Optimizing MapReduce Performance in Apache Spark
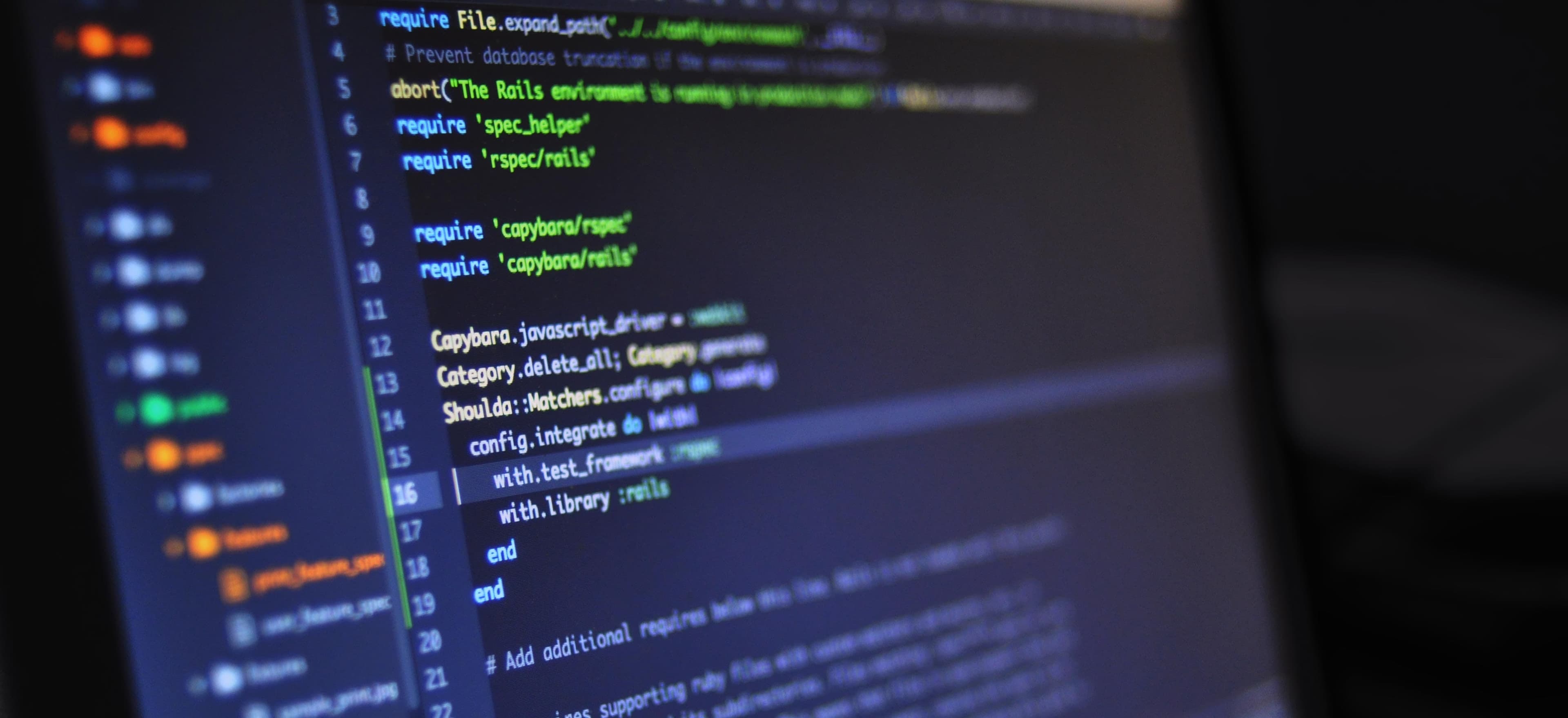
- Published on
Optimizing MapReduce Performance in Apache Spark
Apache Spark is widely acknowledged for its powerful data processing capabilities, particularly when it comes to handling large datasets. At its core, Spark operates on the MapReduce paradigm, which divides the task into smaller sub-tasks—namely Map and Reduce phases. While this simplifies data processing, it's essential for developers and data scientists to ensure that these operations are optimized for performance. In this blog post, we will discuss various techniques for optimizing MapReduce performance in Apache Spark, enriched with practical examples.
