Choosing Between SharedHashMap and Redis: The Key Differences
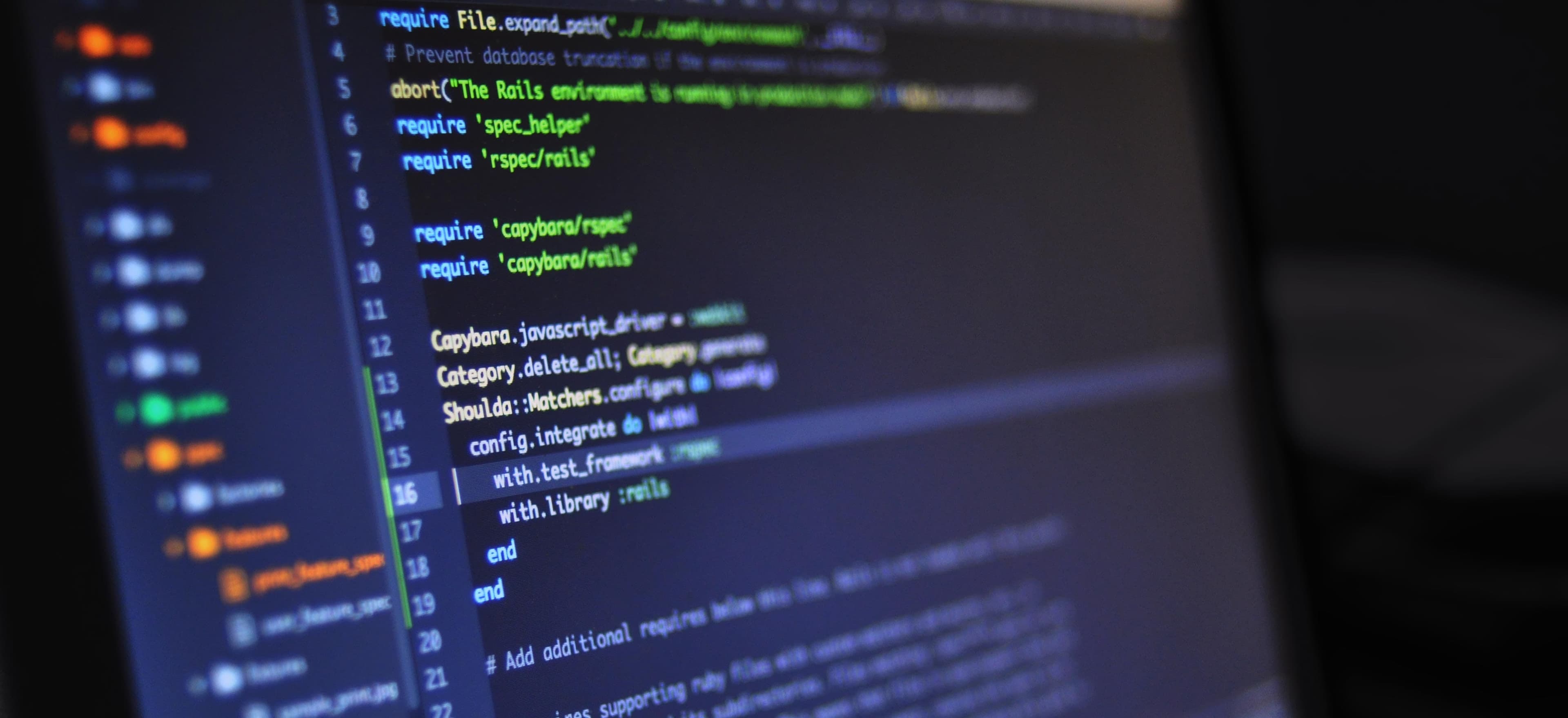
- Published on
Choosing Between SharedHashMap and Redis: The Key Differences
In today’s world of distributed computing, developers have a multitude of options for data storage and management. As applications grow and scale, using the right data structures and services becomes imperative. Among the popular choices are SharedHashMap
and Redis
. This blog post delves into the key differences between these two options, helping you navigate the complexities of data handling in your Java applications.
What is SharedHashMap?
SharedHashMap
is a concurrent and shared map implementation in Java. It is primarily designed for use in environments where multiple threads access the same data concurrently. If you want a simple in-memory solution and are working within a single JVM, SharedHashMap
is often the go-to choice.
Key Features of SharedHashMap
- Thread-Safe: Multiple threads can read/write without fear of data inconsistency.
