Overcoming Java Learning Barriers for Beginners
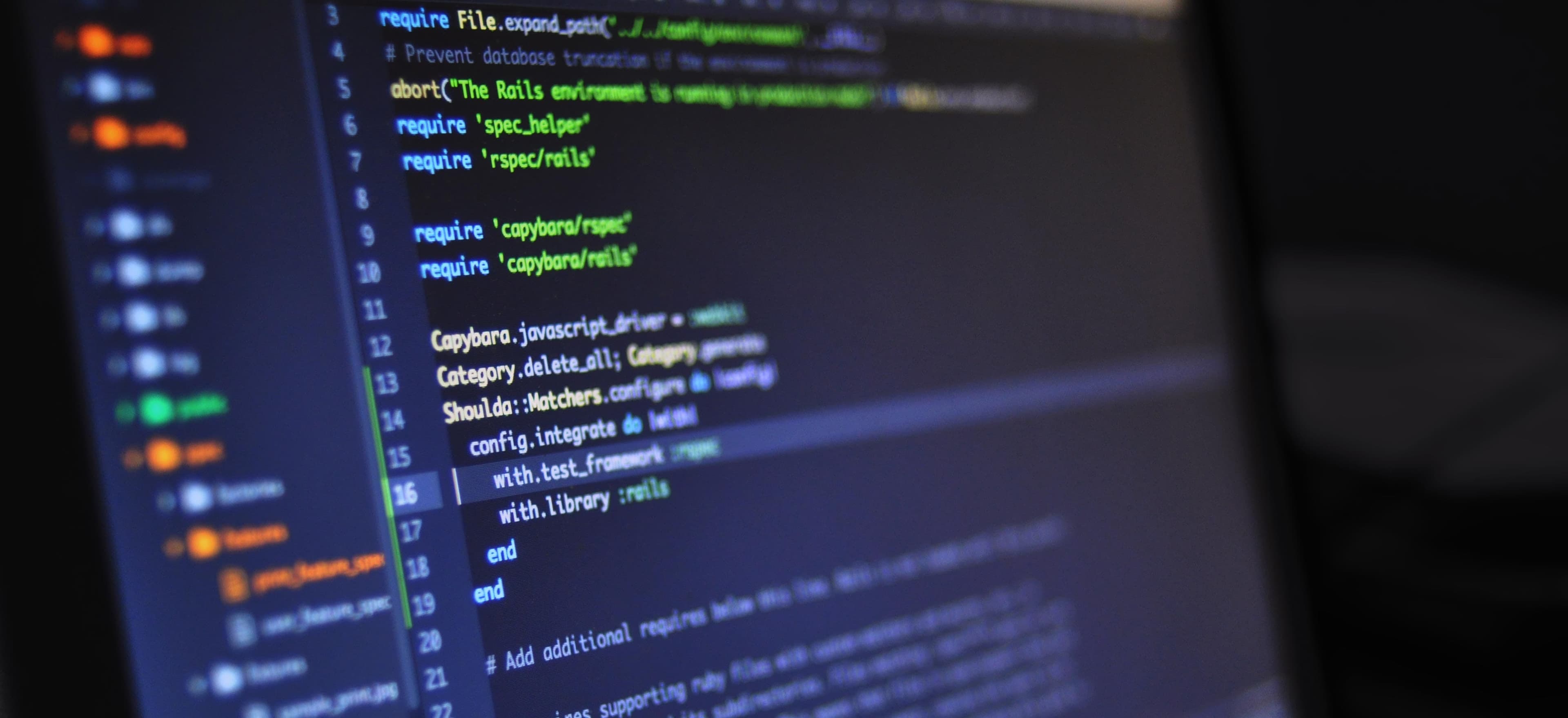
- Published on
Overcoming Java Learning Barriers for Beginners
Learning Java can feel like an uphill battle, especially for those just starting their programming journey. However, with the right strategies and resources, you can conquer these challenges and become proficient in this powerful programming language. In this blog post, we will explore common learning barriers and practical solutions to overcome them.
Understanding Java: The Foundation
Before we delve into the barriers, it is essential to understand why Java is a popular choice for both novices and seasoned programmers. Java is renowned for:
- Platform Independence: The "write once, run anywhere" (WORA) principle allows developers to run Java applications on any device equipped with the Java Virtual Machine (JVM).
- Object-Oriented: Java’s object-oriented nature encourages code reusability, making software maintenance easier.
