Common Pitfalls When Using Stored Procedures with Hibernate
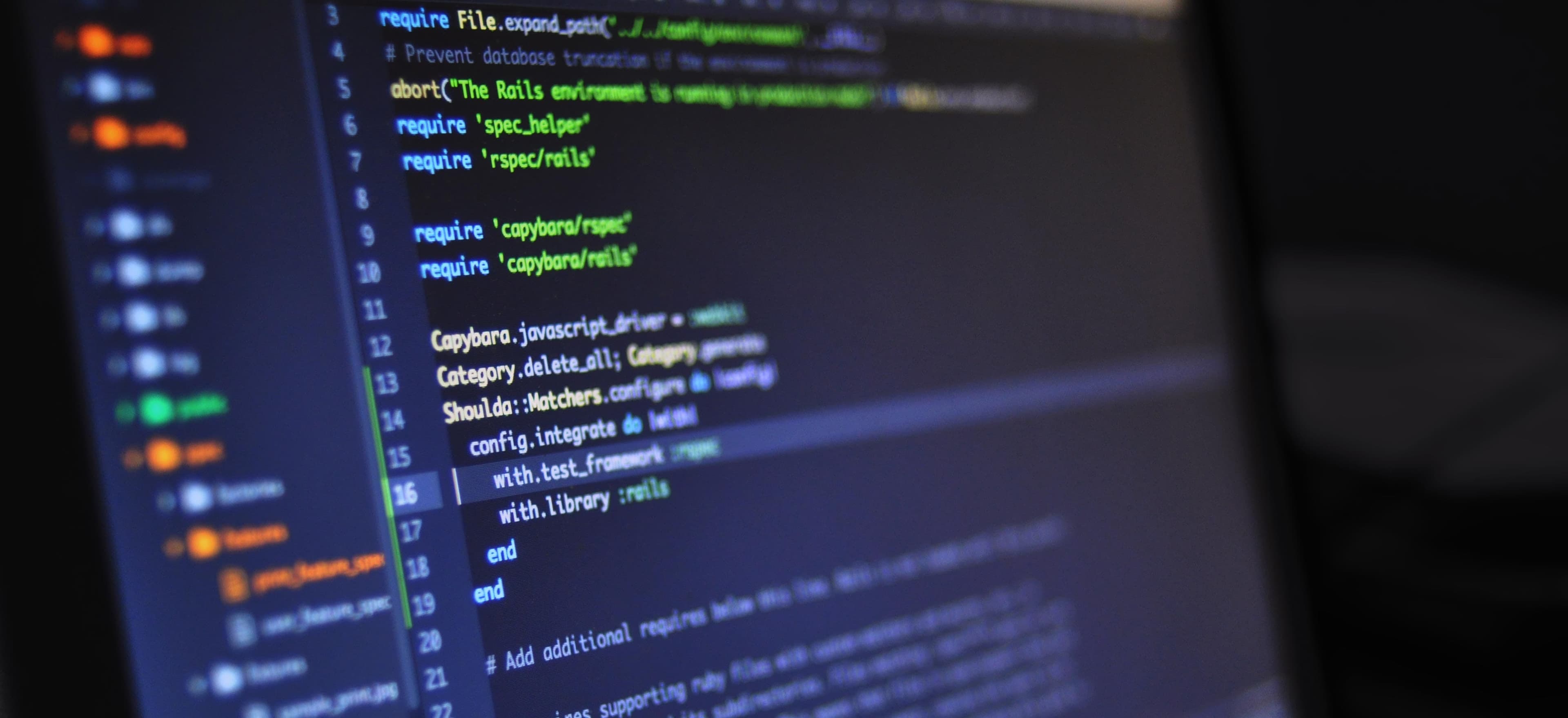
- Published on
Common Pitfalls When Using Stored Procedures with Hibernate
In the world of Java programming, Hibernate stands out as a powerful Object-Relational Mapping (ORM) tool that simplifies database interactions. However, integrating stored procedures with Hibernate can be a double-edged sword. While stored procedures can enhance performance and encapsulate complex business logic, misusing them can lead to a variety of pitfalls. In this blog post, we'll explore common mistakes developers make when using stored procedures with Hibernate and how to avoid them, supported by code snippets for clarity.
Understanding Stored Procedures
Before diving into pitfalls, let's clarify what stored procedures are. They are precompiled SQL statements stored in the database, which can be executed with parameters for better performance. Stored procedures can encapsulate complex logic and improve security by restricting direct access to database tables.
1. Not Using the Correct Configuration
Pitfall
One of the first mistakes developers encounter is incorrect configuration of Hibernate to call stored procedures. It is crucial to ensure that your Hibernate environment is correctly set up for JDBC calls to stored procedures.
Solution
To call a stored procedure, use the following code snippet:
Session session = sessionFactory.openSession();
Transaction tx = null;
try {
tx = session.beginTransaction();
CallableStatement call = session.doReturningWork(connection ->
connection.prepareCall("{call your_stored_procedure_name(?, ?)}"));
call.setInt(1, parameter1);
call.setString(2, parameter2);
call.execute();
tx.commit();
} catch (Exception e) {
if (tx != null) tx.rollback();
e.printStackTrace();
} finally {
session.close();
}
Commentary
This code snippet demonstrates how to set up a CallableStatement
to execute a stored procedure in a transaction. Using doReturningWork
helps directly interact with the JDBC Connection
. The use of transactions ensures that the database state remains consistent.
