Common Pitfalls When Migrating to Spring 4 MVC
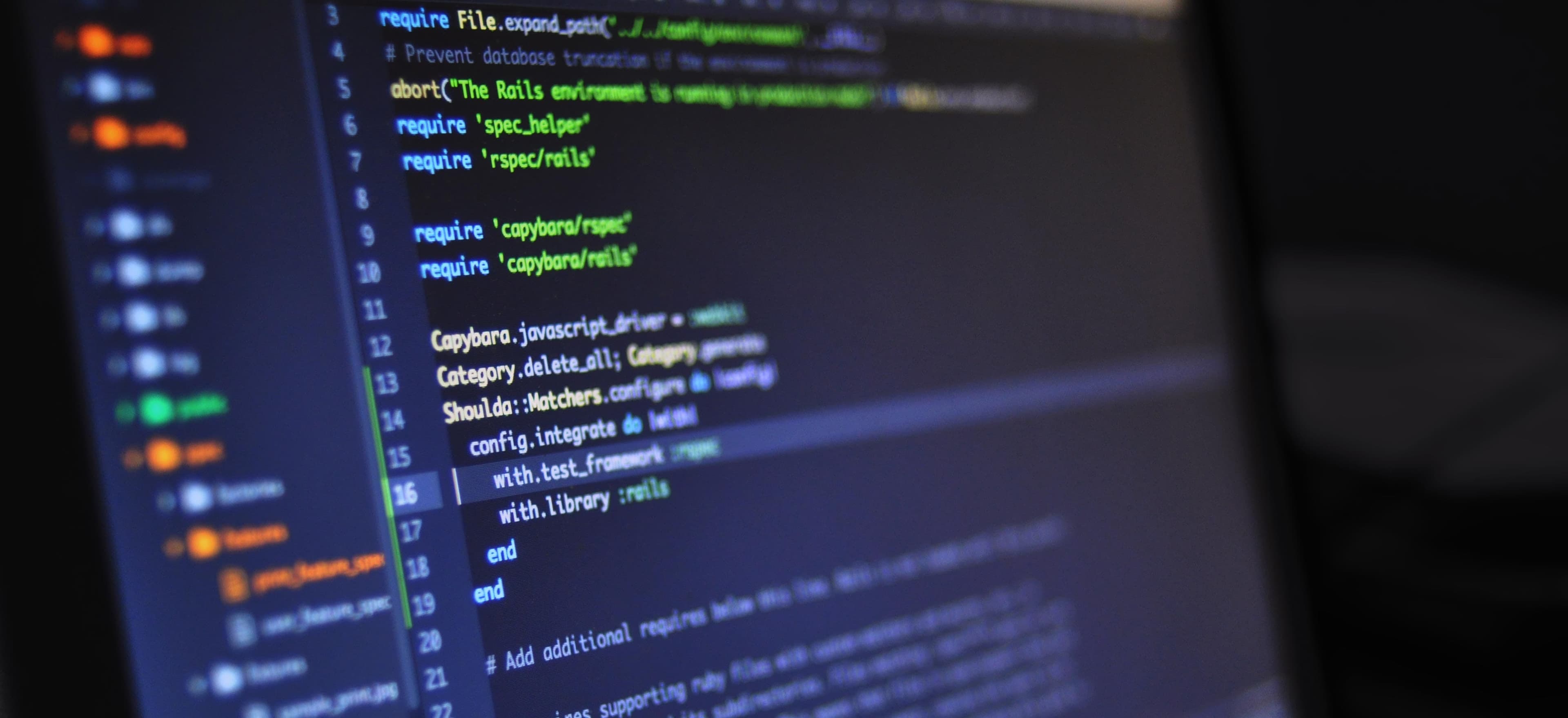
- Published on
Common Pitfalls When Migrating to Spring 4 MVC
Migrating to Spring 4 MVC is an exciting opportunity to take advantage of the latest features and improvements in the framework. However, the migration process may come with a few challenges that could impact the performance and stability of your application. In this blog post, we'll examine common pitfalls that developers face when migrating to Spring 4 MVC and offer insightful solutions to avoid them.
Understanding Spring 4 MVC
Spring 4 MVC is a powerful framework for building web applications in Java, providing a clean separation between the application layer and the user interface. It introduces new features like REST support, enhanced dependency injection, and improvements in the way exceptions are handled. To harness its full potential, a clear understanding of the core components of Spring MVC, such as Controllers, Models, and Views, is essential.
For a comprehensive understanding of Spring MVC, consider reviewing the official Spring documentation.
