Code Review vs. Testing: Understanding the Distinction
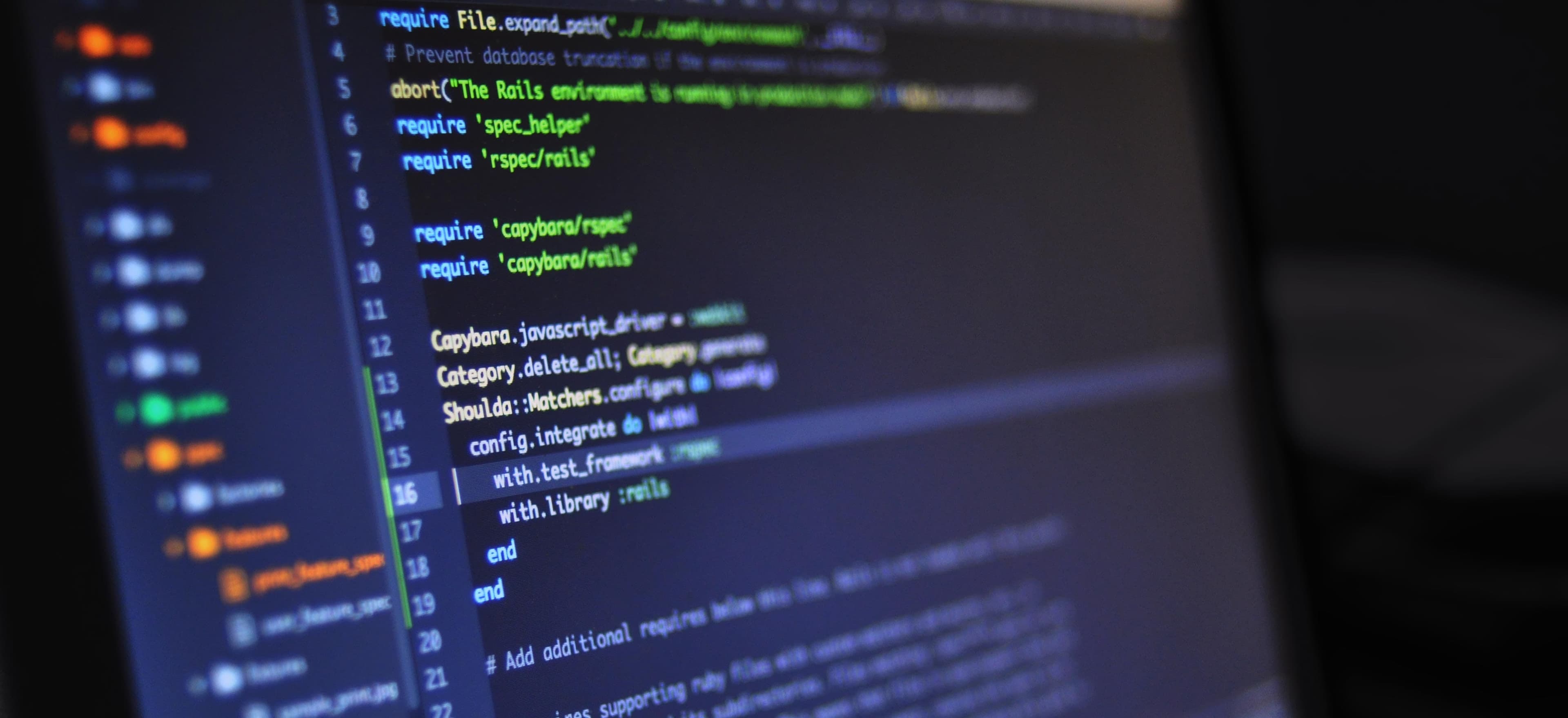
- Published on
Code Review vs. Testing: Understanding the Distinction
In the realm of software development, clarity, quality, and efficiency are paramount. Engineers often hear about code review and testing as key components of the development lifecycle. Both are crucial for creating robust applications, but they serve different purposes and processes. This blog post delves into the distinctions between code review and testing, highlighting their significance, methodologies, and best practices.
What is Code Review?
Code review is the systematic examination of source code with the purpose of identifying mistakes overlooked in the initial development phase. It acts as a second set of eyes for developers, helping catch issues early on.
Why Code Review Matters
- Quality Assurance: Code reviews help in maintaining high standards of code quality. They allow team members to enforce coding standards and best practices which encourage cleaner, more maintainable code.
- Knowledge Sharing: They foster knowledge transfer within the team, ensuring that team members understand different parts of the codebase.