- Rich API: With a wide range of libraries and frameworks, Java simplifies the development process.
For beginners, grasping these fundamental concepts will set the stage for further learning.
Common Barriers in Learning Java
1. Syntax Overwhelm
Many beginners find themselves overwhelmed by Java's syntax. Java is a statically typed language, which means that variable types must be explicitly declared. This strictness can be daunting.
Solution: Break It Down
Start with basic syntax and gradually build complexity. Here’s a simple example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Why this code? This code is the quintessential starting point for any Java programmer. It introduces you to the structure of a Java program, including classes and the main method.
2. Abstract Concepts
Concepts like inheritance, polymorphism, and encapsulation can seem abstract and confusing.
Solution: Relate to Real-World Examples
Consider inheritance like family trees. Just as children inherit traits from their parents, in Java, subclasses inherit attributes and methods from parent classes.
class Animal {
void eat() {
System.out.println("This animal eats food.");
}
}
class Dog extends Animal {
void bark() {
System.out.println("The dog barks.");
}
}
Why this code? Here, Dog
inherits from Animal
, implying it can perform actions defined in Animal
, such as eating, while also having its unique behavior of barking.
3. Abstraction Levels
Java supports various levels of abstraction which beginners may find perplexing. The language has built-in access modifiers: private, protected, public, and default, which determine the visibility of classes and their members.
Solution: Start Small, Gradual Expansion
Focus on understanding one access modifier at a time. For instance, use public to start and then explore others as your comfort grows.
public class Car {
private String color; // only accessible within Car class
public void setColor(String color) {
this.color = color;
}
public String getColor() {
return this.color;
}
}
Why this code? This code portrays encapsulation by restricting direct access to the class's attributes and emphasizes how to use getter and setter methods to manipulate data securely.
4. Lack of Hands-On Experience
Theory without practice can lead to frustration. The best way to understand Java is to code regularly.
Solution: Find Simple Projects
Start with manageable projects that excite you.
- Todo List Application: Build a command-line based Todo List app.
- Simple Calculator: Create a basic calculator to implement user input and logical operations.
import java.util.Scanner;
public class SimpleCalculator {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.print("Enter first number: ");
double num1 = scanner.nextDouble();
System.out.print("Enter second number: ");
double num2 = scanner.nextDouble();
System.out.println("Sum: " + (num1 + num2));
scanner.close();
}
}
Why this code? It introduces essential aspects of Java, such as user input and mathematical operations, in a simple, engaging way.
5. Debugging Challenges
Debugging can be intimidating for beginners. Typing errors, logical errors, and runtime exceptions can hinder progress.
Solution: Embrace Debugging Tools
Use Integrated Development Environments (IDEs) like IntelliJ IDEA or Eclipse, which come with debugging tools that simplify identifying issues.
Best Practices:
- Read error messages carefully; they often indicate where the problem lies.
- Utilize print statements to track variable values and program flow.
6. Community and Resources
Navigating Java's expansive ecosystem can feel isolating without proper guidance.
Solution: Engage with Communities
Join platforms like Stack Overflow, Reddit, or local programming meetups. Resources like Codecademy and Coursera offer structured learning paths.
7. Advanced Concepts
Once beginners grasp the basics, advanced topics like multithreading and Java APIs may appear daunting.
Solution: Build on Basics
Incorporate advanced topics into personal projects. Here’s a quick multithreading example:
class MyThread extends Thread {
public void run() {
System.out.println("Thread is running.");
}
}
public class ThreadExample {
public static void main(String[] args) {
MyThread thread = new MyThread();
thread.start();
}
}
Why this code? This simple example introduces multithreading, allowing you to run tasks simultaneously, showcasing one of Java’s elegant features used in high-performance applications.
8. Inconsistent Practice
Learning a programming language is most effective through consistent practice.
Solution: Set a Routine
Allocate a specific time daily or weekly for coding. Use challenges from websites like LeetCode or HackerRank to reinforce your skills.
Closing Remarks
Overcoming learning barriers in Java is a step-by-step process that requires determination and resourcefulness. By focusing on manageable tasks, utilizing community support, and consistently practicing, you can transform your Java journey into a fulfilling experience. Remember, every expert was once a beginner.
Further Reading
- The Complete Java Developer Course
- Oracle's Java Tutorials
- Java Programming: Solving Problems with Software
Let's Connect!
What barriers are you facing in your Java learning journey? Share in the comments below. Happy coding!
Checkout our other articles