Pitfall 1: Incompatibility with Pre-existing Code
One of the primary challenges in migrating to Spring 4 MVC is incompatible code. Legacy applications may rely on earlier versions of the Spring framework, which can lead to conflicts. The newer features may make certain deprecated methods incompatible, which can cause runtime errors and unexpected behavior.
Solution:
Before starting the migration, conduct a thorough code review. Identify deprecated classes and methods in your application and replace them with their modern alternatives. For example, if your application uses @Controller
annotation from an earlier version without using @RequestMapping
, you should update the code to ensure proper handling of requests.
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestMethod;
@Controller
public class MyController {
@RequestMapping(value = "/example", method = RequestMethod.GET)
public String example() {
return "exampleView";
}
}
Pitfall 2: Misconfiguration of Dependencies
Spring 4 introduced several new dependencies that you might not have included in your project. This can lead to problems during runtime, especially if vital components are missing.
Solution:
Ensure that your pom.xml
(for Maven) or build.gradle
(for Gradle) includes all necessary Spring 4 dependencies. Look out for core libraries like spring-web
, spring-webmvc
, and others that may not have been included in older projects.
Here is a sample Maven dependency for Spring 4 MVC:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>4.3.13.RELEASE</version>
</dependency>
Pitfall 3: Ignoring New Features
Spring 4 MVC comes with many enhancements and new features that can improve your application's performance and maintainability. Ignoring these features may hinder your application from benefiting from the latest best practices in development.
Solution:
Take the time to learn about new features such as the @ResponseStatus annotation, @RestController, and Message Converters. Incorporating these can significantly streamline your code.
import org.springframework.http.HttpStatus;
import org.springframework.web.bind.annotation.*;
@RestController
@RequestMapping("/api")
public class ApiController {
@GetMapping("/data")
@ResponseStatus(HttpStatus.OK)
public String getData() {
return "Hello, this is your data!";
}
}
Pitfall 4: Exception Handling Adjustments
Spring 4 MVC provides more robust exception handling capabilities. However, improper implementation of these can lead to unhandled exceptions and a degraded user experience.
Solution:
Utilize the new @ControllerAdvice
and @ExceptionHandler
annotations to create a centralized exception handling mechanism.
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseStatus;
import org.springframework.web.bind.annotation.ResponseBody;
@ControllerAdvice
public class GlobalExceptionHandler {
@ExceptionHandler(Exception.class)
@ResponseStatus(HttpStatus.INTERNAL_SERVER_ERROR)
@ResponseBody
public String handleException(Exception e) {
return "Error: " + e.getMessage();
}
}
Pitfall 5: Configuration Changes
Spring 4 made significant changes in how configurations are managed. Developers unfamiliar with the new XML schema or Java-based configuration might struggle with the advanced features provided.
Solution:
Consider migrating XML configurations to Java-based configurations using the @Configuration
annotation. This approach benefits from type safety and allows for easier refactoring.
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.web.servlet.config.annotation.EnableWebMvc;
@Configuration
@EnableWebMvc
public class WebConfig {
@Bean
public MyController myController() {
return new MyController();
}
}
Pitfall 6: Testing Compatibility
Testing frameworks used in legacy applications may not be fully compatible with the changes introduced in Spring 4. Transitioning to JUnit 5 or incorporating new testing strategies is essential for maintaining a robust test suite.
Solution:
Update your testing framework to JUnit 5, if you haven't already. It provides important updates that work fluidly with Spring 4's new features.
import static org.springframework.test.web.servlet.request.MockMvcRequestBuilders.get;
import static org.springframework.test.web.servlet.result.MockMvcResultMatchers.status;
@RunWith(SpringRunner.class)
@WebMvcTest(MyController.class)
public class MyControllerTest {
@Autowired
private MockMvc mockMvc;
@Test
public void exampleTest() throws Exception {
mockMvc.perform(get("/example"))
.andExpect(status().isOk());
}
}
Final Considerations
Migrating to Spring 4 MVC is an opportunity to modernize your application and adopt key best practices in web development. By being mindful of common pitfalls—such as incompatibility with existing code, misconfiguration of dependencies, and ineffective exception handling—you'll not only smooth the migration process but also enhance your application's performance.
Take the time to explore new features and update your configurations and tests accordingly. The benefits of a smoother, more maintainable application will be well worth the effort. For further reading on migrating to Spring 4 MVC, consider visiting the Spring Framework Migration Guide.
Happy coding!
Checkout our other articles