Common Pitfalls in Enterprise Spring Integration Testing
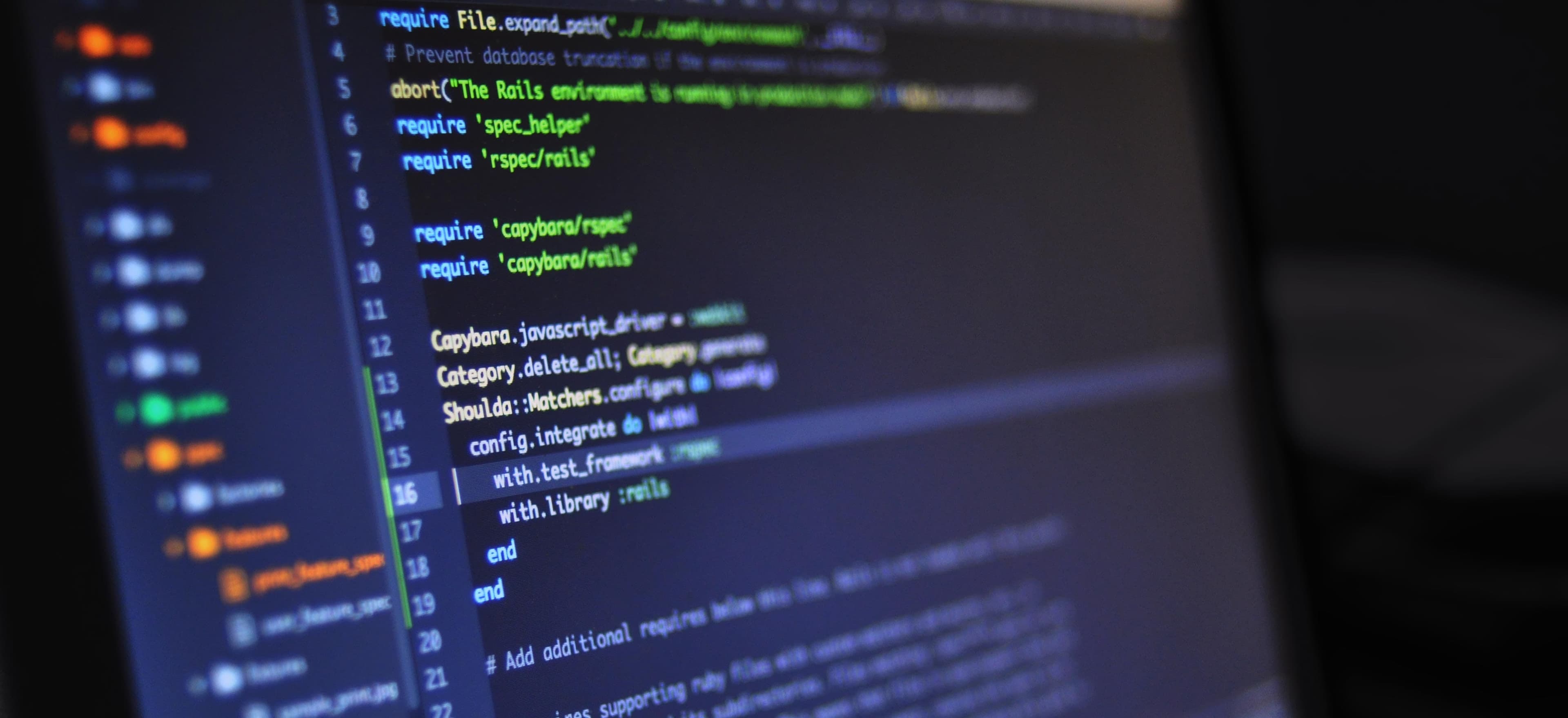
- Published on
Common Pitfalls in Enterprise Spring Integration Testing
Enterprise applications often rely on complex workflows that require efficient testing strategies. When it comes to Spring Integration, this complexity can lead you into various pitfalls if you’re not careful. In this blog post, we’ll explore the common mistakes developers make when integrating Spring testing into their enterprise applications. Alongside this, we’ll provide practical examples to help steer you clear of these traps and ensure your integration testing is robust and reliable.
Understanding Spring Integration Testing
Before we dive into the pitfalls, it's important to understand what Spring Integration testing is and why it's crucial. Spring Integration provides an extension of the Spring programming model to implement messaging patterns in enterprise solutions. Testing these integrations ensures that your components play well together, that data flows correctly, and that the system behaves as expected.
Testing can be categorized into various types:
- Unit Testing - Testing individual components in isolation.
- Integration Testing - Testing interactions between components.
- End-to-End Testing - Testing the entire application flow from start to finish.
