Struggling with Refactoring? A Guide to NodeTable Extraction
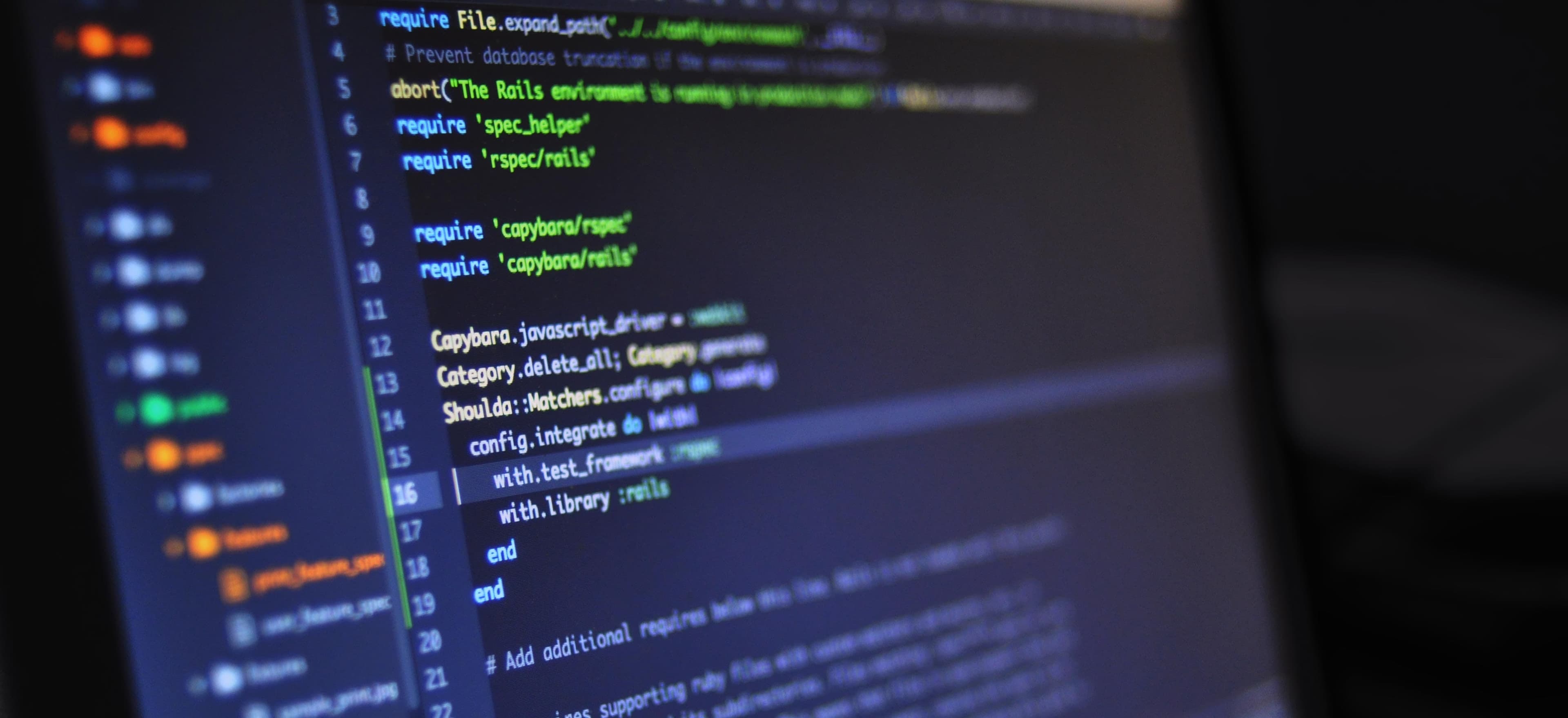
- Published on
Struggling with Refactoring? A Guide to NodeTable Extraction
Refactoring is an essential aspect of maintaining and improving codebases. It helps developers simplify code, increase readability, and reduce the risks of bugs. In this blog post, we will explore a specific technique known as NodeTable Extraction, designed to enhance the organization of complex code.
NodeTable Extraction is especially beneficial when you're dealing with nodes in a data structure like a tree or graph. Instead of having a convoluted, hard-to-manage system, this technique allows you to extract nodes into a structured format. This post will guide you through the process, illustrating its benefits with relevant examples.
What Is NodeTable Extraction?
NodeTable Extraction involves creating a separate table (or data structure) that stores the nodes extracted from a complex entity. This approach not only improves maintainability but also enhances performance, making it easier to traverse nodes and access specific values.