- In-Memory Storage: Provides high-speed data access as all data is stored in memory.
- Simplistic: Straightforward API suitable for basic data storage needs.
Example Code Snippet for SharedHashMap
import java.util.concurrent.ConcurrentHashMap;
public class SharedHashMapExample {
private static ConcurrentHashMap<String, String> map = new ConcurrentHashMap<>();
public static void main(String[] args) {
// Adding elements
map.put("1", "One");
map.put("2", "Two");
// Retrieving an element
String result = map.get("1");
System.out.println("Retrieved: " + result); // Output: Retrieved: One
}
}
Why Use SharedHashMap?
If your application has a limited scope (single JVM, high concurrency), SharedHashMap
can lead to low latency and high throughput due to its in-memory nature. However, remember that its limitations become evident in distributed environments.
What is Redis?
Redis is an in-memory data structure store that can function as a database, cache, and message broker. Since it operates as a server, Redis is designed for high scalability and provides more than just simple key-value storage.
Key Features of Redis
- Persistence Options: Offers various persistence methods, allowing data to survive server restarts.
- Distributed Nature: Can be scaled horizontally to handle high volumes of requests across multiple servers.
- Data Structures: Supports rich data types (strings, hashes, sets, sorted sets, lists).
Example Code Snippet for Redis
To utilize Redis in a Java application, you can use the Jedis library. First, include Jedis in your Maven project:
<dependency>
<groupId>redis.clients</groupId>
<artifactId>jedis</artifactId>
<version>4.0.0</version>
</dependency>
Basic Usage
import redis.clients.jedis.Jedis;
public class RedisExample {
public static void main(String[] args) {
// Connecting to Redis server
Jedis jedis = new Jedis("localhost");
System.out.println("Connection to server successfully");
// Storing data
jedis.set("key", "value");
String value = jedis.get("key");
System.out.println("Stored value: " + value); // Output: Stored value: value
}
}
Why Use Redis?
Redis is an excellent choice for distributed systems needing high availability and persistence. Utilizing Redis is suitable for scenarios such as caching frequently accessed data or implementing data queues.
Key Differences Between SharedHashMap and Redis
Understanding the distinctions between SharedHashMap
and Redis
is crucial for making informed decisions about data management strategies in your projects. Let's explore these differences:
| Criteria | SharedHashMap | Redis | |-------------------------|-----------------------------|---------------------------------| | Storage Type | In-memory | In-memory with disk persistence | | Environment | Single JVM | Distributed system | | Performance | High for multi-thread access| High with persistence options | | Data Structures | Simple Key-Value | Advanced data structures | | Scalability | Limited to single server | Horizontal scaling possible | | Concurrency | Thread-safe | Client-driven concurrency |
Area of Usage
-
SharedHashMap is ideal for applications running in a single JVM where speed and simplicity are paramount. For instance, in a web application backend, storing temporary user session data could effectively use
SharedHashMap
. -
Redis, on the other hand, shines in a distributed architecture. When dealing with multiple instances or microservices, using Redis can help synchronize state across services or provide a centralized data store to reduce redundancy.
Pros and Cons
SharedHashMap
Pros
- Simple API and usage.
- Extremely fast due to in-memory nature.
- Automatic handling of multi-threading.
Cons
- Not suitable for distributed environments.
- Data loss upon JVM shutdown.
Redis
Pros
- High availability and persistence options.
- Supports various data structures.
- Works seamlessly in optimized distributed systems.
Cons
- Additional overhead managing a separate server instance.
- Network latency can impact speed compared to in-memory solutions.
Use Cases
When to Choose SharedHashMap
- You are building a prototype within a single application and need quick performance with concurrent access.
- You have an application that does not require data persistence across restarts.
When to Choose Redis
- You need a highly available solution that spans multiple servers or services.
- You require additional data structures beyond basic key-value pairs.
- Your application could benefit from caching mechanisms for performance enhancement.
My Closing Thoughts on the Matter
Choosing between SharedHashMap
and Redis depends on your application’s specific needs. If you are focusing on a single-threaded, in-memory solution, SharedHashMap
could be suitable. However, if scalability, distributed access, and persistence are essential, adopting Redis would significantly enhance your architecture.
As with most technology choices, understanding the requirements of your system, as well as the trade-offs involved, will help you make the best decision for your application. Happy coding!
Further Learning Resources
By understanding the webs of complexities surrounding these data management solutions, you are well-positioned to optimize your Java applications. Choose wisely!
Checkout our other articles