Pitfall #1: Lack of Comprehensive Test Coverage
Why It Matters
Many developers underestimate the importance of comprehensive test coverage. When significant parts of the system are left untested, you risk missing critical bugs or discrepancies that could lead to system failures.
Solution
You should aim to achieve at least 80% test coverage across your integration tests. Use tools like JaCoCo or Cobertura to measure your coverage.
Here’s an example of how to set up a basic integration test in Spring:
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyIntegrationTest {
@Autowired
private MyService myService;
@Test
public void testServiceMethod() {
String result = myService.process("test input");
assertEquals("expected output", result);
}
}
In this code snippet, we are ensuring that every branch of process
method is tested. Ensure that you cover both happy paths and edge cases.
Pitfall #2: Ignoring Context Configuration Updates
Why It Matters
Spring applications often evolve, which means that the application context configuration will also change. If your tests are not kept in sync with these changes, you risk encountering issues when your application scales or changes.
Solution
Regularly update your integration test configuration to reflect the current structure and dependencies of your application. Having a dedicated test configuration class can help this:
@Configuration
public class TestConfig {
@Bean
public MyService myService() {
return new MyServiceImpl();
}
}
Keep your test configurations modular and segregated from production configurations. This helps avoid misconfigurations that can lead to misleading test results.
Pitfall #3: Not Isolating the Test Environment
Why It Matters
Running tests on shared environments can lead to flaky tests, where the outcome can vary based on other tasks running in the same environment. This undermines the reliability of your tests.
Solution
Isolate your test environments using Spring profiles. This allows you to set up a dedicated environment for integration tests that won’t interfere with the production environment.
Example of using a test profile:
@ActiveProfiles("test")
@RunWith(SpringRunner.class)
@SpringBootTest
public class MyServiceIntegrationTest {
// Test cases go here
}
Be sure to configure your datasource and other external dependencies in a way that they use test instances. This helps maintain the integrity of your tests.
Pitfall #4: Overcomplicating Test Scenarios
Why It Matters
Developers sometimes create overly complex test scenarios, which can make tests hard to maintain and understand. Tests should be clear, concise, and focused on isolated functionality.
Solution
Break down complex tests into multiple simpler tests. Ensure each test case addresses one scenario effectively. The Arrange-Act-Assert (AAA) pattern can help you structure your tests properly.
@Test
public void whenInputValid_thenOutputValid() {
// Arrange
String input = "valid input";
// Act
String output = myService.process(input);
// Assert
assertEquals("expected output", output);
}
This method helps improve readability and aids other developers in understanding what each test verifies.
Pitfall #5: Not Using Mocks and Stubs Judiciously
Why It Matters
While mocks and stubs are useful in isolating components for unit testing, overusing them can lead to unrealistic tests that do not reflect real-world scenarios. This issue becomes even more pronounced in integration tests.
Solution
Use mocks sparingly; prioritize integration tests that validate actual interaction between components. If a third-party service is integral to your application, consider using a real instance or a realistic simulator instead of a moc.
For instance, when testing an integration flow with a messaging system:
@Test
public void testMessageFlow() {
// Send a message to the input channel
inputChannel.send(new GenericMessage<>("Hello, World!"));
// Check the output channel for the result
GenericMessage<?> receivedMessage = (GenericMessage<?>) outputChannel.receive(10000);
assertNotNull(receivedMessage);
assertEquals("Expected response", receivedMessage.getPayload());
}
This snippet not only tests the integration but also the behavior of services.
Pitfall #6: Not Automating Tests
Why It Matters
Manual testing consumes significant time and introduces human error. Automation is critical for maintaining rapid feedback cycles in development.
Solution
Integrate test automation into your Continuous Integration (CI) pipelines. Frameworks like Jenkins or GitHub Actions can be employed to continually run your Spring Integration tests on every commit.
For example, a simple Jenkins pipeline to run tests might look like this:
pipeline {
agent any
stages {
stage('Build') {
steps {
sh 'mvn clean install'
}
}
stage('Test') {
steps {
sh 'mvn test'
}
}
}
}
Automating your tests ensures that defects can be caught early, ultimately leading to a healthier codebase.
Final Considerations
Testing Spring Integrations effectively is paramount for the success of any enterprise application. By avoiding these common pitfalls, you can build a solid and reliable suite of integration tests. Remember, the goal of testing is not only to find bugs but to give you confidence in your application's stability as you innovate and evolve.
Harness the power of Spring Integration testing effectively, and your enterprise applications will stand strong against the tests of time and user demands.
For more information on Spring Integration testing, consider referring to Spring’s official documentation. Happy coding!
Checkout our other articles