- Spotting Bugs Early: Catching bugs before they enter production can save time and resources.
Peer Review Process
Typically, a peer review involves the following steps:
- Preparation: The developer submits their code for review, including documentation and any relevant tests.
- Review: Others examine the code for errors, readability, and maintainability. They can leave inline comments for discussions.
- Discussion: The original author and reviewers engage in discussions about the code, including suggestions for improvements.
- Approval: Once satisfied, the reviewer approves or requests changes before merging the code into the main branch.
Example of Code Review
Here’s a simplified Java method that calculates the factorial of a number, followed by an illustration of what a code review might look like.
public class MathUtil {
/**
* Calculates the factorial of a number.
* @param n The number to calculate the factorial for.
* @return The factorial of the number.
*/
public static int factorial(int n) {
if (n < 0) {
throw new IllegalArgumentException("Input must be non-negative.");
}
int result = 1;
for (int i = 2; i <= n; i++) {
result *= i;
}
return result;
}
}
What to Look for in the Review
- Naming conventions: Is the method name and parameter name clear?
- Error handling: Does the method appropriately handle negative input?
- Efficiency: Could this be optimized further? (In this case, it is already efficient with a linear time complexity of O(n).)
By addressing such questions, a peer review can lead to significant code improvements.
What is Testing?
Testing, on the other hand, entails executing the code with the aim of finding errors and ensuring that the application behaves according to specified requirements. Testing can take many forms, such as unit testing, integration testing, or end-to-end testing.
Why Testing is Essential
- Validation of Functionality: Testing confirms that the code works as intended.
- Regression Prevention: Automated tests can run frequently to ensure changes do not introduce new bugs.
- Documentation: Well-defined tests serve as living documentation of the expected behavior of your code.
Types of Testing
- Unit Testing: Focuses on testing the smallest parts of an application—functions or methods.
- Integration Testing: Tests how different parts of the application work together.
- System Testing: Tests the complete integrated application to verify that it meets the specified requirements.
Example of Unit Testing in Java
Below is a unit test for the factorial method we previously examined.
import static org.junit.jupiter.api.Assertions.assertEquals;
import static org.junit.jupiter.api.Assertions.assertThrows;
import org.junit.jupiter.api.Test;
public class MathUtilTest {
@Test
public void testFactorial() {
assertEquals(1, MathUtil.factorial(0));
assertEquals(1, MathUtil.factorial(1));
assertEquals(2, MathUtil.factorial(2));
assertEquals(6, MathUtil.factorial(3));
assertEquals(24, MathUtil.factorial(4));
}
@Test
public void testFactorialNegative() {
assertThrows(IllegalArgumentException.class, () -> {
MathUtil.factorial(-1);
});
}
}
Explanation of the Test Code
- Assertions: The
assertEquals
method checks if the output of the factorial method is as expected. - Edge Cases: The test validates behavior for both valid inputs and edge cases, like zero and negative values.
- Exception Testing: The test validates that the method throws an
IllegalArgumentException
when given a negative input.
Code Review vs. Testing: A Quick Comparison
| Aspect | Code Review | Testing | |-----------------------|------------------------------------------|---------------------------------------------| | Purpose | Quality assurance through inspection | Validation of application behavior | | Focus | Code structure, readability, standards | Functional correctness of code | | Timing | Before merging code | After code development | | Scope | Individual methods or sections | Entire applications or features |
Choosing the Right Approach
Both code review and testing should coexist in a balanced software development strategy. While code reviews catch issues related to code structure and logic, testing provides insights into functional behavior. Together, they contribute to a higher standard of software quality.
Best Practices for Code Review and Testing
- Establish Guidelines: Define clear code review and testing guidelines for consistency within the team.
- Automate Where Possible: Tools like Jenkins or Travis CI can streamline the testing process, running tests automatically after code commits.
- Foster a Collaborative Environment: Encourage constructive feedback during reviews, promoting a culture of learning rather than criticism.
For more insights on creating effective testing strategies, check out the official Java testing documentation.
A Final Look
Understanding the distinctions between code review and testing is integral to enhancing the software development process. By investing time in both practices, teams can ensure higher quality, more maintainable code that meets user expectations. As developers, embracing both code review and testing will lead to better results and, ultimately, more successful software projects.
In sum, while code reviews and testing serve different functions in a software development pipeline, they complement each other to create robust applications. Harnessing their combined powers effectively is critical for any successful development team. For further reading on the importance of code reviews, visit Code Review Best Practices.
By implementing a well-structured code review and testing strategy, your team can enhance its software quality, leading to a more efficient, productive development environment.
Checkout our other articles