid common pitfalls that can lead to serious issues. By paying attention to proper shutdown procedures, avoiding blocking calls, managing thread pool sizes carefully, and using Callable
, you can create a robust multithreaded application.
For further reading on Java concurrency, consider exploring the following resources:
- Official Java Concurrency Tutorial
- Java Concurrency in Practice
By understanding these pitfalls and best practices, you can become a more competent Java developer in multithreaded programming. Happy coding!