Minimizing Risks in Feature Refactoring: A Step-by-Step Guide
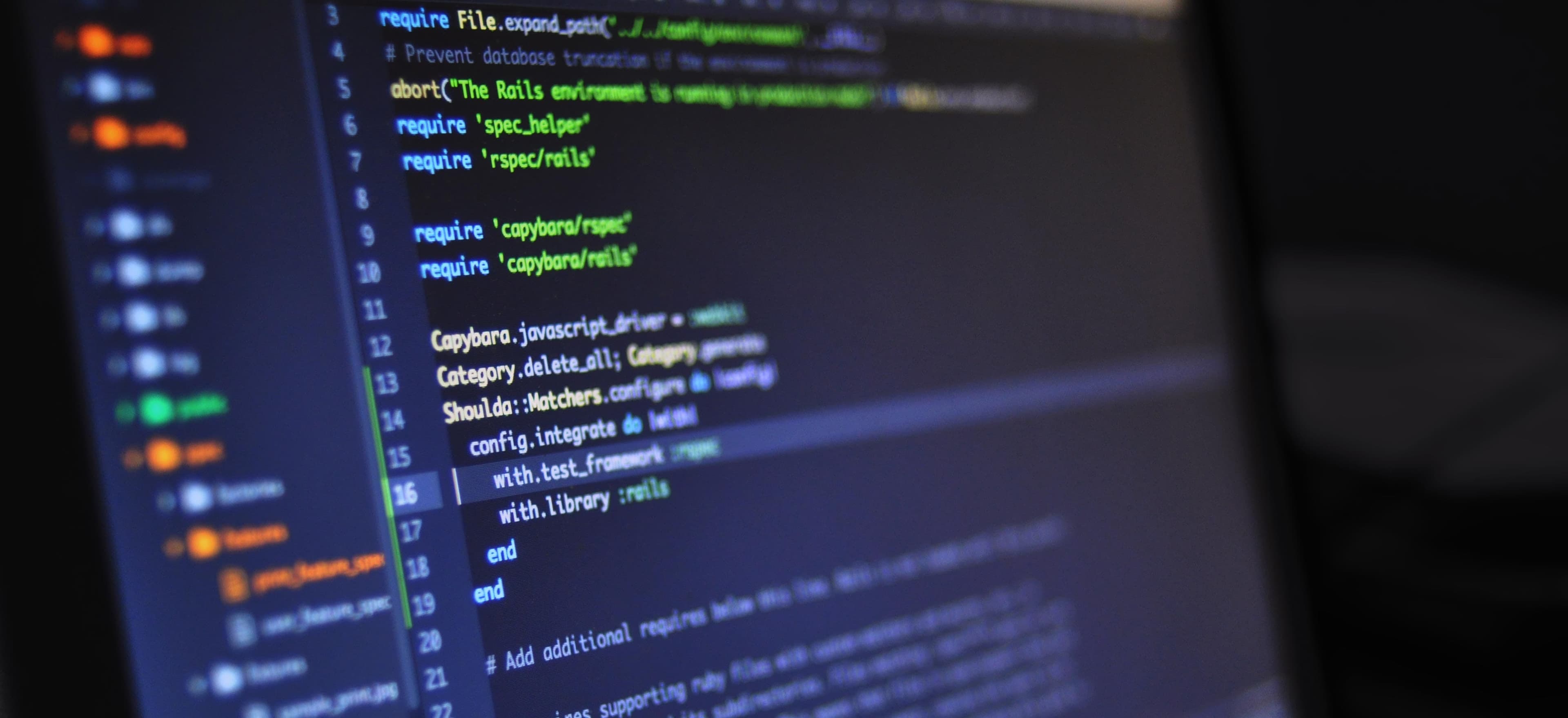
- Published on
Minimizing Risks in Feature Refactoring: A Step-by-Step Guide
Refactoring is an essential part of software development, particularly in Java, where maintaining clean, efficient code is fundamental. While the rewards of refactoring include improved code readability, maintainability, and performance, it also carries risks that can lead to bugs or regressions. This comprehensive guide will outline steps for minimizing risks during the feature refactoring process.
Table of Contents
- Understanding Feature Refactoring
- Identifying the Right Time to Refactor
- Planning Your Refactor
- Creating a Backup
- Implementing Unit Tests
- Applying Incremental Changes
- Using Version Control Effectively
- Conducting Code Reviews
- Monitoring After Refactoring
- Conclusion
Understanding Feature Refactoring
Feature refactoring involves modifying existing code to improve its structure and behavior while preserving its functionality. This critical practice ensures that your application can adapt to new requirements, integrate new features, and maintain performance over time.