Understanding the MapReduce Paradigm
Before diving into optimization strategies, let's quickly recap the MapReduce process within Apache Spark.
- Map Phase: This phase takes input data, processes it, and produces intermediate key-value pairs.
- Shuffle Phase: The system redistributes the data based on the keys generated in the Map phase.
- Reduce Phase: The Reduce phase processes these intermediate key-value pairs to generate the final output.
The efficiency of each phase can dramatically impact the overall performance of Spark applications.
1. Efficient Data Serialization
Serialization refers to the process of converting an object into a byte stream. In Spark, efficient serialization can help improve performance by reducing memory usage and speeding up data transfer.
Use Kryo Serialization
By default, Spark uses Java serialization, which can be inefficient for large datasets. Switching to Kryo serialization can lead to significant performance improvements.
import org.apache.spark.SparkConf
val conf = new SparkConf()
.setAppName("KryoSerializationExample")
.set("spark.serializer", "org.apache.spark.serializer.KryoSerializer")
val sc = SparkContext.getOrCreate(conf)
Why Kryo?
Kryo is faster and more compact than Java serialization. Configuration is straightforward, and for complex object types, it offers better compression, thus optimizing memory usage.
Learn more about Spark Configuration
2. Partitioning Data Appropriately
Data partitioning plays a crucial role in the performance of MapReduce jobs in Apache Spark. Improper data partitioning can lead to data skew, where some partitions are overloaded.
Use the Right Number of Partitions
The default number of partitions in Spark can often be insufficient for very large datasets. A good rule of thumb is to set 2-4 partitions for each CPU core available.
val rdd = sc.textFile("data.txt", numPartitions = 4)
Consider using the repartition
or coalesce
methods judiciously for an optimal partition size.
val repartitionedRDD = rdd.repartition(8) // More partitions for better parallelism
Why does this matter?
Properly sized partitions ensure that each executor can perform computations efficiently without causing overhead due to excessive data being shuffled or uneven workloads.
3. Avoiding Shuffles
Shuffles are the most expensive operations in a Spark job. They occur when data is redistributed across different partitions or nodes.
Use Aggregate Functions
Instead of performing multiple transformations that require shuffling, consider using built-in aggregation functions.
val result = rdd.map(x => (x.split(",")(0), x.split(",")(1).toInt))
.reduceByKey((a, b) => a + b)
Why aggregate?
Aggregation functions are inherently optimized for performance and utilize less network bandwidth, reducing shuffle duration.
4. Caching Intermediate Results
If an RDD will be reused multiple times throughout the operations, caching it in memory can significantly speed up the execution.
Use Cache or Persist
val cachedRDD = rdd.cache()
Why cache?
Caching allows you to store intermediate results in memory, so when RDDs are accessed again, Spark can skip recalculating them, saving both time and resources.
Understanding Caching in Spark
5. Optimal Use of Broadcast Variables
Broadcast variables allow the programmer to keep a read-only variable cached on each machine instead of sending it to each task.
Use Broadcast Variables Wisely
val broadcastVar = sc.broadcast(Map("a" -> 1, "b" -> 2))
rdd.map(x => (x, broadcastVar.value.getOrElse(x, 0)))
Why use broadcast variables?
They prevent the overhead of sending the same data multiple times across the nodes, significantly reducing shuffle costs.
6. Monitoring And Tuning Performance
Utilizing the Spark UI and logging can provide insights into performance bottlenecks. By profiling your Spark applications, you can identify areas that require optimization.
- Spark UI: Access your Spark job's web UI to monitor job execution, stages, tasks, and storage.
- Event Logs: Enable event logs to capture detailed information during Spark application execution.
My Closing Thoughts on the Matter
Optimizing MapReduce performance in Apache Spark requires a thoughtful approach to data serialization, partitioning, aggregation, caching, and monitoring. By following these strategies, you can ensure that your Spark applications are not just effective but also efficient.
To further enhance your understanding and expertise, consider diving into the official Apache Spark Documentation, which provides extensive resources on various aspects of the framework.
By applying these best practices, you can take the performance of your Spark applications to new heights and perform data processing tasks effectively and efficiently. Happy coding!