Benefits of NodeTable Extraction
- Improved Readability: By separating nodes into a distinct table, you can avoid clutter in your code.
- Easier Debugging: Issues are easier to identify when nodes are organized systematically.
- Enhanced Performance: Accessing nodes is faster when dealing with a dedicated structure.
- Reusability: Extracted nodes can be easily reused across different parts of your application.
When to Use NodeTable Extraction
NodeTable Extraction should be considered when:
- You have a deeply nested structure that is difficult to navigate.
- There are repeated references to the same nodes throughout your code.
- Performance issues are noticeable when accessing node values.
If you're facing any of these issues, it's time to consider refactoring your code using NodeTable Extraction.
How to Implement NodeTable Extraction
Let's walk through a simple example in Java. We will refactor a basic tree structure and extract nodes into a NodeTable.
Original Code Example
Start with a simple representation of a tree:
class TreeNode {
int value;
TreeNode left;
TreeNode right;
TreeNode(int value) {
this.value = value;
this.left = null;
this.right = null;
}
}
In this implementation, nodes are tightly coupled within the structure. Accessing nodes and their values requires a complicated traversal mechanism.
Step 1: Define a NodeTable Class
We'll create a NodeTable
class that will store our nodes in a more accessible manner.
import java.util.HashMap;
class NodeTable {
private HashMap<Integer, TreeNode> nodeMap;
public NodeTable() {
this.nodeMap = new HashMap<>();
}
public void addNode(TreeNode node) {
nodeMap.put(node.value, node);
}
public TreeNode getNode(int value) {
return nodeMap.get(value);
}
public boolean containsNode(int value) {
return nodeMap.containsKey(value);
}
// Additional methods for additional functionality can be added here.
}
Why This Works
By using a HashMap
to store nodes, we're optimizing the look-up time to average O(1). This sharpens performance substantially, especially in larger trees.
Step 2: Modifying TreeNode
Now, modify the TreeNode
to include association with the NodeTable
.
class TreeNode {
int value;
TreeNode left;
TreeNode right;
NodeTable nodeTable;
TreeNode(int value, NodeTable nodeTable) {
this.value = value;
this.left = null;
this.right = null;
this.nodeTable = nodeTable;
this.nodeTable.addNode(this);
}
}
Why This Change Matters
With NodeTable
embedded in every node, we ensure that every created node is automatically registered in the node table. This not only streamlines node management but also centralizes node data access.
Step 3: Refactoring Tree Operations
Lastly, you will need to refactor tree operations to utilize the NodeTable
. For example, the following method demonstrates searching for a value:
public static TreeNode searchNode(NodeTable nodeTable, int value) {
return nodeTable.getNode(value);
}
Why Refactor?
This function makes it much simpler to find nodes without traversing the entire tree, showcasing the power of NodeTable Extraction effectively.
Example of Usage
After the refactoring, user navigation will look cleaner:
public class TreeExample {
public static void main(String[] args) {
NodeTable nodeTable = new NodeTable();
TreeNode root = new TreeNode(10, nodeTable);
root.left = new TreeNode(5, nodeTable);
root.right = new TreeNode(20, nodeTable);
TreeNode searchedNode = searchNode(nodeTable, 5);
if (searchedNode != null) {
System.out.println("Node found: " + searchedNode.value);
} else {
System.out.println("Node not found.");
}
}
}
What's New?
This example demonstrates:
- Simplified node creation.
- Efficient node searching.
- Clarity and maintainability in your tree structure.
When to Avoid NodeTable Extraction
While NodeTable Extraction typically brings numerous advantages, it's crucial to evaluate its use in the following scenarios:
- Small Structures: If a data structure is minimal and rarely accessed, the overhead of extracting to a NodeTable may not be justified.
- Performance Bottlenecks Elsewhere: If performance is an issue due to factors other than node access, focusing on NodeTable extraction may detract from addressing the actual performance problem.
To sum up, always assess the practical implications to find a balance between simplicity and functionality.
The Bottom Line
NodeTable Extraction serves as an effective refactoring technique that can greatly enhance the management of complex data structures. Through systematic extraction and organization of nodes, you can improve code readability, debugging, and performance.
In Java, implementing a NodeTable
helps developers maintain cleaner, more efficient code. As you tackle your next refactoring challenge, keep in mind the benefits of NodeTable Extraction. Not only will it streamline your data management, but it will also lay down a robust foundation for further enhancements.
For further reading on code refactoring best practices, check out Martin Fowler's Refactoring Book and Refactoring Guru.
Happy coding!