Refactoring can touch upon several aspects, including:
- Renaming variables or classes to be more descriptive.
- Breaking down large methods into smaller, reusable components.
- Optimizing algorithms for better performance.
A well-executed refactor can make your Java application more agile and flexible. Learning the art of refactoring is crucial for any Java developer eager to write better code.
Identifying the Right Time to Refactor
Recognizing when to refactor is just as important as the act itself. Here are some signs that your codebase might be due for a refactor:
- Code Smells: Look for indicators such as long methods, duplicated code, or large classes.
- Inflexibility: If adding new features demands extensive changes to existing code, it's time to consider a refactor.
- Accumulating Technical Debt: Regularly postponing clean-ups can lead to a tangled mess. It's essential to address technical debt promptly.
Planning Your Refactor
Before diving in, it's crucial to plan your refactoring. This ensures that your motivation aligns with project objectives:
- Define Goals: Identify what you aim to achieve. Is it code clarity, performance improvement, or something else?
- Map Dependencies: Understand how the code interacts with other components in your application. A stunning refactor can wreak havoc if dependencies are not considered.
Creating a Backup
Creating a backup of the current state of your application is an elementary yet crucial step in reducing risk during refactoring:
Use version control systems such as Git:
git checkout -b feature/refactor-branch
This command creates a new branch, allowing you to work on your refactor independently without affecting the main codebase.
Implementing Unit Tests
Unit tests are your safety net during feature refactoring:
- Write Tests First: Prioritize writing tests for the current functionality you plan to refactor. This way, you have benchmarks against which you can measure your changes.
Here’s a simple example for a class that calculates the area of a rectangle:
public class Rectangle {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double area() {
return length * width;
}
}
Unit Test:
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class RectangleTest {
@Test
public void testArea() {
Rectangle rectangle = new Rectangle(5, 4);
assertEquals(20, rectangle.area());
}
}
The importance of writing tests first lies in ensuring that there can be no regression following your refactor. If any test fails post-refactor, you know there’s a problem.
Applying Incremental Changes
Instead of doing everything at once, build your refactor incrementally. This method not only reduces risk but also allows you to identify breakages in the code more easily.
Here's how you can approach it:
- Change One Thing at a Time: Refactor one functionality or class and rerun tests to ensure everything works.
- Test Frequently: After each incremental change, run your existing unit tests to validate that you haven't broken any functionality.
Example Incremental Refactoring
Let’s say you need to refactor the Rectangle
class to allow for better flexibility:
public class Rectangle {
private double length;
private double width;
public Rectangle(double length, double width) {
this.length = length;
this.width = width;
}
public double area() {
return calculateArea(length, width);
}
private double calculateArea(double length, double width) {
return length * width;
}
}
In this incremental refactor, we've moved the area calculation to a separate method for potential reuse.
Using Version Control Effectively
Version control is critical for minimizing risks during feature refactoring. Here are best practices:
- Frequent Commits: Commit changes with clear messages that document what has been altered. This practice leads to easy roll-backs if necessary.
git commit -m "Refactored area method in Rectangle class"
- Branching Strategy: Use well-defined branching strategies, such as Git Flow, to maintain the stability of your production code while working on new features.
Conducting Code Reviews
Once you complete your refactor, having another developer review your code can help ensure its quality.
Key points for effective code reviews:
- Focus on the Purpose: Ensure that the functionality remains intact despite the changes.
- Commenting: Write comments explaining complex decisions made during refactoring.
Monitoring After Refactoring
Once the refactor is complete and deployed, monitoring your application’s performance and functionality is critical.
-
Use Logging: Add logging around the components that underwent significant changes.
Example:
private double calculateArea(double length, double width) { log.debug("Calculating area for length: " + length + ", width: " + width); return length * width; }
-
Performance Benchmarks: Compare the performance of your application pre and post-refactor to ensure that the changes had the desired effect.
The Closing Argument
Refactoring is a powerful practice that, when done mindfully, can significantly improve code quality. It is, however, crucial to mitigate risks through a well-structured process that involves planning, testing, incremental changes, and peer reviews.
By following the steps outlined in this guide, Java developers can refactor features for better design and performance without introducing unnecessary risks. Maintain a proactive attitude towards refactoring as a key part of software maintenance and development.
For more insights into Java practices, check out Java Documentation and Refactoring Guru.
Happy coding!