2. Ignoring Parameter Mapping
Pitfall
Another common issue arises from neglecting the proper mapping of input and output parameters in stored procedures. Hibernate requires explicit handling of these parameters, and failing to do so can lead to unexpected results or runtime exceptions.
Solution
You can handle parameters as shown below:
CallableStatement call = session.doReturningWork(connection ->
connection.prepareCall("{call your_stored_procedure_name(?, ?)}"));
call.setInt(1, parameter1); // Input Parameter
call.registerOutParameter(2, Types.VARCHAR); // Output Parameter
call.execute();
String output = call.getString(2);
Commentary
In this code, we clearly define both input and output parameters. Ignoring output parameters may lead to data loss, and ensuring proper mapping is essential for retrieving results correctly.
3. Overcomplicating Stored Procedures
Pitfall
Developers often tend to implement overly complex stored procedures that do too much. This complication can lead to maintenance difficulties, decreased performance (due to long execution times), and an overall poor user experience.
Solution
When designing stored procedures, adhere to the Single Responsibility Principle. Simplify stored procedures so that each one serves a single, specific operation. A clear, focused procedure is easier to maintain and debug. Here's a simple example:
CREATE PROCEDURE GetUserName(IN userId INT, OUT userName VARCHAR(255))
BEGIN
SELECT name INTO userName FROM users WHERE id = userId;
END
Commentary
In this SQL example, the procedure gets and returns a username based on the provided user ID—an operation that is simple and efficient. This is a perfect candidate for integration with Hibernate.
4. Failing to Handle Transactions Properly
Pitfall
A crucial mistake is neglecting transaction management when executing stored procedures. Improper handling can lead to data integrity issues, such as partial updates or incorrect database states.
Solution
Each stored procedure call should be wrapped in a transaction. Here’s a better way of handling it:
Transaction transaction = null;
try (Session session = sessionFactory.openSession()) {
transaction = session.beginTransaction();
// your stored procedure call
transaction.commit();
} catch (Exception e) {
if (transaction != null) transaction.rollback();
e.printStackTrace(); // log error
}
Commentary
Always surround your stored procedure calls with transaction handling. This guarantees that any procedural logic is treated atomically, ensuring that partial changes do not occur.
5. Lack of Error Handling and Logging
Pitfall
Ignoring error handling and logging can quickly lead to challenges when things go wrong. Without robust error handling, troubleshooting becomes a nightmare.
Solution
Use try-catch blocks extensively and leverage logging frameworks like SLF4J or Log4j for better clarity:
try {
// stored procedure logic
} catch (SQLException sqlEx) {
logger.error("SQL Exception: ", sqlEx);
} catch (HibernateException hibernateEx) {
logger.error("Hibernate Exception: ", hibernateEx);
} catch (Exception ex) {
logger.error("General Exception: ", ex);
}
Commentary
This approach enables you to catch specific exceptions, making your application resilient and easier to debug. Ensuring that logs provide helpful messages will boost your response time to errors.
6. Stored Procedure Result Mismatch
Pitfall
Another common issue is a mismatch between the expected results from stored procedures and what Hibernate is prepared to handle. If your stored procedure returns multiple rows or complex object types without corresponding mapping, it can cause runtime exceptions.
Solution
When dealing with multiple result sets, utilize Hibernate's @SqlResultSetMapping
or call the stored procedure in a suitable way. Here's an example of how to map results:
@Entity
@SqlResultSetMapping(name = "UserResult",
columns = { @ColumnResult(name = "name"), @ColumnResult(name = "email") })
public class User {
// fields, getters and setters
}
Commentary
By defining a result set mapping, you tell Hibernate how to map the output of your stored procedure to your entity. This improves your code's ability to handle complex results.
7. Lack of Testing and Performance Monitoring
Pitfall
Finally, neglecting to test stored procedures thoroughly and assessing their performance can lead to poor application performance and user dissatisfaction.
Solution
Testing stored procedures should be a part of your development cycle. Write unit tests to validate their behavior. Also, use tools like JProfiler, VisualVM, or database-specific performance monitoring tools to assess and improve performance.
Commentary
Regular performance checks allow you to enhance your procedures, ensuring they run efficiently even as your data grows.
Wrapping Up
Using stored procedures with Hibernate can yield speed and organizational advantages if done correctly. However, by being aware of common pitfalls, developers can enhance the quality and performance of their applications. Remember, simplicity, robust error handling, proper transaction management, and testing are crucial elements to effective stored procedure usage.
For additional information on Hibernate, you can refer to the official Hibernate documentation. Also, explore Java Persistence API Specification for more insights into JPA and stored procedure usage.
Incorporating these practices can lead to successful and efficient use of stored procedures in your Hibernate-based applications. Happy coding!